一元运算符
[]
来源: https://www.runoob.com/cplusplus/unary-operators-overloading.html
#include <iostream>
using namespace std;
class Distance
{
private:
int feet;
int inches;
public:
Distance(){
feet = 0;
inches = 0;
}
Distance(int f, int i){
feet = f;
inches = i;
}
void displayDistance()
{
cout << "F: " << feet << " I:" << inches <<endl;
}
Distance operator- ()
{
feet = -feet;
inches = -inches;
return Distance(feet, inches);
}
};
int main()
{
Distance D1(11, 10), D2(-5, 11);
-D1;
D1.displayDistance();
-D2;
D2.displayDistance();
return 0;
}
()
来源: https://www.jianshu.com/p/d3b132f6de2c
class FuncClass
{
public:
void operator()(const string& str) const
{
std::cout << str << std::endl;
}
};
int main()
{
FuncClass myFuncClass;
myFuncClass("hello world");
}
二元运算符
+, 输入输出流
来源: 谭浩强 c++程序设计第三版 p316
#include <iostream>
using namespace std;
class Complex {
public:
Complex();
Complex(double r, double i);
Complex operator+(Complex& c2);
friend istream& operator << (istream&, Complex&);
friend ostream& operator >> (ostream&, Complex&);
double real;
double imag;
};
Complex::Complex() {
real = 0;
imag = 0;
}
Complex::Complex(double r, double i) {
real = r;
imag = i;
}
istream& operator >> (istream& input, Complex& c) {
cout << "input real part and imaginary part of complex number:"<< endl;
input >> c.real >> c.imag;
return input;
}
ostream& operator << (ostream& output, Complex& c) {
output << "(" << c.real << "+" << c.imag << "i)";
return output;
}
int main() {
Complex c1, c2;
cin >> c1 >> c2;
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
}
转换构造函数
来源: 谭浩强 c++程序设计第三版 p323
#include <iostream>
using namespace std;
class Complex{
public:
Complex();
Complex(double r, double i);
operator double();
double real;
double imag;
};
Complex::Complex() {
real = 0;
imag = 0;
}
Complex::Complex(double r, double i) {
real = r;
imag = i;
}
Complex::operator double(){
return real;
}
int main() {
Complex c1(3, -4);
double d;
d = 2.5 + c1;
cout << d << endl;
}
更多
https://www.runoob.com/cplusplus/cpp-overloading.html 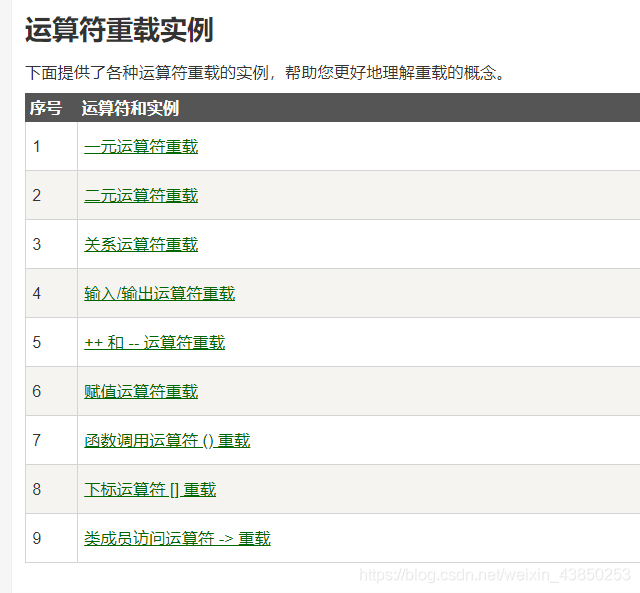
|