苹果CMS版本是V10,源码在他官方的github上可以获取到。
接入的个人支付系统是GOGO支付,插件主要为以下两个文件:
1.Gogozhifu.php(下单提交、回调通知代码)
<?php
namespace app\common\extend\pay;
class Gogozhifu
{
public $name = 'GoGo支付 - www.gogozhifu.com';
public $ver = '1.0';
public function submit($user, $order, $name)
{
$appId = trim($GLOBALS['config']['pay']['ggf']['appid']);
$appSecret = trim($GLOBALS['config']['pay']['ggf']['appsecret']);
$url = "https://www.gogozhifu.com/shop/api/createOrder";
$payId = $order['order_code'];
$param = 'go';
$type = $order['order_pay_method'];
$price = $order['order_price'];
$sign = md5($appId . $payId . $param . $type . $price. $appSecret);
$data = [
'payId' => $payId,
'param' => $param,
'type' => $type,
'price' => $price,
'sign' => $sign,
'isHtml' => 0,
'notifyUrl' => $GLOBALS['http_type'] . $_SERVER['HTTP_HOST'] . '/index.php/payment/notify/pay_type/ggf',//通知地址
'returnUrl' => $GLOBALS['http_type'] . $_SERVER['HTTP_HOST'] . '/payment/res/type/' . $order['order_type'],//跳转地址
];
return $this->ggPost($url, $data);
}
public function notify()
{
$data = input();
model('Order')->notify($data['payId'], 'ggf');
// 返回200
echo "success";
}
/**
* ggf使用的post
* @param $url
* @param $data
* @return mixed
*/
public function ggPost($url, $data)
{
$headerArray = [
"App-Id: " . trim($GLOBALS['config']['pay']['ggf']['appid']),
"App-Secret: " . trim($GLOBALS['config']['pay']['ggf']['appsecret'])
];
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headerArray);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$output = curl_exec($curl);
curl_close($curl);
//将返回的json对象解码成数组对象并返回
//$output = json_decode($output,true);
return $output;
}
}
2.Gogozhifu.html (这是cms后台支付模块配置里的填写参数页面,配置GOGO支付参数)
<div class="layui-tab-item">
<fieldset class="layui-elem-field layui-field-title" style="margin-top: 30px;">
<legend>GOGO支付设置 </legend>
</fieldset>
<div class="layui-form-item">
<label class="layui-form-label">APPID:</label>
<div class="layui-input-inline w400">
<input type="text" name="pay[ggf][appid]" placeholder="" value="{$config['pay']['ggf']['appid']}" class="layui-input">
</div>
</div>
<div class="layui-form-item">
<label class="layui-form-label">Secret:</label>
<div class="layui-input-inline w400">
<input type="text" name="pay[ggf][appsecret]" placeholder="" value="{$config['pay']['ggf']['appsecret']}" class="layui-input">
</div>
</div>
</div>
请把Gogozhifu.php放到/application/common/extend/pay/目录下。 请把Gogozhifu.html放到/application/admin/view/extend/pay/目录下。
调用支付接口所需的APPID和APPSecret请去官网注册即可获取,在商户后台首页(如下图)就可以直接复制,获取之后填写到自己的后台支付模块配置中就可以了。
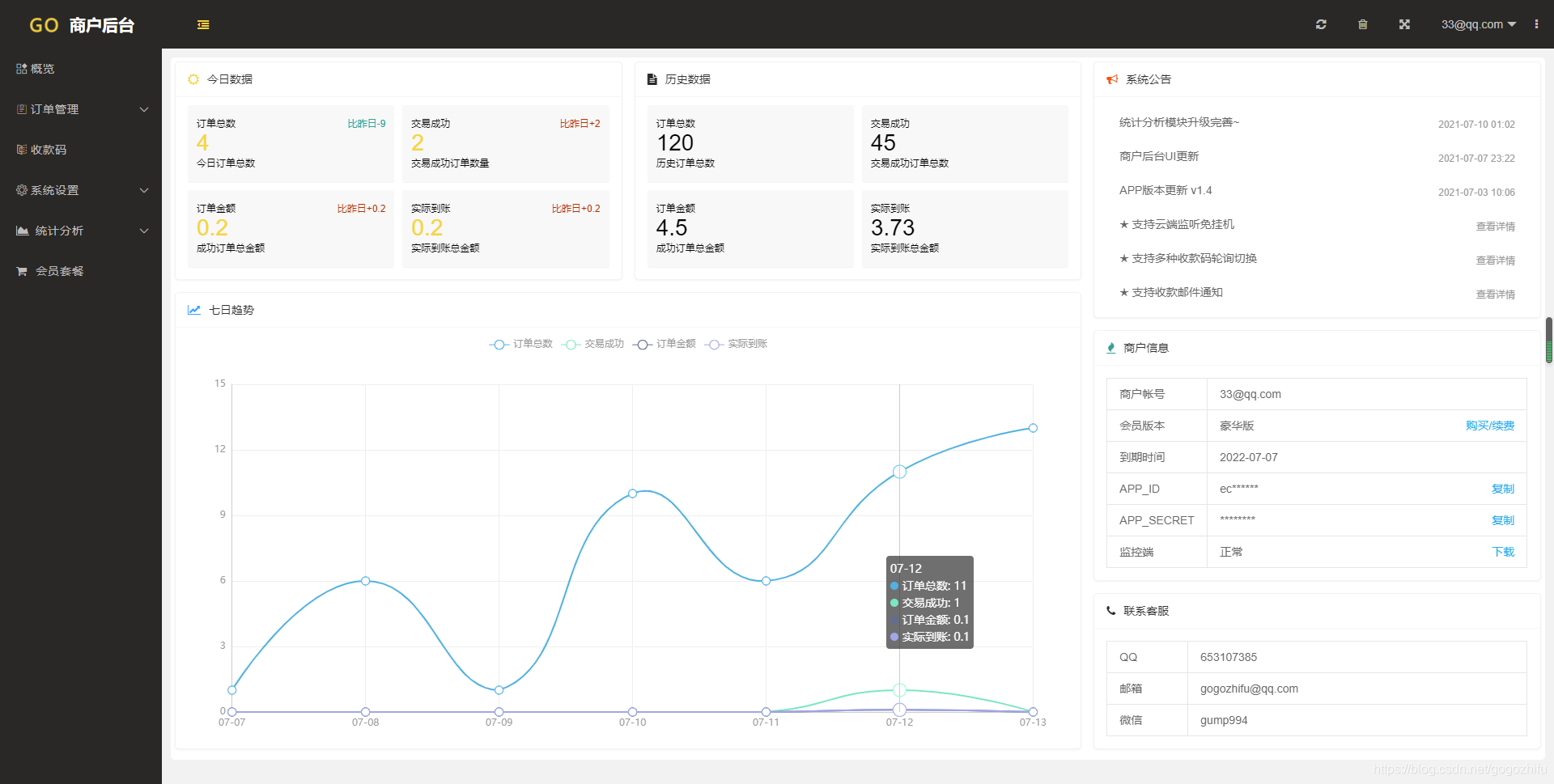
支付调用方式参考代码(比如我这里要充值积分,调起支付):
//充值提交
public function submit()
{
$param = input('post.');
$items = config('charge.combos');
$item = $items[$param['item_key']];
if (empty($item)) {
return $this->error("系统错误!要充值的套餐不存在!");
}
$config = config('ggcms');
$payType = $config['site']['pay_module'];
if (empty($payType)) {
return ['code' => 1004, 'msg' => '未开通在线支付模块'];
}
//记录支付流水
$data = [];
$data['user_id'] = $GLOBALS['user']['user_id'];
$data['order_code'] = 'CZ' . gan_get_uniqid_code();
$data['order_price'] = $item['price'];
$data['order_time'] = time();
$data['order_points'] = intval($item['points']);
$data['order_pay_type'] = $payType;
$data['order_pay_method'] = $param['pay_method']; // 1微信2支付宝
$orderRes = model('Order')->saveData($data);
if ($orderRes['code'] > 1) {
return $orderRes;
}
//支付模块选择
$cp = 'app\\common\\extend\\pay\\' . ucfirst($payType);
if (class_exists($cp)) {
$c = new $cp;
$paymentRes = $c->submit($this->user, $data, $item['item_name']);
//dump($paymentRes);die;
if ($payType == 'gogozhifu') {
$params = json_decode($paymentRes, true);
$redirectUrl = 'https://www.gogozhifu.com/shop/index/pay.html?orderId=' . $params['data']['orderId'];
return ['code' => 1, 'url' => $redirectUrl];
}
}
return ['code' => 5001, 'msg' => '未知错误'];
}
调用接口其他的字段和扩展参考https://www.gogozhifu.com/develop.html里面的API文档,可以根据开发文档进一步自定义开发自己想要的效果。
安全起见,回调方法里要做好签名校验,验证sign是否一致,代码参考如下:
<?php
ini_set("error_reporting","E_ALL & ~E_NOTICE");
$appId = "www.gogozhifu.com商户自己APPID"; //APPID
$appSecret = "www.gogozhifu.com商户自己APPSECRET"; //APPSECRET
$payId = $_GET['payId'];//商户订单号
$param = $_GET['param'];//创建订单的时候传入的参数
$type = $_GET['type'];//支付方式 :微信支付为1 支付宝支付为2
$price = $_GET['price'];//订单金额
$reallyPrice = $_GET['reallyPrice'];//实际支付金额
$sign = $_GET['sign'];//校验签名,计算方式 = md5(appId + payId + param + type + price + reallyPrice + appSecret)
//开始校验签名
$_sign = md5($appId . $payId . $param . $type . $price . $reallyPrice . $appSecret);
if ($_sign != $sign) {
echo "error_sign";//sign校验不通过
exit();
}
echo "success";
//继续业务流程
//echo "商户订单号:".$payId ."<br>自定义参数:". $param ."<br>支付方式:". $type ."<br>订单金额:". $price ."<br>实际支付金额:". $reallyPrice;
?>
有问题欢迎评论、私信交流~谢谢~
|