GUI编程
该怎么学?
- 这是什么?
- 它该怎么玩?
- 该如何去在我们平时运用?
- 反编译:把 class –> 可阅读 文件
需要学的东西
组件
- 窗口
- 弹窗
- 面板
- 文本框
- 列表框
- 按钮
- 图片
- 监听事件
- 鼠标
- 键盘事件
- 外挂:java写
- 破解工具
细分两个模块
1、简介
GUI 核心技术 :Swing AWT
(学java,就是在学一个一个的类)
不流行的原因:
- 界面不美观
- 需要 jre 环境!
为什么要学习?
它是 MVC 架构的基础
以后学习MVC的架构,在 GUI 编程里面会发现很多思想
包括 监听器 的思想
- 可以写出自己心中想要的一些小工具
- 工作时候,也可能需要维护到 swing 界面,概率极小!
- 了解 MVC 架构 ,了解监听!
2、AWT
它是 Swing 的前身
它 里面有大量原生的代码,会用得到
它和 Swing 的逻辑十分相似
用 AWT 讲解一些底层的实现
2.1、AWT介绍
java里面万物皆对象
new 类!
AWT 里边有很多一些列的类
AWT:抽象的窗口工具
- 包含了很多类和接口!
用于 GUI编程
GUI:图形用户界面编程(画窗口的)
Eeclipse:用java写的
-
AWT 里面有各种各样的元素 元素:窗口,按钮,文本框 -
java.awt 包
包的位置
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-yTgUGNyx-1631435319213)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210907164805074.png)]](https://img-blog.csdnimg.cn/7df660b8cea34c3aab5bbfcdf803a19c.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-PRdryCwY-1631435319215)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210907164827249.png)]](https://img-blog.csdnimg.cn/aca1d12f130746c78946a808cfe3b589.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16)
AWT 知识 和 类!
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-9Px9yTvF-1631435319216)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210907171924724.png)]](https://img-blog.csdnimg.cn/2c6aedc4fddb401dbcbef0954334369a.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16)
2.2、组件和容器
1、框架 Frame
package com.kuang.lesson01;
import java.awt.*;
public class TestFrame {
public static void main(String[] args) {
Frame frame = new Frame("我的第一个Java图像界面窗口");
frame.setSize(400,400);
frame.setLocation(200,200);
frame.setBackground(new Color(22, 127, 220));
frame.setResizable(false);
frame.setVisible(true);
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-GmKIheuM-1631435319218)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911151517334.png)]](https://img-blog.csdnimg.cn/ada4b3e0f8624eb59ec4d15e02a6aed0.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_14,color_FFFFFF,t_70,g_se,x_16)
问题:发现窗口关闭不掉,解决:停止java程序!
尝试回顾封装,继承:
展示多个窗口
package com.kuang.lesson01;
import java.awt.*;
public class TestFrame2 {
public static void main(String[] args) {
MyFrame myFrame1 = new MyFrame(100, 100, 200, 200, Color.blue);
MyFrame myFrame2 = new MyFrame(300, 100, 200, 200, Color.yellow);
MyFrame myFrame3 = new MyFrame(100, 300, 200, 200, Color.orange);
MyFrame myFrame4 = new MyFrame(300, 300, 200, 200, Color.pink);
}
}
class MyFrame extends Frame{
static int id = 0;
public MyFrame(int x,int y,int w,int h,Color color){
super("Myframe"+(++id));
setBackground(color);
setBounds(x,y,w,h);
setVisible(true);
setResizable(false);
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-MsMwQGy2-1631435319220)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911154233174-1631346153823-1631346154155.png)]](https://img-blog.csdnimg.cn/13304e3d595e4a2694a9ab388066e9cb.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_14,color_FFFFFF,t_70,g_se,x_16)
2、面板 panel
解决了关闭事件!
package com.kuang.lesson01;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
public class TextPanel {
public static void main(String[] args) {
Frame frame = new Frame();
Panel panel = new Panel();
frame.setLayout(null);
frame.setBounds(300, 300, 500, 500);
frame.setBackground(new Color(0x8E8EE1));
panel.setBounds(50, 50, 400, 400);
panel.setBackground(new Color(0xBBBB2C));
frame.add(panel);
frame.setVisible(true);
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
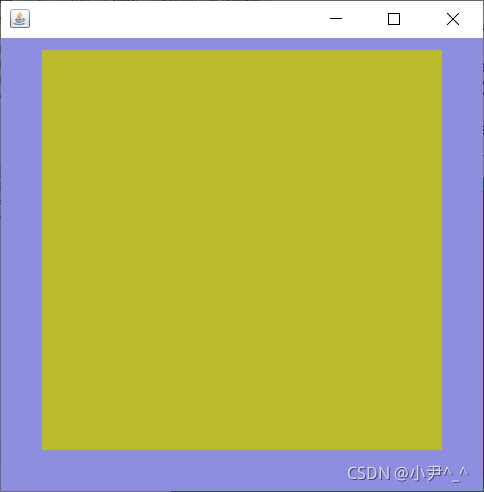
2.3、布局管理器 Layout
-
流失布局 从左到右 Flow package com.kuang.lesson01;
import java.awt.*;
public class TestFlowLayout {
public static void main(String[] args) {
Frame frame = new Frame();
Button button1 = new Button("button1");
Button button2 = new Button("button2");
Button button3 = new Button("button3");
frame.setLayout(new FlowLayout(FlowLayout.RIGHT));
frame.setSize(200,200);
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.setVisible(true);
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7sAfYwWq-1631435319221)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911165333231.png)]](https://img-blog.csdnimg.cn/913c10d55a7545aa84d735d5fbbba3e7.png) -
东西南北中 上下结构 Border ![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-OWOQXFkH-1631435319222)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911165431530.png)]](https://img-blog.csdnimg.cn/29b9d5c9ec834554950980e14ba709bb.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16) package com.kuang.lesson01;
import java.awt.*;
public class TestBorderLayout {
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
Button east = new Button("East");
Button west = new Button("West");
Button south = new Button("South");
Button north = new Button("North");
Button center = new Button("Center");
frame.add(east,BorderLayout.EAST);
frame.add(west,BorderLayout.WEST);
frame.add(south,BorderLayout.SOUTH);
frame.add(north,BorderLayout.NORTH);
frame.add(center,BorderLayout.CENTER);
frame.setSize(200,200);
frame.setVisible(true);
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-M0zJRBTt-1631435319223)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911171424598.png)]](https://img-blog.csdnimg.cn/6bbb4158c5454ed785c31fd9109b0202.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16) -
表格布局 Grid 三行两列 package com.kuang.lesson01;
import java.awt.*;
public class TestGridLayout {
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
Button btin1 = new Button("btin1");
Button btin2 = new Button("btin2");
Button btin3 = new Button("btin3");
Button btin4 = new Button("btin4");
Button btin5 = new Button("btin5");
Button btin6 = new Button("btin6");
frame.setLayout(new GridLayout(3,2));
frame.add(btin1);
frame.add(btin2);
frame.add(btin3);
frame.add(btin4);
frame.add(btin5);
frame.add(btin6);
frame.pack();
frame.setVisible(true);
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-31OYL8jx-1631435319223)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911172510847.png)]](https://img-blog.csdnimg.cn/3a9b9eb25afe4d8b80691510b81d893e.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_15,color_FFFFFF,t_70,g_se,x_16)
课堂练习讲解总结
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-tiJ4a8mN-1631435319224)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911173248984.png)]](https://img-blog.csdnimg.cn/c72159d7805a431982e64e302b6f49c1.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16)
分解过程
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-WToy9Tpj-1631435319225)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911173951606.png)]](https://img-blog.csdnimg.cn/68d4272582cd486eb19c3b5a119f82a0.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_20,color_FFFFFF,t_70,g_se,x_16)
代码实现
package com.kuang.lesson01;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class ExDemo {
public static void main(String[] args) {
Frame frame = new Frame();
frame.setSize(400,300);
frame.setLocation(300,200);
frame.setBackground(Color.blue);
frame.setVisible(true);
frame.setLayout(new GridLayout(2,1));
Panel p1 = new Panel(new BorderLayout());
Panel p2 = new Panel(new GridLayout(2,1));
Panel p3 = new Panel(new BorderLayout());
Panel p4 = new Panel(new GridLayout(2,2));
p1.add(new Button("East-1"),BorderLayout.EAST);
p1.add(new Button("East-1"),BorderLayout.WEST);
p2.add(new Button("p2-btn-1"));
p2.add(new Button("p2-btn-2"));
p1.add(p2,BorderLayout.CENTER);
p3.add(new Button("East-2"),BorderLayout.EAST);
p3.add(new Button("East-2"),BorderLayout.WEST);
for (int i = 0; i < 4; i++) {
p4.add(new Button("for-" +i));
}
p3.add(p4,BorderLayout.CENTER);
frame.add(p1);
frame.add(p3);
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-0NC6gpk4-1631435319225)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210911193749514.png)]](https://img-blog.csdnimg.cn/6875feeb88d3478795cda74b378aeea6.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBA5bCP5bC5Xl9e,size_14,color_FFFFFF,t_70,g_se,x_16)
总结:
-
Frame 是一个顶级窗口 -
Panel 无法单独显示,必须添加到某个容器中 -
Layout 布局管理器
-
流式 FlowLayout frame.setLayout(new FlowLayout(FlowLayout.RIGHT));
-
东西南北中 BorderLayout Button east = new Button("East");
frame.add(east,BorderLayout.EAST);
-
网格 GridLayout Button btin1 = new Button("btin1");
frame.setLayout(new GridLayout(3,2));
frame.add(btin1);
-
属性
-
大小 frame.setSize(100,100);
-
定位 //弹出的初始位置
//定位
frame.setLocation(200,200);
-
背景颜色 frame.setBackground(new Color(22, 127, 220));
-
可见性 frame.setVisible(true);
-
监听 frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
2.4、事件监听
事件监听:当某个事情发生的时候,他要干什么?
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestActionEvent {
public static void main(String[] args) {
Frame frame = new Frame();
Button button = new Button();
MyActionListener myActionListener = new MyActionListener();
button.addActionListener(myActionListener);
frame.add(button,BorderLayout.CENTER);
frame.pack();
frame.setVisible(true);
windowClose(frame);
}
private static void windowClose(Frame frame){
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
class MyActionListener implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("aaa");
}
}
多个按钮共用一个事件
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLOutput;
public class TestActionEventTwo {
public static void main(String[] args) {
Frame frame = new Frame("开始-停止");
Button button1 = new Button("start");
Button button2 = new Button("stop");
button2.setActionCommand("button-stop");
MyMonitor myMonitor = new MyMonitor();
button1.addActionListener(myMonitor);
button2.addActionListener(myMonitor);
frame.add(button1,BorderLayout.NORTH);
frame.add(button2,BorderLayout.SOUTH);
frame.pack();
frame.setVisible(true);
}
}
class MyMonitor implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("按钮被被点击了:msg=>"+e.getActionCommand());
}
}
2.5、输入框 TextField 需要被监听
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestText01 {
public static void main(String[] args) {
new MyFrame();
}
}
class MyFrame extends Frame{
public MyFrame(){
TextField textField = new TextField();
add(textField);
MyActionListener2 myActionListener2 = new MyActionListener2();
textField.addActionListener(myActionListener2);
textField.setEchoChar('*');
setVisible(true);
pack();
}
}
class MyActionListener2 implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
TextField field= (TextField) e.getSource();
System.out.println(field.getText());
field.setText("");
}
}
2.6、简易计算器,组合+内部类回顾复习!
oop原则:组合,大于继承!
class A extends B{
}
class A {
public B b;
}
耦合:
两个类的方法或者逻辑放在了一起,导致无法抽出来,不能很好 地维护
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ZWkRJq6u-1631435319226)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210912144552295.png)]](https://img-blog.csdnimg.cn/e121ad164dec4ee29d951e26cbee6764.png)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-v92P4IdH-1631435319226)(GUI%E7%BC%96%E7%A8%8B.assets/image-20210912152948575.png)]](https://img-blog.csdnimg.cn/b9469ca935e14452bb67dd3ad5cd31c2.png)
目前代码 面向过程!
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestCalc {
public static void main(String[] args) {
new Calculator();
}
}
class Calculator extends Frame{
public Calculator(){
TextField num1 = new TextField(10);
TextField num2 = new TextField(10);
TextField num3 = new TextField(20);
Button button = new Button(" = ");
button.addActionListener(new MyCalculatorListener(num1,num2,num3));
Label label = new Label(" + ");
setLayout(new FlowLayout());
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
class MyCalculatorListener implements ActionListener{
private TextField num1,num2,num3;
public MyCalculatorListener(TextField num1,TextField num2,TextField num3) {
this.num1 = num1;
this.num2 = num2;
this.num3 = num3;
}
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(num1.getText());
int n2 = Integer.parseInt(num2.getText());
num3.setText(""+(n1+n2));
num1.setText("");
num2.setText("");
}
}
优化代码 面向对象!
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestCalc {
public static void main(String[] args) {
new Calculator().loadFrame();
}
}
class Calculator extends Frame{
TextField num1,num2,num3;
public void loadFrame(){
num1 = new TextField(10);
num2 = new TextField(10);
num3 = new TextField(20);
Button button = new Button(" = ");
Label label = new Label(" + ");
button.addActionListener(new MyCalculatorListener(this));
setLayout(new FlowLayout());
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
class MyCalculatorListener implements ActionListener{
Calculator calculator = null;
public MyCalculatorListener(Calculator calculator) {
this.calculator = calculator;
}
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(calculator.num1.getText());
int n2 = Integer.parseInt(calculator.num2.getText());
calculator.num3.setText(""+(n1+n2));
calculator.num1.setText("");
calculator.num2.setText("");
}
}
完全改造成 面向对象!
内部类:
- 更好的包装
- 内部类最大的好处,就是可以畅通无阻的访问外部类的属性和方法
package com.kuang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestCalc {
public static void main(String[] args) {
new Calculator().loadFrame();
}
}
class Calculator extends Frame{
TextField num1,num2,num3;
public void loadFrame(){
num1 = new TextField(10);
num2 = new TextField(10);
num3 = new TextField(20);
Button button = new Button(" = ");
Label label = new Label(" + ");
button.addActionListener(new MyCalculatorListener());
setLayout(new FlowLayout());
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
private class MyCalculatorListener implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
int n1 = Integer.parseInt(num1.getText());
int n2 = Integer.parseInt(num2.getText());
num3.setText(""+(n1+n2));
num1.setText("");
num2.setText("");
}
}
}
2.7、画笔
3、Swing
用 Swing 画一些界面,做一些想做的东西
|