Stripe支付 :https://dashboard.stripe.com/dashboard
?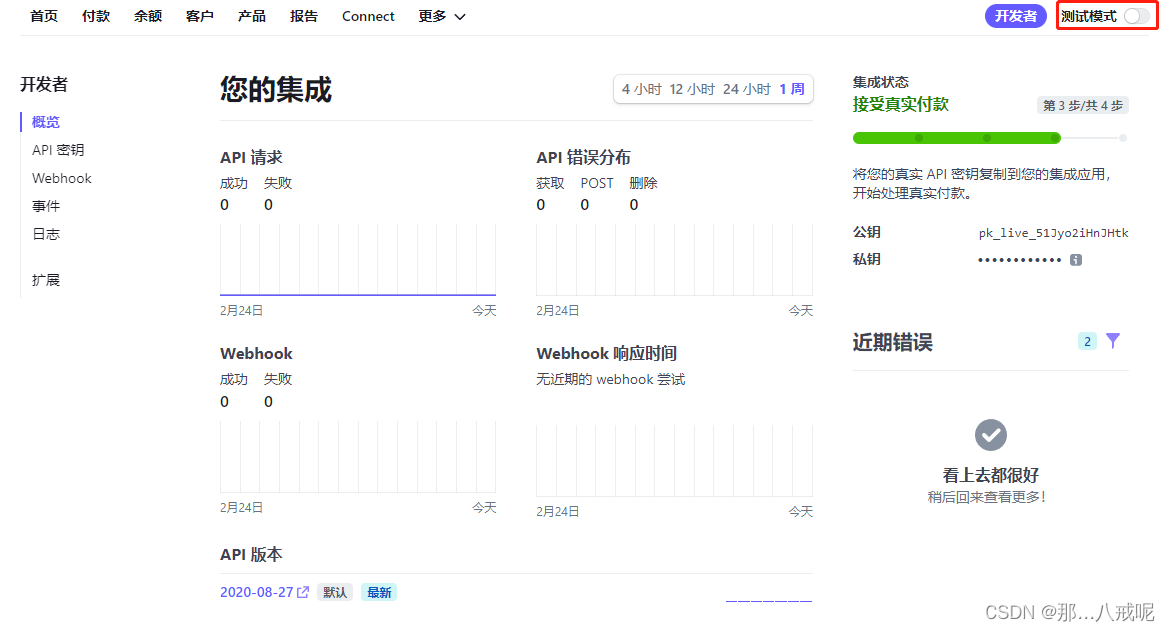
1. 安装Stripe:
composer?require?stripe/stripe-php
2. 获取密钥:https://dashboard.stripe.com/test/apikeys
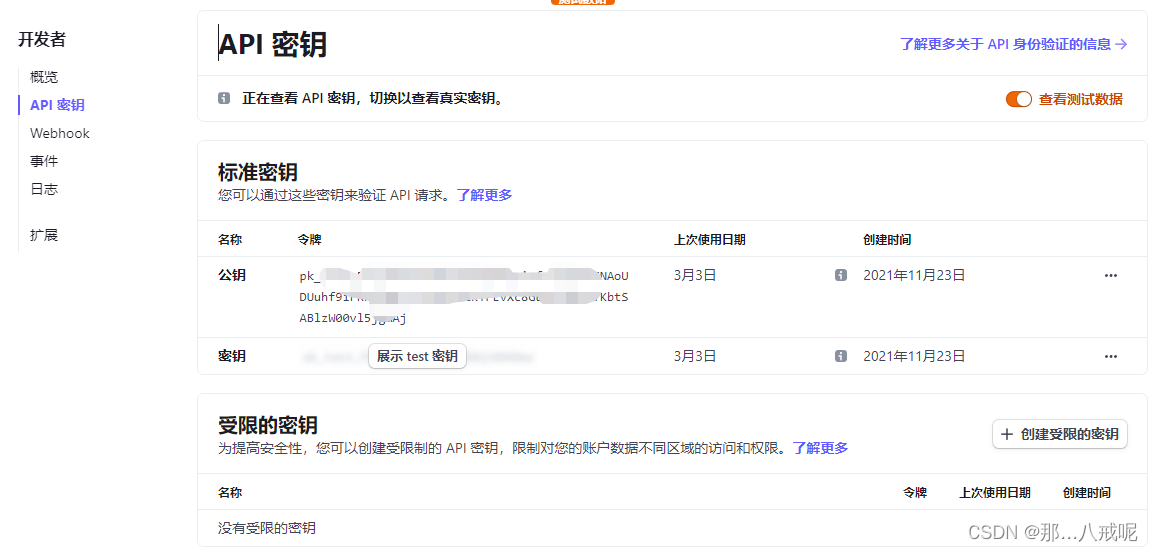
3. 创建产品:https://dashboard.stripe.com/test/products
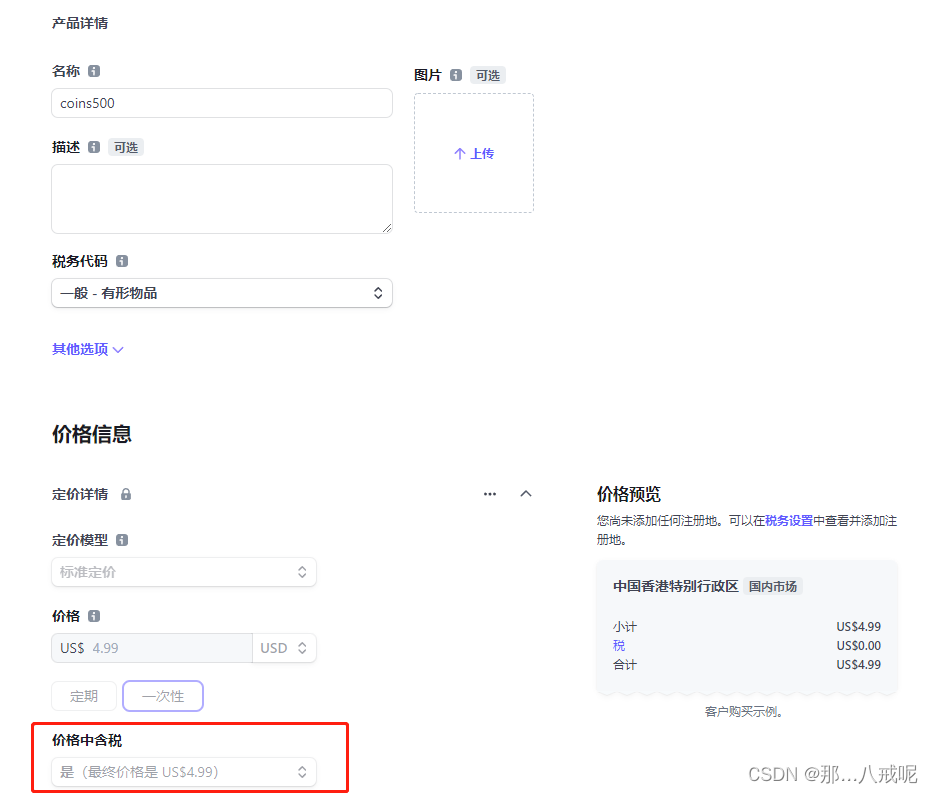 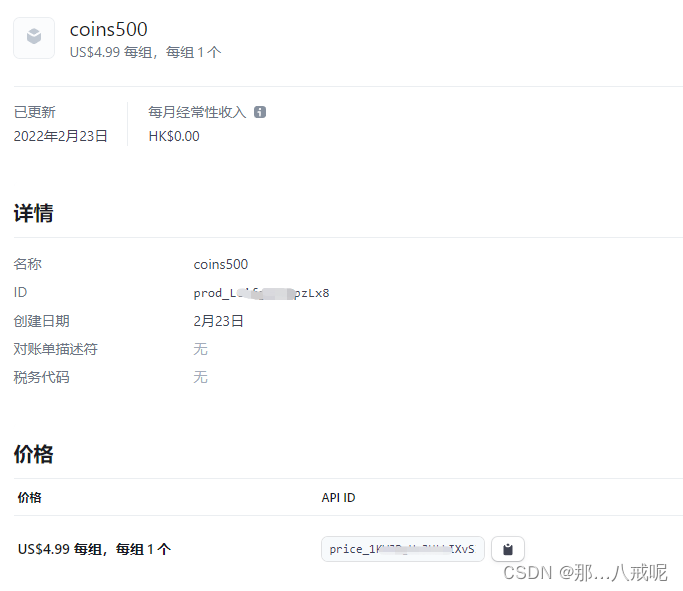
4. php 代码:
官方发起支付请求示例:https://stripe.com/docs/checkout/quickstart
/**
* stripe支付
*/
public function actionStripe()
{
$_key = 'sk_test_51Jyo2iHnJH***********************************';
// stripe 生成订单
$url = 'https://www.baidu.com';
\Stripe\Stripe::setApiKey($_key);
$checkout_session = \Stripe\Checkout\Session::create([
'line_items' => [[
'price' => {{PRICE_ID}}, // 产品id
'quantity' => 1,
]],
'mode' => 'payment',
'success_url' => $url . '/success.html',
'cancel_url' => $url . '/cancel.html',
'automatic_tax' => [
'enabled' => true,
],
]);
$arrGoodsInfo['payment_intent'] = $checkout_session->payment_intent;
// 创建本地订单操作,并payment_intent保留
// ......
//$checkout_session->url stripe跳转支付url
// $checkout_session->payment_intent是识别支付与通知关系的唯一凭证
return ['url' => $checkout_session->url, 'id' => $checkout_session->id, 'payment_intent' => $checkout_session->payment_intent];
}
出现tax_behavior 相关问题时需查看产品是否配置税务信息
var_dump($checkout_session );结果
object(Stripe\Checkout\Session)#149 (40) {
["id"]=>
string(66) "cs_test_a1sCxxxxJXHyXBgkPhsDTQV6Yw2AQtNL0ATkO2I6ekAUID"
["object"]=>
string(16) "checkout.session"
["after_expiration"]=>
NULL
["allow_promotion_codes"]=>
NULL
["amount_subtotal"]=>
int(99)
["amount_total"]=>
int(99)
["automatic_tax"]=>
object(Stripe\StripeObject)#160 (2) {
["enabled"]=>
bool(false)
["status"]=>
NULL
}
["billing_address_collection"]=>
NULL
["cancel_url"]=>
string(31) "https://baidu.com/cancel"
["client_reference_id"]=>
NULL
["consent"]=>
NULL
["consent_collection"]=>
NULL
["currency"]=>
string(3) "usd"
["customer"]=>
NULL
["customer_creation"]=>
string(6) "always"
["customer_details"]=>
NULL
["customer_email"]=>
NULL
["expires_at"]=>
int(1646384155)
["livemode"]=>
bool(false)
["locale"]=>
NULL
["metadata"]=>
object(Stripe\StripeObject)#161 (0) {
}
["mode"]=>
string(7) "payment"
["payment_intent"]=>
string(27) "pi_3xxxxxxxkIXvS1oQs8VqO"
["payment_link"]=>
NULL
["payment_method_options"]=>
array(0) {
}
["payment_method_types"]=>
array(1) {
[0]=>
string(4) "card"
}
["payment_status"]=>
string(6) "unpaid"
["phone_number_collection"]=>
object(Stripe\StripeObject)#165 (1) {
["enabled"]=>
bool(false)
}
["recovered_from"]=>
NULL
["setup_intent"]=>
NULL
["shipping"]=>
NULL
["shipping_address_collection"]=>
NULL
["shipping_options"]=>
array(0) {
}
["shipping_rate"]=>
NULL
["status"]=>
string(4) "open"
["submit_type"]=>
NULL
["subscription"]=>
NULL
["success_url"]=>
string(33) "https://baidu.com/success.html"
["total_details"]=>
object(Stripe\StripeObject)#169 (3) {
["amount_discount"]=>
int(0)
["amount_shipping"]=>
int(0)
["amount_tax"]=>
int(0)
}
["url"]=>
string(357) "https://checkout.stripe.com/pay/cs_test_xxxxbWppYWB3dic%2FcXdwYHgl"
}
链接跳转内容
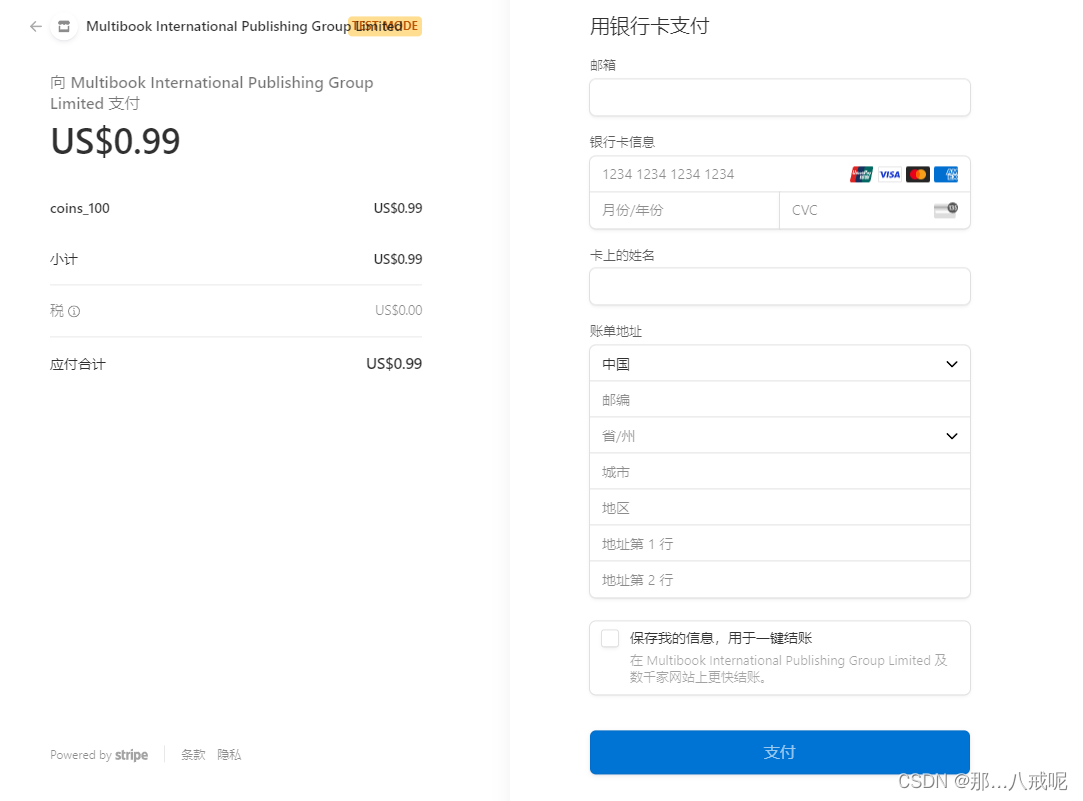
官方提供得测试账号:https://stripe.com/docs/testing
支付成功会自动跳转到success.html
回调:
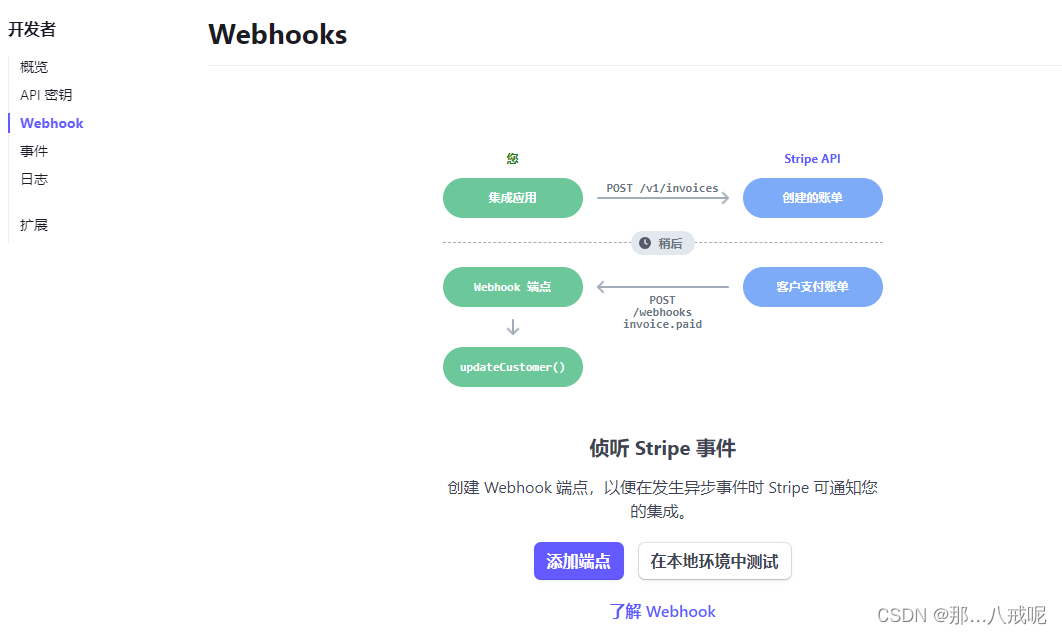
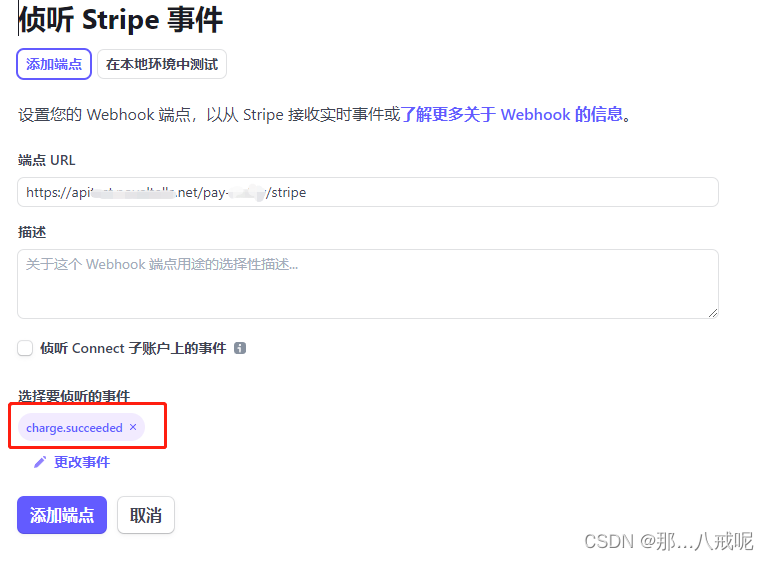
<?php
/**
* 支付回调
*/
namespace api\controllers;
use yii\web\Controller;
use Yii;
class PayNotifyController extends Controller
{
/**
* stripe支付回调
*/
public function actionStripe()
{
$_key = 'sk_test_51Jyo2iHnJH***********************************';
\Stripe\Stripe::setApiKey($_key);
$payload = @file_get_contents('php://input');
$event = null;
try {
$event = \Stripe\Event::constructFrom(
json_decode($payload, true)
);
} catch(\UnexpectedValueException $e) {
// Invalid payload
http_response_code(400);
exit();
}
// Handle the event
switch ($event->type) {
case 'charge.succeeded':
$succeeded = $event->data->object;
$content = "=========".date('Y-m-d H:i:s',time())."==========\r\n";
$content .= json_encode($succeeded);
file_put_contents(dirname(__DIR__).'/runtime/logs/stripe_success.log',$content . "\r\n",FILE_APPEND);
if ($succeeded->status == 'succeeded'){
$payment_intent = $succeeded->payment_intent;
}
break;
default:
echo 'Received unknown event type ' . $event->type;
break;
}
return true;
}
}
|