php-原型模式实现
概述
原型模式(Prototype)是用于创建重复的对象,同时又能保证性能。这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。
我们这里还是以前面几篇文章(php-工厂模式实现、php-抽象工厂模式实现、php-建造者模式实现)的摩托车制造厂为例。客户要求生产蓝色和红色的踏板摩托车,除颜色外其他属性都一样。这时候我们就可以考虑共用踏板车除车身颜色以外的所有生产环节,在软件工程中我们可以通过克隆(clone-本文采用了深拷贝)轻松实现,而不用每次都去new一个对象,毕竟在任何时候重复New一个对象对于系统的开销还是挺大的。因为本文主要用来解释原型模式实现,故对代码做了些许精简。
模式结构
以本文实例代码为参考
- MotorcycleProduce-摩托组装抽象类:建立摩托组装标准工艺
- MotorcycleScooterProduce-踏板摩托车组装者:负责踏板摩托车的组装工作
- MotocycleProduct:摩托车产品实体:最终形成产品并形成产品实体
UML图例
以本文实例代码为参考
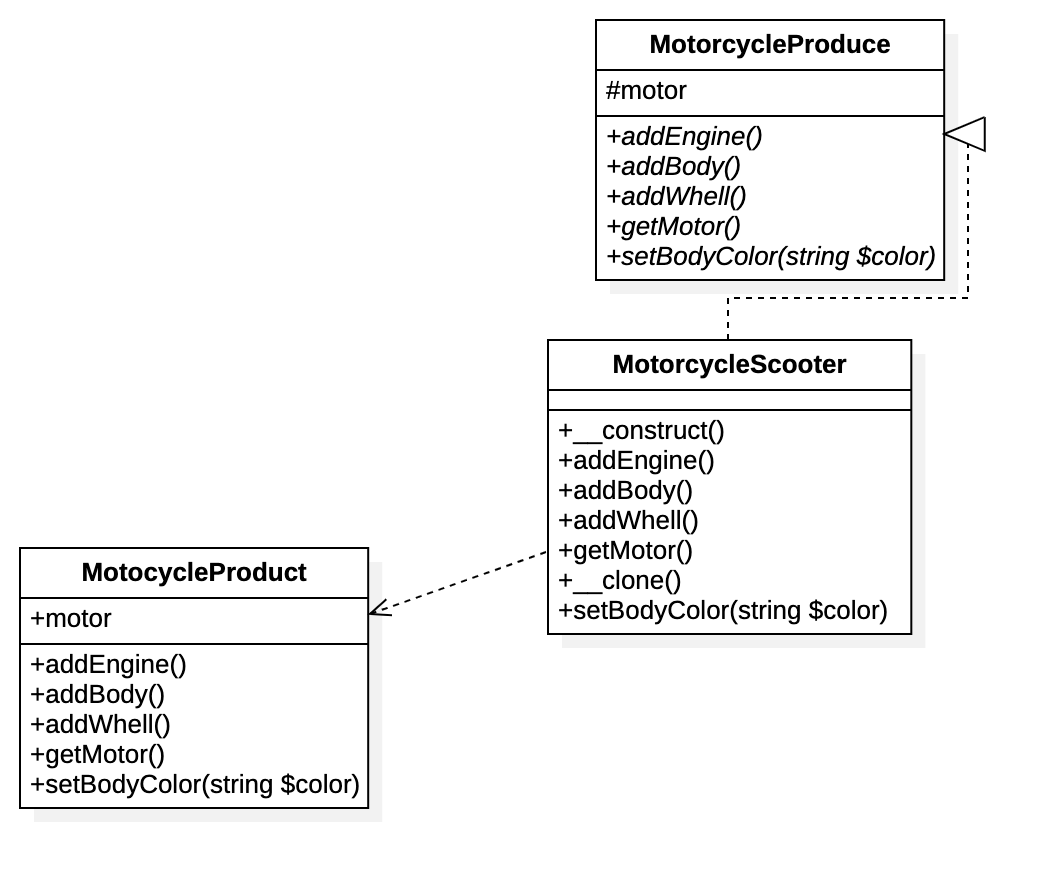
代码实例
<?php
namespace MotorcycleProduce;
abstract class MotorcycleProduce
{
protected $motor;
public abstract function addEngine();
public abstract function addBody();
public abstract function addWhell();
public abstract function setBodyColor(string $color);
public abstract function getMotor();
}
class MotocycleProduct{
private $motor = [
"engine"=>"",
"body"=>"",
"whell"=>"",
"bodyColor"=>"blue"
];
public function addEngine($engine){
$this->motor["engine"] = $engine;
}
public function addBody($body){
$this->motor["body"] = $body;
}
public function addWhell($whell){
$this->motor["whell"] = $whell;
}
public function setBodyColor($color){
$this->motor["bodyColor"] = $color;
}
public function getMotor(){
return $this->motor;
}
}
class motorcycleScooter extends MotorcycleProduce
{
public function __construct()
{
$this->motor = new MotocycleProduct();
}
public function __clone()
{
$this->motor = clone $this->motor;
}
public function addEngine()
{
$this->motor->addEngine("踏板摩托-发动机已装好");
}
public function addBody(){
$this->motor->addBody("踏板摩托-车身已装好");
}
public function addWhell(){
$this->motor->addWhell("踏板摩托-车轮已装好");
}
public function setBodyColor($color){
$this->motor->setBodyColor($color);
}
public function getMotor(){
return $this->motor;
}
}
$motorcycleScooter = new MotorcycleScooter;
$motorcycleScooter->addEngine();
$motorcycleScooter->addBody();
$motorcycleScooter->addWhell();
$motor = $motorcycleScooter->getMotor();
var_dump($motor);
echo "<br>";
$motorcycleScooterRed = clone $motorcycleScooter;
$motorcycleScooter->addEngine();
$motorcycleScooter->addBody();
$motorcycleScooter->addWhell();
$motorcycleScooterRed->setBodyColor("red");
$motor = $motorcycleScooterRed->getMotor();
var_dump($motor);
模式分析
可以看出clone是原型模式的核心,原型模式主要用来解决复杂系统中重复创建对象的问题。已规避重复创建对象所带来的高额系统消耗。
|