对接paypal支付平台
【前言】:最近公司需要做一款海外股票的app,其中有需要购买会员权益的一个模块,这里需要国际类型的支付。支付宝及微信在国内比较活跃,国外的话可能不太理想,所以就用了paypal.
<?php
namespace Home\Controller;
require_once "Modules/Lib/PayPal-PHP-SDK/autoload.php";
use PayPal\Api\Amount;
use PayPal\Api\Details;
use PayPal\Api\Item;
use PayPal\Api\ItemList;
use PayPal\Api\Payer;
use PayPal\Api\Payment;
use PayPal\Api\RedirectUrls;
use PayPal\Api\Transaction;
use PayPal\Api\PaymentExecution;
use PayPal\Exception\PayPalConnectionException;
use PayPal\Rest\ApiContext;
use PayPal\Auth\OAuthTokenCredential;
class PayInfoController extends CommonController
{
const clientId = '';
const clientSecret = '';
const Currency = 'USD';
public static $accept_url = '';
protected $PayPal;
public function __construct() {
self::$accept_url = "http://".$_SERVER['SERVER_NAME'].'/wxapp.php?s=/PayInfo/Callback';
$this->PayPal = new ApiContext(
new OAuthTokenCredential(
self::clientId,
self::clientSecret
)
);
$this->PayPal->setConfig([
'mode' => 'sandbox',
]);
}
public function pay() {
$request = I("request.");
$interes_id = $request['interes_id'];
$price = $request['price'];
if(!$request['interes_id'] || !$request['price']){
echo json_encode(['code'=>1,'msg'=>'参数不全']);exit;
}
$shipping = 0;
$order = time().rand("1111,9999");
$product = '会员权益';
$description = '会员权益';
$paypal = $this->PayPal;
$total = $price + $shipping;
$payer = new Payer();
$payer->setPaymentMethod('paypal');
$item = new Item();
$item->setName($product)->setCurrency(self::Currency)->setQuantity(1)->setPrice($price);
$itemList = new ItemList();
$itemList->setItems([$item]);
$details = new Details();
$details->setShipping($shipping)->setSubtotal($price);
$amount = new Amount();
$amount->setCurrency(self::Currency)->setTotal($total)->setDetails($details);
$transaction = new Transaction();
$transaction->setAmount($amount)->setItemList($itemList)->setDescription($description)->setInvoiceNumber(uniqid());
$redirectUrls = new RedirectUrls();
$redirectUrls->setReturnUrl(self::$accept_url . "&success=true&orderId=$order&interes_id=$interes_id")
->setCancelUrl(self::$accept_url . "&success=false&orderId=$order");
$payment = new Payment();
$payment->setIntent('sale')->setPayer($payer)->setRedirectUrls($redirectUrls)->setTransactions([$transaction]);
try {
$payment->create($paypal);
} catch (PayPalConnectionException $e) {
echo json_encode(['code'=>1,'msg'=>$e->getData()]);exit;
}
$approvalUrl = $payment->getApprovalLink();
echo json_encode(['code'=>0,'msg'=>'success','url'=>$approvalUrl]);exit;
}
public function Callback() {
$success = trim($_GET['success']);
if ($success == 'false' && !isset($_GET['paymentId']) && !isset($_GET['PayerID'])) {
echo json_encode(['code'=>1,'msg'=>'支付失败','data'=>"取消付款"]);exit;
}
$paymentId = trim($_GET['paymentId']);
$PayerID = trim($_GET['PayerID']);
if (!isset($success, $paymentId, $PayerID)) {
echo json_encode(['code'=>1,'msg'=>"支付失败"]);exit;
}
if ((bool)$_GET['success'] === 'false') {
echo json_encode(['code'=>1,'msg'=>'支付失败']);exit;
}
$payment = Payment::get($paymentId, $this->PayPal);
$execute = new PaymentExecution();
$execute->setPayerId($PayerID);
try {
$payment->execute($execute, $this->PayPal);
} catch (\Exception $e) {
echo json_encode(['code'=>1,'msg'=>$e->getData()]);exit;
}
echo json_encode(['code'=>0,'msg'=>'支付成功']);
}
}
在paypal返回的地址直接web访问就可以进行测试,测试账号填写沙箱的个人账号就可以支付,支付后登录后台就可以看到是否成功付款。 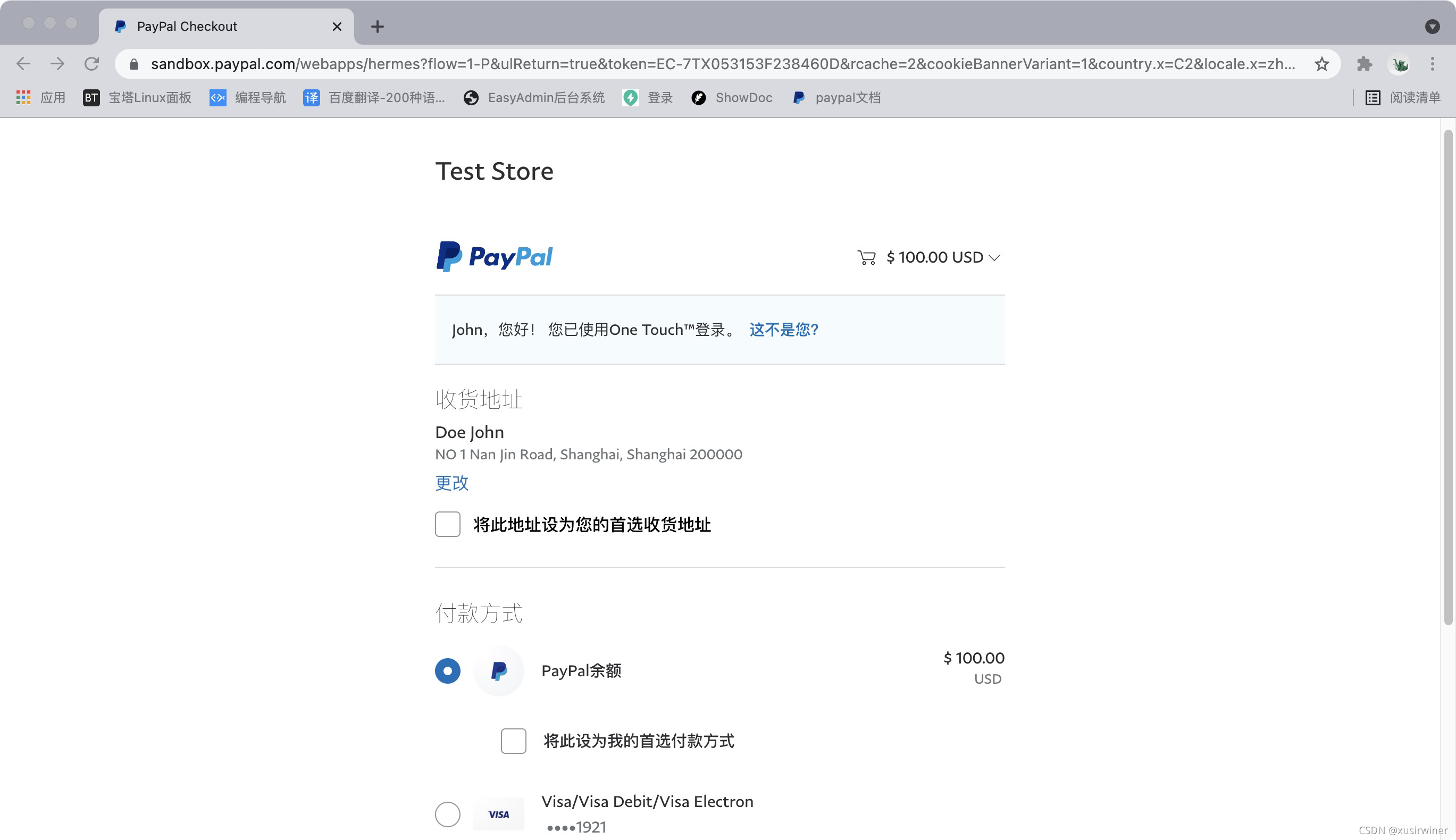
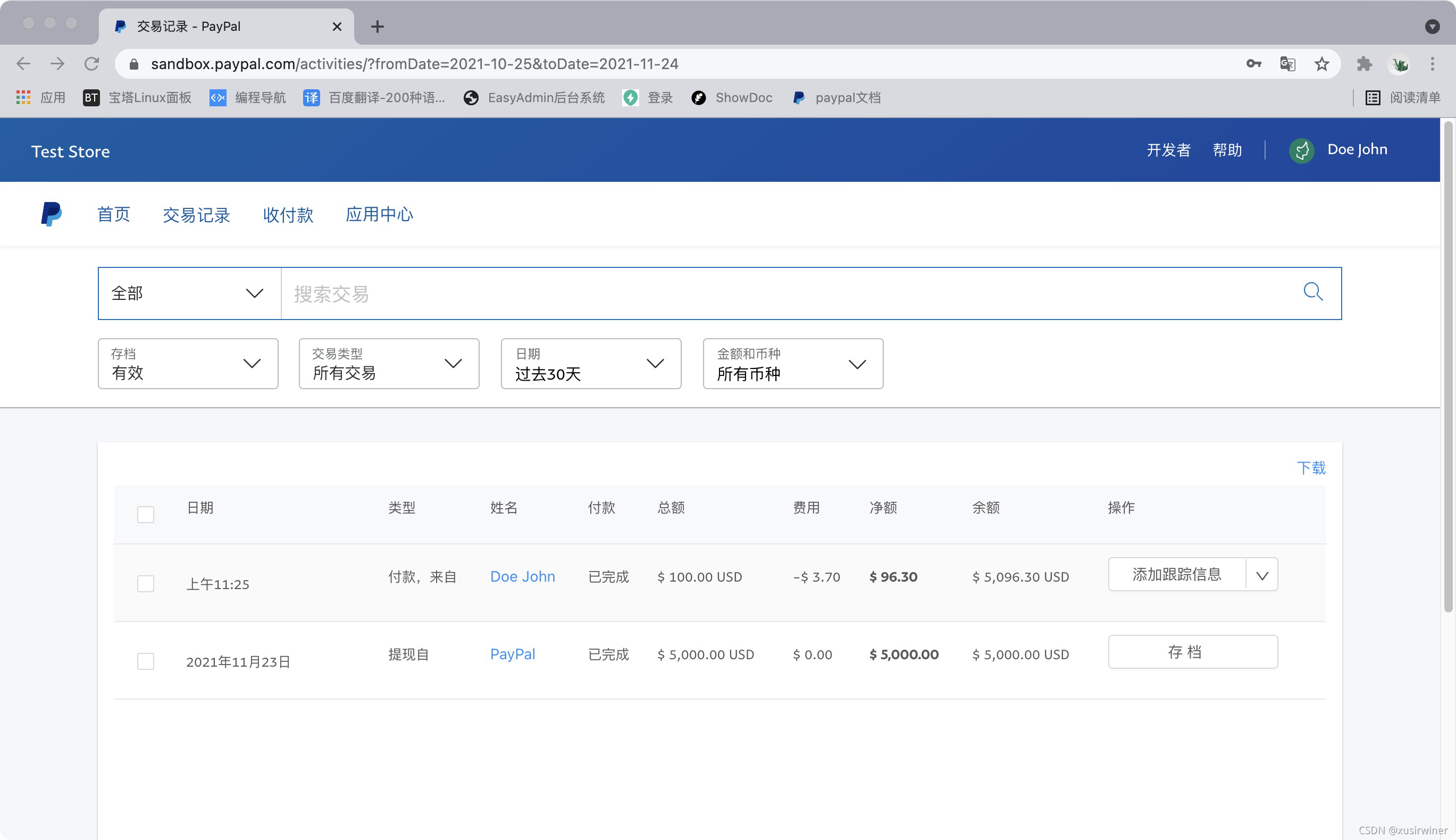
|