学习笔记…
#include<stdio.h>
#include<stdlib.h>
#define Error( Str ) fprintf( stderr, "%s\n", Str ), exit( 1 )
#define EmptyTOS -1
#define MinStackSize 5
typedef int ElementType;
typedef struct StackRecord{
int TopOfStack;
int Capacity;
ElementType *array;
}*Stack;
int IsEmpty(Stack S)
{
return S->TopOfStack == EmptyTOS;
}
int IsFull(Stack S)
{
return S->TopOfStack == S->Capacity - 1;
}
void MakeEmpty(Stack S)
{
S->TopOfStack = EmptyTOS;
}
Stack CreateStack(int MaxElement)
{
Stack S;
if(MaxElement < MinStackSize){
Error("Stack Size is too small!");
}
S = malloc(sizeof(struct StackRecord));
if(S == NULL)
Error("Out of space!!!");
S->array = malloc(sizeof(ElementType) * MaxElement);
if(S->array == NULL)
Error("Out of space!");
S->Capacity = MaxElement;
MakeEmpty(S);
return S;
}
void Push(ElementType X, Stack S)
{
if(IsFull(S))
Error("Full Stack");
S->array[++S->TopOfStack] = X;
}
void Pop(Stack S)
{
if(IsEmpty(S))
Error("Empty Stack");
S->TopOfStack--;
}
ElementType TopAndPop(Stack S)
{
if(!IsEmpty(S))
return S->array[S->TopOfStack--];
else
{
Error("Empty Stack");
return 0;
}
}
void DisposeStack(Stack S)
{
if(S != NULL)
{
free(S->array);
free(S);
}
}
ElementType Top(Stack S)
{
if(!IsEmpty(S))
return S->array[S->TopOfStack];
else
{
Error("Empty Stack");
return 0;
}
}
void TravalStack(Stack S)
{
if(!IsEmpty(S)){
for(; S->TopOfStack != EmptyTOS; S->TopOfStack--){
printf("%d\n",Top(S));
}
}
else
Error("Empty Stack");
}
int main(){
Stack S;
S = CreateStack(10);
Push(7,S);
Push(6,S);
Push(5,S);
Push(4,S);
Push(3,S);
printf("%d\n",Top(S));
Pop(S);
Pop(S);
TravalStack(S);
DisposeStack(S);
return 0;
}
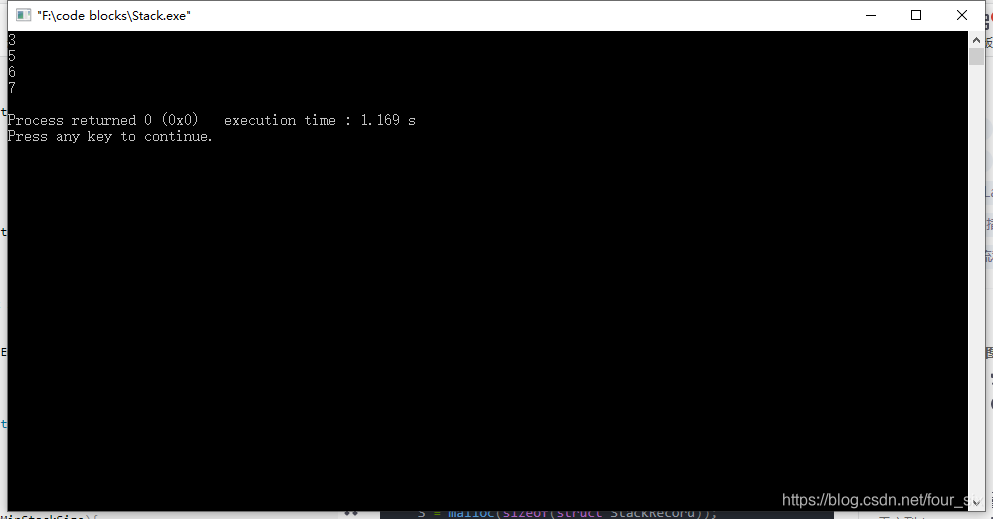 用数组实现栈花费时间为很小的常数 应该尽可能写出各种错误检测,如果错误检测的代码量确实很大,可以省略
|