C++的正则表达式在regex头文件中,常用的表达式函数如下:
basic_regex | 正则表达式对象,是一个通用的模板,有typedef?basic_regex<char> regex 和 typedef basic_regex<char_t>wregex; | regex_match | 将一个字符序列和正则表达式匹配 | regex_search | 寻找字符序列中的子串中与正则表达式匹配的结果,在找到第一个匹配的结果后就会停止查找 | regex_replace | 使用格式化的替换文本,替换正则表达式匹配到字符序列的地方 | regex_iterator | 迭代器,用来匹配所有 的子串? | match_results | 容器类,保存正则表达式匹配的结果。 | sub_match | 容器类,保存子正则表达式匹配的字符序列. |
?使用regex_match匹配数字范围1-3500,小数点1-3位的小数。
直接上代码:
#include <regex>
#include <iostream>
#include <string>
using namespace std;
int main()
{
std::string tmp = "3500";
string tmp1 = "3501";
std::string pattern("[1-9]|[1-9][0-9]|[1-9][0-9][0-9]|[1-3][0-4][0-9][0-9]|[1-3][0-5][0][0]");
//匹配1-3500
std::regex r(pattern);
bool bTmpSucess = false;
smatch mathResult;
bTmpSucess = regex_match(tmp, mathResult,r); //保存匹配结果到mathResult
std::cout << "tmp result is " << (bTmpSucess == true ? "success" :"fail" )<< std::endl;
if (bTmpSucess)
{
for (int i = 0; i < mathResult.size();i++)
{
std::cout << "tmp result is " << mathResult.str(i) << std::endl;
}
}
bool bTmp1Success = false;
bTmp1Success = regex_match(tmp1, r);//不保存匹配结果
std::cout << "tmp1 result is " << (bTmp1Success == true ? "success" : "fail") << std::endl;
bool bNumMatchSuccess = false;
std::string strNum = "0.1266";
string strNum1 = "0.123";
string strNum2 = "0.1";
string strNum3 = "0.12";
std::string pattern1("[0][\.][0-9]{1,3}");//匹配小数点1-3位的小数
std::regex r1(pattern1);
bNumMatchSuccess = regex_match(strNum, r1);//false
bNumMatchSuccess = regex_match(strNum1, r1);//true
bNumMatchSuccess = regex_match(strNum2, r1);//true
bNumMatchSuccess = regex_match(strNum3, r1);//true
}
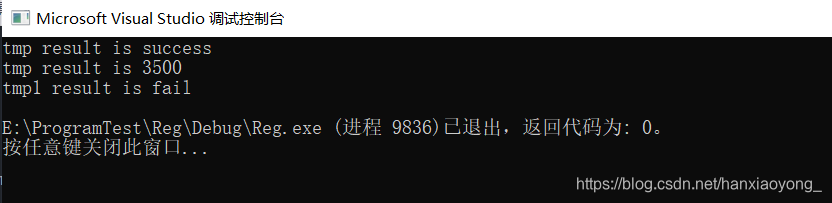
保存匹配结果的类型有下面几种,根据匹配的结果选择对应保存的类型:
typedef match_results<const wchar_t *> wcmatch; typedef match_results<string::const_iterator> smatch; typedef match_results<wstring::const_iterator> wsmatch;
匹配结果输出函数smatch.str(i)函数原型如下:
???????_NODISCARD string_type str(size_type _Sub = 0) const ?? ??? ?{?? ?// 返回匹配内容的子串 ?? ??? ?return (string_type((*this)[_Sub])); ?? ??? ?}
----------------------------------------------------------------------------------------------------------------------------
?后续再补充。
常用的正则表达式见:js正则表达式-匹配空格/数字范围/网址/电话等常用表达式_hanxiaoyong_的博客-CSDN博客_js正则表达式匹配空格
正则表达式匹配介绍的文章:C++中的也能使用正则表达式 - 我是一只C++小小鸟 - 博客园 (cnblogs.com)
|