# 需求背景
1. 后台权限盘查,需要导出后台所有用户的所属角色和所拥有的权限。
# 导出数据格式预览图
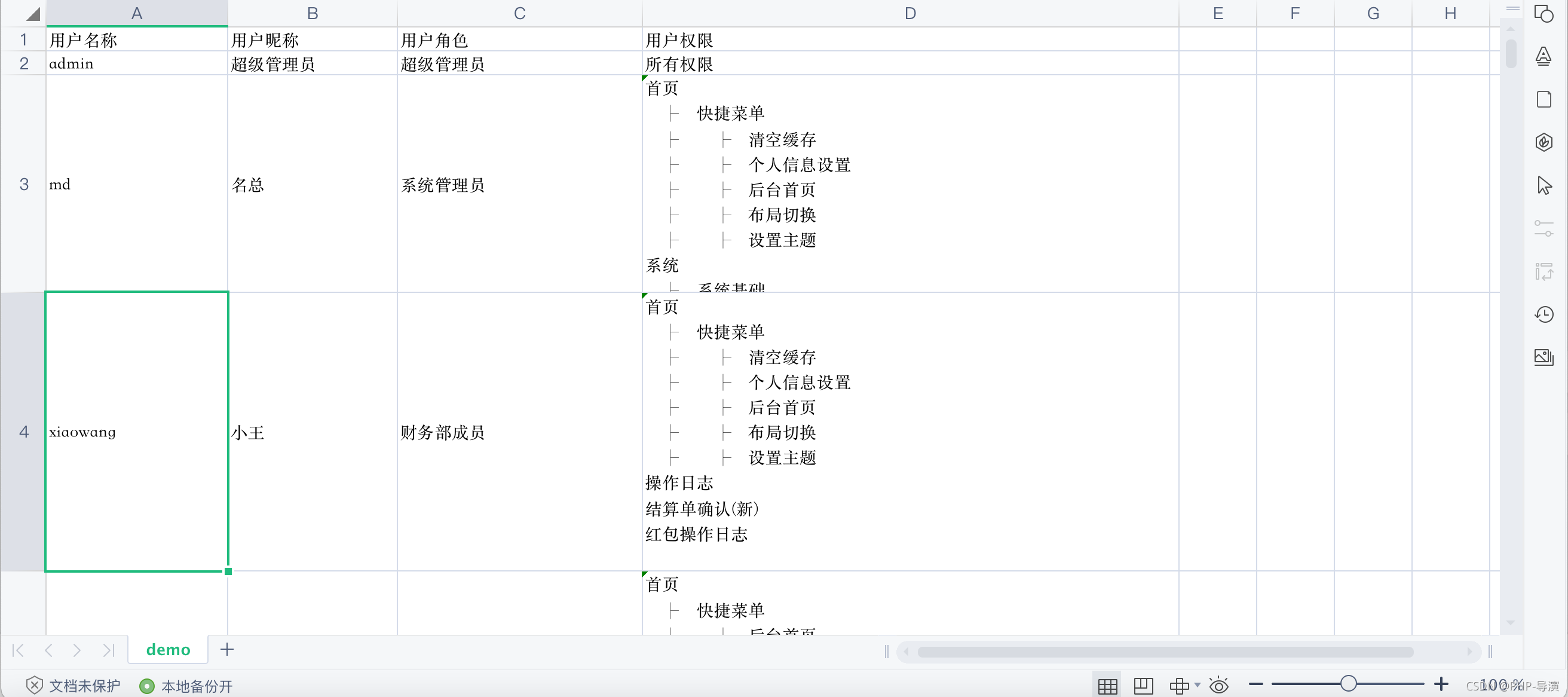
?详情展示:
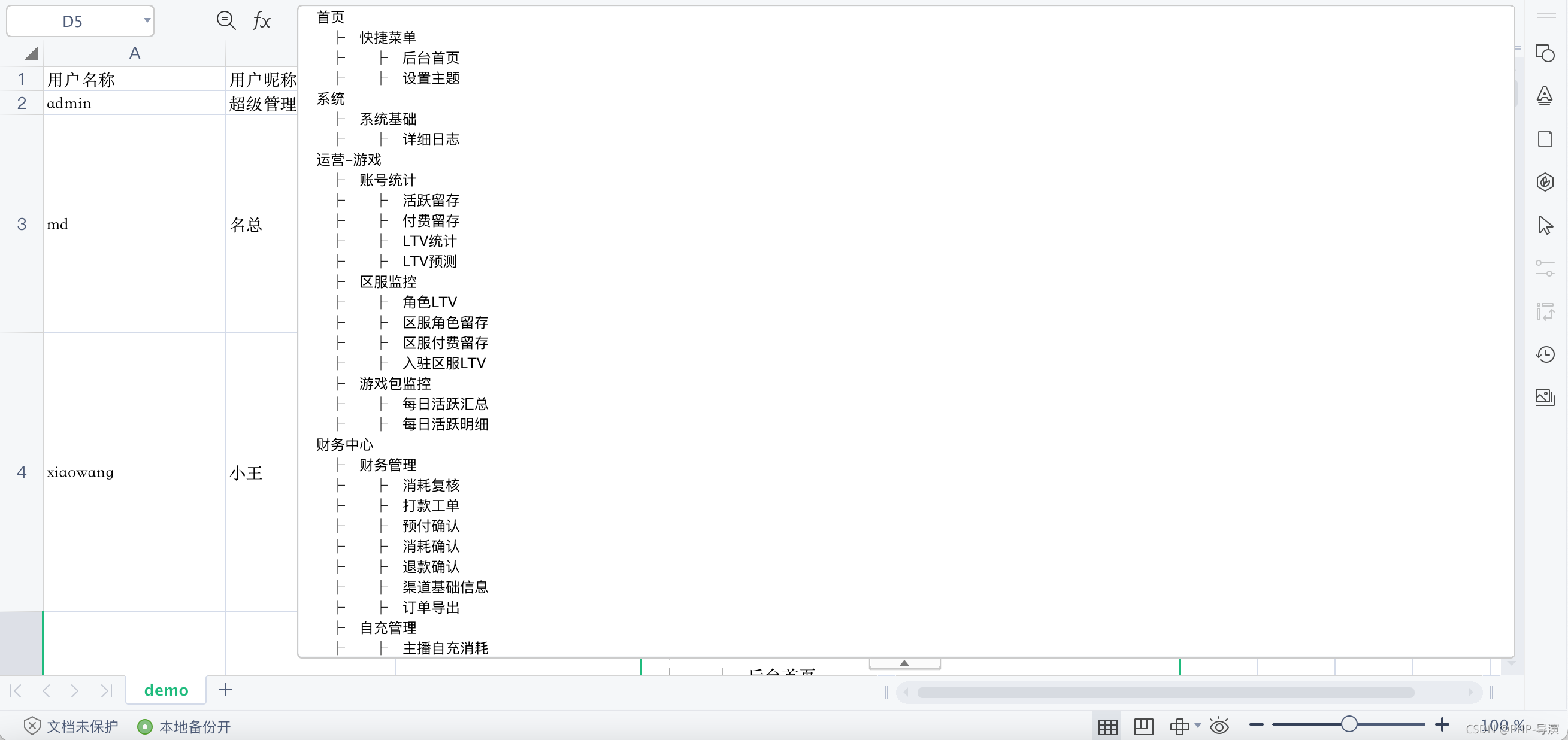
?# 需求分析
1. 需要拿到所有用户
2. 拿到用户所属角色权限
3. 拿到角色,对应的菜单权限
/*
* 导出用户角色权限信息
* */
public function export(){
$userList = Db::table('system_user')->select();
foreach ($userList as &$user){
$role = Db::table('system_role')->where('id',$user['role_id'])->find();
$user['role'] = $role['name'];
if (empty($role['auth'])){
$permission = "所有权限";
}else{
$treeMeun = Db::table('system_menu')->whereIn('id',json_decode($role['auth'], true))->field('id,pid,title')->select();
$permission = "";
foreach (Data::arr2table(Data::arr2tree($treeMeun)) as $item) $permission .= $item['spl'].$item['title']."\n";
}
$user['permission'] = $permission;
}
Csv::header("demo.csv",["用户名称","用户昵称","用户角色","用户权限"]);
Csv::body($userList,['username','nick','role','permission']);
}
#?一维数据数组生成数据树
/**
* 一维数据数组生成数据树
* @param array $list 数据列表
* @param string $id ID Key
* @param string $pid 父ID Key
* @param string $path
* @param string $ppath
* @return array
*/
public static function arr2table(array $list, $id = 'id', $pid = 'pid', $path = 'path', $ppath = '')
{
$tree = [];
foreach (self::arr2tree($list, $id, $pid) as $attr) {
$attr[$path] = "{$ppath}-{$attr[$id]}";
$attr['sub'] = isset($attr['sub']) ? $attr['sub'] : [];
$attr['spt'] = substr_count($ppath, '-');
$attr['spl'] = str_repeat(" ├ ", $attr['spt']);
$sub = $attr['sub'];
unset($attr['sub']);
$tree[] = $attr;
if (!empty($sub)) $tree = array_merge($tree, self::arr2table($sub, $id, $pid, $path, $attr[$path]));
}
return $tree;
}
#?一维数据数组生成数据树
/**
* 一维数据数组生成数据树
* @param array $list 数据列表
* @param string $id 父ID Key
* @param string $pid ID Key
* @param string $son 定义子数据Key
* @return array
*/
public static function arr2tree($list, $id = 'id', $pid = 'pid', $son = 'sub')
{
list($tree, $map) = [[], []];
foreach ($list as $item) $map[$item[$id]] = $item;
foreach ($list as $item) {
if (isset($item[$pid]) && isset($map[$item[$pid]])) {
$map[$item[$pid]][$son][] = &$map[$item[$id]];
} else {
$tree[] = &$map[$item[$id]];
}
}
unset($map);
return $tree;
}
#?Csv 导出类
/**
* 写入CSV文件头部
* @param string $filename 导出文件
* @param array $headers CSV 头部(一级数组)
*/
public static function header($filename, array $headers)
{
header('Content-Type: application/octet-stream');
header("Content-Disposition: attachment; filename=" . iconv('UTF-8', 'GB2312//IGNORE', $filename));
$handle = fopen('php://output', 'w');
foreach ($headers as $key => $value) $headers[$key] = iconv("UTF-8", "GB2312//IGNORE", $value);
fputcsv($handle, $headers);
if (is_resource($handle)) fclose($handle);
}
/**
* 写入CSV文件内容
* @param array $list 数据列表(二维数组或多维数组)
* @param array $rules 数据规则(一维数组)
*/
public static function body(array $list, array $rules)
{
$handle = fopen('php://output', 'w');
foreach ($list as $data) {
$rows = [];
foreach ($rules as $rule) $rows[] = self::parseKeyDotValue($data, $rule);
fputcsv($handle, $rows);
}
if (is_resource($handle)) fclose($handle);
}
/**
* 根据数组key查询(可带点规则)
* @param array $data 数据
* @param string $rule 规则,如: order.order_no
* @return mixed
*/
public static function parseKeyDotValue(array $data, $rule)
{
list($temp, $attr) = [$data, explode('.', trim($rule, '.'))];
while ($key = array_shift($attr)) $temp = isset($temp[$key]) ? $temp[$key] : $temp;
return (is_string($temp) || is_numeric($temp)) ? @iconv('UTF-8', 'GB2312//IGNORE', "{$temp}") : '';
}
# 总结
实现这个需求的核心不是在于数据,而是充分利用数组树形列表加上CSV文件格式导出。
如有表达不详细的地方,大家可以加我微信互相交流或在线留言
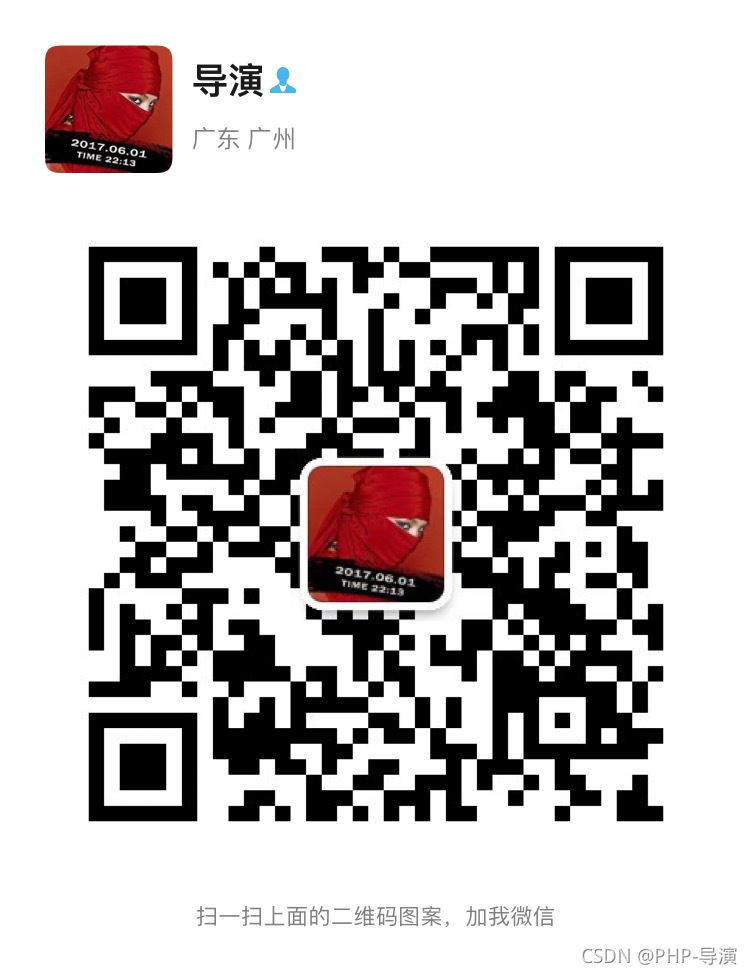
|