目录结构
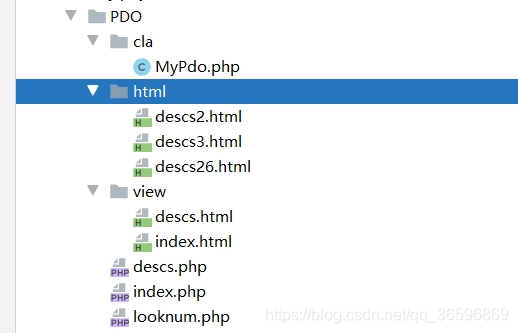
?
MyPdo.php类
<?php
class MyPdo
{
private static $_instance = null;
protected $dbName = '';
protected $dsn;
protected $dbh;
public function __construct($dbHost, $dbUser, $dbPasswd, $dbName, $dbCharset = 'utf8')
{
try {
$this->dsn = 'mysql:host=' . $dbHost . ';dbname=' . $dbName;
$this->dbh = new \PDO($this->dsn, $dbUser, $dbPasswd);
$this->dbh->setAttribute(\PDO::ATTR_EMULATE_PREPARES, false);
$this->dbh->setAttribute(\PDO::ATTR_ERRMODE, \PDO::ERRMODE_EXCEPTION);
$this->dbh->exec('SET character_set_connection=' . $dbCharset . ';SET character_set_client=' . $dbCharset . ';SET character_set_results=' . $dbCharset);
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public static function getInstance($dbHost, $dbUser, $dbPasswd, $dbName, $dbCharset = 'utf8mb4')
{
if (self::$_instance === null) {
self::$_instance = new self($dbHost, $dbUser, $dbPasswd, $dbName, $dbCharset);
}
return self::$_instance;
}
public function fetchAll($sql, $params = array())
{
try {
$stm = $this->dbh->prepare($sql);
if ($stm && $stm->execute($params)) {
return $stm->fetchAll(\PDO::FETCH_ASSOC);
}
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function fetchOne($sql, $params = array())
{
try {
$result = false;
$stm = $this->dbh->prepare($sql);
if ($stm && $stm->execute($params)) {
$result = $stm->fetch(\PDO::FETCH_ASSOC);
}
return $result;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function fetchColumn($sql, $params = array())
{
$result = '';
try {
$stm = $this->dbh->prepare($sql);
if ($stm && $stm->execute($params)) {
$result = $stm->fetchColumn();
}
return $result;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function insert($table, $params = array(), $returnLastId = true)
{
$_implode_field = '';
$fields = array_keys($params);
$_implode_field = implode(',', $fields);
$_implode_value = '';
foreach ($fields as $value) {
$_implode_value .= ':' . $value . ',';
}
$_implode_value = trim($_implode_value, ',');
$sql = 'INSERT INTO ' . $table . '(' . $_implode_field . ') VALUES (' . $_implode_value . ')';
try {
$stm = $this->dbh->prepare($sql);
$result = $stm->execute($params);
if ($returnLastId) {
$result = $this->dbh->lastInsertId();
}
return $result;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function update($sql)
{
try {
$stm = $this->dbh->exec($sql);
return $stm;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function delete($sql, $params = array())
{
try {
$stm = $this->dbh->prepare($sql);
$result = $stm->execute($params);
return $result;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
public function exec($sql, $params = array())
{
try {
$stm = $this->dbh->prepare($sql);
$result = $stm->execute($params);
return $result;
} catch (Exception $e) {
$this->outputError($e->getMessage());
}
}
private function outputError($strErrMsg)
{
throw new Exception("MySQL Error: " . $strErrMsg);
}
public function __destruct()
{
$this->dbh = null;
}
}
展示页面? index.php
<?php
include_once './cla/MyPdo.php';
$pdo = MyPdo::getInstance('localhost', 'root', 'root', 'test');
$sql = "select * from news";
$res = $pdo->fetchAll($sql);
include './view/index.html';
展示页面index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Layui</title>
<meta name="renderer" content="webkit">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1">
<link rel="stylesheet" href="//res.layui.com/layui/dist/css/layui.css" media="all">
<!-- 注意:如果你直接复制所有代码到本地,上述css路径需要改成你本地的 -->
</head>
<body>
<div class="layui-form">
<table class="layui-table">
<colgroup>
<col width="150">
<col width="150">
<col width="200">
<col>
</colgroup>
<thead>
<tr>
<th>ID</th>
<th>标题</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<?php
foreach($res as $v){
echo "<tr>";
echo "<td>$v[id]</td>";
echo "<td>$v[name]</td>";
echo "<td><a href='descs.php?id=$v[id]'>详情</a></td>";
echo "</tr>";
}
?>
</tbody>
</table>
</div>
<script src="//res.layui.com/layui/dist/layui.js" charset="utf-8"></script>
<script>
</script>
</body>
</html>
descs.php
<?php
$id = $_GET['id'];
include './cla/MyPdo.php';
$pdo = MyPdo::getInstance('localhost', 'root', 'root', 'test');
$sql = "select * from news where id = $id";
$res = $pdo->fetchOne($sql);
$html_static = './html/descs' . $id . '.html';
if (file_exists($html_static)) {
include $html_static;
}else{
if ($res['static'] == 1) {
//开启缓存
ob_start();
include './view/descs.html';
//存入文件后取出文件
$file = ob_get_contents();
//生成静态文件
file_put_contents($html_static, $file);
ob_flush();
} else {
include './view/descs.html';
}
}
descs.html文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://apps.bdimg.com/libs/jquery/2.1.4/jquery.min.js">
</script>
</head>
<body>
<input type="hidden" value="<?php echo $res['id']; ?>" class="ids">
<div>标题:<?php echo $res['name']; ?></div>
<div>内容:<?php echo $res['desc']; ?></div>
访问量:<span class="num"></span>
</body>
</html>
<script>
$.ajax({
url:"looknum.php",
data:{
id:$(".ids").val()
},
dataType:"json",
success:res=>{
console.log(res)
$(".num").html(res.num)
}
})
</script>
浏览量增加页面 looknum.php
<?php
$id = $_GET['id'];
include_once './cla/MyPdo.php';
$pdo = MyPdo::getInstance('localhost', 'root', 'root', 'test');
$sql = "select num from news where id = $id";
$res = $pdo->fetchOne($sql);
$sql1 = "update news set num= num+1 where id = $id";
$res1= $pdo->update($sql1);
echo json_encode($res);
|