- C++规定在创建一个变量或常量时,必须要指定相应的数据类型,否则无法给变量分配内存。
- 数据类型存在的意义:给变量分配一个合适的内存空间
1. 整型
数据类型 | 占用空间 | 取值范围 |
---|
short (短整型) | 2字节 |
?
2
15
-2^{15}
?215 ~
2
15
2^{15}
215-1(-32768–32767) | int (整型) | 4字节 |
?
2
31
-2^{31}
?231 ~
2
31
2^{31}
231-1 | long (长整型) | Win4字节,Linux:32位4字节,64位8字节 |
?
2
31
-2^{31}
?231 ~
2
31
2^{31}
231-1 | longlong(长长整型) | 8字节 |
?
2
62
-2^{62}
?262 ~
2
62
2^{62}
262-1 |
#include<iostream>
using namespace std;
int main()
{
short num1 = 32768;
int num2 = 32768;
long num3 = 10;
long long num4 = 10;
cout << "num1 = " << num1 << endl;
cout << "num2 = " << num2 << endl;
cout << "num3 = " << num3 << endl;
cout << "num4 = " << num4 << endl;
system("pause");
return 0;
}
2. sizeof关键字
- 作用: 利用sizeof关键字统计数据类型所占内存大小
- 语法: sizeof(数据类型/变量)
#include<iostream>
using namespace std;
int main()
{
short num1 = 10;
cout << "num1 size:" << sizeof(num1) << endl;
cout << "short size:" << sizeof(short) << endl;
int num2 = 2;
cout << "int size:" << sizeof(int) << endl;
long num3 = 4;
cout << "long size:" << sizeof(long) << endl;
long long num4 = 70;
cout << "long long size:" << sizeof(long long) << endl;
system("pause");
return 0;
}
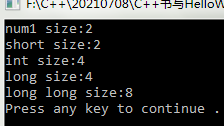
3. 实型(浮点型)
- 作用: 表示小数
- 类型:
? ? ? ? 单精度float:4字节,7位有效数字 ? ? ? ? 双精度double:8字节,15~16位有效数字
#include<iostream>
using namespace std;
int main()
{
float f1 = 3.1415926f;
cout << "f1:" << f1 << endl;
double d1 = 3.1415926;
cout << "d1:" << d1 << endl;
cout << "float size:" << sizeof(float) << endl;
cout << "double size:" << sizeof(double) << endl;
float f2 = 3e2;
cout << "f2:" << f2 << endl;
float f3 = 3e-2;
system("pause");
return 0;
}
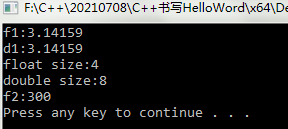
4. 字符型
- 作用: 字符型变量用于显示单个字符
- 语法: char ch = ‘a’; 单引号、单引号内只有单个字符
C++和C中字符型变量只占1个字节 字符型变量是将对应的ASCII编码(对应的编号)放入储存单元 eg:a — 97、b — 98
#include<iostream>
using namespace std;
int main()
{
char ch1 = 'a';
cout << "ch1:" << ch1 << endl;
cout << "char size:" << sizeof(char) << endl;
cout << int(ch1) << endl;
system("pause");
return 0;
}
5. 转义字符
- 作用: 用于表示一些不能显示出来的ASCII字符
- 常用: \ \n \t
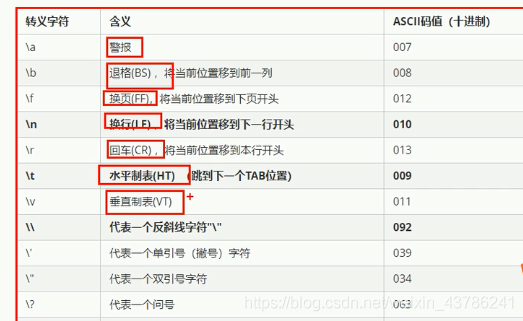
#include<iostream>
using namespace std;
int main()
{
cout << "hello word\n" << endl;
cout<<"\\"<<endl;
cout << "aaa\thello" << endl;
cout << "aaaaa\thello" << endl;
cout << "aaaaaa\thello" << endl;
system("pause");
return 0;
}
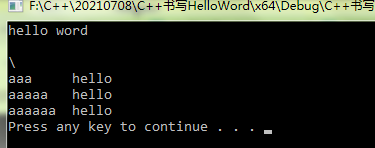
6. 字符串型
- 作用: 表示一串字符
- 风格:
????C风格字符串:char 名称[] = “字符串”; ????C++风格字符串:string 名称 = “字符串”;
#include<iostream>
using namespace std;
#include<string>
int main()
{
char str1[] = "hello word";
cout << "str1:"<<str1 << endl;
string str2 = "hi excel";
cout << "str2:" << str2 << endl;
system("pause");
return 0;
}
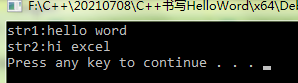
7. 布尔类型
- 作用: 代表真或假的值(占1个字节大小)
- true:1
- false:0
#include<iostream>
using namespace std;
int main()
{
bool flag = true;
cout << flag << endl;
flag = false;
cout << flag << endl;
cout << "bool size:" << sizeof(bool) << endl;
system("pause");
return 0;
}
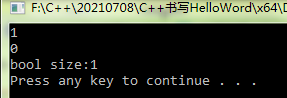
|