?
#include<cstdio>
#include<cstdlib>
#include<iostream>
#include <iomanip>
using namespace std;
int main()
{
double a = 3.1415926;
cout << setprecision(2) << a << endl;
cout << fixed << setprecision(2) << a << endl;//设置精度语句写一次即可
double s = 121.345;
cout << s << endl;
cout << setprecision(3) << s << endl;
cout.setf(ios::showpoint);//输出小数点
cout << s << endl;
cout.setf(ios::showbase);
cout << s << endl;
//C语言中的内存申请
/*int* pc = (int*)malloc(sizeof(int));
free(pc);
pc = nullptr;
//C++中的内存申请 new delete
int* pcpp = new int; //申请内存
delete pcpp; //释放内存
pcpp = nullptr;
//申请一段内存
//C语言
int* pArrayC = (int*)malloc(sizeof(int) * 4);
free(pArrayC);
pArrayC = nullptr;
//C++
int* pArrayCPP = new int[4];
delete[] pArrayCPP;//数组(一段)内存的释放过程
pArrayCPP = nullptr;
char* str = new char[3];
delete[] str;
//申请内存并且做初始化 calloc
//赋值一个数据
int* pint = new int(100);
cout << *pint << endl;
cout << pint[0] << endl;//同上等价
delete pint;
pint = nullptr;
//赋值一堆数据*/
int* pArray = new int[4]{ 1,2,3,4 };
for (int i = 0; i < 4; i++)
{
cout << pArray[i] << "\t";
}
//delete[] pArray;
// pArray = nullptr;
return 0;
}
?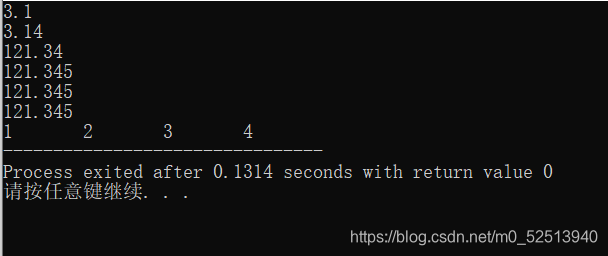
?
|