php-适配器模式实现
概述
适配器模式(Adapter) :将一个接口转换成客户希望的另一个接口,适配器模式使接口不兼容的那些类可以一起工作,其别名为包装器(Wrapper)。适配器模式既可以作为类结构型模式,也可以作为对象结构型模式。
我们这里还是以摩托车制造为例。客户要求生产蓝色和红色的踏板摩托车,除颜色外其他属性都一样。我们先前的工艺是把车身喷漆部分单独视作一个流程,现在考虑到流程优化尝试,我们想把车身喷漆这一流程合并到车身组装环节。但并不想破坏原来的流程,所以我们需要定制两套车身组装标准(新标准基于原有标准的拓展)。此时我们就需要利用适配器模式来实现。具体代码实现如下。
模式结构
类结构型模式
- MotorcycleProduce-摩托组装抽象类:建立摩托组装标准工艺 Target 目标类
- MotocycleProduct 摩托车产品本身
- motorcycleScooter 摩托车组装- Adapter 适配器类
- motorcycleScooterAdapter 摩托车组装-Adaptee 适配者类
对象结构型模式
- MotorcycleProduce-摩托组装抽象类:建立摩托组装标准工艺 Target 目标类
- MotocycleProduct 摩托车产品本身
- motorcycleScooter 摩托车组装- Adapter 适配器类
- motorcycleScooterAdapter 摩托车组装-Adaptee 适配者类
UML图例
类结构型模式
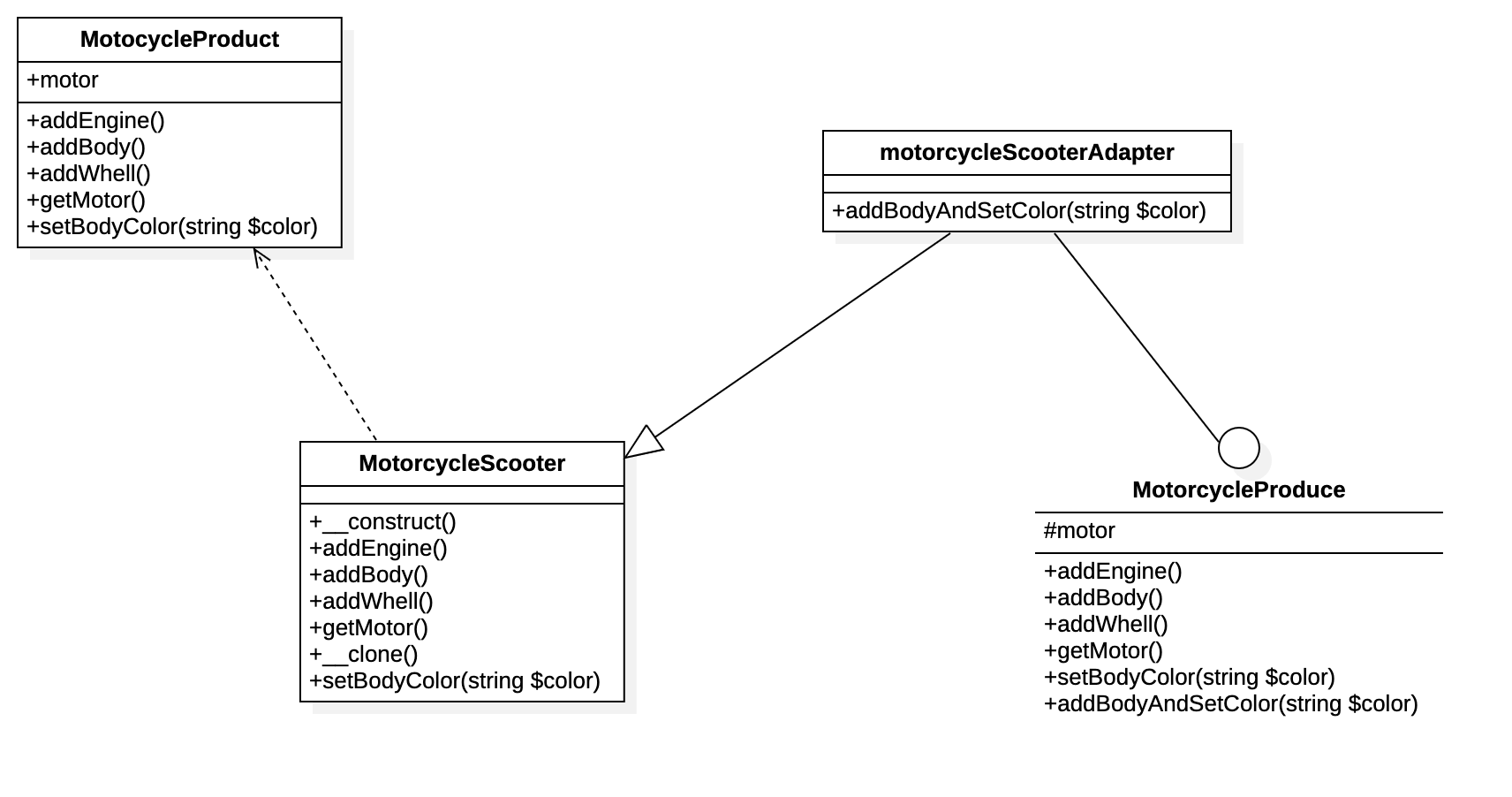
对象结构型模式
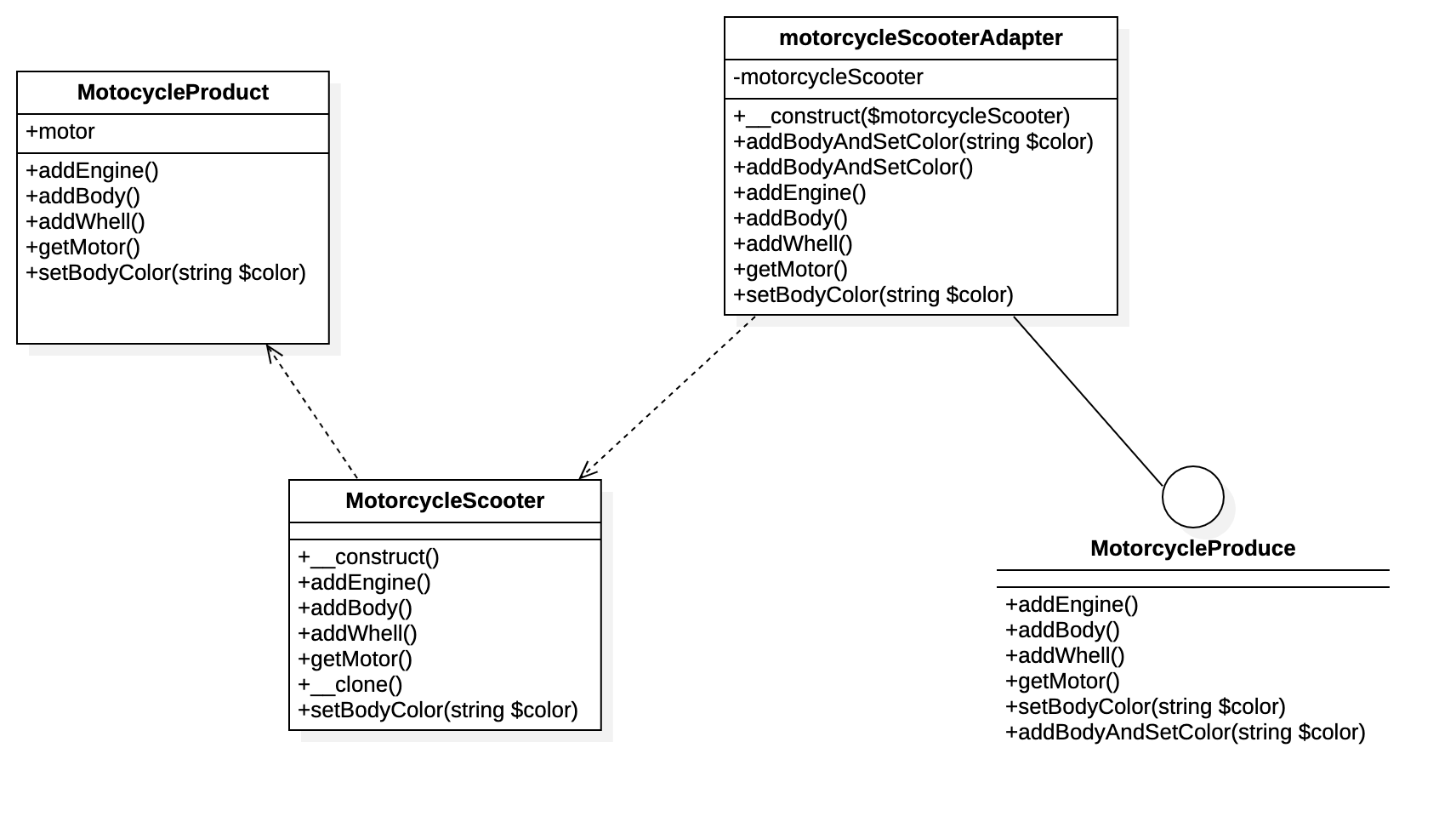
代码实例
类结构型模式
<?php
namespace Adapter;
interface MotorcycleProduce
{
public function addEngine();
public function addBody();
public function addBodyAndSetColor(string $color);
public function addWhell();
public function setBodyColor(string $color);
public function getMotor();
}
class MotocycleProduct{
private $motor = [
"engine"=>"",
"body"=>"",
"whell"=>"",
"bodyColor"=>"blue"
];
public function addEngine($engine){
$this->motor["engine"] = $engine;
}
public function addBody($body){
$this->motor["body"] = $body;
}
public function addWhell($whell){
$this->motor["whell"] = $whell;
}
public function setBodyColor(string $color){
$this->motor["bodyColor"] = $color;
}
public function getMotor(){
return $this->motor;
}
}
class motorcycleScooter
{
protected $motor;
public function __construct()
{
$this->motor = new MotocycleProduct();
}
public function addEngine()
{
$this->motor->addEngine("踏板摩托-发动机已装好");
}
public function addBody(){
$this->motor->addBody("踏板摩托-车身已装好");
}
public function addWhell(){
$this->motor->addWhell("踏板摩托-车轮已装好");
}
public function setBodyColor($color){
$this->motor->setBodyColor($color);
}
public function getMotor(){
return $this->motor;
}
}
class motorcycleScooterAdapter extends motorcycleScooter implements MotorcycleProduce{
public function addBodyAndSetColor($color){
$this->addBody();
$this->setBodyColor("green");
}
}
$motorcycleScooter = new motorcycleScooterAdapter;
$motorcycleScooter->addEngine();
$motorcycleScooter->addBody();
$motorcycleScooter->addWhell();
$motorcycleScooter->setBodyColor("red");
$motor = $motorcycleScooter->getMotor();
var_dump($motor);
echo "<br>";
$motorcycleScooter = new motorcycleScooterAdapter;
$motorcycleScooter->addEngine();
$motorcycleScooter->addBodyAndSetColor("green");
$motorcycleScooter->addWhell();
$motor = $motorcycleScooter->getMotor();
var_dump($motor);
对象结构型模式
<?php
namespace AdapterObj;
interface MotorcycleProduce
{
public function addEngine();
public function addBody();
public function addBodyAndSetColor(string $color);
public function addWhell();
public function setBodyColor(string $color);
public function getMotor();
}
class MotocycleProduct{
private $motor = [
"engine"=>"",
"body"=>"",
"whell"=>"",
"bodyColor"=>"blue"
];
public function addEngine($engine){
$this->motor["engine"] = $engine;
}
public function addBody($body){
$this->motor["body"] = $body;
}
public function addWhell($whell){
$this->motor["whell"] = $whell;
}
public function setBodyColor(string $color){
$this->motor["bodyColor"] = $color;
}
public function getMotor(){
return $this->motor;
}
}
class motorcycleScooter
{
protected $motor;
public function __construct()
{
$this->motor = new MotocycleProduct();
}
public function addEngine()
{
$this->motor->addEngine("踏板摩托-发动机已装好");
}
public function addBody(){
$this->motor->addBody("踏板摩托-车身已装好");
}
public function addWhell(){
$this->motor->addWhell("踏板摩托-车轮已装好");
}
public function setBodyColor($color){
$this->motor->setBodyColor($color);
}
public function getMotor(){
return $this->motor;
}
}
class motorcycleScooterAdapter implements MotorcycleProduce{
private $motorcycleScooter;
public function __construct($motorcycleScooter){
$this->motorcycleScooter = $motorcycleScooter;
}
public function addBodyAndSetColor($color){
$this->motorcycleScooter->addBody();
$this->motorcycleScooter->setBodyColor($color);
}
public function addBody(){
$this->motorcycleScooter->addBody();
}
public function addEngine(){
$this->motorcycleScooter->addEngine();
}
public function addWhell(){
$this->motorcycleScooter->addWhell();
}
public function setBodyColor(string $color){
$this->motorcycleScooter->setBodyColor($color);
}
public function getMotor(){
return $this->motorcycleScooter->getMotor();
}
}
$motorcycleScooter = new motorcycleScooterAdapter(new motorcycleScooter);
$motorcycleScooter->addEngine();
$motorcycleScooter->addBody();
$motorcycleScooter->addWhell();
$motorcycleScooter->setBodyColor("red");
$motor = $motorcycleScooter->getMotor();
var_dump($motor);
echo "<br>";
$motorcycleScooter = new motorcycleScooterAdapter(new motorcycleScooter);
$motorcycleScooter->addEngine();
$motorcycleScooter->addBodyAndSetColor("green");
$motorcycleScooter->addWhell();
$motor = $motorcycleScooter->getMotor();
var_dump($motor);
模式分析
适配器模式(Adapter)通常适用于以下场景。
- 以前开发的系统存在满足新系统功能需求的类,但其接口同新系统的接口不一致。
- 使用第三方提供的组件,但组件接口定义和自己要求的接口定义不同。
|