1.在项目中的 composer.json 文件中添加 JSMS 依赖:
"require": {
"jiguang/jsms": "~1.0"
}
2. 执行composer update
composer update
3.在app.php中配置全局变量,极光的一些参数
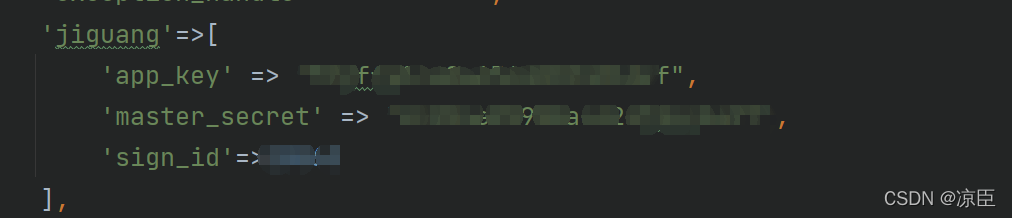
?4.封装发送短信函数
<?php
/*发送短信*/
namespace app\common\facade;
use JiGuang\JSMS;
use think\facade\Config;
class SendMs
{
public static function sendmsg($phone, $content)
{
$s=Config::get();
$app_key = $s['app']['jiguang']['app_key'];
$master_secret = $s['app']['jiguang']['master_secret'];
$sign_id= $s['app']['jiguang']['sign_id'];
$client = new JSMS($app_key, $master_secret, [ 'disable_ssl' => true ]);
$temp_id = '1';
$temp_para = ['code' => $content];
//单条短信发送
//参数说明:
//$phone: 接收验证码的手机号码
//$temp_id: 模板 ID,这个是需要去极光平台自己创建模板的
//$temp_para: 模板参数,需要替换的参数名和 value 的键值对,仅接受数组类型的值
//$time: 定时短信发送时间,格式为 yyyy-MM-dd HH:mm:ss,默认为 null 表示立即发送
//$sign_id: 签名ID,null 表示使用应用默认签名
$res = $client->sendMessage($mobile=$phone, $temp_id, $temp_para, $time = null,$sign_id);
// 判断请求是否发送失败
if(empty($res['body']['error'])) {
// 短信接口调用成功
return true;
} else {
// 短信接口调用失败
return $res['body']['error']['message'];
}
}
}
5.封装发送验证码和验证的函数
<?php
namespace app\common\controller;
use app\common\controller\Base;
use app\common\facade\SendMs;
use app\common\model\AppSendMs;
use think\facade\Cache;
class SendCode extends Base
{
public function send($phone,$type)
{
/*不同的类型使用不同的方法*/
$type_time=$type.'_time_';
$type_code=$type.'_code_';
$param['phone']=$phone;
$validate = $this->validate($param, [
'phone'=>'require|regex:1[3-9]\d{9}'
]);
if($validate !== true) {
$res = [
'code' => 400,
'msg' => '手机号格式不正确'
];
return $res;
}
// 获取上一次发送验证码的时间
$last_time = Cache::store('redis')->get($type_time . $phone);
// 判断当前发送验证码的时间减去上一次发送验证码的时间,是否小于 5
if((time() - $last_time) <60) {
$res = [
'code' => 400,
'msg' => '发送频繁,稍后再试'
];
return $res;
}
// 发送验证码 (生成验证码、生成短信内容、发送短信)
$code = mt_rand(1000, 9999);
$result = SendMs::sendmsg($phone, $code); // 为了不减少第三方短信接口的发送次数,这里暂时屏蔽,开发完毕后自行打开
// 将结果存到数据库中,不管是否成功
AppSendMs::create(['phone'=>$phone,'result'=>$result,'code'=>$code,'type'=>$type]);
if($result === true) {
// 将验证码存储到缓存,用于后续的验证
Cache::store('redis')->set($type_code . $phone, $code, 300);
// 将当前发送验证码的时间存到缓存,用于防止用户频繁发送验证码
Cache::store('redis')->set($type_time . $phone, time(), 300);
// 返回成功结果
$res = [
'code' => 200,
'msg' => '短信发送成功'
];
return $res;
} else {
// 返回失败结果
$res = [
'code' => 400,
'msg' => $result
];
return $res;
}
}
public function check($phone,$type,$code)
{
// 验证码校验
$type_code=$type.'_code_';
$s = Cache::store('redis')->get($type_code .$phone);
if($code != $s) {
return ['code' => 204 , 'msg' => '验证码错误'];
}
// 验证码校验成功一次后,将当前缓存中对应手机号的验证码删除
Cache::store('redis')->set($type_code .$phone, null);
return ['code' => 20000 , 'msg' => '成功'];
}
}
6.调用函数发送验证码
$SendCode=new SendCode();
$s=$SendCode->send($phone,'register');
7.验证验证码的准确性
// 验证码校验
$SendCode=new SendCode();
$s=$SendCode->check($phone,'register',$code);
if ($s['code']!=20000){
return $s;
}
|