 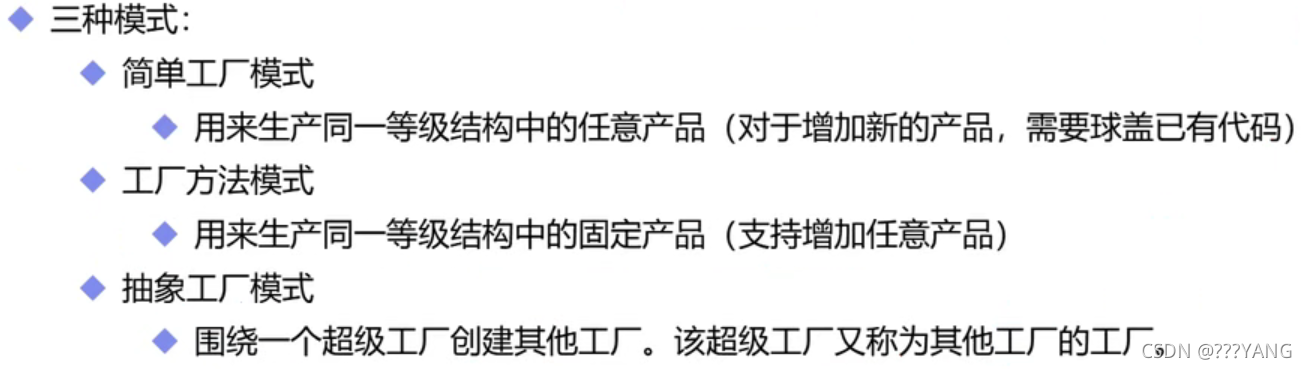
简单工厂模式
步骤 1 创建一个接口:
public interface Shape {
void draw();
}
步骤 2 创建实现接口的实体类。
Rectangle.java
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Inside Rectangle::draw() method.");
}
}
Square.java
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Inside Square::draw() method.");
}
}
Circle.java
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
步骤 3 创建一个工厂,生成基于给定信息的实体类的对象。
ShapeFactory.java
public class ShapeFactory {
public Shape getShape(String shapeType){
if(shapeType == null){
return null;
}
if(shapeType.equalsIgnoreCase("CIRCLE")){
return new Circle();
} else if(shapeType.equalsIgnoreCase("RECTANGLE")){
return new Rectangle();
} else if(shapeType.equalsIgnoreCase("SQUARE")){
return new Square();
}
return null;
}
}
步骤4 测试
FactoryPatternDemo.java
public class FactoryPatternDemo {
public static void main(String[] args) {
ShapeFactory shapeFactory = new ShapeFactory();
Shape shape1 = shapeFactory.getShape("CIRCLE");
shape1.draw();
Shape shape2 = shapeFactory.getShape("RECTANGLE");
shape2.draw();
Shape shape3 = shapeFactory.getShape("SQUARE");
shape3.draw();
}
}
步骤5 结果
Inside Circle::draw() method.
Inside Rectangle::draw() method.
Inside Square::draw() method.
注:大多数情况下用的都是简单工厂模式,这种模式仍有不足,如果再增加一辆车,则会修改CarFactory.java的getCar方法,违反了开闭原则,我们应该扩展,不应该修改
工厂方法模式
我们新建一个CarFactory的接口,然后为每种Car都建一个Factory类。这样就可以使得每次新加入一种车时,只为这种车建立一个对应的工厂就行,不会影响到原来的代码。
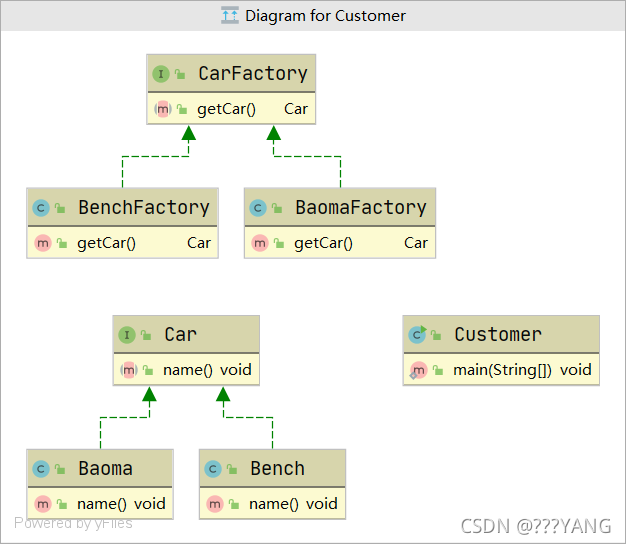
public interface CarFactory {
Car getCar();
}
public class BenchFactory implements CarFactory{
@Override
public Car getCar() {
return new Bench();
}
}
public class Customer {
public static void main(String[] args) {
Car car = new BenchFactory().getCar();
car.name();
}
}
优点:下次加入一种大众车,只需要新建一个 DazhongFactory 就行,不会影响到别的工厂。 缺点:代码量加大,管理复杂,结构复杂,实际业务一般使用简单工厂模式
|