Qt MVC model 加载 大量数据的性能优化
自定义 canFetchMore() 、fetchMore()
canFetchMore() 函数的作用是:检查父节点是否有更多可用的数据,并相应地返回true或false。fetchMore() 函数的作用是:根据指定的父对象获取数据。例如,可以在涉及增量数据的数据库查询中组合这两个函数,以填充QAbstractItemModel。我们重新实现canFetchMore()来指示是否有更多的数据需要获取,并根据需要fetchMore()来填充模型。 例如动态填充树模型,当树模型中的一个分支展开时,我们将重新实现。 如果fetchMore()的重新实现向模型中添加了行,则需要调用beginInsertRows()和endInsertRows()。同样,canFetchMore()和fetchMore()都必须被重新实现。
filelistmodel.h
#ifndef FILELISTMODEL_H
#define FILELISTMODEL_H
#include <QAbstractListModel>
#include <QList>
#include <QStringList>
class FileListModel : public QAbstractListModel
{
Q_OBJECT
public:
FileListModel(QObject *parent = 0);
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
signals:
void numberPopulated(int number);
public slots:
void setDirPath(const QString &path);
void setPAGE_ITEM_COUNT(int value);
protected:
bool canFetchMore(const QModelIndex &parent) const override;
void fetchMore(const QModelIndex &parent) override;
private:
QStringList fileList;
int fileCount;
int PAGE_ITEM_COUNT;
};
#endif
filelistmodel.cpp
#include "filelistmodel.h"
#include <QApplication>
#include <QBrush>
#include <QDir>
#include <QPalette>
FileListModel::FileListModel(QObject *parent)
: QAbstractListModel(parent)
,PAGE_ITEM_COUNT(5)
{
}
int FileListModel::rowCount(const QModelIndex & ) const
{
return fileCount;
}
QVariant FileListModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
if (index.row() >= fileList.size() || index.row() < 0)
return QVariant();
if (role == Qt::DisplayRole) {
return fileList.at(index.row());
} else if (role == Qt::BackgroundRole) {
int batch = (index.row() / 100) % 2;
if (batch == 0)
return qApp->palette().base();
else
return qApp->palette().alternateBase();
}
return QVariant();
}
bool FileListModel::canFetchMore(const QModelIndex & ) const
{
if (fileCount < fileList.size())
return true;
else
return false;
}
void FileListModel::fetchMore(const QModelIndex & )
{
int remainder = fileList.size() - fileCount;
int itemsToFetch = qMin(PAGE_ITEM_COUNT, remainder);
if (itemsToFetch <= 0)
return;
beginInsertRows(QModelIndex(), fileCount, fileCount+itemsToFetch-1);
fileCount += itemsToFetch;
endInsertRows();
emit numberPopulated(itemsToFetch);
}
void FileListModel::setPAGE_ITEM_COUNT(int value)
{
PAGE_ITEM_COUNT = value;
}
void FileListModel::setDirPath(const QString &path)
{
QDir dir(path);
beginResetModel();
fileList = dir.entryList();
fileCount = 0;
endResetModel();
}
使用
FileListModel *model = new FileListModel(this);
model->setDirPath(QLibraryInfo::location(QLibraryInfo::PrefixPath));
QLabel *lb = new QLabel(tr("Fetch Max Count:"));
QSpinBox *sbMaxCount = new QSpinBox();
QLabel *label = new QLabel(tr("&Directory:"));
QLineEdit *lineEdit = new QLineEdit;
label->setBuddy(lineEdit);
QListView *view = new QListView;
view->setModel(model);
logViewer = new QTextBrowser;
logViewer->setSizePolicy(QSizePolicy(QSizePolicy::Preferred, QSizePolicy::Preferred));
connect(sbMaxCount, QOverload<int>::of(&QSpinBox::valueChanged),
model, &FileListModel::setPAGE_ITEM_COUNT);
sbMaxCount->setValue(3);
connect(lineEdit, &QLineEdit::textChanged,
model, &FileListModel::setDirPath);
connect(lineEdit, &QLineEdit::textChanged,
logViewer, &QTextEdit::clear);
connect(model, &FileListModel::numberPopulated,
this, [&](int number)
{
logViewer->append(tr("%1 items added.").arg(number));
});
QGridLayout *layout = new QGridLayout;
layout->addWidget(lb,0,0);
layout->addWidget(sbMaxCount,0,1);
layout->addWidget(label, 1, 0);
layout->addWidget(lineEdit, 1, 1);
layout->addWidget(view, 2, 0, 1, 2);
layout->addWidget(logViewer, 3, 0, 1, 2);
setLayout(layout);
setWindowTitle(tr("Fetch More Example"));
说明
当滑动滚动条,模型还没完全加载数据,继续加载显示 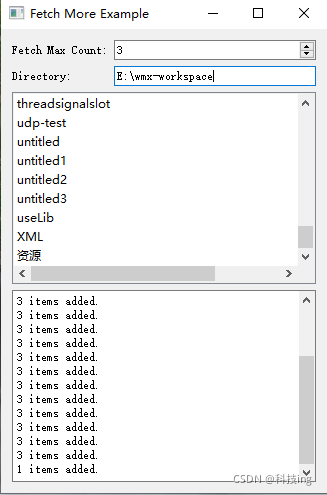
|