构造与析构
#include <iostream>
#include <string>
#include "stdio.h"
#include "string.h"
class stu {
public:
stu(std::string Name, int Age, char * Stu_ogo = nullptr) {
std::cout << "构造函数" << std::endl;
this->name = Name;
this->age = Age;
this->stu_logo = new char[strlen(Stu_ogo)+1];
strcpy(this->stu_logo, Stu_ogo);
}
stu(const stu& other_stu) {
std::cout << "拷贝构造函数" << std::endl;
this->name = other_stu.name;
this->age = other_stu.age;
this->stu_logo = new char[strlen(other_stu.stu_logo)+1];
strcpy(this->stu_logo, other_stu.stu_logo);
}
stu& operator=(const stu& other_stu) {
std::cout << "赋值构造函数" << std::endl;
if (this == &other_stu) {
return *this;
}
this->age = other_stu.age;
this->name = other_stu.name;
delete[] this->stu_logo; //释放之前创建的对象
this->stu_logo = nullptr;
this->stu_logo = new char[strlen(other_stu.stu_logo)+1];
strcpy(this->stu_logo, other_stu.stu_logo);
return *this;
}
~stu() {
std::cout << "析构函数" << std::endl;
delete[] this->stu_logo;
}
void show(){
std::cout << "姓名 = " << name << std::endl;
std::cout << " 年龄 = " << age << std::endl;
std::cout << " logo = " << stu_logo << std::endl;
}
private:
std::string name;
int age;
char* stu_logo;
};
int main()
{
{
char messg1[] = "hello world!";
char *logo1 = new char[strlen(messg1)+1];
strcpy(logo1, messg1);
stu demo1("张三",15, logo1);
char messg2[] = "bye bye~";
char *logo2 = new char[strlen(messg2)+1];
strcpy(logo2, messg2);
stu demo2("李四",17, logo2);
stu demo3(demo1);
demo3.show();
std::cout << std::endl;
demo1 = demo2;
demo1.show();
}
return 0;
}
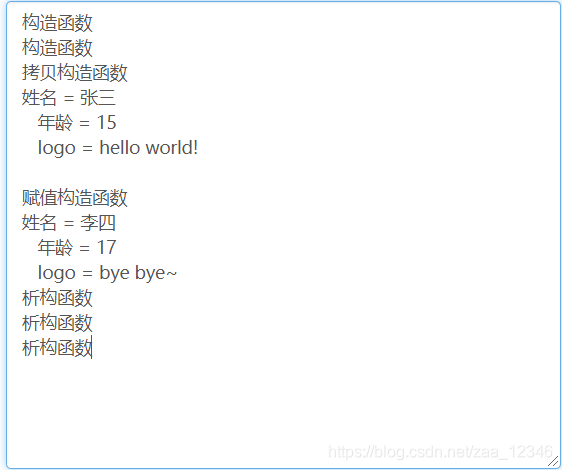
|