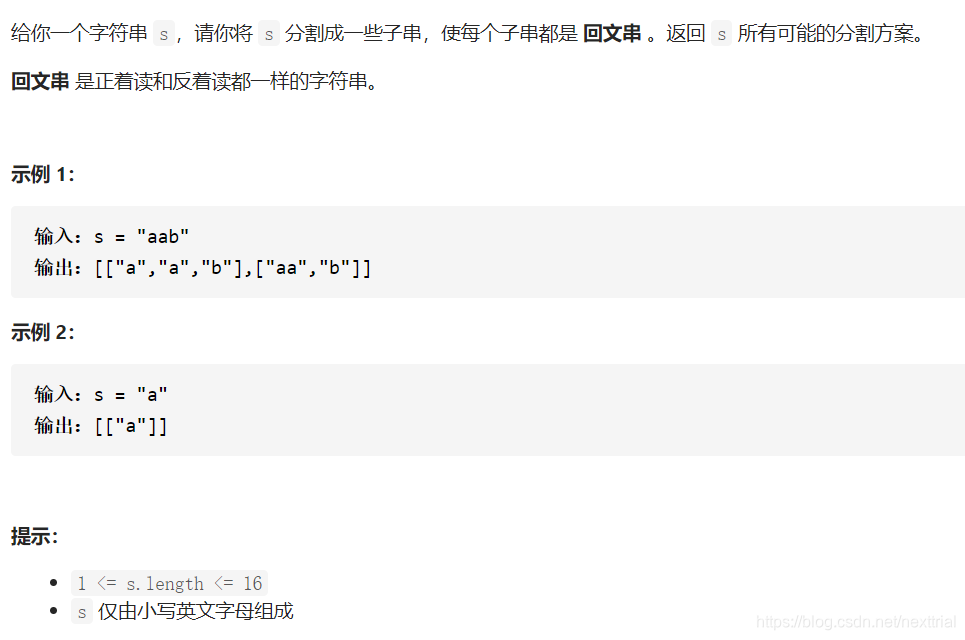
class Solution {
private $rlt = [];
private $ans = [];
private $dp = null;
function partition($s) {
$len = strlen($s);
$this->dp = array_fill(0, $len, array_fill(0, $len, true));
for ($j=1; $j<$len; $j++) {
for ($i=0; $i<$j; $i++) {
$this->dp[$i][$j] = $this->dp[$i+1][$j-1] && $s[$i] == $s[$j];
}
}
$this->dfs($s, 0);
return $this->rlt;
}
function dfs($s, $i) {
if ($i == strlen($s)) {
$this->rlt[] = $this->ans;
}
for ($j=$i; $j<strlen($s); $j++) {
if ($this->dp[$i][$j]) {
array_push($this->ans, substr($s, $i, $j-$i+1));
$this->dfs($s, $j+1);
array_pop($this->ans);
}
}
}
}
递归回溯+记忆化
class Solution {
private $f = null;
private $rlt = [];
private $ans = [];
function partition($s) {
$this->dfsV2($s, 0);
return $this->rlt;
}
function dfsV2($s, $i) {
if ($i == strlen($s)) {
$this->rlt[] = $this->ans;
}
for ($j=$i; $j<strlen($s); $j++) {
if ($this->isPalindrome($s, $i, $j)) {
array_push($this->ans, substr($s, $i, $j-$i+1));
$this->dfsV2($s, $j+1);
array_pop($this->ans);
}
}
}
function isPalindrome($s, $i, $j) {
if (isset($this->f[$i][$j])) {
return $this->f[$i][$j];
}
if ($i >= $j) {
$this->f[$i][$j] = true;
return true;
} else if ($s[$i] == $s[$j]) {
return $this->isPalindrome($s, $i+1, $j-1);
} else {
$this->f[$i][$j] = false;
return false;
}
}
}
|