//Observer.h
#pragma once
#include <stdio.h>
#include <mutex>
#include <list>
#include <iostream>
using namespace std;
class CObserver
{
public:
CObserver(int nType);
virtual ~CObserver() {};
virtual void OnNotify(int nEvent, const char* pMsg, int nLen) {};
virtual void SetType(int nType);
virtual int GetType() const;
private:
int m_nType;
};
CObserver::CObserver(int nType) :m_nType(nType)
{
//TODU
}
void CObserver::SetType(int nType)
{
m_nType = nType;
}
int CObserver::GetType() const
{
return m_nType;
}
class CSubject
{
public:
CSubject();
virtual ~CSubject();
virtual void Add(CObserver* po);
virtual void Del(CObserver* po);
virtual void Notify(CObserver* pSender, int nEvent, const char* pMsg, int dwLen);
void ClearObserver();
std::list<CObserver*>* GetObservers();
CObserver* GetObserver(int nType);
private:
list<CObserver*>* m_pListObserver;
mutex* m_pListLock;
};
CSubject::CSubject() :m_pListObserver(NULL), m_pListLock(NULL)
{
m_pListObserver = new list<CObserver*>;
m_pListLock = new mutex;
}
CSubject::~CSubject()
{
if(m_pListObserver)
{
m_pListObserver->clear();
delete m_pListObserver;
m_pListObserver = NULL;
}
if (m_pListLock)
{
delete m_pListLock;
m_pListLock = NULL;
}
}
void CSubject::Add(CObserver* po)
{
if (NULL == po)
{
return;
}
m_pListLock->lock();
if (find(m_pListObserver->begin(), m_pListObserver->end(), po) == m_pListObserver->end())
{
m_pListObserver->push_back(po);
}
m_pListLock->unlock();
}
void CSubject::Del(CObserver* po)
{
if (NULL == po)
{
return;
}
m_pListLock->lock();
if (find(m_pListObserver->begin(), m_pListObserver->end(), po) != m_pListObserver->end())
{
m_pListObserver->remove(po);
}
m_pListLock->unlock();
}
void CSubject::Notify(CObserver* pSender, int nEvevt, const char* pMsg, int dwLen)
{
if (NULL == pSender)
{
return;
}
m_pListLock->lock();
list<CObserver*>::iterator ite;
ite = m_pListObserver->begin();
while (ite != m_pListObserver->end())
{
CObserver* po = *(ite);
//if (po == pSender) && (po != NULL) //点对点通知 通知pSender
if ((pSender == NULL || po == pSender) && (po != NULL)) //广播
{
po->OnNotify(nEvevt, pMsg, dwLen);
}
ite++;
}
m_pListLock->unlock();
}
void CSubject::ClearObserver()
{
m_pListLock->lock();
m_pListObserver->clear();
m_pListLock->unlock();
}
list<CObserver*>* CSubject::GetObservers()
{
return m_pListObserver;
}
CObserver* CSubject::GetObserver(int nType)
{
m_pListLock->lock();
list<CObserver*>::iterator ite;
ite = m_pListObserver->begin();
while (ite != m_pListObserver->end())
{
CObserver* po = *(ite);
if (po != NULL && po->GetType() == nType)
{
return po;
}
ite++;
}
m_pListLock->unlock();
return NULL;
}
//派生类
class CMyObserver : public CObserver
{
public:
CMyObserver(int nType);
void OnNotify(int nEvent, const char* pMsg, int nLen);
};
CMyObserver::CMyObserver(int nType) : CObserver(nType) {}
void CMyObserver::OnNotify(int nEvent, const char* pMsg, int nLen)
{
cout << nEvent << endl;
cout << pMsg << endl;
cout << nLen << endl;
}
//测试例
#include <stdlib.h>
#include "Observer.h"
int main()
{
/****************** 观察者模式验证 **********************/
CObserver* po1 = new CMyObserver(1);
CObserver* po2 = new CMyObserver(2);
CSubject* psub = new CSubject();
psub->Add(po1);
psub->Add(po2);
psub->Notify(po1,2021,"hello world",12);
psub->Notify(po2, 2022, "hello world", 12);
}
//测试结果截图? 消息通知成功
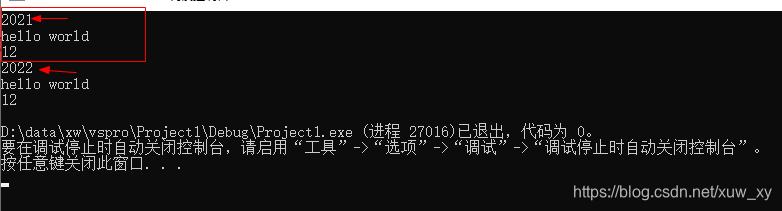
?
?注:观察者模式 vs 发布订阅模式 介绍可参考下述链接
参考:https://zhuanlan.zhihu.com/p/51357583
|