Artisan 是 Laravel 自带的命令行接口,他提供了许多使用的命令来帮助你构建 Laravel 应用 。
1、php artisan 命令列表
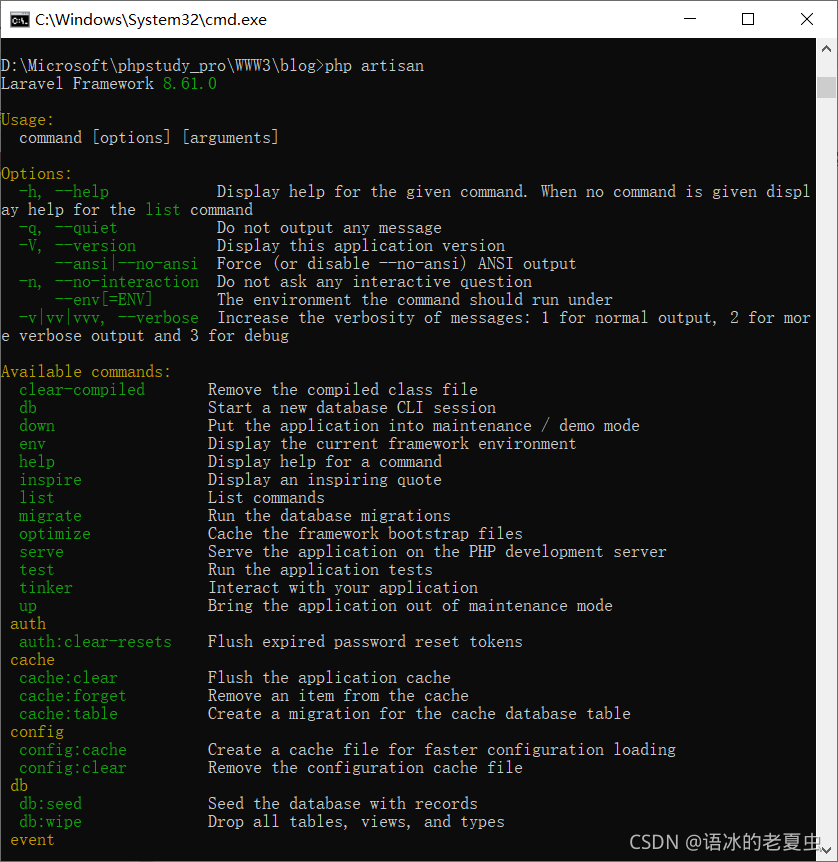 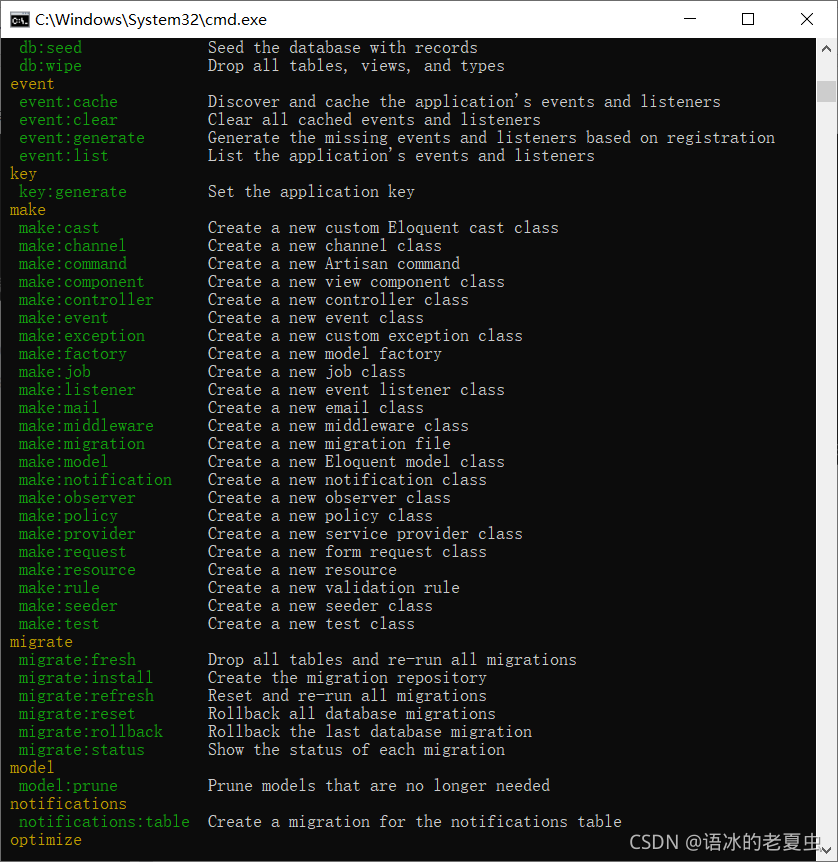 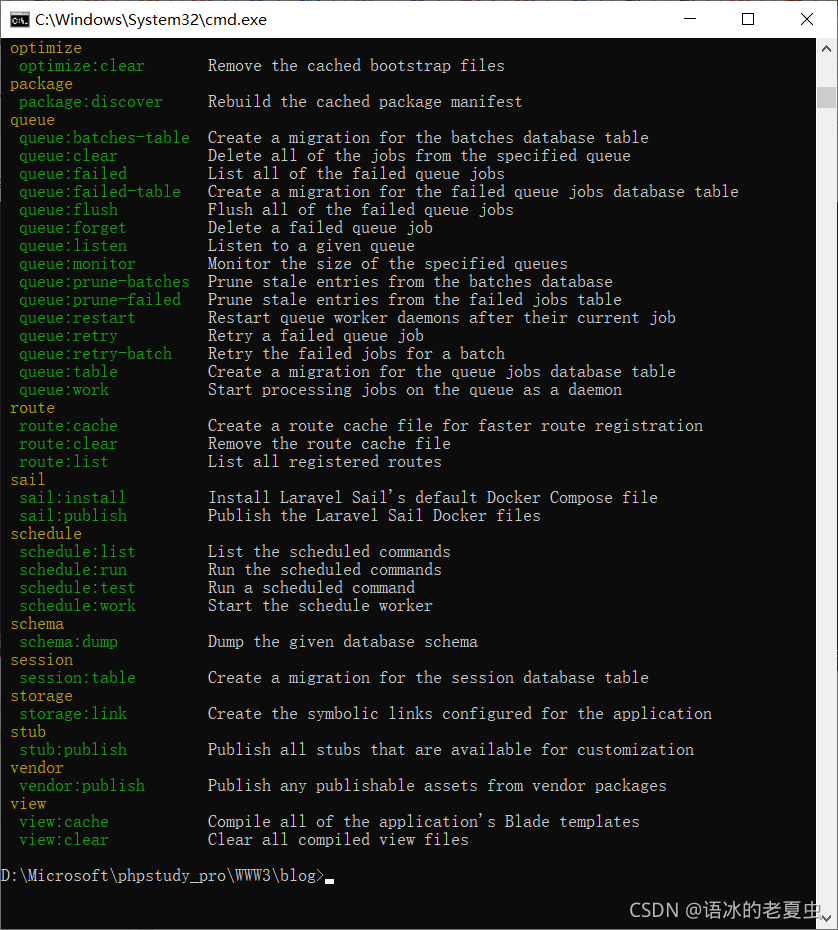
2、常用命令
查看artisan命令 php artisan php artisan list
查看某个帮助命令 php artisan help make:model
查看laravel版本 php artisan --version  使用 PHP 内置的开发服务器启动应用 php artisan serve 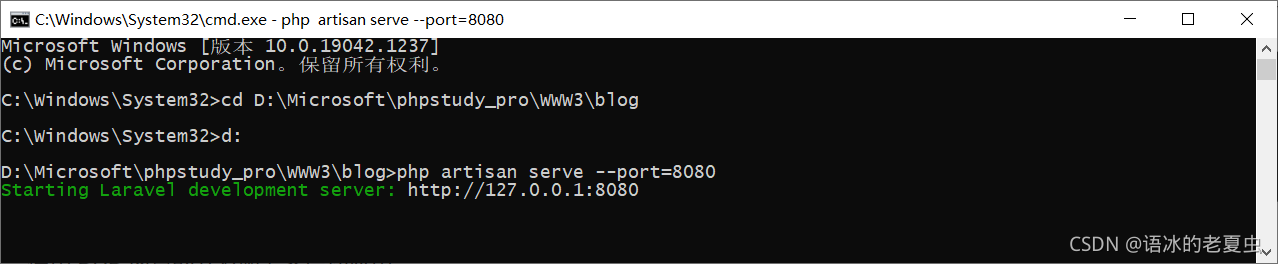
生成一个随机的 key,并自动更新到文件.env里的APP_KEY的键值。 php artisan key:generate   开启维护模式和关闭维护模式(显示503) php artisan down php artisan up
进入tinker工具 php artisan tinker
3、php artisan route
列出所有的路由 php artisan route:list 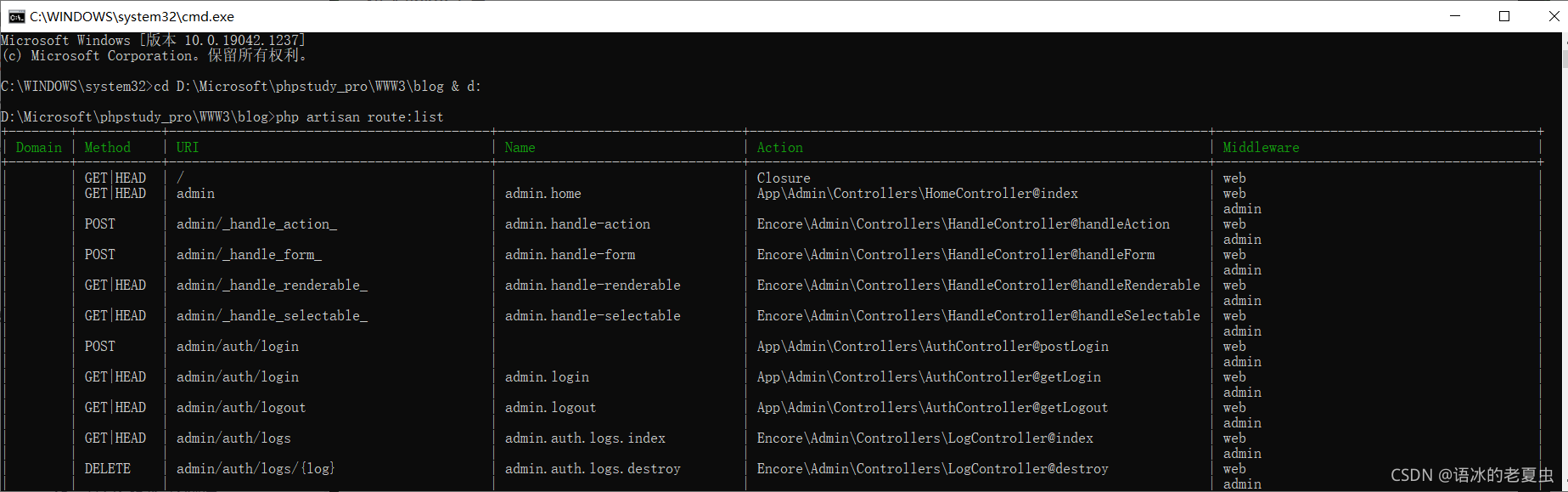 生成路由缓存以及移除缓存路由文件 php artisan route:cache php artisan route:clear
4、php artisan make
4.1 php artisan make:controller
//在目录 \app\Http\Controllers\生成控制器TestController.php,一个空控制器 php artisan make:controller TestController
// 指定创建位置 在app目录下创建TestController php artisan make:controller App\TestController
// 创建Rest风格资源控制器(带有index、create、store、edit、update、destroy、show方法) php artisan make:controller TestController –resource
4.2 php artisan make:model
创建模型 php artisan make:model Student
php artisan make:model App\Models\Test // 指定路径app\Models\创建 生成模型Test.php php artisan make:controller TestController --resource --model=Test //创建前台资源控制器附带模型
创建模型的时候同时生成新建表的迁移+控制器+路由 php artisan make:model Order -m -c -r
4.4 php artisan make:migration
创建新建表的迁移和修改表的迁移 //创建students表 php artisan make:migration create_users_table --create=students
//给students表增加votes字段 php artisan make:migration add_votes_to_users_table --table=students
//修改表 php artisan make:migration alter_pub_size_info_table --table=pub_size_info
5、php artisan migrate
php artisan migrate php artisan migrate:rollback php artisan migrate:reset php artisan migrate:refresh
命令 | 中文 | English |
---|
migrate | 执行迁移 | migrate | migrate:fresh | 删除所有表并重新运行所有迁移 | Drop all tables and re-run all migrations | migrate:install | 创建迁移存储库 | Create the migration repository | migrate:refresh | 重置并重新运行所有迁移 | Reset and re-run all migrations | migrate:reset | 回滚所有数据库迁移 | Rollback all database migrations | migrate:rollback | 回滚上次数据库迁移 | Rollback the last database migration | migrate:status | 显示每次迁移的状态 | Show the status of each migration |
5.1 Create Model and Migration
参考文章 创建模型的时候同时生成新建表的迁移
php artisan make:model Student -m
 (1)在文件夹D:\Microsoft\phpstudy_pro\WWW3\blog\app\Models,自动创建Model文件Student.php。 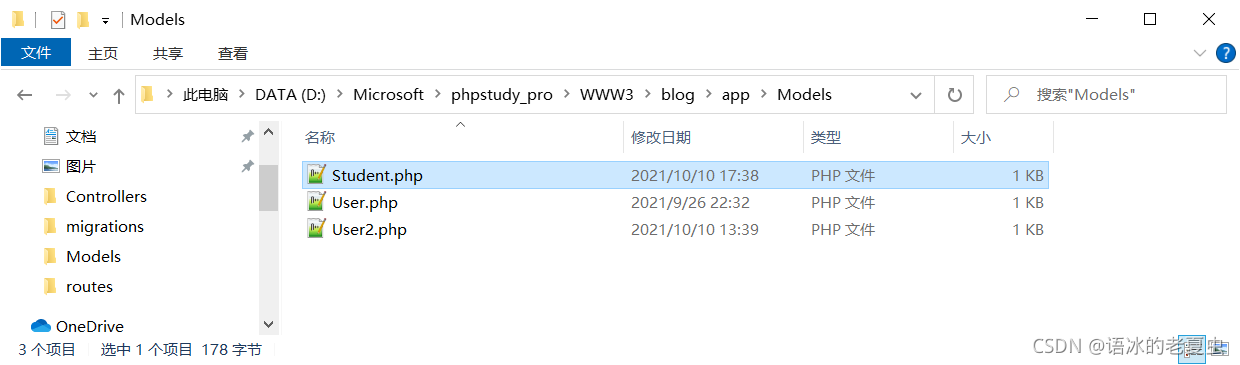 修改文件如下:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Student extends Model
{
use HasFactory;
protected $fillable = [
'name', 'detail'
];
}
(2)在文件夹D:\Microsoft\phpstudy_pro\WWW3\blog\database\migrations,自动创建迁移文件2021_10_10_093837_create_students_table.php。 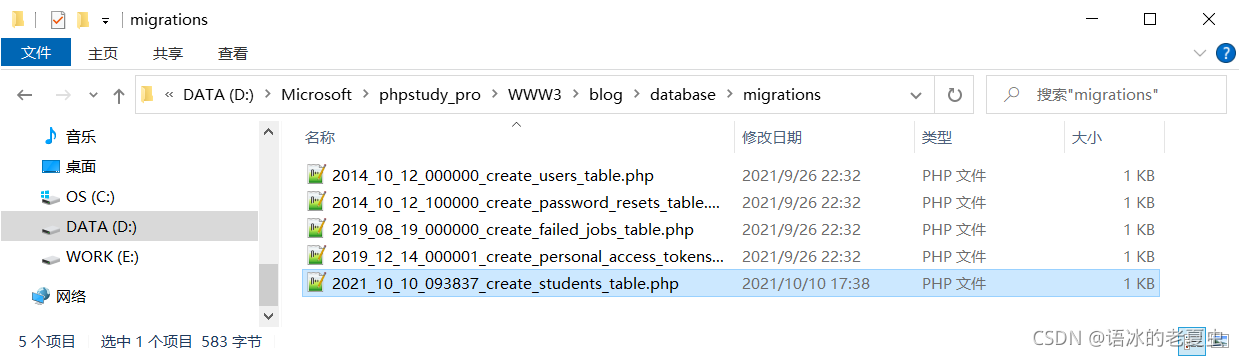 修改文件如下:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateStudentsTable extends Migration
{
public function up()
{
Schema::create('students', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('detail');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('students');
}
}
5.2 Create Factory
命令行输入命令:
php artisan make:factory StudentFactory --model=Student
 自动生成文件D:\Microsoft\phpstudy_pro\WWW3\blog\database\factories\StudentFactory.php。 文件修改为:
<?php
namespace Database\Factories;
use App\Models\Student;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
class StudentFactory extends Factory
{
protected $model = Student::class;
public function definition()
{
return [
'name' => $this->faker->name,
'detail' => $this->faker->text,
];
}
}
5.3 Fill Database
(1)composer dump-autoload (2)运行php artisan tinker (3)输入命令:
// 数据库中自动生成5条随机记录
App\models\Student::factory()->count(5)->create()
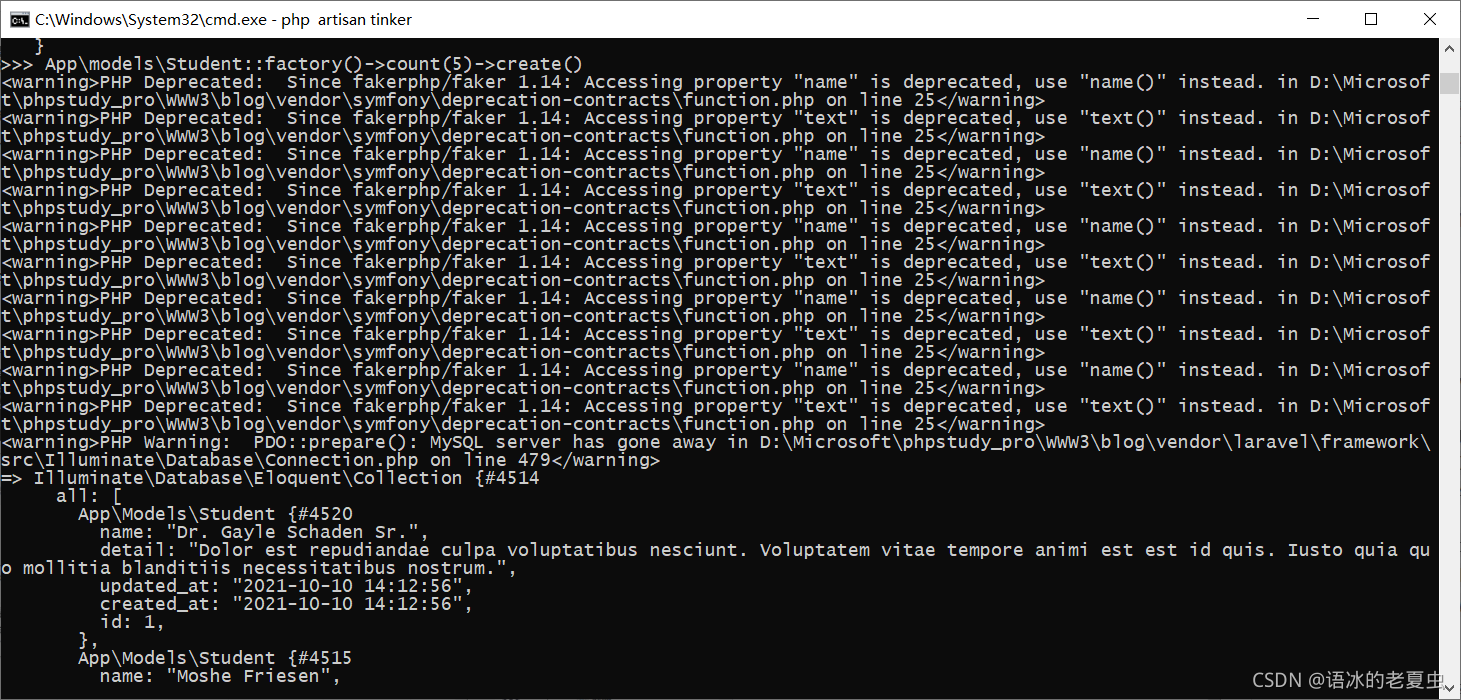 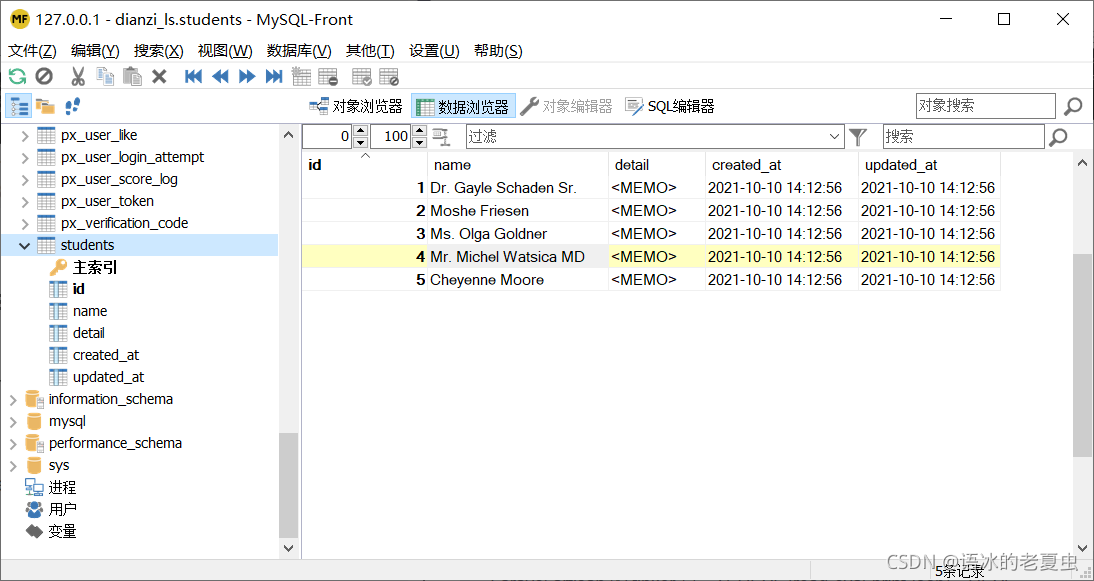 注意:如果数据库中的数据表Student没有自动生成的话,可能是迁移命令出错了。此时可以将文件夹D:\Microsoft\phpstudy_pro\WWW3\blog\database\migrations下除了2021_10_10_093837_create_students_table.php的其他文件移除掉再执行迁移命令php artisan migrate或php artisan migrate:refresh即可自动生成。 
5.4 另一种方式的迁移流程
(1)生成数据库迁移文件 创建生成数据表的迁移文件
php artisan make:migration create_order_table
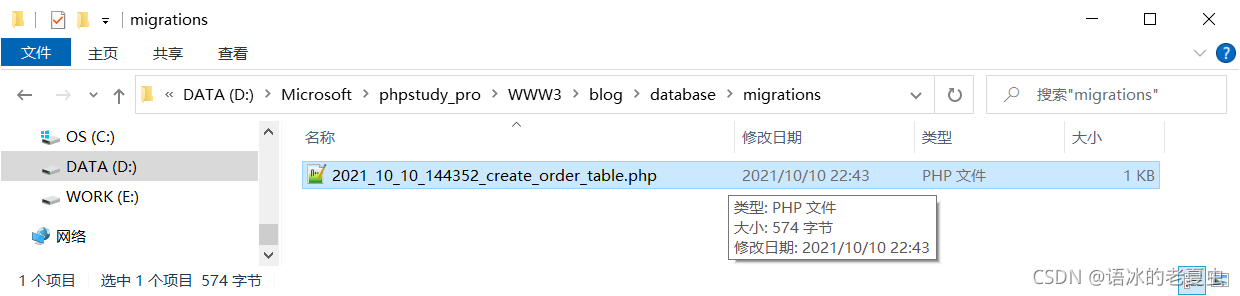 操作之后会在database/migrations/目录下生成创建表文件。 注意:在创建一张表的时候需要将其他的表文件移出文件夹,否则其他表也会同时运行一遍。 修改如下:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateOrderTable extends Migration
{
public function up()
{
Schema::create('order', function (Blueprint $table) {
$table->engine = "MyISAM";
$table->increments('user_id');
$table->string('user_name')->default('')->comment('//名称');
$table->integer('user_order')->default('0')->comment('//排序');
});
}
public function down()
{
Schema::dropIfExists('order');
}
}
(2)生成数据库的数据表 在写完需要创建的字段后,执行以下命令,建立数据表。
php artisan migrate
 (3)数据填充
php artisan make:seeder OrderTableSeeder
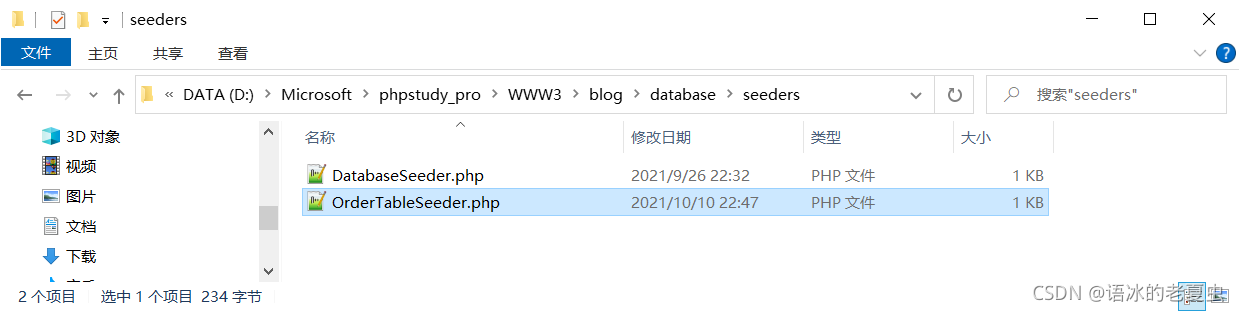 操作以上命令database/seeder/目录下生成OrderTableSeeder.php,包含了一个方法,run()添加数据方法,写法如下:
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Str;
class OrderTableSeeder extends Seeder
{
public function run()
{
DB::table('order')->insert([
'user_id' => mt_rand(1,1000),
'user_name' => \Str::random(10).'@gmail.com',
'user_order' => mt_rand(1,100)
]);
}
}
同时修改DatabaseSeeder.php的方法run()如下:
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
public function run()
{
$this->call([OrderTableSeeder::Class]);
$this->command->info('Order table seeded!');
}
}
执行命令: php artisan db:seed 或 php artisan db:seed --class=OrderTableSeeder 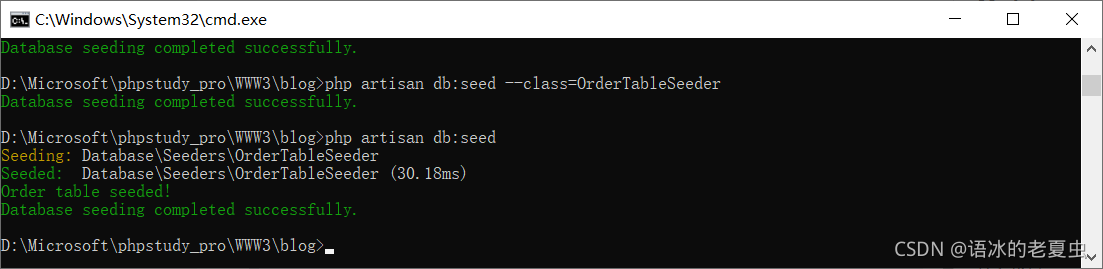 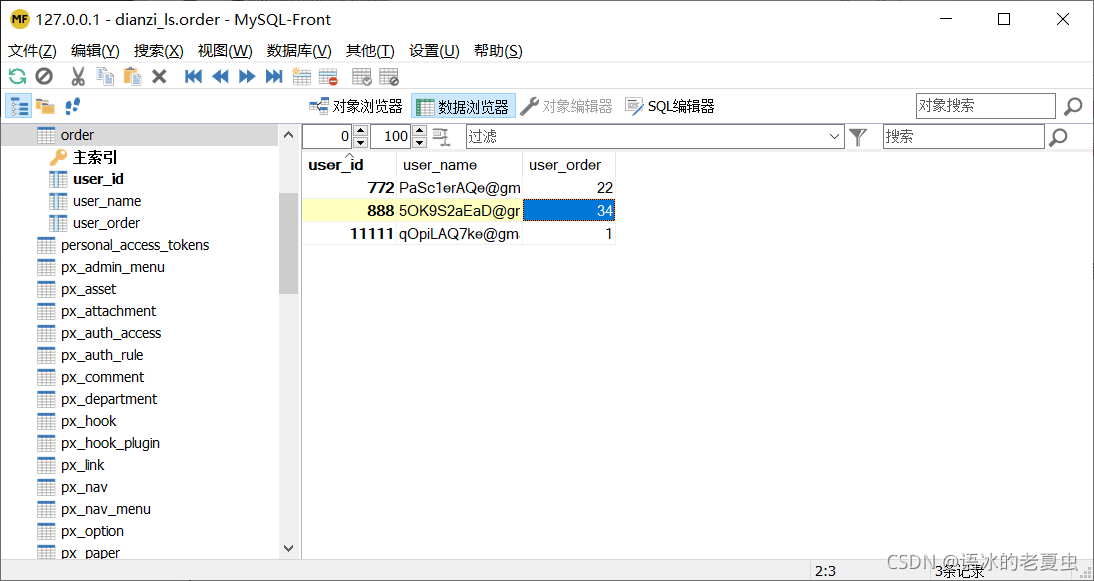 您也可以使用 命令填充数据库,将会回滚并重新运行所有迁移: php artisan migrate:refresh --seed 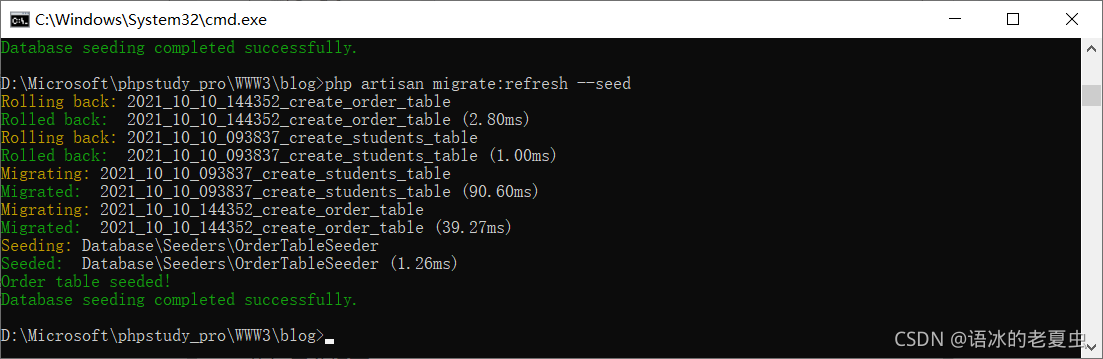 注意: 如果在运行迁移的时候收到一个 “class not found” 的错误,请尝试运行 composer dump-autoload 命令。
6、php artisan db:seed
创建填充 php artisan make:seeder StudentTableSeeder
执行单个填充 php artisan db:seed --class=StudentTableSeeder
执行所有填充 php artisan db:seed
7、php artisan tinker
Laravel artisan 的 tinker 是一个 REPL (read-eval-print-loop),REPL 是指交互式命令行界面,它可以让你输入一段代码去执行,并把执行结果直接打印到命令行界面里。>
7.1 运行tinker
cd D:\Microsoft\phpstudy_pro\WWW3\blog
php artisan tinker
 定义Model类,用于管理数据库中表px_user_score_log的数据:D:\Microsoft\phpstudy_pro\WWW3\blog\app\Models\User2.php 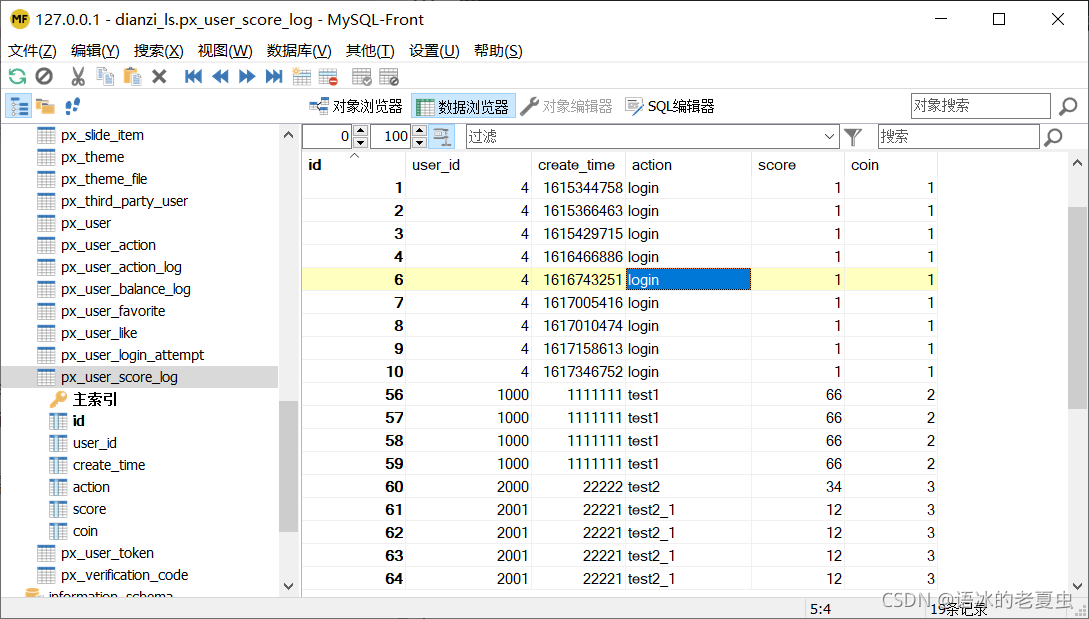
7.2 查询数据表的记录个数。
App\models\User2::count();
结果为19。 
7.3 查询数据表的满足条件的记录
App\models\User2::where('user_id', 2)->first();
结果为null。
App\models\User2::where('user_id', 4)->first();
结果为: App\Models\User2 {#3535 id: 1, user_id: 4, create_time: 1615344758, action: “login”, score: 1, coin: 1, } 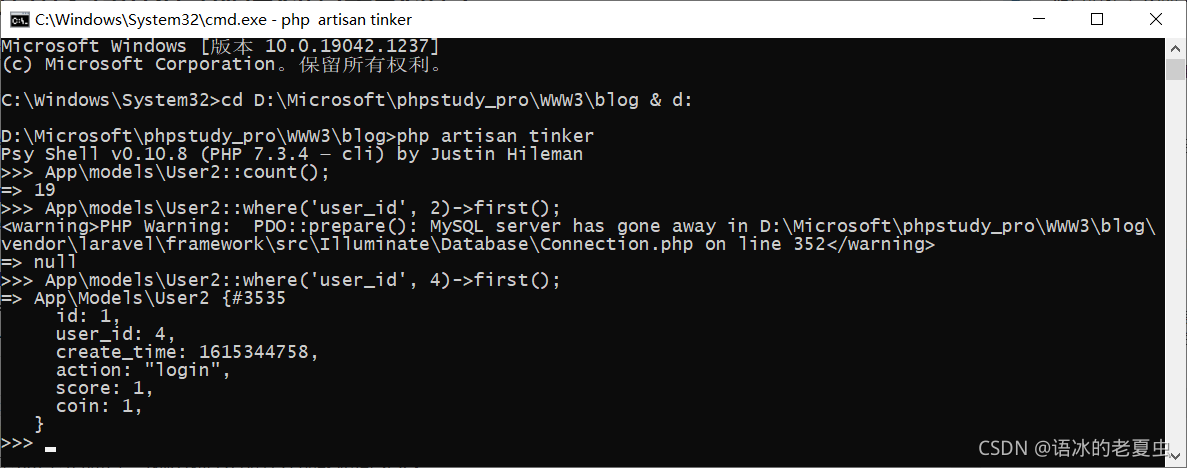
8、其他命令
创建中间件(app/Http/Middleware 下) php artisan make:middleware Activity
创建队列(数据库)的表迁移(需要执行迁移才生效) php artisan queue:table
创建队列类(app/jobs下): php artisan make:job SendEmail
创建请求类(app/Http/Requests下) php artisan make:request CreateArticleRequest
开启Auth用户功能(开启后需要执行迁移才生效) php artisan make:auth
后续
如果你觉得该方法或代码有一点点用处,可以给作者点个赞;╮( ̄▽ ̄)╭ 如果你感觉方法或代码不咋地//(ㄒoㄒ)//,就在评论处留言,作者继续改进。o_O??? 谢谢各位童鞋们啦( ′ ▽ )ノ ( ′ ▽> )っ!!!
|