php+mysql实现简单的图书管理系统
ps:前端页面设计得有点丑,将就着用,哈哈哈。不具备用户和管理员功能,如要实现只需将首页的功能根据数据库中账号信息选择性使用就可以了。数据库关系设计什么的完全没考虑 (借图) 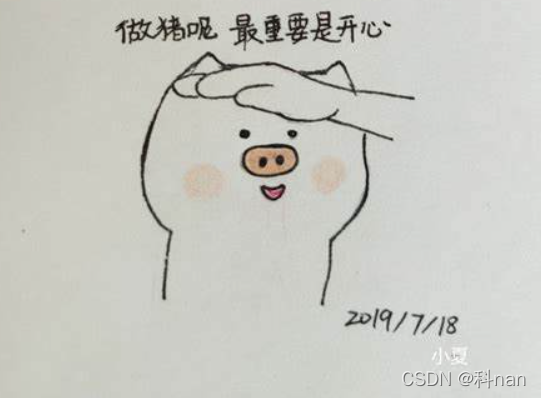
首页

代码
homepage.html
这个可以自己来
成为一员(类似于注册会员)
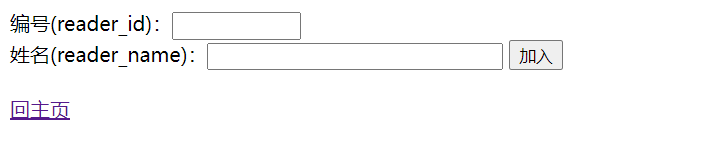 思路: html表单通过post方法向php文件传递两个参数,id和姓名,php文件接受数据后与数据库建立连接,向用户表中插入数据。用户表中available项用于标识是否有借阅资格,默认为1,借书超过期限后设置为0,下一次借书前会检查该项的值,如果为0将不会有资格借书
reader表 reader_id+reader_name+available
代码
readerinfo.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>to be a reader</title>
</head>
<body>
<form method="post" action="addreader.php">
编号(reader_id):<input type="text" name="id" size="10"><br>
姓名(reader_name):<input type="text" name="name" size="30">
<input type="submit" name="join" value="加入"><br><br>
<a href="homepage.html">回主页</a>
</form>
</body>
</html>
addreader.php
<?php
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$id = $_POST['id'];
$name = $_POST['name'];
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$mysql->set_charset('UTF-8');
$sql = "INSERT INTO reader (reader_id,reader_name) VALUES ('$id','$name')";
$result = $mysql->query($sql);
if ($result)
echo "<script>alert('Information added successfully!')</script>";
else echo "Failed to add, back to add again.<br>";
$mysql->close();
}
?>
<a href="readerinfo.html">继续加入</a> 或者 <a href="homepage.html">回到首页</a>
采购书籍
网页端 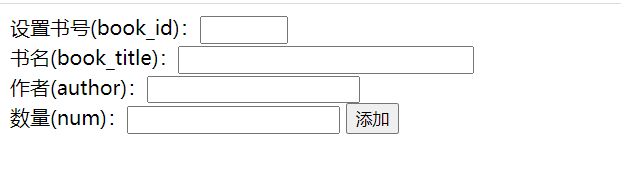 数据库中bookinfo表 book_id+book_title+author+on_shelf+num
on_shelf表示是否在架,添加时默认值为“是”
代码
addbook.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Add book</title>
</head>
<body>
<form method="post" action="addbook.php">
设置书号(book_id):<input type="text" name="id" size="5"><br>
书名(book_title):<input type="text" name="name" size="30"><br>
作者(author):<input type="text " name="author" size="20"><br>
数量(num):<input type="number" min="1" name="num">
<input type="submit" name="add" value="添加"><br>
</form>
</body>
</html>
addbook.php
<?php
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$mysql = new mysqli($host, $username, $password, $dbname);
$title = $_POST['name'];
$title_id = $_POST['id'];
$author = $_POST['author'];
$num = $_POST['num'];
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$sql = "INSERT into bookinfo (book_id,book_title,author,num)
values ('$title_id','$title','$author',$num)";
$result = $mysql->query($sql);
if ($result)
echo "<script>alert('添加成功')</script>";
else echo "Failed to add, back to add again.<br>";
$mysql->close();
}
?>
<br>
<a href="addbook.html">返回继续添加</a> or <a href="homepage.html">返回首页</a>
查看书籍
思路: 在php文件中加入html内容,用于将查询到的数据以表格的形式显示出来
代码
allbook.php
<?php
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$sql = "select * from bookinfo";
$result = $mysql->query($sql);
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>All Information</title>
</head>
<body>
<table width="800px" border="1" style="border-collapse: collapse;">
<thead>
<th>书号</th>
<th>书名</th>
<th>作者</th>
<th>是否可借</th>
<th>现存量</th>
</thead>
<?php
while ($row = mysqli_fetch_row($result)) {
?>
<tr>
<td align="center"><?php echo $row[0]; ?></td>
<td align="center"><?php echo $row[1]; ?></td>
<td align="center"><?php echo $row[2]; ?></td>
<td align="center"><?php echo $row[3]; ?></td>
<td align="center"><?php echo $row[4]; ?></td>
</tr>
<?php
}
?>
</table>
<br>
<a href="homepage.html">返回首页</a>
</body>
</html>
借阅书籍
思路: 首先根据读者id确认是否有资格进行借阅,readerinfo表中不存在记录或者存在记录但available项为0时,没有资格借阅,将回到主页,可以选择成为一员功能添加用户信息,再进行借阅,但是对于借书不还的来添加信息后继续借阅,没办法,哎。若有资格将进入借阅页面。为了避免重复输入id,使用php的session函数将检测时传递的id保存,向数据库表b_r插入数据时再用session获得该id,在文件最后清除这个id缓存。 如果借书的时候,在架书籍现存量为0,就会提示无法借阅,如果恰有一本,需要将书本的现存量减1,将是否可借调整为否 表b_r用于记录借阅情况,start_date用于记录借阅开始时间,默认为当下时间:CURRENT_TIMESTAMP reader_id+book_id+start_date+keep_time
资格检查 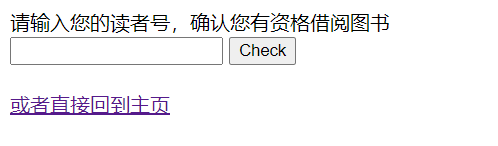 没资格 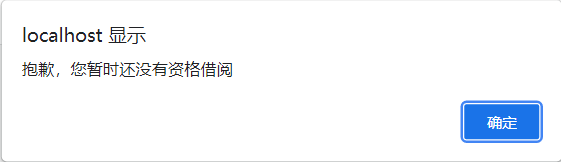 有资格 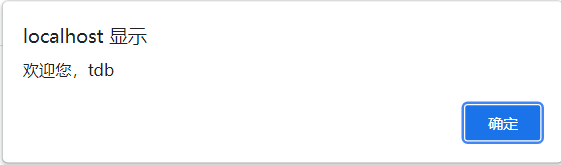  现存量为0时进行借阅的提示,然后回到主页 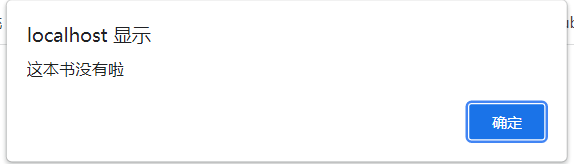
代码
borrowbook.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>借书</title>
<style type="text/css">
h1{
background-color: #678;
color:white;
text-align: center;
}
body{
height: 100%;
width: 100%;
border: none;
overflow-x: hidden;
}
div{
width:100%;
text-align: center;
}
</style>
</head>
<body>
<h1>借书</h1>
<div>
<form method="post" action="borrowbook.php">
借阅书号:<input type="text" name="book_id"/>
借阅时间(天):<input type="number"min="0" name="keep_time">
<input type="submit" value="借书"/>
</form>
</div>
</body>
</html>
borrowbook.php
<?php
session_start();
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$id=$_SESSION['id'];
$book_id=$_POST['book_id'];
$keep=$_POST['keep_time'];
$url1="homepage.html";
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else{
$sql1="SELECT num from bookinfo where book_id=$book_id";
$result1=$mysql->query($sql1);
$row1=mysqli_fetch_row($result1);
if($row1[0]==0){
echo "<script>alert('这本书没有啦')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}
if($row1[0]==1){
$sql2="UPDATE bookinfo SET num=0,on_shelf='否' where book_id='$book_id'";
$sql3="INSERT INTO b_r(reader_id,book_id,keep_time) VALUES($id,$book_id,$keep)";
$result2=$mysql->query($sql2);
$result3=$mysql->query($sql3);
if($result2&&$result3){
echo "<script>alert('借阅成功')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}else{
echo "<script>alert('借阅失败')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}
}
if($row1[0]>1){
$sql4="INSERT INTO b_r(reader_id,book_id,keep_time) VALUES($id,$book_id,$keep)";
$sql5="UPDATE bookinfo SET num=num-1 where book_id='$book_id'";
$result4=$mysql->query($sql4);
$result5=$mysql->query($sql5);
if($result4&&$result5){
echo "<script>alert('借阅成功')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
}
else{
echo "<script>alert('借阅失败')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
}
}
}
$mysql->close();
unset($_SESSION['id']);
?>
淘汰书籍
bookinfo表中的book_id唯一确定一本书,根据id号进行淘汰,如果要淘汰的数量超过了现存量,现存量改为0,on_shelf改为否 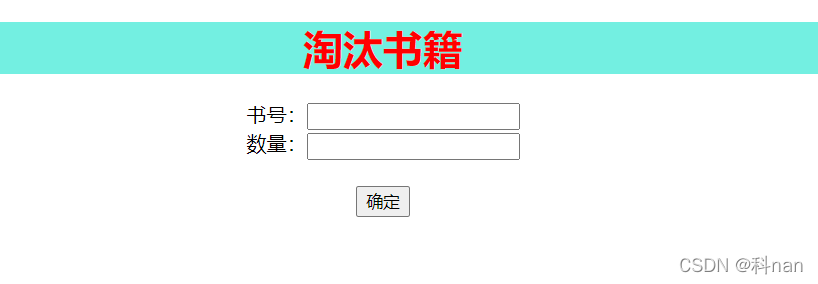
代码
delete.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>删除</title>
<style>
h1{
background-color: #73EFE1;
color:red;
text-align: center;
}
</style>
</head>
<body>
<form onSubmit="return submit_sure()" method="post" action="delete.php" >
<h1>淘汰书籍</h1>
<div align="center">
书号:<input type="text" name="id" size="20" ><br>
数量:<input type="number" min="0" size="10" name="num">
<br><br>
<input type="submit" value="确定">
</div>
</form>
</body>
</html>
<script language="javascript">
function submit_sure(){
var gnl=confirm("确定删除?");
if(gnl==true){
return true;
}else{
return false;
}
}
</s
delete.php
<?php
$host = 'localhost';
$username = ';
$password = '';
$dbname = '';
$id = $_POST['id'];
$num = $_POST['num'];
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$sql0="SELECT * from bookinfo where book_id=$id";
$result0=$mysql->query($sql0);
$row = mysqli_fetch_row($result0);
if($row[4]<=$num){
$sql1 = "UPDATE bookinfo SET num=0,on_shelf='否' WHERE book_id=$id";
$result1=$mysql->query($sql1);
if ($result1) {
echo "<script>alert('操作完成')</script>";
} else {
echo "<script>alert('操作失败')</script>";
}
}else{
$sql2="UPDATE bookinfo SET num=num-$num WHERE book_id=$id";
$result2=$mysql->query($sql2);
if ($result2)
{
echo "<script>alert('操作完成')</script>";
} else
{
echo "<script>alert('操作失败')</script>";
}
}
$mysql->close();
}
?>
<a href="delete.html">继续</a> 或者 <a href="homepage.html">回到首页</a>
归还书籍
还书时需要根据book_id检测这本书有没有被人借阅,不同情况会进行提示。归还成功时需要将b_r表记录删除,同时若bookinfo表的on_shelf项为否,需改为是,然后将现存量加1 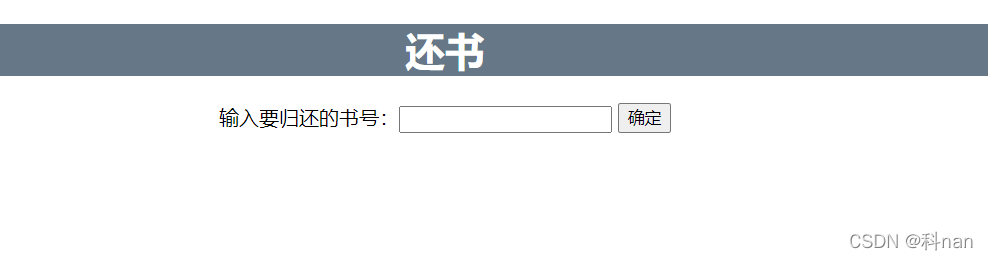 没有被借时提示 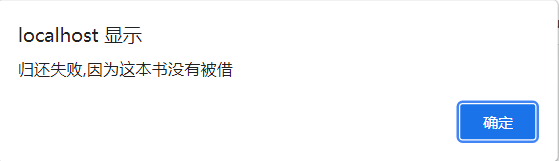 在借时提示 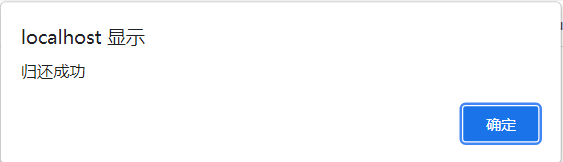
代码
returnbook.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>还书</title>
<style>
h1{
background-color: #678;
color:white;
text-align: center;
}
</style>
</head>
<body>
<h1>还书</h1>
<div align="center">
<form method="post" action="return.php">
输入要归还的书号:<input type="text" name="id">
<input type="submit" value="确定">
</form>
</div>
</body>
</html>
return.php
<?php
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$id = $_POST['id'];
$url1 = "homepage.html";
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$sql1 = "SELECT book_id from b_r where book_id=$id";
$result1 = $mysql->query($sql1);
$row = mysqli_fetch_row($result1);
if ($result1 && $row[0] == $id) {
$sql2 = "DELETE from b_r where book_id=$id";
$result2 = $mysql->query($sql2);
$sql3 = "SELECT on_shelf,num from bookinfo where book_id=$id";
$result3 = $mysql->query($sql3);
$row1 = mysqli_fetch_row($result3);
if ($row1[0] == '否' || $row1[1] == 0) {
$sql4 = "UPDATE bookinfo SET on_shelf='是',num=num+1 where book_id=$id";
$result4 = $mysql->query($sql4);
if ($result4) {
echo "<script>alert('归还成功')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
} else {
echo "<script>alert('归还失败')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}
} else {
$sql5 = "UPDATE bookinfo SET num=num+1 where book_id=$id";
$result5 = $mysql->query($sql5);
if ($result5) {
echo "<script>alert('归还成功')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
} else {
echo "<script>alert('归还失败')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}
}
} else {
echo "<script>alert('归还失败,因为这本书没有被借')</script>";
echo "<script language='javascript' type='text/javascript'>";
echo "window.location.href='$url1'";
echo "</script>";
$mysql->close();
}
}
借阅情况
跟查看书籍相似,将b_r表数据以表格的形式显示
代码
Borrow.php
<?php
$host = 'localhost';
$username = '';
$password = '';
$dbname = '';
$mysql = new mysqli($host, $username, $password, $dbname);
if ($mysql->connect_errno) {
die('数据库连接失败:' . $mysql->connect_errno);
} else {
$sql = "select * from b_r";
$result = $mysql->query($sql);
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>All Information</title>
</head>
<body>
<table width="800px" border="1" style="border-collapse: collapse;">
<thead>
<th>读者编号</th>
<th>书号</th>
<th>借阅时间</th>
<th>借阅天数</th>
</thead>
<?php
while ($row = mysqli_fetch_row($result)) {
?>
<tr>
<td align="center"><?php echo $row[0]; ?></td>
<td align="center"><?php echo $row[1]; ?></td>
<td align="center"><?php echo $row[2]; ?></td>
<td align="center"><?php echo $row[3]; ?></td>
</tr>
<?php
}
?>
</table>
<br>
<a href="homepage.html">返回首页</a>
</body>
</html>
工具
- XAMPP
很好用且免费,下载地址, 附 一篇安装和配置的博文 - Dreamweaver
很好用但正版要付费 - SQLyog
一个好用的数据库管理软件,下载地址
|