看如下代码:
class parent
{
public:
int m_i;
int m_j;
};
class child :public parent
{
public:
int m_k;
int m_l;
};
void main()
{
printf("parent::m_i = %d\n", &parent::m_i);
printf("parent::m_j = %d\n", &parent::m_j);
printf("parent::m_i = %d\n", &child::m_i);
printf("parent::m_j = %d\n", &child::m_j);
printf("parent::m_i = %d\n", &child::m_k);
printf("parent::m_j = %d\n", &child::m_l);
system("pause");
}
结果: 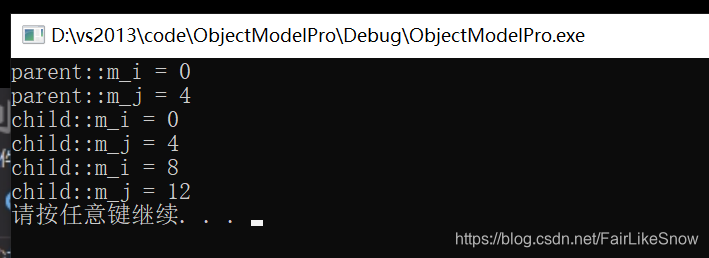 (1)一个子类对象,所包含的内容,是他自己的成员,加上他父类的成员的总和。 (2)从偏移值看,父类成员先出现,然后才是子类的成员。 下图是一个变量布局,左边是父类,右边是子类。 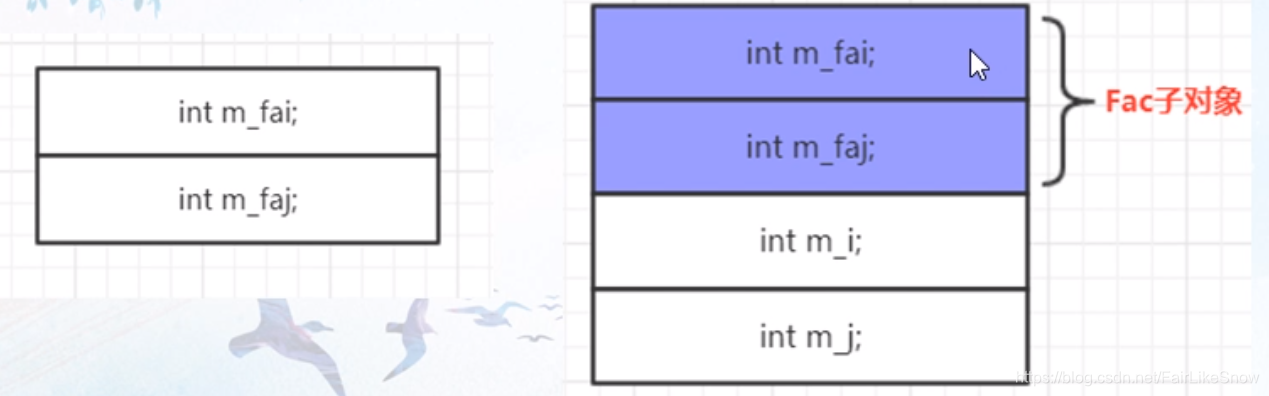 继续看代码:
class Base
{
public:
int m_i1;
char m_c1;
char m_c2;
char m_c3;
};
void main()
{
cout << "sizeof(Base) = " << sizeof(Base) << endl;
printf("Base::m_i1 = %d\n", &Base::m_i1);
printf("Base::m_c1 = %d\n", &Base::m_c1);
printf("Base::m_c2 = %d\n", &Base::m_c2);
printf("Base::m_c3 = %d\n", &Base::m_c3);
system("pause");
}
结果: 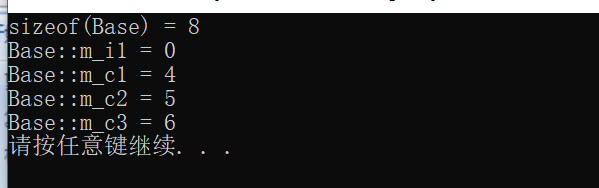 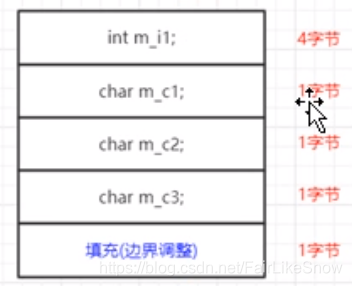 接下来将这个类做一个拆分,如下:
class Base1
{
public:
int m_i1;
char m_c1;
};
class Base2:public Base1
{
public:
char m_c2;
};
class Base3 :public Base2
{
public:
char m_c3;
};
void main()
{
cout << sizeof(Base1) << endl;
cout << sizeof(Base2) << endl;
cout << sizeof(Base3) << endl;
system("pause");
}
运行结果: 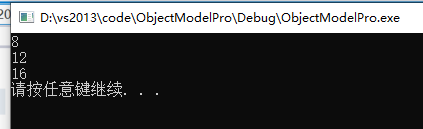 变量的个数依然是四个,但是大小却差别很大,之前在一起的时候是8个字节,现在拆开后成了16个字节。 接下来打印一下偏移值,如下:
void main()
{
cout << sizeof(Base1) << endl;
cout << sizeof(Base2) << endl;
cout << sizeof(Base3) << endl;
printf("Base3::m_i1 = %d\n", &Base3::m_i1);
printf("Base3::m_c1 = %d\n", &Base3::m_c1);
printf("Base3::m_c2 = %d\n", &Base3::m_c2);
printf("Base3::m_c3 = %d\n", &Base3::m_c3);
system("pause");
}
结果: 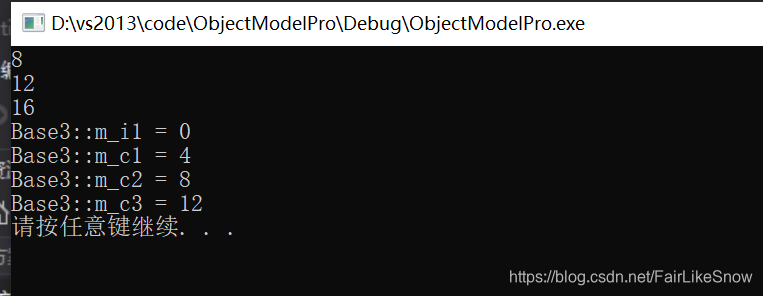 下面是base1的布局: 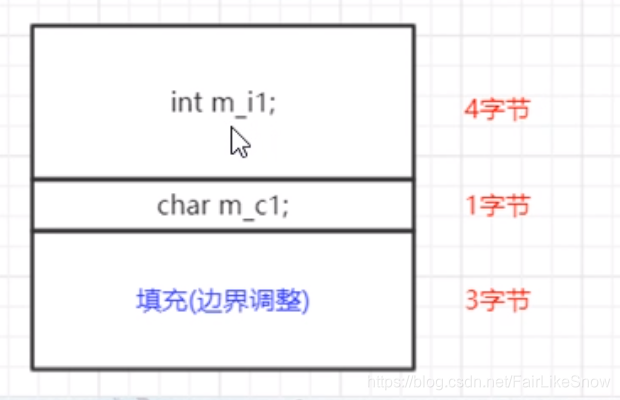 所以占了8个字节。 下面是Base2的布局: 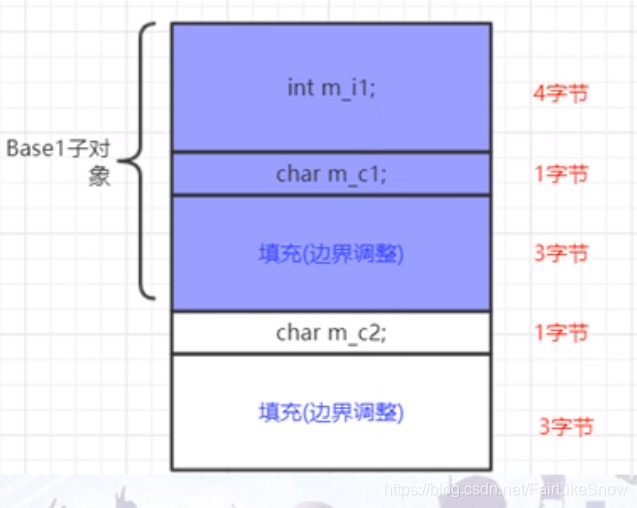 这个要包含Base1的调整(8字节),然后加上自己的1字节,9个字节,然后调整为12字节。 同理Base3也就类推,为16个字节,布局如下: 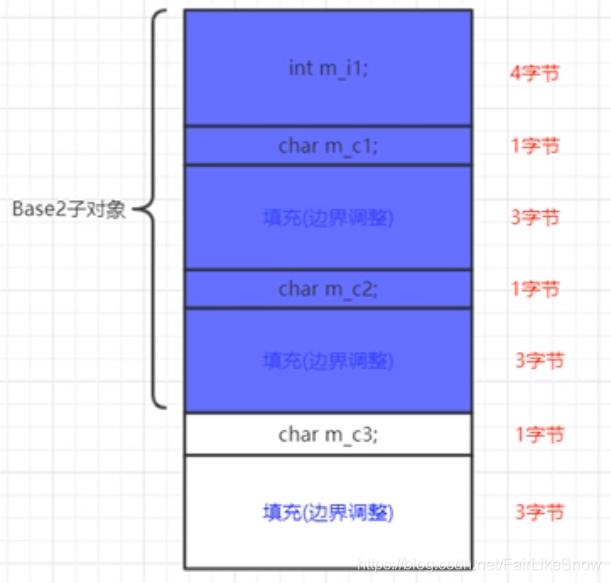
|