<?php
namespace app\api\controller;
use app\api\controller\Common;
use think\Db;
use think\Image;
class ImageTools extends Common
{
public function _initialize()
{
}
public function radiusImage($imgpath,$imageResouce)
{
$im = imagecreatetruecolor(372, 226);
$bgcolor = imagecolorallocate($im, 255, 255, 255);
imagefill($im, 0, 0, $bgcolor);
$radius = 20;
$img = imagecreatefromstring($imageResouce);
imagecopy($im, $img, 0, 0, 0, 0, 372, 226);
$lt_corner = $this->get_lt_rounder_corner($radius, 0xef, 0xef, 0xe1);
$this->myradus($im, 0, 0, $lt_corner, $radius, 226, 372);
imagepng($im, $imgpath);
imagedestroy($img);
}
public function imagebackgroundmycard($im, $dst_x, $dst_y, $image_w, $image_h, $radius)
{
$resource = imagecreatetruecolor($image_w, $image_h);
$bgcolor = imagecolorallocate($resource, 0xef, 0xef, 0xe1);
imagefill($resource, 0, 0, $bgcolor);
$lt_corner = $this->get_lt_rounder_corner($radius, 255, 255, 255);
imagecopymerge($resource, $lt_corner, 0, 0, 0, 0, $radius, $radius, 100);
$lb_corner = imagerotate($lt_corner, 90, 0);
imagecopymerge($resource, $lb_corner, 0, $image_h - $radius, 0, 0, $radius, $radius, 100);
$rb_corner = imagerotate($lt_corner, 180, 0);
imagecopymerge($resource, $rb_corner, $image_w - $radius, $image_h - $radius, 0, 0, $radius, $radius, 100);
$rt_corner = imagerotate($lt_corner, 270, 0);
imagecopymerge($resource, $rt_corner, $image_w - $radius, 0, 0, 0, $radius, $radius, 100);
imagecopy($im, $resource, $dst_x, $dst_y, 0, 0, $image_w, $image_h);
}
public function get_lt_rounder_corner($radius, $color_r, $color_g, $color_b)
{
$img = imagecreatetruecolor($radius, $radius);
$bgcolor = imagecolorallocate($img, 255, 255, 255);
$fgcolor = imagecolorallocate($img, 0, 0, 0);
imagefill($img, 0, 0, $bgcolor);
imagefilledarc($img, $radius, $radius, $radius * 2, $radius * 2, 180, 270, $fgcolor, IMG_ARC_PIE);
imagecolortransparent($img, $fgcolor);
return $img;
}
public function myradus($im, $lift, $top, $lt_corner, $radius, $image_h, $image_w)
{
imagecopymerge($im, $lt_corner, $lift, $top, 0, 0, $radius, $radius, 100);
$lb_corner = imagerotate($lt_corner, 90, 0);
imagecopymerge($im, $lb_corner, $lift, $image_h - $radius+$top, 0, 0, $radius, $radius, 100);
$rb_corner = imagerotate($lt_corner, 180, 0);
imagecopymerge($im, $rb_corner, $image_w + $lift - $radius, $image_h + $top - $radius, 0, 0, $radius, $radius, 100);
$rt_corner = imagerotate($lt_corner, 270, 0);
imagecopymerge($im, $rt_corner, $image_w - $radius + $lift, $top, 0, 0, $radius, $radius, 100);
}
public function circleImg($imgpath,$imageResouce){
$ext = pathinfo($imgpath);
$src_img = null;
switch ($ext['extension']) {
case 'jpg':
$src_img = imagecreatefromstring($imageResouce);
break;
case 'png':
$src_img = imagecreatefromstring($imageResouce);
break;
}
$wh = getimagesize($imgpath);
$w = $wh[0];
$h = $wh[1];
$w = min($w, $h);
$h = $w;
$img = imagecreatetruecolor($w, $h);
imagesavealpha($img, true);
$bg = imagecolorallocatealpha($img, 255, 255, 255, 127);
imagefill($img, 0, 0, $bg);
$r = $w / 2;
$y_x = $r;
$y_y = $r;
for ($x = 0; $x < $w; $x++) {
for ($y = 0; $y < $h; $y++) {
$rgbColor = imagecolorat($src_img, $x, $y);
if (((($x - $r) * ($x - $r) + ($y - $r) * ($y - $r)) < ($r * $r))) {
imagesetpixel($img, $x, $y, $rgbColor);
}
}
}
imagepng($img,$imgpath);
imagedestroy($img);
}
public function shareImage()
{
$host = "http://xiaoshiliu.com/";
$type = input('type',1);
$gonsis_id = input('id') ? input("id") : 81;
$info = $this->getFind('gonsis',array('id'=>$gonsis_id),'title,content,uid,images');
$userinfo = $this->getFind('user',array('id'=>$info['uid']),'user_name,avatar');
if(!$info || !$userinfo)$this->ajaxReturn(1,'信息异常',[$info,$userinfo]);
$type = $info['images'] ? 1 : 2;
if($info['images'] !== '[]'){
$info['images'] = json_decode($info['images'])[0];
}
$root = ROOT_PATH;
$back_path = $root."public/uploads/back_code/{$gonsis_id}.png";
if(is_file($back_path))@unlink($back_path);
$path = $root."public/uploads/vcode/{$gonsis_id}.png";
if(!is_file($path)){
$qrcode = $this->getWxCode('id='.$gonsis_id,'pages/continfo/continfo',97);
if(!$qrcode['resource'] || isset($qrcode['resource']['data']))return array('status'=>0,'msg'=>'二维码生成失败','data'=>$qrcode['data']);
$qrcode = $qrcode['resource'];
$file = fopen($path,'w+');
fwrite($file,$qrcode);
fclose($file);
$path_thumb = Image::open($path);
$path_thumb->thumb(97,97,$path_thumb::THUMB_SCALING);
$path_thumb->save($path);
$this->circleImg($path,file_get_contents($host."public/uploads/vcode/{$gonsis_id}.png"));
}
$backimg = $root.'/public/static/mobile/image/back.png';
$image = Image::open($backimg);
$size = 20;
$font = $root.'/public/static/api/microsoftyahei.ttf';
$font_1 = $root.'/public/static/api/fangzheng.ttf';
$font_2 = $root.'/public/static/api/bold.TTF';
$font_3 = $root.'/public/static/api/EXTRALIGHT.ttf';
$font_4 = $root.'/public/static/api/HEAVY.ttf';
$font_5 = $root.'/public/static/api/LIGHT.ttf';
$font_6 = $root.'/public/static/api/medium.TTF';
$font_7 = $root.'/public/static/api/REGULAR.ttf';
$color = '#bb560a';
list($back_width,$back_height) = $image->size();
$ad_text = "你的见识与品位值得引起更多人的关注";
$ad_text_size = imagettfbbox(20, 0, $font_1,$ad_text);
$ad_width = $ad_text_size[4] - $ad_text_size[6];
$ad_x = (750 - $ad_width)/2+10;
$image->text($ad_text,$font_1,20,$color,[$ad_x,185]);
$hea_pic_default = $host.'public/static/mobile/image/tx.png';
$hea_pic = $userinfo['avatar'];
if($hea_pic){
if(!strstr($hea_pic,'http')){
$hea_pic = $host.$hea_pic;
}
}else{
$hea_pic = $hea_pic_default;
}
$head_pic_path = $root."public/uploads/vcode/{$gonsis_id}_headpic.png";
$head_pic = fopen($head_pic_path,'w+');
fwrite($head_pic,file_get_contents($hea_pic));
fclose($head_pic);
$avatar_thumb = Image::open($head_pic_path);
$avatar_thumb = $avatar_thumb->thumb(105,105,$avatar_thumb::THUMB_CENTER);
$avatar_thumb->save($head_pic_path);
$this->circleImg($head_pic_path,file_get_contents($host."public/uploads/vcode/{$gonsis_id}_headpic.png"));
$image->water($head_pic_path,[325,335]);
@unlink($head_pic_path);
$title_text = $info['title'];
$title_width_area = 485;
$title_size = imagettfbbox($size, 0, $font_2, $title_text);
$title_width = $title_size[4] - $title_size[6];
$title_height = $title_size[3] - $title_size[5];
if($title_width > $title_width_area){
$title_length = mb_strlen($info['title']);
$ll = bcmul($title_length,(bcdiv($title_width_area,$title_width,2)));
$title_text = substr($info['title'],0,$ll);
$title_size = imagettfbbox($size, 0, $font_2, $title_text);
$title_width = $title_size[4] - $title_size[6];
$title_height = $title_size[3] - $title_size[5];
}
$ttile_x = bcdiv(bcsub($back_width,$title_width),2);
$title_y = 470;
$image->text($title_text,$font_2,22,$color,array($ttile_x,$title_y));
$content_width = 440;
$result = $this->autowrap(20,0,$font_6,$info['content'],$content_width);
$x = ($back_width - 440)/2 + 10;
$y = 470+10;
$line_height = $type == 2 ? 50 : 40;
foreach ($result['array'] as $k1=>$v1) {
if($type == 1 && $k1 > 2)continue;
if($type == 2 && $k1 > 4)continue;
$y += $line_height;
if($k1 == 2 ||$k1 == 4){
$v1 = substr($v1,0,-6).'...';
}
$image = $image->text($v1, $font_6, 20, $color, [$x, $y]);
}
$y +=50;
$username = $this->getValue('user',array('id'=>$info['uid']),'user_name');
$nickname = '-- '.$username;
$name_text_size = imagettfbbox(20,0,$font_2,$nickname);
$name_width = $name_text_size[4] - $name_text_size[6];
$name_x = (750 - $name_width - 140);
$image->text($nickname,$font,$size,$color,[$name_x,$y]);
$y+=50;
if($type == 1){
$content_height = 920;
$content_pic = $root.$info['images'];
$image_thumb = Image::open($content_pic);
$image_thumb->thumb(372,226,$image_thumb::THUMB_CENTER);
$thumb_url = $root.'public/uploads/back_code/back_thumb.jpg';
$image_thumb->save($thumb_url);
$this->radiusImage($thumb_url,file_get_contents($host.'public/uploads/back_code/back_thumb.jpg'));
$image_x = (750 - 372)/2 + 39;
$image->water($thumb_url,[$image_x,$y]);
@unlink($thumb_url);
}
$back_code_path = $root.'public/static/mobile/image/code_back.png';
$back_code = Image::open($back_code_path);
list($back_w,$back_h) = $back_code->size();
$back_code->rotate(2);
$back_code_path = $root.'public/uploads/back_code/code_back1.png';
$back_code->save($back_code_path);
$back_code_x = 750 - $back_w - 30;
$image->water($back_code_path,[$back_code_x,810]);
@unlink($back_code_path);
$image->water($path,[575,920]);
$image->save($back_path);
$url = $host."public/uploads/back_code/{$gonsis_id}.png";
echo '<img src="'.$url.'" style="padding:10px;width:750px">';
}
public function autowrap($fontsize, $angle, $fontface, $string, $width)
{
$content = "";
preg_match_all("/./u", $string, $arr);
$letter = $arr[0];
$num = 0;
$arr = [];
foreach ($letter as $k=>$l) {
if($num > 5)continue;
$teststr = $content . $l;
$testbox = imagettfbbox($fontsize, $angle, $fontface, $teststr);
if (($testbox[2] > $width) && ($content !== "")) {
$content .= PHP_EOL;
$num++;
}
$content .= $l;
}
$array = explode(PHP_EOL,$content);
$result['num'] = $num + 1;
$result['array'] = $array;
$result['content'] = $content;
return $result;
}
}
效果图 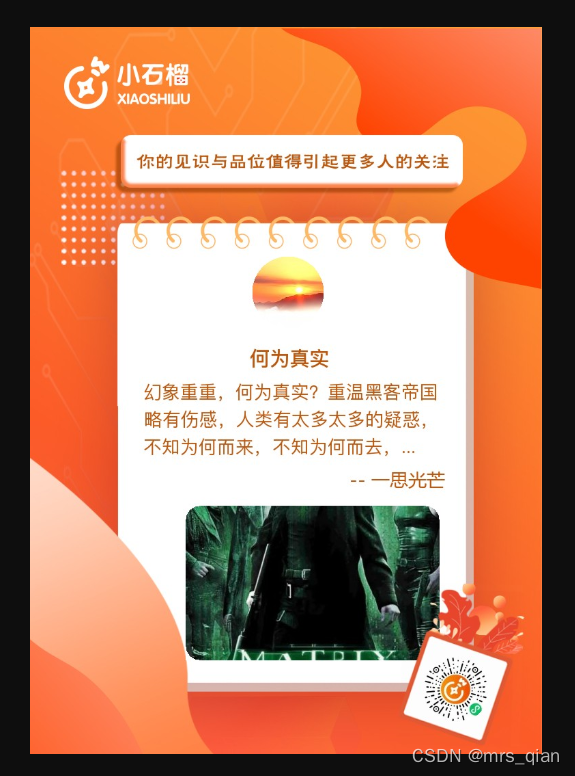
|