*10.15编写一个方法,为二维平面上一系列点返回一个边框
题目
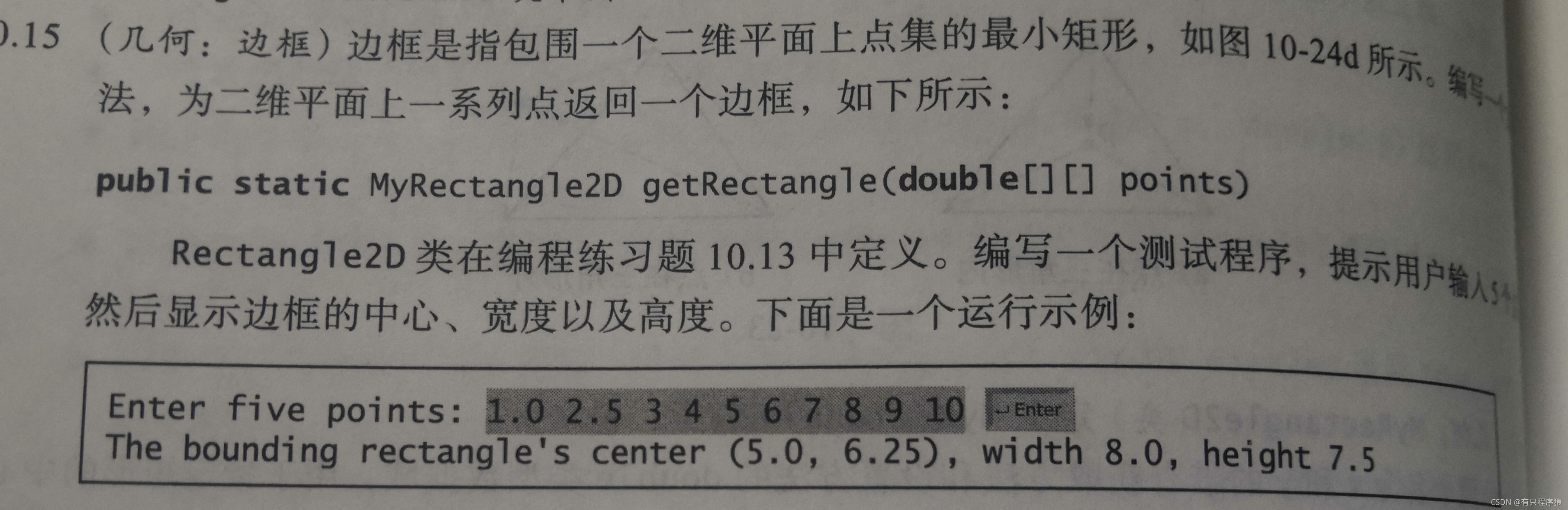 点击这里跳转编程练习题10.13
程序
Test15.java:测试程序 Test13_MyRectangle2D.java:编程练习题10.13构造程序
代码
Test15.java
import java.util.Scanner;
public class Test15 {
public static void main(String[] args) {
double[][] points = new double[5][2];
System.out.print("Enter five points: ");
Scanner input = new Scanner(System.in);
for (int m = 0 ; m < 5 ; m++){
for (int n = 0 ; n < 2 ; n++){
points[m][n] = input.nextDouble();
}
}
Test13_MyRectangle2D mt = getRectangle(points);
System.out.print("The bounding rectangle's center (" + mt.getX() + ", " + mt.getY() +
"), width " +mt.getWidth() + ", height " + mt.getHeight());
}
public static Test13_MyRectangle2D getRectangle(double[][] points){
double xMax = points[0][0], yMax = points[0][1], xMin = points[0][0], yMin = points[0][1];
for (int a = 0 ; a < points.length ; a++){
if (points[a][0] > xMax)
xMax = points[a][0];
if (points[a][0] < xMin)
xMin = points[a][0];
if (points[a][1] > yMax)
yMax = points[a][1];
if (points[a][1] < yMin)
yMin = points[a][1];
}
return new Test13_MyRectangle2D((xMax + xMin) / 2, (yMax + yMin) / 2, xMax - xMin, yMax - yMin);
}
}
Test13_MyRectangle2D.java
public class Test13_MyRectangle2D {
private double x, y;
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
private double width, height;
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public Test13_MyRectangle2D(){
x = 0;
y = 0;
width = 1;
height = 1;
}
public Test13_MyRectangle2D(double x, double y, double width, double height){
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public double getArea(){
return width * height;
}
public double getPerimeter(){
return 2 * (width + height);
}
public boolean contains(double x, double y){
boolean bool1 = false, bool2 = false;
if (x > this.x - width / 2 && x < this.x + width / 2)
bool1 = true;
if (y > this.y - height / 2 && y < this.y + height / 2)
bool2 = true;
return bool1 && bool2;
}
public boolean contains(Test13_MyRectangle2D r){
return ((Math.abs(x - r.x)) < width / 2) && (Math.abs(y - r.y) < height / 2);
}
public boolean overlaps(Test13_MyRectangle2D r){
return ((Math.abs(x - r.x)) < (width / 2 + r.width / 2)) && (Math.abs(y - r.y) < (height / 2 + r.height / 2));
}
}
运行结果
Enter five points:1.0 2.5 3 4 5 6 7 8 9 10
The bounding rectangle's center (5.0, 6.25), width 8.0, height 7.5
|