Set 接口基本介绍
- 无序(添加和取出的顺序不一致),没有索引
- 不允许重复元素,所以最多包含一个null
package collection_;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
public class SetMethod {
public static void main(String[] args) {
Set set = new HashSet();
set.add(null);
set.add("Gin");
set.add("Vodka");
set.add("Sherry");
set.add("Vermouth");
set.add("Vodka");
set.add(null);
for(int i = 0; i < 5; i++) {
System.out.println(set);
}
System.out.println("==迭代器==");
Iterator iterator = set.iterator();
while (iterator.hasNext()) {
Object next = iterator.next();
System.out.println(next);
}
System.out.println("==增强 for==");
for (Object o : set) {
System.out.println(o);
}
}
}
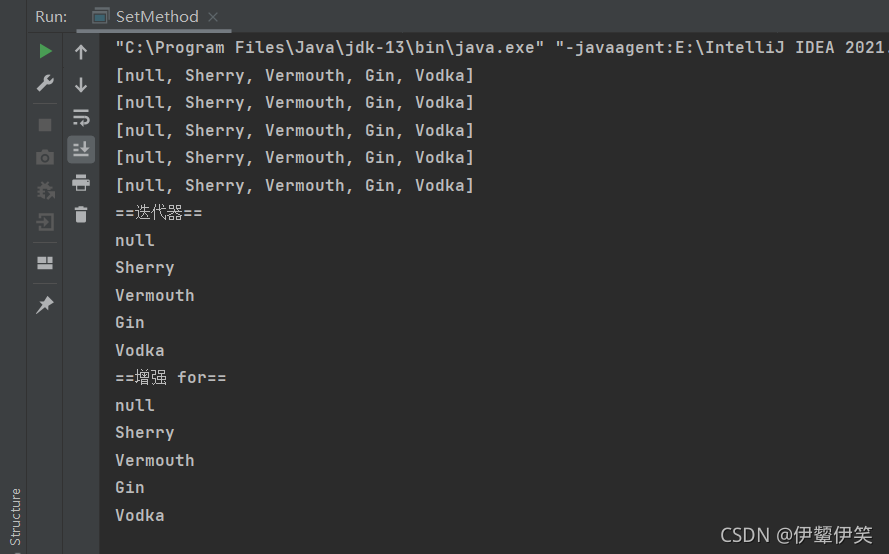
HashSet
- HashSet实现了Set接口
- HashSet实际上是HashMap
- 可以存放null值,但是只能有一个null
- HashSet不保证元素是有序的,取决于hash后,再确定索引的结果(即,不保证存放元素的)
- 不能有重复元素/对象
- String字符出存放位置(常量池)
package collection_;
import java.util.HashSet;
import java.util.Set;
public class HashSet_ {
public static void main(String[] args) {
Set set = new HashSet();
set.add(null);
set.add(null);
System.out.println(set);
System.out.println(set.add("Gin"));
System.out.println(set.add("Gin"));
System.out.println(set.add("Vodka"));
System.out.println(set.add("Sherry"));
System.out.println(set.add("Vermouth"));
System.out.println(set);
System.out.println(set.remove("Vodka"));
System.out.println(set);
set = new HashSet();
set.add("lucy");
set.add("lucy");
set.add(new Tiger("Gin"));
set.add(new Tiger("Gin"));
System.out.println(set);
set = new HashSet();
set.add(new String("Gin"));
set.add(new String("Gin"));
System.out.println(set);
}
}
class Tiger{
private String name;
public Tiger(String name) {
this.name = name;
}
@Override
public String toString() {
return "name='" + name ;
}
}
数组链表模拟
HashSet 底层就是 HashMap,HashMap 底层是(数组 + 链表 + 红黑树) 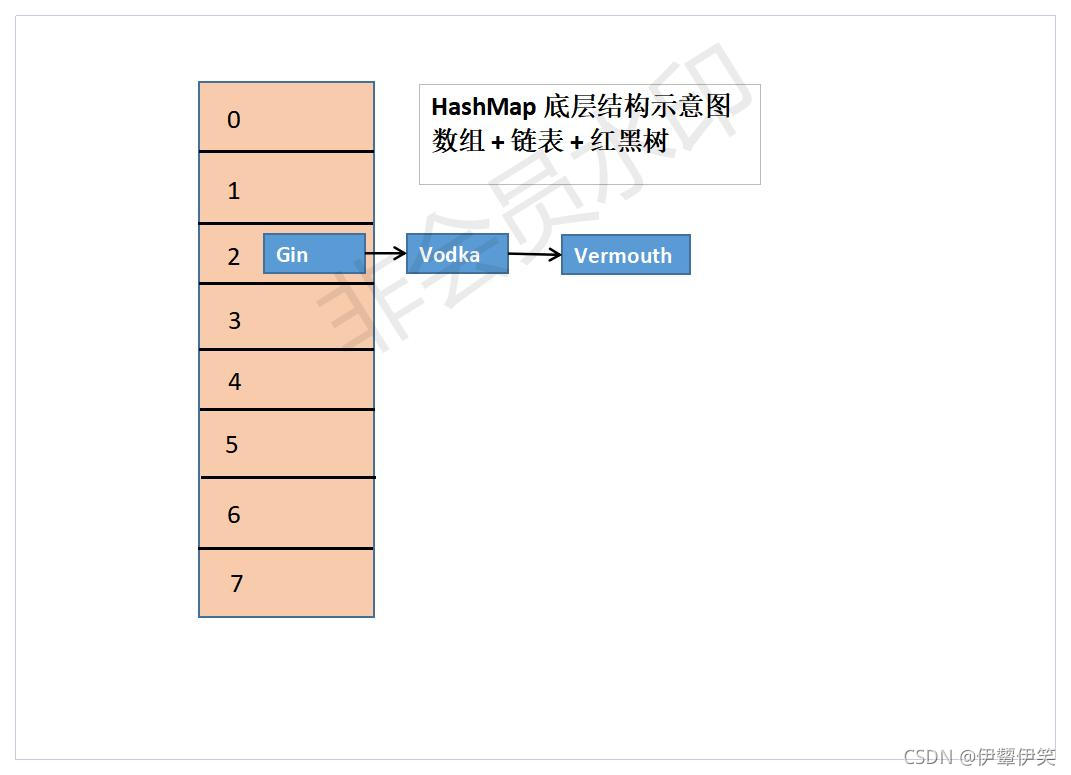
package collection_;
public class HashSetStructure {
public static void main(String[] args) {
Nod[] table = new Nod[16];
System.out.println("table = " + table);
Nod Gin = new Nod("Gin", null);
table[2] = Gin;
Nod Vodka = new Nod("Vodka", null);
Gin.next = Vodka;
Nod Vermouth = new Nod("Vermouth", null);
Vodka.next = Vermouth;
System.out.println("table = " + table);
}
}
class Nod{
Object item;
Nod next;
public Nod(Object item, Nod next) {
this.item = item;
this.next = next;
}
}
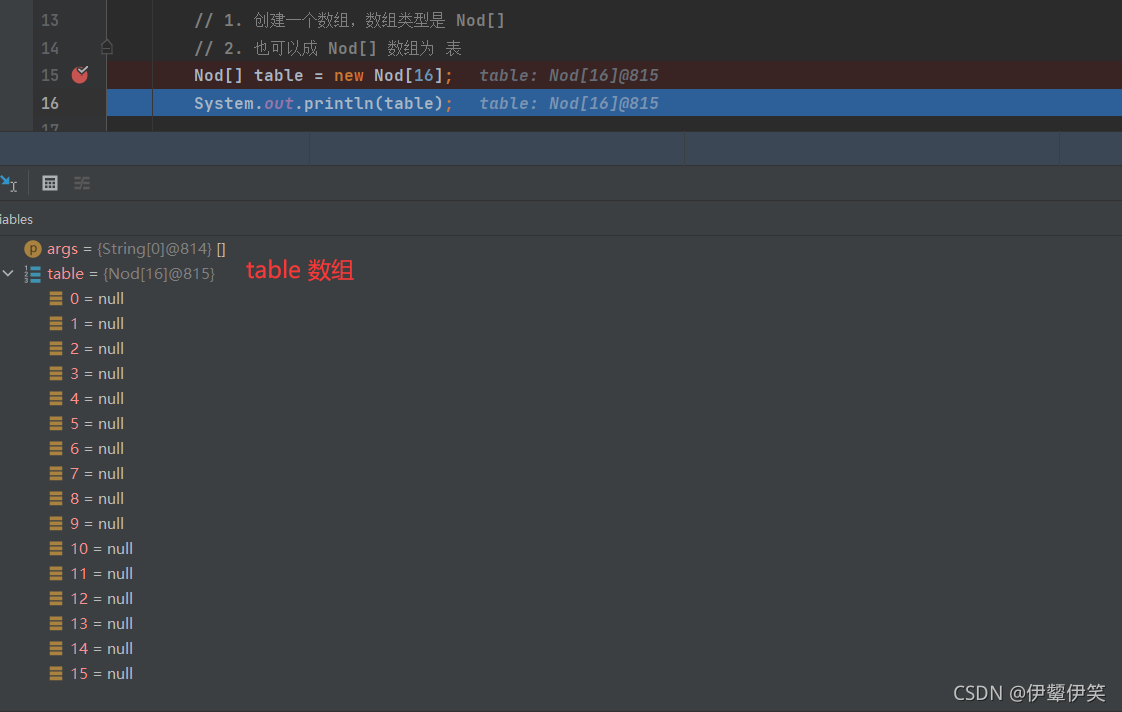
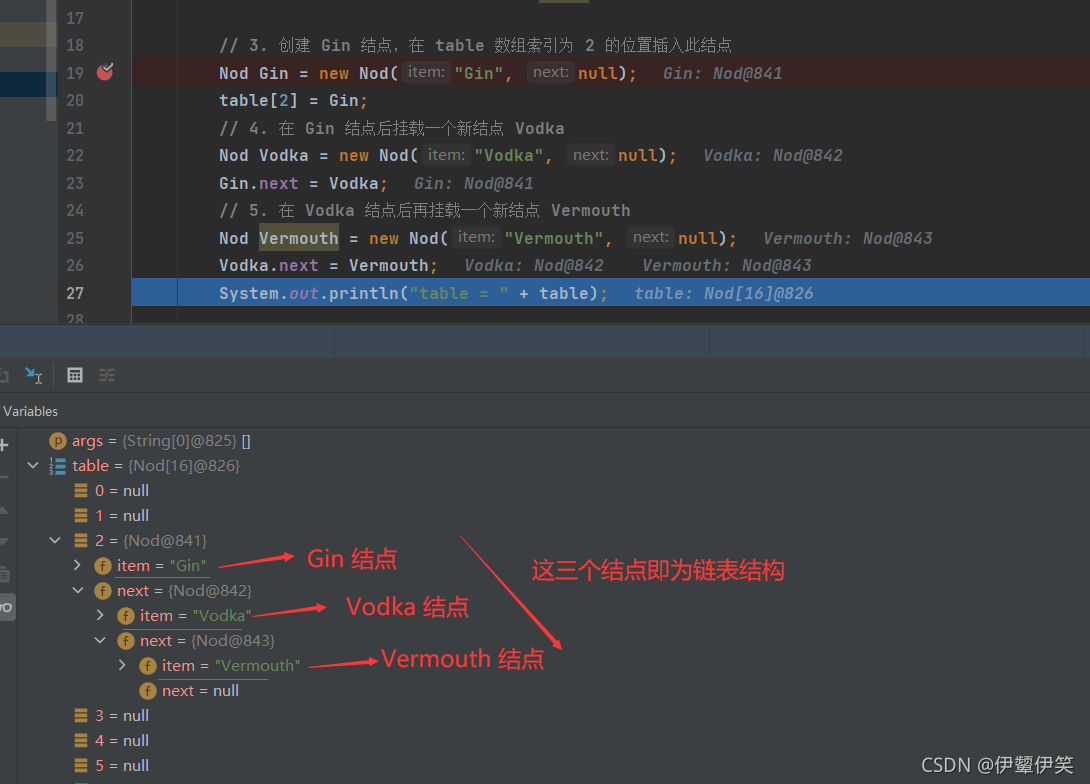
HashSet 源码解读(1)
向空的 HashSet 中加入第一个元素
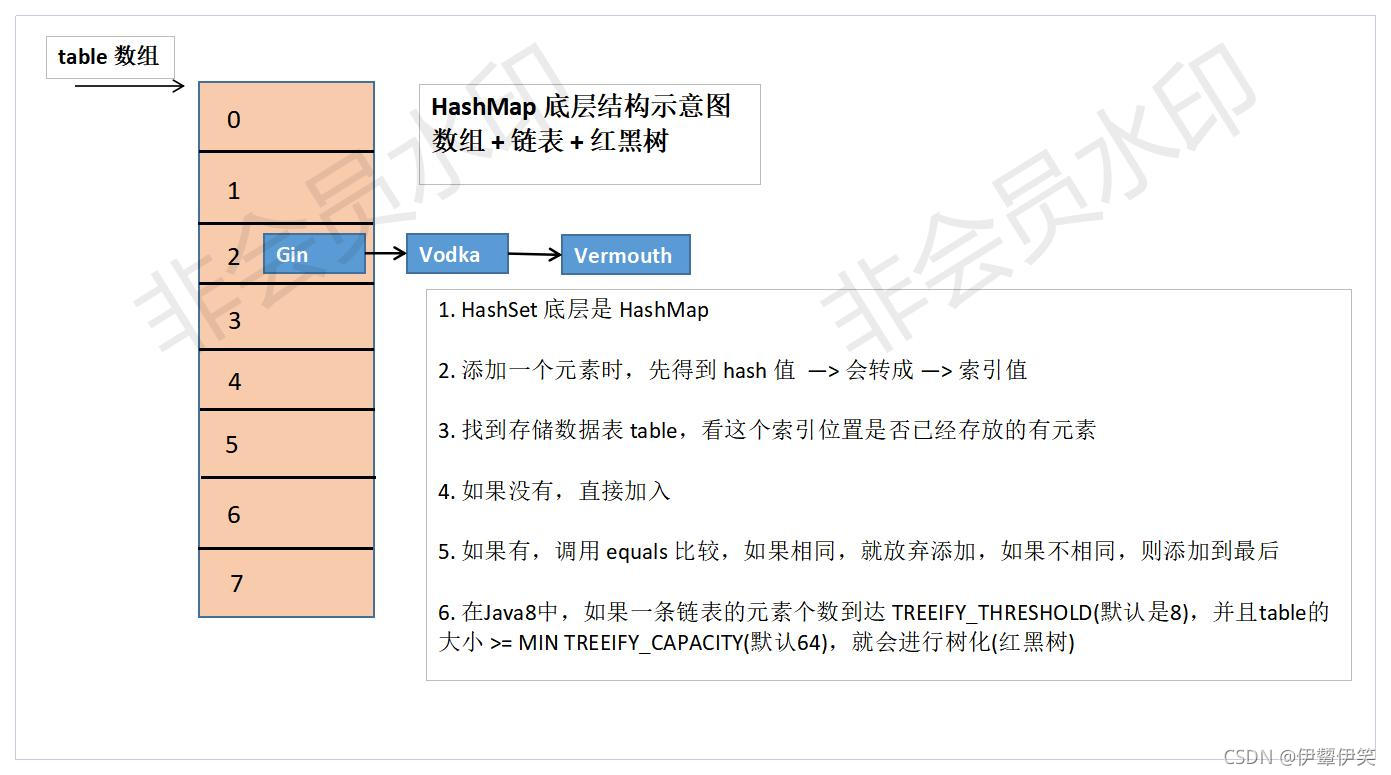
package collection_;
import java.util.HashSet;
import java.util.Set;
public class HashSetSource {
public static void main(String[] args) {
Set set = new HashSet();
set.add("java");
set.add("php");
set.add("java");
System.out.println(set);
}
}
如图表示第一次添加成功后,tab数组(即table数组)存放数据的情况 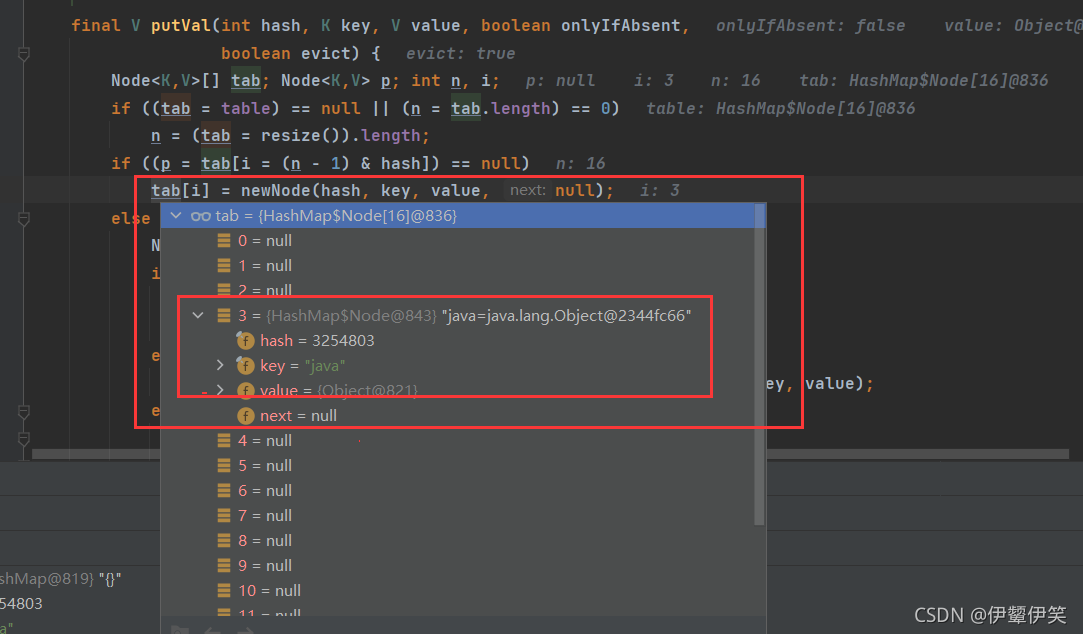
HashSet 源码解读(2)
如何判断添加的元素与链表的元素是否相同
package collection_;
import java.util.HashSet;
import java.util.Set;
public class HashSetSource {
public static void main(String[] args) {
Set set = new HashSet();
set.add("java");
set.add("php");
set.add("java");
System.out.println(set);
}
}
|