1.手动监听
<?php
declare (strict_types = 1);
namespace app\index\controller;
use think\facade\Event;
class Index
{ public function __construct(){
Event::listen('first', function($param){
echo $param . '+';
});
}
public function index()
{
event('first', 'first success');
return '您好!这是一个[index]示例应用';
}
}
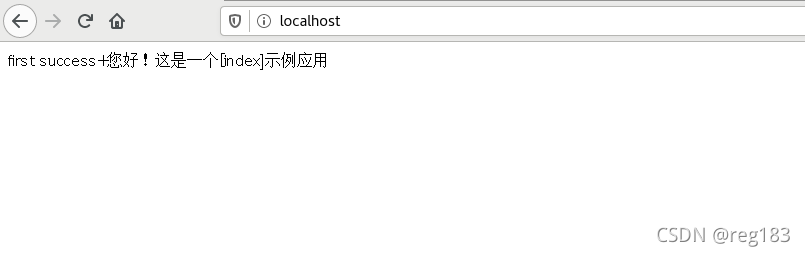
2.监听器监听
<?php
/**
* Created by PhpStorm.
* User: Administrator
* Date: 2021/9/18
* Time: 14:46
*/
namespace app\index\listener;
class TestListener
{
public function handle($event)
{
//
echo "testListner监听成功";
}
}
<?php
declare (strict_types = 1);
namespace app\index\controller;
use think\facade\Event;
class Index
{
public function index()
{
Event::listen('test', 'app\index\listener\TestListener');
event('test');
return '您好!这是一个[index]示例应用';
}
}
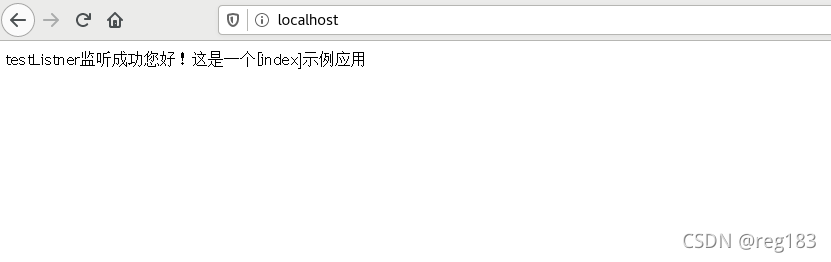
3.修改event.php文件,添加事件监听
监听器还是上面的监听器
<?php
/**
* Created by PhpStorm.
* User: Administrator
* Date: 2021/9/18
* Time: 14:46
*/
namespace app\index\listener;
class TestListener
{
public function handle($event)
{
//
echo "testListner监听成功";
}
}
修改event.php
<?php
// 这是系统自动生成的event定义文件
return [
'listen' => [
'test' => ['app\index\listener\TestListener']
]
];
修改调用的代码
<?php
declare (strict_types = 1);
namespace app\index\controller;
use think\facade\Event;
class Index
{
public function index()
{
event('test');
return '您好!这是一个[index]示例应用';
}
}
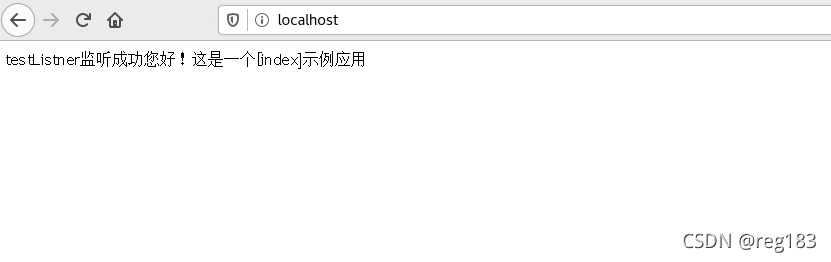
4.事件订阅
添加订阅类
<?php
/**
* Created by PhpStorm.
* User: Administrator
* Date: 2021/9/18
* Time: 15:00
*/
namespace app\index\subscribe;
class TestSub
{
public function onTestSub1(){
echo "testSub1";
}
public function onTestSub2(){
echo "testSub2";
}
}
修改event.php,绑定订阅类
<?php
// 这是系统自动生成的event定义文件
return [
'listen' => [
'test' => ['app\index\listener\TestListener']
],
'subscribe' => [
'app\index\subscribe\TestSub'
],
];
调用订阅的方法
<?php
declare (strict_types = 1);
namespace app\index\controller;
use think\facade\Event;
class Index
{
public function index()
{
event('test');
event('TestSub1');
event('TestSub2');
return '您好!这是一个[index]示例应用';
}
}
效果如下 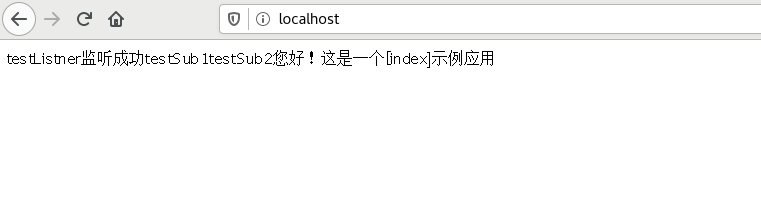
|