商品信息管理系统
背景介绍
在大一暑假小学期,老师布置了用C语言写800行以上代码的任务,我是选择了管理系统这方面,完成了我的商品信息管理系统的设计,可以供大家参考一下。
代码简要介绍
- 商品信息管理系统中包括管理者和购物者。
- 在刚开始进入的是主页面,可以选择管理者和购物者的身份,选择后要先输入账户和密码方能进入管理者系统或者购物者系统(账户和密码是放在了text3.txt和text4.txt中,要先在这两个文本中先输入账户和密码两个字符数据)。
- 在管理者界面,管理者可以进行数据的增删添改等操作,也可以根据商品的名称、编号和品牌进行查找商品信息,还能将数据存储到文件中,当然也可以从文件中提取数据。
- 在购物者界面中,购物者可以进行商品信息的查找,查看所有商品信息以及购买商品等操作。
代码展示(C语言)
#include <stdio.h>
#include <stdlib.h>
#include<string.h>
#define Length sizeof(struct commodity)
struct commodity
{
char name[10];
char brand[10];
long int number;
int price;
int t;
int l;
struct commodity *next;
};
struct commodity *head,*p,*p1,*p2;
struct commodity *head2,*q,*q1,*q2;
int n=0;
void print();
void find1();
void find2();
void find3();
void Delete();
void amend();
void save();
void read();
void buynumber();
void sort();
void save2();
void buy();
void read()
{
FILE *fp;
if((fp=fopen("text.txt","a+"))==NULL)
{
printf("Can not open file!");
exit(0);
}
p2=p1=(struct commodity *)malloc(Length);
head==NULL;
while(fscanf(fp,"%s%s%d%d%d\n",p1->name,p1->brand,&p1->number,&p1->price,&p1->t)!=EOF)
{
p1->l=0;
if(head==NULL)
head=p1;
else
p2->next=p1;
p2=p1;
p1=(struct commodity *)malloc(Length);
}
p2->next=NULL;
fclose(fp);
printf("读取完成!\n\n");
system("pause");
system("cls");
}
void add(){
system("cls");
p1=(struct commodity *)malloc(Length);
if(head==0)
head=p1;
else p2->next=p1;
printf("请输入商品基本信息\n");
printf("商品名称\n");
scanf("%s",&p1->name);
printf("商品品牌\n");
scanf("%s",&p1->brand);
printf("商品编号\n");
scanf("%d",&p1->number);
printf("商品价格\n");
scanf("%d",&p1->price);
printf("商品剩余数量\n");
scanf("%d",&p1->t);
p1->l=0;
p2=p1;
p2->next=NULL;
n+=1;
system("pause");
system("cls");
}
void print()
{
system("cls");
if(head!=NULL)
{
p=head;
printf("所有商品的信息\n");
do
{
printf("商品名称:%-8s 品牌:%-8s 编号:%-8d 价格:%-8d 剩余数量:%-8d 购买数量:%-8d\n",p->name,p->brand,p->number,p->price,p->t,p->l);
p=p->next;
}while(p!=NULL);
}
else{
printf("仓库中无数据,请管理者先添加数据!\n");
}
system("pause");
system("cls");
}
void find1 ()
{
system("cls");
int x=0;
char a[20];
printf("请输入要查找商品的名称:");
scanf("%s",&a);
p=head;
do
{
if(strcmp(a,p->name)==0)
{
x=1;
printf("\n信息已被找到:\n");
printf("商品名称:%-8s 品牌:%-8s 编号:%-8d 价格:%-8d 剩余数量:%-8d 购买数量:%-8d\n",p->name,p->brand,p->number,p->price,p->t,p->l);
}
p=p->next;
}while(p!=NULL);
if(x==0)printf("没有找到该商品\n");
system("pause");
system("cls");
}
void find2 ()
{
system("cls");
int x=0;
int a;
printf("请输入要查找商品编号:");
scanf("%d",&a);
p=head;
do
{
if(a==p->number)
{
x=1;
printf("\n信息已被找到:");
printf("商品名称:%-8s 品牌:%-8s 编号:%-8d 价格:%-8d 剩余数量:%-8d 购买数量:%-8d\n",p->name,p->brand,p->number,p->price,p->t,p->l);
}
p=p->next;
}while(p!=NULL);
if(x==0)
{
printf("没有找到该商品\n");
}
system("pause");
system("cls");
}
void find3()
{
system("cls");
int x=0;
char a[10];
printf("请输入要查找商品牌子:");
scanf("%s",a);
p=head;
do
{
if(strcmp(a,p->brand)==0)
{
x=1;
printf("\n信息已被找到:");
printf("商品名称:%-8s 品牌:%-8s 编号:%-8d 价格:%-8d 剩余数量:%-8d 购买数量:%-8d\n",p->name,p->brand,p->number,p->price,p->t,p->l);
}
p=p->next;
}while(p!=NULL);
if(x==0)printf("没有找到该商品\n");
system("pause");
system("cls");
}
void Delete()
{
if(head!=NULL)
{
struct commodity *f,*l;
char a[20];
printf("请输入要删除商品的名称:");
scanf("%s",&a);
p=head;
if(strcmp(head->name,a)==0)
{
f=head;
head=head->next;
free(f);
printf("数据已经被删除\n");
}
else
{
do
{
if(strcmp(p->name,a)==0)
{
f=p;
l->next=p->next;
free(f);
printf("数据已经被删除\n");
break;
}
l=p;
p=p->next;
}while(p!=NULL);
}
}
else {
printf("您还没有添加数据哟!\n");
}
system("pause");
system("cls");
}
void amend()
{
if(head!=NULL)
{
int c;
char a[20];
printf("请输入要修改商品的名称:\n");
scanf("%s",&a);
p=head;
do
{
if(strcmp(a,p->name)==0)
{
printf("被修改人信息如下:\n");
printf("%s %s %d %d %d\n",p->name,p->brand,p->number,p->price,p->t);
break;
}
p=p->next;
}while(p!=NULL);
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 1.修改名称 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 2.修改牌子 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 3.修改编号 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 4.修改价格 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 5.修改数量 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 6.不做修改 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("请输入对应数字进行修改操作:");
scanf("%d",&c);
if(c==1||c==2||c==3||c==4||c==5||c==6)
{
switch(c)
{
case 1:
printf("名称修改为:");
scanf("%s",&p->name);
break;
case 2:
printf("牌子修改为:");
scanf("%s",&p->brand);
break;
case 3:
printf("编号修改为:");
scanf("%s",&p->number);
break;
case 4:
printf("价格修改为:");
scanf("%d",&p->price);
break;
case 5:
printf("数量修改为:");
scanf("%d",&p->t);
break;
case 6:
break;
}
printf("\n\n操作成功!\n\n");
}
else
{
printf("输入错误,结束操作\n");
}
}
else
printf("******您还没有添加数据哟!\n");
system("pause");
system("cls");
}
void save()
{
remove("text.txt");
FILE *fp;
struct commodity *p;
if((fp=fopen("text.txt","a"))==NULL)
printf("Can not open the file!");
p=head;
while(p!=NULL)
{
fprintf(fp,"%s %s %d %d %d\n",p->name,p->brand,p->number,p->price,p->t);
p=p->next;
}
fclose(fp);
printf("存储完成\n");
system("pause");
system("cls");
}
void buy()
{
int x;
while(1)
{
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 1:输入编号购买商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 2:输入商品名称购买商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("\n");
printf("\n");
printf("购买者您好!\n");
printf("请选输入对应数字择您的购买方式:");
scanf("%d",&x);
if(x==1){
int t=0;
int a;
printf("请输入要购买商品的编号:");
scanf("%d",&a);
p=head;
do
{
if(a==p->number)
{
t=1;
printf("\n信息已被找到:");
printf("%s %s %d %d %d\n",p->name,p->brand,p->number,p->price,p->t);
printf("请输入要够买的数量\n");
int m;
while(1)
{
scanf("%d",&m);
if(m>p->t)
{
printf("很遗憾,本商品在仓库中没有这么多的数量,请重新输入要购买的数量\n");
}
else
{
printf("购买成功!\n");
printf("价格为%d元\n",p->price*m);
printf("本商品还剩余%d个\n",p->t-m);
p->t=p->t-m;
p->l=p->l+m;
break;
}
}
}
p=p->next;
}while(p!=NULL);
if(t==0)printf("没有找到该商品\n");
system("pause");
system("cls");
break;
}
if(x==2){
int t=0;
char a[10];
printf("请输入要购买商品的名称:");
scanf("%s",a);
p=head;
do
{
if(strcmp(a,p->name)==0)
{
t=1;
printf("\n信息已被找到:");
printf("%s %s %d %d %d\n",p->name,p->brand,p->number,p->price,p->t);
printf("请输入要够买的数量\n");
int m;
while(1)
{
scanf("%d",&m);
if(m>p->t)
{
printf("很遗憾,本商品在仓库中没有这么多的数量,请重新输入要购买的数量\n");
}
else
{
printf("购买成功!\n");
printf("价格为%d元\n",p->price*m);
printf("本商品还剩余%d个\n",p->t-m);
p->t=p->t-m;
p->l=p->l+m;
break;
}
}
}
p=p->next;
}while(p!=NULL);
if(t==0)printf("没有找到该商品\n");
system("pause");
system("cls");
break;
}
else
{
printf("输入错误,请重新输入!\n");
system("pause");
system("cls");
}
}
save();
}
void buynumber()
{
if(head!=NULL)
{
p=head;
printf("所有商品售卖数量的信息\n");
do
{
printf("%s %d\n",p->name,p->l);
p=p->next;
}while(p!=NULL);
}
else{
printf("仓库中无数据,请管理者先添加数据!\n");
}
system("pause");
}
void sort()
{
struct commodity *pfirst=NULL,*psecond=NULL,*pend=NULL;
pfirst=head;
psecond=head;
int temp;
char x[20];
while(pfirst != pend)
{
while(pfirst->next != pend)
{
if(psecond->l < pfirst->next->l)
{
temp=psecond->l;
psecond->l=pfirst->next->l;
pfirst->next->l=temp;
temp=psecond->t;
psecond->t=pfirst->next->t;
pfirst->next->t=temp;
temp=psecond->number;
psecond->number=pfirst->next->number;
pfirst->next->number=temp;
temp=psecond->price;
psecond->price=pfirst->next->price;
pfirst->next->price=temp;
strcpy(x,psecond->name);
strcpy(psecond->name,pfirst->next->name);
strcpy(pfirst->next->name,x);
strcpy(x,psecond->brand);
strcpy(psecond->brand,pfirst->next->brand);
strcpy(pfirst->next->brand,x);
}
pfirst=pfirst->next;
}
psecond=psecond->next;
pfirst=psecond;
}
print();
}
void save2()
{
sort();
remove("text2.txt");
FILE *fp;
struct commodity *p;
if((fp=fopen("text2.txt","a"))==NULL)
printf("Can not open the file!");
p=head;
while(p!=NULL)
{
fprintf(fp,"%-20s %-20s %-20d %-20d %-20d %-20d\n",p->name,p->brand,p->number,p->price,p->t,p->l);
p=p->next;
}
fclose(fp);
printf("存储完成\n");
system("pause");
system("cls");
}
int judge1(char count[],char password[])
{
char managercount[20],managerpassword[20];
FILE *fp;
if((fp=fopen("text3.txt","a+"))==NULL)
{
printf("Can not open file!");
exit(0);
}
while((fscanf(fp,"%s %s",managercount,managerpassword))!=EOF)
{
if(strcmp(managercount,count)==0&&strcmp(managerpassword,password)==0)
{
fclose(fp);
return 1;
}
}
fclose(fp);
return 0;
system("pause");
system("cls");
}
int judge2(char count[],char password[])
{
char usercount[20],userpassword[20];
FILE *fp;
if((fp=fopen("text4.txt","a+"))==NULL)
{
printf("Can not open file!");
exit(0);
}
while((fscanf(fp,"%s %s",usercount,userpassword))!=EOF)
{
if(strcmp(usercount,count)==0&&strcmp(userpassword,password)==0)
{
fclose(fp);
return 1;
}
}
fclose(fp);
return 0;
system("pause");
system("cls");
}
int main()
{
char a[20],b[20];
int x,y;
A:while(1)
{
system("cls");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 ☆ 欢迎来到:商品管理系统 ☆ 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 ☆ 制作者: 夏克冰 ☆ 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 ☆ 计算机三班 ☆ 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 管理者请输入:1 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 购物者请输入:2 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 退出系统请输入:3 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("\n");
printf("请选择您的身份或操作:");
scanf("%d",&x);
system("cls");
if(x==1)
{
printf("请输入管理者得账户和密码\n");
printf("账户:");
scanf("%s",a);
printf("密码:");
scanf("%s",b);
if(judge1(a,b)==1){
printf("输入正确,您已成功进入管理者系统\n");
while(1)
{
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 0:返回上一级菜单 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 1:添加商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 2:显示所有商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 3:按名称查找对应商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 4:按编号查找对应商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 5:查找同一牌子的所有商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 6:删除商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 7:修改商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 8:保存商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 9:从文件中读取数据 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 10:输出所有商品售卖的数量 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 11:按商品售卖数量排序输出 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 12:按商品售卖数量排序打印新文件〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 13:结束程序 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("您好,管理员!\n");
printf("请输入对应数字进行您的操作:");
scanf("%d",&y);
switch(y)
{
case 0:
goto A;
break;
case 1:
add();
break;
case 2:
print();
break;
case 3:
find1();
break;
case 4:
find2();
break;
case 5:
find3();
break;
case 6:
Delete();
break;
case 7:
amend();
break;
case 8:
save();
break;
case 9:
read();
break;
case 10:
buynumber();
break;
case 11:
sort();
break;
case 12:
save2();
break;
case 13:
return 0;
break;
}
}
}
else{
printf("您输入的账户或者密码不正确,返回初始菜单\n");
system("pause");
}
}
if(x==2)
{
printf("请输入管理者得账户和密码\n");
printf("账户:");
scanf("%s",a);
printf("密码:");
scanf("%s",b);
if(judge2(a,b)==1) {
printf("输入正确,您已成功进入购买者系统\n");
while(1)
{
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 0:返回上一级菜单 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 1:输入商品编号查询商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 2:输入商品名称查询商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 3:输入商品品牌查看本品牌所有商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 4:查看所有商品信息 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 5:购买商品 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 6:结束程序 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓 〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓〓\n");
printf("您好,客户!\n");
printf("请输入对应数字进行您的操作:");
scanf("%d",&y);
switch(y)
{
case 0:
goto A;
break;
case 1:
find2();
break;
case 2:
find1();
break;
case 3:
find3();
break;
case 4:
print();
break;
case 5:
buy();
break;
case 6:
return 0;
break;
}
}
}
else{
printf("您输入的账户或者密码不正确,返回初始菜单\n");
system("pause");
}
}
if(x==3){
return 0;
}
}
}
(下边是运行结果展示图片)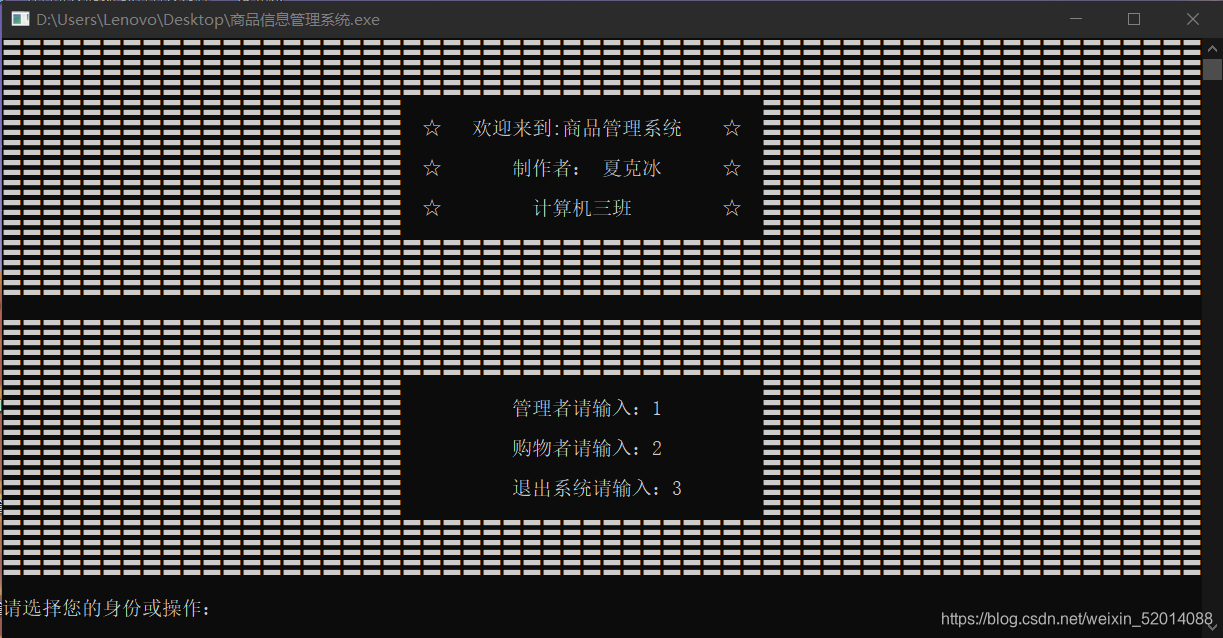 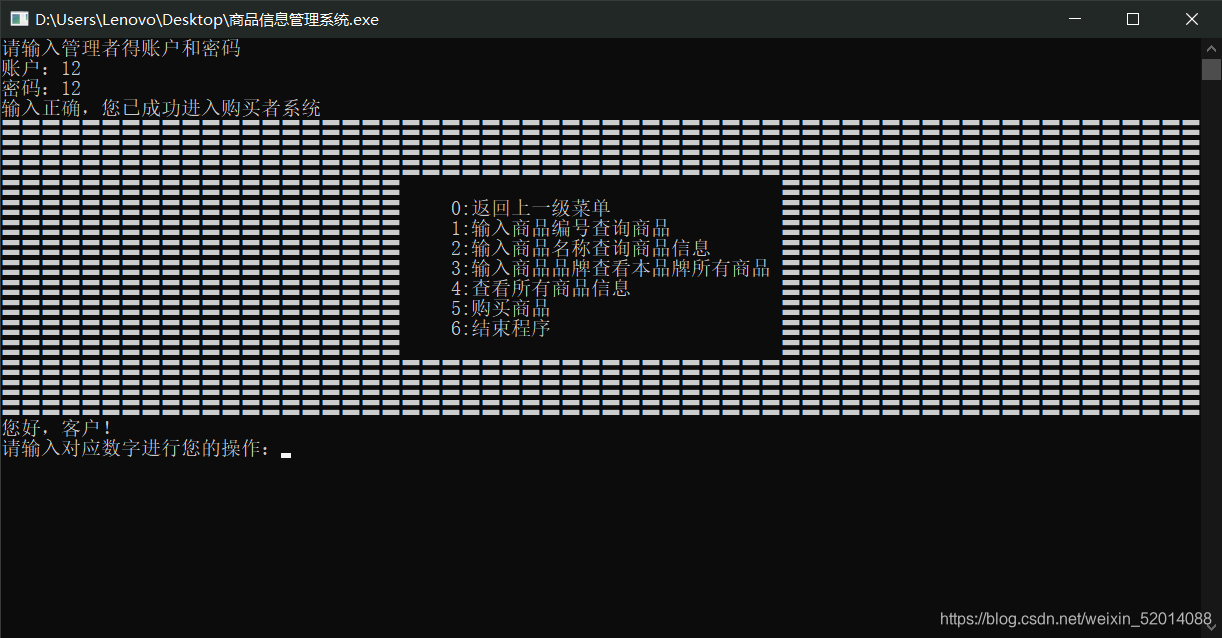 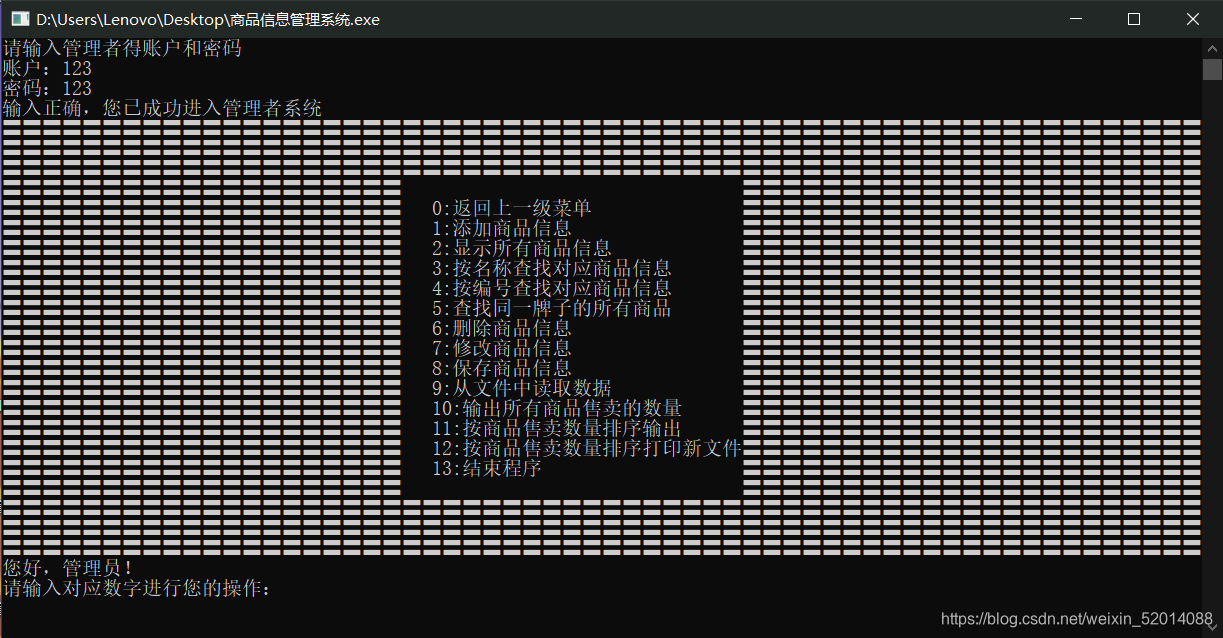
|