安装tp6 和配置
1安装tp6:命令: composer create-project topthink/think tp
2 创建多应用模式安装扩展
composer require topthink/think-multi-app
3 快速生成模块应用 php think build demo 4 创建模块下的 控制器类php think make:controller index@Blog
wx login登录
1 控制器登录层
<?php
declare (strict_types = 1);
namespace app\api\controller;
use app\api\model\user as UserModel;
use app\api\server\Token as TokenServer;
use think\Request;
class Login
{
public function wxLogin(Request $request){
$code = $request->get('code');
$url = sprintf(config('wx.url'),config('wx.AppID'),config('wx.AppSecret'),$code);
$data = curlGet($url);
$user = UserModel::where('openid',$data['openid'])->find();
if (empty($user)){
$user = UserModel::create([
'openid'=>$data['openid']
]);
}
$token = (new TokenServer())->generateToken($user->id);
return json(['token'=>$token,'error_code'=>0,'msg'=>'登录成功']);
}
}
在模块下的common.php 文件里配置 系统自动生成的公共文件
<?php
function curlGet($url){
$headerArray =array("Content-type:application/json;","Accept:application/json");
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch,CURLOPT_HTTPHEADER,$headerArray);
$output = curl_exec($ch);
curl_close($ch);
$output = json_decode($output,true);
return $output;
}
token使用
1安装jwt命令composer require firebase/php-jwt 2.在模块下创建server服务层:下的Token控制器:
<?php
namespace app\api\server;
use Firebase\JWT\JWT;
class Token
{
protected $salt;
public function __construct()
{
$this->salt = config('jwt.salt');
}
function generateToken($uid)
{
$currentTime = time();
$data = array(
"iss" => 'keZuo',
"aud" => '',
"iat" => $currentTime,
"nbf" => $currentTime,
"exp" => $currentTime + 7200,
"data" => [
'uid' => $uid,
]
);
$token = JWT::encode($data, $this->salt, "HS256");
return $token;
}
public function chekToken($token)
{
$status=array("code"=>2);
try {
JWT::$leeway = 60;
$decoded = JWT::decode($token, $this->salt, array('HS256'));
$arr = (array)$decoded;
$res['code']=1;
$res['data']=$arr['data'];
return $res;
} catch(\Firebase\JWT\SignatureInvalidException $e) {
$status['msg']="签名不正确";
return $status;
}catch(\Firebase\JWT\BeforeValidException $e) {
$status['msg']="token失效";
return $status;
}catch(\Firebase\JWT\ExpiredException $e) {
$status['msg']="token失效";
return $status;
}catch(\Exception $e) {
$status['msg']="未知错误";
return $status;
}
}
}
创建config 的配置文件 jwt.php
<?php return [ 'salt'=>'a426bcc5f31e42527755afa13cc5f191' ]; wx.php
<?php
return[
'AppID' =>'wxf9c53e0a497cc457',
'AppSecret' => '47646ed2a1f74e056ab42cd6777cd500',
'url' => 'https://api.weixin.qq.com/sns/jscode2session?appid=%s&secret=%s&js_code=%s&grant_type=authorization_code'
];
中间件使用
1, 安装中间命令:
php think make:middleware Check
2middleware目录下的CheckToken.php文件下 配置
<?php
declare (strict_types = 1);
namespace app\middleware;
use app\api\server\Token as TokenServer;
use think\response\Json;
class CheckToken
{
public function handle($request, \Closure $next)
{
$token=$request->header('token');
$res=(new TokenServer())->chekToken($token);
if ($res['code']!=1){
return json(['error_code'=>999,'msg'=>$res['msg'],'data'=>''],400);
}
$request->uid=$res['data']->uid;
return $next($request);
}
}
路由:
Route::group(function (){
Route::get('slideshow','Slideshow/index');
Route::post('wxupload','Slideshow/upload');
Route::get('getFangAttr','Publish/getFangAttr');
Route::post('AddShared','Publish/AddShared');
})->middleware('check');
创建config 的配置文件 oss.php
<?php
return[
"accessKeyId"=>"LTAI5tGsyR7ppvCCFWw3Ykee",
"accessKeySecret"=>"MeQyMHXrKlnUK9nbtcUo4gm2i94p9j",
"bucket"=>"zhaochongbin",
"endpoint"=>"oss-cn-hangzhou.aliyuncs.com"
];
middleware.php
<?php
return [
'alias' => [
'check' => [app\middleware\CheckToken::class],
],
'priority' => [],
];
阿里云上传
1 先安装,我使用composer安装 在项目的根目录运行 composer require aliyuncs/oss-sdk-php
2.在模块下创建server服务层:下的Oss控制器:
<?php
namespace app\api\server;
use OSS\OssClient;
use OSS\Core\OssException;
class Oss
{
public function uploadFile($filePath)
{
$accessKeyId = config('oss.accessKeyId');
$accessKeySecret = config('oss.accessKeySecret');
$endpoint = config('oss.endpoint');
$bucket = config('oss.bucket');
$fileName = date('Y-m-d', time()) . '/' . md5(time() . rand(1111, 9999999)) . '.png';
try {
$ossClient = new OssClient($accessKeyId, $accessKeySecret, $endpoint);
$result = $ossClient->putObject($bucket, $fileName, file_get_contents($filePath));
} catch (OssException $e) {
print $e->getMessage();
}
return $result['info']['url'];
}
}
3,在控制器中;
<?php
declare (strict_types = 1);
namespace app\api\controller;
use app\api\model\Slideshow as SlideshowModel;
use app\api\server\Oss;
class Slideshow
{
public function upload()
{
$filePath = $_FILES['file']['tmp_name'];
$fileName = (new Oss())->uploadFile($filePath);
return json(['code'=>200,'msg'=>'上传成功','url'=>$fileName]);
}
public function index(){
$data = SlideshowModel::select()->toArray();
return json_encode($data);
}
}
小程序
存取Token
wx.setStorageSync('token', token)
var tonken2 = wx.getStorageSync('token')
console.log(tonken2);
带Token发送请求
wx.request({
url: 'http://www.lianxi.com/api/slideshow',
header:{
'token':wx.getStorageSync('token')
},
success:res=>{
this.setData({
slideshow:res.data
})
}
})
没了 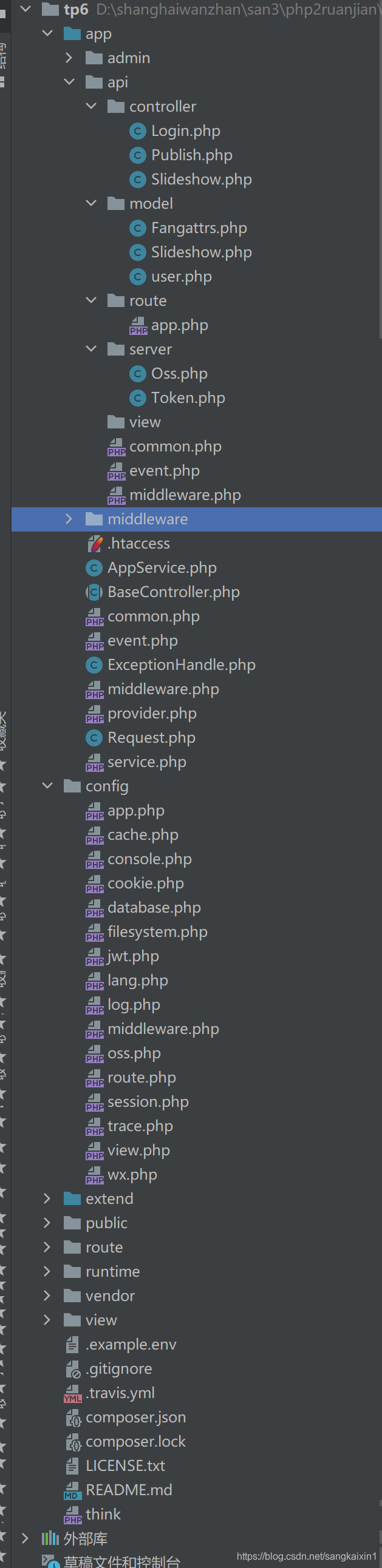
|