前言
项目中使用到事务的地方基本上是增删改,即对数据库的无论是新增、删除、修改都是修改操作,一般情况下一个接口中的多表修改操作基本上要用到@Transacational注解,但是后来发现没有用到@Transacational?注解也实现了这个效果,询问了一下老同事应该是已经全局定义了事务,如下
DruidDataSource数据源的自定义配置
package com.wscar.xny.auth.config;
import com.alibaba.druid.pool.DruidDataSource;
import com.wscar.xny.commons.utils.Base64;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@ToString
@Slf4j
public class DateSourceConfig {
@Value("${druid.driverClassName:}")
private String driverClassName;
@Value("${druid.url:}")
private String url;
@Value("${druid.username:}")
private String userName;
@Value("${druid.password:}")
private String password;
@Value("${druid.filters:}")
private String filters;
@Value("${druid.initialSize:}")
private Integer initialSize;
@Value("${druid.maxActive:}")
private Integer maxActive;
@Value("${druid.minIdle:}")
private Integer minIdle;
@Value("${druid.maxWait:}")
private Integer maxWait;
@Value("${druid.timeBetweenEvictionRunsMillis:}")
private Integer timeBetweenEvictionRunsMillis;
@Value("${druid.minEvictableIdleTimeMillis:}")
private Integer minEvictableIdleTimeMillis;
@Value("${druid.validationQuery:}")
private String validationQuery;
@Value("${druid.testWhileIdle:true}")
private boolean testWhileIdle;
@Value("${druid.testOnBorrow:true}")
private boolean testOnBorrow;
@Value("${druid.testOnReturn:true}")
private boolean testOnReturn;
@Value("${druid.removeAbandoned:true}")
private boolean removeAbandoned;
@Value("${druid.logAbandoned:true}")
private boolean logAbandoned;
@Value("${druid.removeAbandonedTimeout:1800}")
private Integer removeAbandonedTimeout;
@Bean
public DruidDataSource dataSource() {
log.info("数据库配置文件:" + this.toString());
try {
String username = Base64.decode(this.userName, "utf-8");
String password = Base64.decode(this.password, "utf-8");
boolean removeAbandoned = true;
boolean logAbandoned = true;
Integer removeAbandonedTimeout = 1800;
DruidDataSource dataSource = new DruidDataSource();
dataSource.setDriverClassName(this.driverClassName);
dataSource.setUrl(this.url);
dataSource.setUsername(username);
dataSource.setPassword(password);
dataSource.setFilters(this.filters);
dataSource.setInitialSize(this.initialSize);
dataSource.setMaxActive(this.maxActive);
dataSource.setMinIdle(this.minIdle);
dataSource.setMaxWait(this.maxWait);
dataSource.setTimeBetweenEvictionRunsMillis(this.timeBetweenEvictionRunsMillis);
dataSource.setMinEvictableIdleTimeMillis(this.minEvictableIdleTimeMillis);
dataSource.setValidationQuery(this.validationQuery);
dataSource.setTestOnBorrow(this.testOnBorrow);
dataSource.setTestOnReturn(this.testOnReturn);
dataSource.setRemoveAbandoned(removeAbandoned);// 打开removeAbandoned功能
dataSource.setRemoveAbandonedTimeout(removeAbandonedTimeout);
dataSource.setLogAbandoned(logAbandoned);// 关闭abanded连接时输出错误日志
dataSource.setTestWhileIdle(this.testWhileIdle);
dataSource.fill(1);//做数据库连接测试
return dataSource;
} catch (Exception e) {
log.error("初始化dataSource异常", e);
}
return null;
}
}
Aop切面进行事务统一处理
package com.wscar.xny.auth.config;
import org.springframework.aop.Advisor;
import org.springframework.aop.aspectj.AspectJExpressionPointcut;
import org.springframework.aop.support.DefaultPointcutAdvisor;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import org.springframework.transaction.interceptor.TransactionInterceptor;
import javax.sql.DataSource;
import java.util.Properties;
@Configuration
public class DbAopTransConfig {
@Bean
public TransactionInterceptor txAdvice(@Autowired DataSourceTransactionManager transactionManager) throws Exception {
//针对add、save、insert、update、modify、remove、delete、change、cancel等开头的方法进行事务处理
Properties properties = new Properties();
properties.setProperty("add*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("save*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("insert*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("update*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("modify*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("delete*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("remove*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("change*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("cancel*", "PROPAGATION_REQUIRED,-Throwable");
properties.setProperty("*WithSqlTrans", "PROPAGATION_REQUIRED,-Throwable");
TransactionInterceptor tsi = new TransactionInterceptor(transactionManager, properties);
return tsi;
}
@Bean
public DataSourceTransactionManager transactionManager(@Qualifier("dataSource") DataSource dataSource) {
DataSourceTransactionManager bean = new DataSourceTransactionManager();
bean.setDataSource(dataSource);
return bean;
}
@Bean
public Advisor txAdviceAdvisor(@Autowired TransactionInterceptor txAdvice) {
AspectJExpressionPointcut pointcut = new AspectJExpressionPointcut();
//切入点是impl包下的所有包或者类下的方法
pointcut.setExpression("execution(* com.wscar.xny.auth.service.impl.*.*(..))");
return new DefaultPointcutAdvisor(pointcut, txAdvice);
}
}
?项目结构
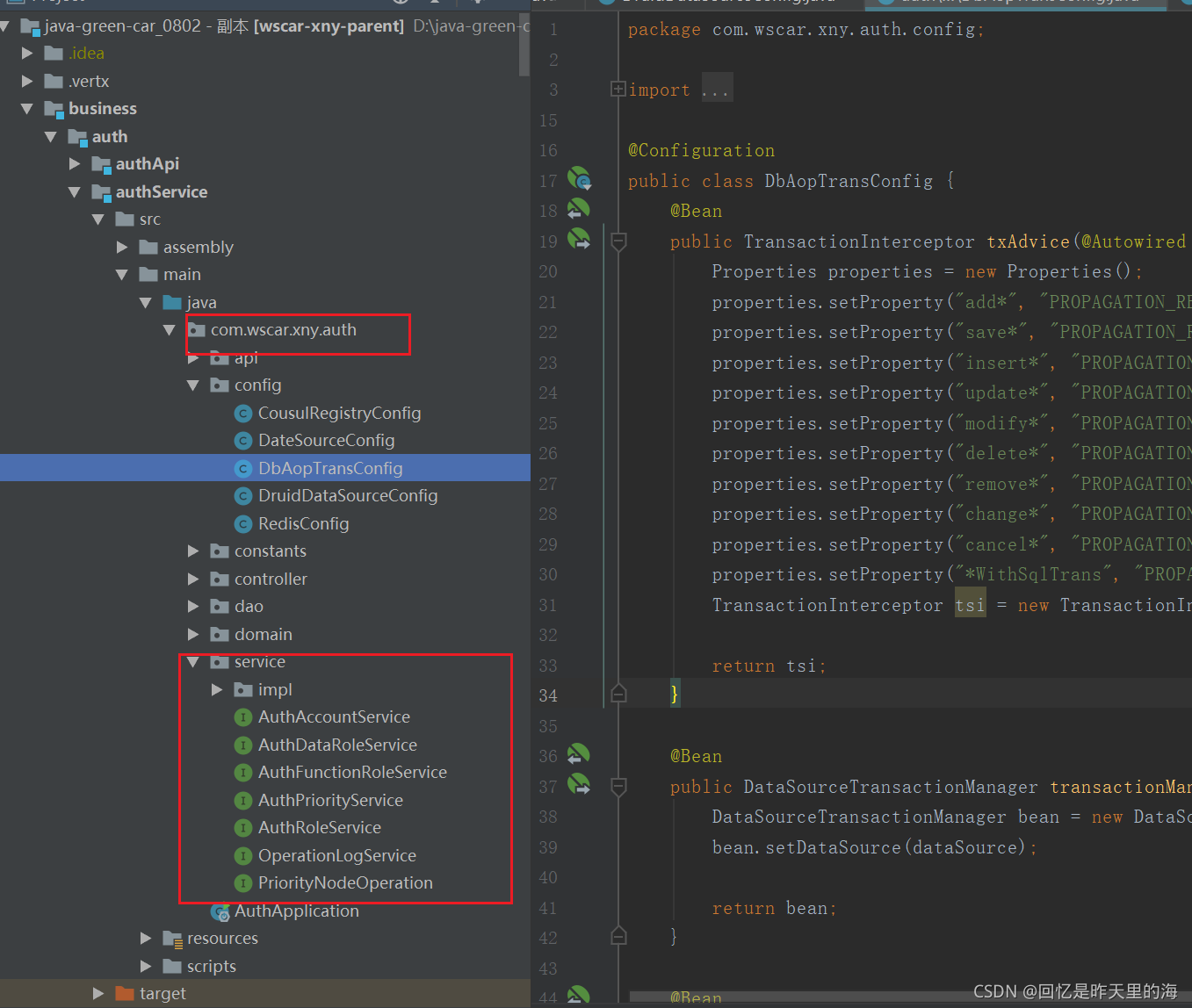
?推荐博客
?Springboot的切点可配置化-DefaultPointcutAdvisor_sinat_31396769的博客-CSDN博客
如遇到知识性的错误,请留言?
?
|