map基本概念: map中所有元素都是pair对组 pair中第一个元素为key(键值),起到索引作用,第二个元素为value(实值) 所有元素都会根据元素的键值自动排序
本质: map/multimap属于关联式容器,底层结构是用二叉树实现。
优点: 可以根据key值快速找到value值
map和multimap区别: map不允许容器中有重复key值元素 multimap允许容器中有重复key值元素
构造: map<T1, T2> mp; //map默认构造函数: map(const map &mp); //拷贝构造函数
赋值: map& operator=(const map &mp); //重载等号操作符
#include <iostream>
#include <map>
using namespace std;
void printmap(const map<int, int>&m)
{
for (map<int, int>::const_iterator it = m.begin(); it != m.end(); it++) {
cout << "键值: " << it->first << " 值: " << it->second << endl;
}
cout << endl;
}
void test()
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));
m1.insert(pair<int, int>(3, 20));
m1.insert(pair<int, int>(2, 30));
m1.insert(pair<int, int>(4, 40));
m1.insert(pair<int, int>(5, 50));
printmap(m1);
map<int, int>m2(m1);
printmap(m2);
map<int, int>m3;
m3 = m2;
printmap(m3);
}
int main()
{
test();
system("pause");
return 0;
}
map大小和交换:
size(); //返回容器中元素的数目 empty(); //判断容器是否为空 swap(st); //交换两个集合容器
map插入和删除:
insert(elem); //在容器中插入元素。 clear(); //清除所有元素 erase(pos); //删除pos迭代器所指的元素,返回下一个元素的迭代器。 erase(beg, end); //删除区间[beg,end)的所有元素 ,返回下一个元素的迭代器。 erase(key); //删除容器中值为key的元素。
map插入的多种方式:
#include <iostream>
#include <map>
using namespace std;
void printmap(map<int, int>&m)
{
for (map<int, int>::iterator it = m.begin(); it != m.end(); it++) {
cout << "key: " << it->first << " value: " << it->second << endl;
}
}
void test()
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));
m1.insert(make_pair(2, 20));
m1.insert(map<int, int>::value_type(3, 30));
m1[4] = 40;
cout << m1[5] << endl;
printmap(m1);
}
int main()
{
test();
system("pause");
return 0;
}
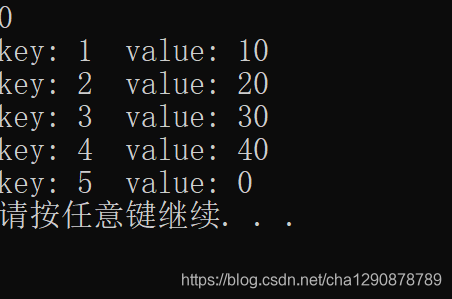 map查找和统计
find(key); //查找key是否存在,若存在,返回该键的元素的迭代器;若不存在,返回set.end(); count(key); //统计key的元素个数(对于map由于不允许有重复元素,所以find只能是0或1;但是multimap 统计可能不为0和1)
使用find加一个判断条件,如果find返回的是m.end(),就说明没有找到,如果不为m.end()说明找到
map容器排序 利用仿函数
#include <iostream>
#include <map>
using namespace std;
class compare {
public:
bool operator() (int data1, int data2)
{
return data1 > data2;
}
};
void test01()
{
map<int, int,compare>m1;
m1.insert(make_pair(1, 10));
m1.insert(make_pair(3, 30));
m1.insert(make_pair(2, 20));
m1.insert(make_pair(5, 50));
m1.insert(make_pair(4, 40));
cout << "降序排序" << endl;
for (map<int, int>::iterator it = m1.begin(); it != m1.end(); it++) {
cout << "key: " << it->first << " value: " << it->second << endl;
}
}
void test02()
{
map<int, int>m1;
m1.insert(make_pair(1, 10));
m1.insert(make_pair(3, 30));
m1.insert(make_pair(2, 20));
m1.insert(make_pair(5, 50));
m1.insert(make_pair(4, 40));
cout << "默认排序" << endl;
for (map<int, int>::iterator it = m1.begin(); it != m1.end(); it++) {
cout << "key: " << it->first << " value: " << it->second << endl;
}
}
int main()
{
test02();
test01();
system("pause");
return 0;
}
|