功能图解
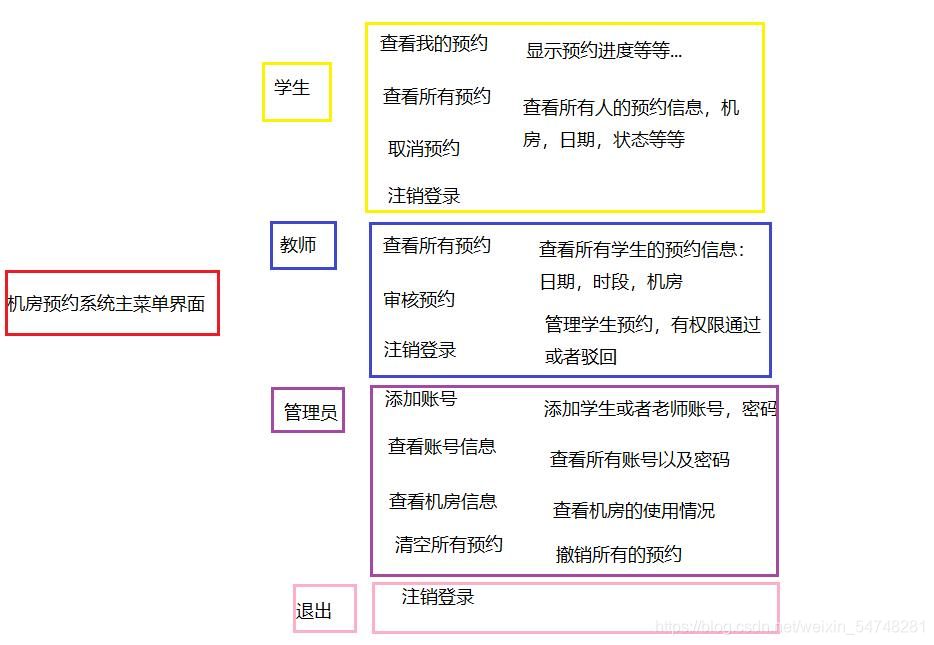
代码区
机房预约系统.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include <iostream>
#include "Identity.h"
#include <fstream>
#include <string>
#include "student.h"
#include "teacher.h"
#include "caretaker.h"
#include "globalFile.h"
using namespace std;
void caretakerMenu(Identity * &caretaker)
{
while (true)
{
caretaker->operMenu();
Caretaker* care = (Caretaker*)caretaker;
int select = 0;
cin >> select;
if (select == 1)
{
cout << "添加账号" << endl;
care->addPerson();
}
else if (select == 2)
{
cout << "查看账号" << endl;
care->showPerson();
}
else if (select == 3)
{
cout << "查看机房" << endl;
care->showComputer();
}
else if (select == 4)
{
cout << "清空预约" << endl;
care->clearFile();
}
else
{
delete caretaker;
cout << "注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
void studentMenu(Identity * &student)
{
while (true)
{
student->operMenu();
Student * stu = (Student*)student;
int select = 0;
cin >> select;
if (select == 1)
{
stu->applyOrder();
}
else if (select == 2)
{
stu->showMyOrder();
}
else if (select == 3)
{
stu->showAllOrder();
}
else if (select == 4)
{
stu->cancelOrder();
}
else if (select == 0)
{
delete student;
system("pause");
system("cls");
return;
}
}
}
void teacherMenu(Identity * &teacher)
{
while (true)
{
teacher->operMenu();
Teacher * tea = (Teacher*)teacher;
int select = 0;
cin >> select;
if (select == 1)
{
tea->showAllOrder();
}
else if (select == 2)
{
tea->valiOrder();
}
else
{
delete teacher;
cout <<"注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
void Login(string FileName, int type)
{
Identity *person = NULL;
ifstream ifs;
ifs.open(FileName, ios::in);
if (!ifs.is_open())
{
cout << "文件不存在!" << endl;
ifs.close();
return;
}
int id=0;
string name;
string pwd;
if (type == 1)
{
cout << "请输入你的学号:" << endl;
cin >> id;
}
else if (type==2)
{
cout << "请输入你的职工号:" << endl;
cin >> id;
}
cout << "请输入用户名:" << endl;
cin >> name;
cout << "请输入密码:" << endl;
cin >> pwd;
if (type == 1)
{
int fId;
string fName;
string fPwd;
while (ifs >> fId && ifs >> fName && ifs >> fPwd)
{
if (id == fId&&name == fName&&pwd == fPwd)
{
cout << "学生验证登录成功!" << endl;
system("pause");
system("cls");
person = new Student(id, name, pwd);
studentMenu(person);
return;
}
}
}
else if (type == 2)
{
int fId;
string fName;
string fPwd;
while (ifs >> fId&&ifs >> fName&&ifs >> fPwd)
{
if (id == fId&&name == fName&&pwd == fPwd)
{
cout << "教师成功登录!" << endl;
system("pause");
system("cls");
person = new Teacher(id,name, pwd);
teacherMenu(person);
return;
}
}
}
else if (type==3)
{
string fName;
string fPwd;
while (ifs >> fName&&ifs>>fPwd)
{
if (name == fName&&pwd == fPwd)
{
cout << "管理员成功登录" << endl;
system("pause");
system("cls");
person = new Caretaker(name, pwd);
caretakerMenu(person);
return;
}
}
}
cout << "验证登录失败!" << endl;
system("pause");
system("cls");
}
int main()
{
while (true)
{
cout << "-----------------欢迎使用机房预约系统------------------" << endl;
cout << endl << "请输入你的身份:" << endl;
cout << "\t\t——————————————————" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 1.学生或职工 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 2.机房教师 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 3.管理员 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 4.退出 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t——————————————————" << endl;
cout << "请输入你的选择:";
int select = 0;
cin >> select;
switch (select)
{
case 1://学生
Login(STUDENT_FILE, 1);
break;
case 2://教师
Login(TEACHER_FILE, 2);
break;
case 3://管理员
Login(CARETAKER_FILE, 3);
break;
case 4://退出系统
cout << "欢迎下次使用!" << endl;
system("pause");
return 0;
break;
default:
cout << "输入有误请重新选择!" << endl;
system("pause");
system("cls");
break;
}
}
system("pause");
return 0;
}
caretaker.h
#pragma once
#include "Identity.h"
#include <iostream>
#include <fstream>
#include <vector>
#include "student.h"
#include "teacher.h"
#include "globalFile.h"
#include "computerRoom.h"
#include <algorithm>
using namespace std;
class Caretaker :public Identity
{
public:
Caretaker();
Caretaker(string name, string pwd);
virtual void operMenu();
void addPerson();
void showPerson();
void showComputer();
void clearFile();
void initVector();
bool checkRepeat(int id,int type);
vector<Student>vStu;
vector<Teacher>vTea;
vector<ComputerRoom>vCom;
};
computerRoom.h
#pragma once
#include<iostream>
using namespace std;
class ComputerRoom
{
public:
int m_ComId;
int m_MaxNum;
};
golbalFile.h
#pragma once
#define STUDENT_FILE "student.txt"
#define TEACHER_FILE "teacher.txt"
#define CARETAKER_FILE "caretaker.txt"
#define COMPUTER_FILE "computerroom.txt"
#define ORDER_FILE "order.txt"
Identity.h
#pragma once
#include <iostream>
#include <string>
using namespace std;
class Identity
{
public:
virtual void operMenu() = 0;
string m_Name;
string m_Pwd;
};
orderFile.h
#pragma
#include <iostream>
#include <fstream>
#include <algorithm>
#include "Identity.h"
using namespace std;
#include <map>
#include "globalFile.h"
class OrderFile
{
public:
OrderFile();
void updateOrder();
map<int, map<string, string>> m_orderData;
int m_Size;
};
student.h
#pragma once
#include <iostream>
#include <fstream>
#include <vector>
#include "globalFile.h"
#include "computerRoom.h"
#include "Identity.h"
using namespace std;
class Student :public Identity
{
public:
Student();
Student(int id, string name, string pwd);
virtual void operMenu();
void applyOrder();
void showMyOrder();
void showAllOrder();
void cancelOrder();
int m_ID;
vector<ComputerRoom> vCom;
};
teacher.h
#pragma once
#include <iostream>
#include<string>
#include<vector>
#include "Identity.h"
using namespace std;
class Teacher :public Identity
{
public:
Teacher();
Teacher(int empid, string name, string pwd);
virtual void operMenu();
void showAllOrder();
void valiOrder();
int m_EmpId;
};
caretaker.cpp
#define _CRT_SECURE_NO_WARNINGS 1
# include "caretaker.h"
Caretaker::Caretaker()
{
}
Caretaker::Caretaker(string name, string pwd)
{
this->m_Name = name;
this->m_Pwd = pwd;
this->initVector();
ifstream ifs;
ifs.open(COMPUTER_FILE, ios::in);
ComputerRoom c;
while (ifs >> c.m_ComId&&ifs >> c.m_MaxNum)
{
vCom.push_back(c);
}
cout << "当前机房数量为: " << vCom.size() << endl;
ifs.close();
}
void Caretaker::operMenu()
{
cout << "欢迎管理员:" << this->m_Name << "登录" << endl;
cout << "\t\t————————————————————"<<endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 1.添加账号 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 2.查看账号 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 3.查看机房 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 4.清空预约 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 0.注销登录 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t————————————————————" << endl;
cout << "请选择您的操作:" << endl;
}
void Caretaker::addPerson()
{
cout << "请输入要添加账号的类型" << endl;
cout << "1.添加学生" << endl;
cout << "2.添加教师" << endl;
string fileName;
string tip;
string errorTip;
ofstream ofs;
while (true)
{
int select = 0;
cin >> select;
if (select == 1)
{
fileName = STUDENT_FILE;
tip = "请输入学号: ";
errorTip = "学号重复,请重新输入!";
}
else if (select == 2)
{
fileName = TEACHER_FILE;
tip = "请输入教职工编号:";
errorTip = "职工号重复,请重新输入!";
}
else
{
system("pause");
system("cls");
break;
}
ofs.open(fileName, ios::out | ios::app);
int id;
string name;
string pwd;
cout << tip << endl;
while (true)
{
cin >> id;
bool ret = checkRepeat(id,select);
if (ret)
{
cout << errorTip << endl;
}
else
{
break;
}
}
cout << "请输入姓名: " << endl;
cin >> name;
cout << "请输入密码:" << endl;
cin >> pwd;
ofs << id << " " << name << " " << pwd << " " << endl;
cout << "添加成功!" << endl;
system("pause");
system("cls");
ofs.close();
this->initVector();
}
}
void printStudent(Student & s)
{
cout << "学号: " << s.m_ID << " 姓名: " << s.m_Name << " 密码: " << s.m_Pwd << endl;
}
void printTeacher(Teacher & t)
{
cout << "职工号: " << t.m_EmpId << " 姓名: " << t.m_Name << " 密码: " << t.m_Pwd << endl;
}
void Caretaker::showPerson()
{
cout << "请选择要查看的账号类型:" << endl;
cout << "1.查看所有学生" << endl;
cout << "2.查看所有老师" << endl;
int select = 0;
cin >> select;
if (select == 1)
{
cout << "所有学生信息如下:" << endl;
for_each (vStu.begin(), vStu.end(), printStudent);
}
else
{
cout << "所有老师信息如下:" << endl;
for_each(vTea.begin(), vTea.end(), printTeacher);
}
system("pause");
system("cls");
}
void Caretaker::showComputer()
{
cout << "机房信息如下:" << endl;
for (vector<ComputerRoom>::iterator it = vCom.begin(); it != vCom.end(); it++)
{
cout << "机房编号: " << it->m_ComId << " 最大容量:"<<it->m_MaxNum<<endl;
}
system("pause");
system("cls");
}
void Caretaker::clearFile()
{
ofstream ofs(COMPUTER_FILE, ios::trunc);
ofs.close();
cout << " 清空成功!" << endl;
system("pause");
system("cls");
}
void Caretaker::initVector()
{
vStu.clear();
vTea.clear();
ifstream ifs;
ifs.open(STUDENT_FILE, ios::in);
if (!ifs.is_open())
{
cout << "文件读取失败!" << endl;
return;
}
Student s;
while (ifs >> s.m_ID&&ifs >> s.m_Name&&ifs >> s.m_Pwd)
{
vStu.push_back(s);
}
cout << "当前学生账号数量为: " << vStu.size() << endl;
ifs.close();
ifs.open(TEACHER_FILE, ios::in);
Teacher t;
while (ifs >> t.m_EmpId&&ifs >> t.m_Name&&ifs >> t.m_Pwd)
{
vTea.push_back(t);
}
cout << "当前教职工账号的数量为: " << vTea.size() << endl;
ifs.close();
}
bool Caretaker::checkRepeat(int id, int type)
{
if (type == 1)
{
for (vector<Student>::iterator it = vStu.begin(); it != vStu.end(); it++)
{
if (id == it->m_ID)
{
return true;
}
}
}
else
{
for (vector<Teacher>::iterator it = vTea.begin(); it != vTea.end(); it++)
{
if (id == it->m_EmpId)
{
return true;
}
}
return false;
}
}
orderFile.cpp
```cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include "orderFile.h"
OrderFile::OrderFile()
{
ifstream ifs;
ifs.open(ORDER_FILE, ios::in);
string data;
string time;
string stuId;
string stuName;
string roomId;
string status;
this->m_Size = 0;
while (ifs >> data&&ifs >> time&&ifs >> stuId&&ifs >> stuName&&ifs >> roomId&&ifs >> status)
{
string key;
string value;
map<string, string>m;
int pos = data.find(":");
if (pos != -1)
{
key = data.substr(0, pos);
value = data.substr(pos + 1, data.size() - pos);
m.insert(make_pair(key, value));
}
pos = time.find(":");
if (pos != -1)
{
key = time.substr(0, pos);
value = time.substr(pos + 1, time.size() - pos);
m.insert(make_pair(key, value));
}
pos = stuId.find(":");
if (pos != -1)
{
key = stuId.substr(0, pos);
value = stuId.substr(pos + 1, stuId.size() - pos);
m.insert(make_pair(key, value));
}
pos = stuName.find(":");
if (pos != -1)
{
key = stuName.substr(0, pos);
value = stuName.substr(pos + 1, stuName.size() - pos);
m.insert(make_pair(key, value));
}
pos = roomId.find(":");
if (pos != -1)
{
key = roomId.substr(0, pos);
value = roomId.substr(pos + 1, roomId.size() - pos);
m.insert(make_pair(key, value));
}
pos = status.find(":");
if (pos != -1)
{
key = status.substr(0, pos);
value = status.substr(pos + 1, status.size() - pos);
m.insert(make_pair(key, value));
}
this->m_orderData.insert(make_pair(this->m_Size, m));
this->m_Size++;
}
}
void OrderFile::updateOrder()
{
if (this->m_Size == 0)
{
return;
}
ofstream ofs(ORDER_FILE, ios::out | ios::trunc);
for (int i = 0; i < m_Size; i++)
{
ofs << "date:" << this->m_orderData[i]["data"] << " ";
ofs << "time:" << this->m_orderData[i]["time"] << " ";
ofs << "stuId:" << this->m_orderData[i]["stuId"] << " ";
ofs << "stuName" << this->m_orderData[i]["stuName"] << " ";
ofs << "roomId:" << this->m_orderData[i]["roomId"] << " ";
ofs << "status:" << this->m_orderData[i]["status"] << " ";
}
ofs.close();
}
student.cpp
```cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include "student.h"
# include "orderFile.h"
//默认构造
Student::Student()
{
}
//有参构造
Student::Student(int id, string name, string pwd)
{
//初始化属性
this->m_ID = id;
this->m_Name = name;
this->m_Pwd = pwd;
//初始化机房信息
ifstream ifs;
ifs.open(COMPUTER_FILE, ios::in);
ComputerRoom com;
while (ifs >> com.m_ComId&&ifs >> com.m_MaxNum)
{
vCom.push_back(com);
}
ifs.close();
}
//菜单界面
void Student::operMenu()
{
cout << "欢迎学生: " << this->m_Name << "--登录" << endl;
cout << "\t\t————————————————————" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 1.申请预约 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 2.查看我的预约 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 3.查看所有预约 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 4.取消预约 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t| 0.注销登录 |" << endl;
cout << "\t\t| |" << endl;
cout << "\t\t————————————————————" << endl;
cout << "请选择您的操作:" << endl;
}
//申请预约
void Student::applyOrder()
{
cout << "机房开房时间为周一至周五" << endl;
cout << "请选择想要预约的时间段:" << endl;
cout << "1.周一" << endl;
cout << "2.周二" << endl;
cout << "3.周三" << endl;
cout << "4.周四" << endl;
cout << "5.周五" << endl;
int date = 0;//日期
int time = 0;//时间段
int roomId = 0;//机房编号
while (true)
{
cin >> date;
if (date >= 1 && date <= 5)
{
break;
}
cout << "请输入正确的时间段!" << endl;
}
cout << "请选择需要预约的时间段:" << endl;
cout << "1.上午" << endl;
cout << "2.下午" << endl;
while (true)
{
cin >> time;
if (time >= 1 && time <= 2)
{
break;
}
cout << "请选择正确的时间段!" << endl;
}
cout << "请选择想要预约的机房:" << endl;
for (int i = 0; i < vCom.size(); i++)
{
cout << vCom[i].m_ComId << "号机房--" << "容量为: " << vCom[i].m_MaxNum << endl;
}
while (true)
{
cin >> roomId;
if (roomId >= 1 && roomId <= 3)
{
break;
}
cout << "请输入正确的机房编号!" << endl;
}
cout << "预约成功,审核中!" << endl;
ofstream ofs;
ofs.open(ORDER_FILE, ios::app);
ofs << "date:" << date << " ";
ofs << "time:" << time << " ";
ofs << "stuId:" << this->m_ID << " ";
ofs << "stuName:" << this->m_Name << " ";
ofs << "roomId:" << roomId << " ";
ofs << " status:" << 1 << " ";
ofs.close();
system("pause");
system("cls");
}
//显示我的预约
void Student::showMyOrder()
{
OrderFile of;
if (of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
for (int i = 0; i < of.m_Size; i++)
{
if (atoi(of.m_orderData[i]["stuId"].c_str()) == this->m_ID)
{
cout << "预约日期: 周" << of.m_orderData[i]["date"];
cout << " 时段: " << (of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << " 机房编号: " << of.m_orderData[i]["roomId"];
string status = "状态: ";//0取消预约 1审核中 2已预约 -1预约失败
if (of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (of.m_orderData[i]["status"] == "-1")
{
status += "预约失败,审核未通过";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
}
}
//显示所有预约
void Student::showAllOrder()
{
OrderFile of;
if (of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
for (int i = 0; i < of.m_Size; i++)
{
cout << i + 1 << ", ";
cout << "预约日期: 周" << of.m_orderData[i]["date"];
cout << " 时段: " << (of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << " 学号: " << of.m_orderData[i]["stuId"];
cout << " 姓名: " << of.m_orderData[i]["stuName"];
cout << " 机房编号: " << of.m_orderData[i]["roomId"];
string status = "状态: ";//0取消预约 1审核中 2已预约 -1预约失败
if (of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (of.m_orderData[i]["status"] == "-1")
{
status += "预约失败,审核未通过";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
system("pause");
system("cls");
}
//取消预约
void Student::cancelOrder()
{
OrderFile of;
if (of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
cout << "状态为审核中或预约成功的记录可以取消,请输入想取消的预约号" << endl;
vector<int>v;
int index = 1;
for (int i = 0; i < of.m_Size; i++)
{
if (atoi(of.m_orderData[i]["stuId"].c_str()) == this->m_ID)
{
if (of.m_orderData[i]["status"] == "1" || of.m_orderData[i]["status"] == "2")
{
v.push_back(i);
cout << index++ << ", ";
cout << "预约日期: 周" << of.m_orderData[i]["date"];
cout << " 时段: " << (of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << " 机房编号: " << of.m_orderData[i]["roomId"];
string status = "状态: ";//0取消预约 1审核中 2已预约 -1预约失败
if (of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (of.m_orderData[i]["status"] == "-1")
{
status += "预约失败,审核未通过";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
}
}
cout << "请输入想取消的记录编号,0代表返回" << endl;
int select = 0;
while (true)
{
cin >> select;
if (select >= 0 && select <= v.size())
{
if (select == 0)
{
break;
}
else
{
of.m_orderData[v[select - 1]]["status"] = "0";
of.updateOrder();
cout << "已取消预约" << endl;
break;
}
}
cout << "编号不存在!" << endl;
}
}
teacher.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include "teacher.h"
# include "orderFile.h"
Teacher::Teacher()
{
}
Teacher::Teacher(int empid, string name, string pwd)
{
this->m_EmpId = empid;
this->m_Name = name;
this->m_Pwd = pwd;
}
void TeacherMenu(Identity * &teacher)
{
while (true)
{
teacher->operMenu();
Teacher* tea = (Teacher*)teacher;
int select = 0;
cin >> select;
if (select == 1)
{
tea->showAllOrder();
}
else if (select == 2)
{
tea->valiOrder();
}
else
{
delete teacher;
cout << "注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
void Teacher::operMenu()
{
cout << "欢迎教师" << this->m_Name << "登录!" << endl;
cout << "\t\t----------------------------------\n";
cout << "\t\t| |\n";
cout << "\t\t| 1.查看所有预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 2.审核预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 0.注销登录 |\n";
cout << "\t\t| |\n";
cout << "\t\t| |\n";
cout << "\t\t----------------------------------\n";
}
void Teacher::showAllOrder()
{
OrderFile of;
if (of.m_Size == 0)
{
cout << "当前无预约记录" << endl;
system("pause");
system("cls");
return;
}
for (int i = 0; i < of.m_Size; i++)
{
cout << i + 1 << ", ";
cout << "预约日期: 周" << of.m_orderData[i]["data"];
cout << "时段: " << (of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << "学号: " << of.m_orderData[i]["stuId"];
cout << "姓名: " << of.m_orderData[i]["stuName"];
cout << "机房: " << of.m_orderData[i]["roomId"];
string status = "状态";
if (of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (of.m_orderData[i]["status"] == "-1")
{
status += "审核未通过,预约失败";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
system("pause");
system("cls");
}
void Teacher::valiOrder()
{
OrderFile of;
if (of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
cout << "待审核预约如下:" << endl;
vector<int>v;
int index = 0;
for (int i = 0; i < of.m_Size; i++)
{
if (of.m_orderData[i]["status"] == "1")
{
v.push_back(i);
cout << ++index << ", ";
cout << "预约日期: 周" << of.m_orderData[i]["date"];
cout << "时段: " << (of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << "机房: " << of.m_orderData[i]["roomId"];
string status = "状态: ";
if (of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
cout << status << endl;
}
}
cout << "请输入审核的预约记录,0代表反悔" << endl;
int select = 0;
int ret = 0;
while (true)
{
cin >> select;
if (select >= 0 && select <= v.size())
{
break;
}
else
{
cout << "请输入审核结果:" << endl;
cout << "1. 通过" << endl;
cout << "2. 不通过" << endl;
cin >> ret;
if (ret == 1)
{
of.m_orderData[v[select - 1]]["status"] = "2";
}
else
{
cout << "请输入审核结果" << endl;
cout << "1.通过" << endl;
cout << "2.不通过" << endl;
cin >> ret;
if (ret == 1)
{
of.m_orderData[v[select - 1]]["status"] = "2";
}
else
{
of.m_orderData[v[select - 1]]["status"] = "-1";
}
of.updateOrder();
cout << "审核完毕" << endl;
break;
}
}
cout << "输入有误,请重新输入" << endl;
}
system("pause");
system("cls");
}
|