// StudyThread_2.cpp : 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include <thread>
#include <iostream>
#include <future>
//简单函数
int addFunc(int i, int j)
{
std::cout << "sub thread:" << std::this_thread::get_id() << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
return i + j;
}
//复杂函数: 求阶乘
long long factorial(int x)
{
std::cout << "sub thread:" << std::this_thread::get_id() << std::endl;
long long f;
if (x == 0 || x == 1)
f = 1;
else
f = factorial(x - 1)*x;
std::cout << "factorial finish..." << std::endl;
return f;
}
void printSomething()
{}
int main()
{
std::cout << "Main thread:" << std::this_thread::get_id() << std::endl;
//直接使用std::thread启动线程,我们无法获取到线程的返回值
// std::thread t(addFunc, 1, 2);
// if (t.joinable())
// {
// t.join();
// }
//---------------------------------------------------------------------------------------------------------
//这个时候我们引入另一种启动线程的方式std::async
//注意std::async的返回值是std::future
// int a = 1;
// int b = 2;
// std::future<int> iResFut = std::async(std::launch::async, addFunc, a, b);
// std::cout << "iResFut.vaild() = " << iResFut.valid() << std::endl; //true:由std::asyc返回的std::future,其vaild是true
// iResFut.wait();
// std::cout << "iResFut.vaild() = " << iResFut.valid() << std::endl; //true:wait()返回之后,vaild为true
// int c = iResFut.get(); //使用get()获取future中的值
// std::cout << "iResFut.vaild() = " << iResFut.valid() << std::endl; //false:在对std::future调用get()之后,vaild为false
// //int d = iResFut.get();
// std::cout << a << "+" << b << "=" << c << std::endl;
//---------------------------------------------------------------------------------------------------------
//std::future<int> fut;
//std::cout << fut.valid() << std::endl; //false:直接构造一个future对象,它没有关联任何共享状态,vaild是false
//std::cout << fut.get() << std::endl; //此时会抛出异常
//---------------------------------------------------------------------------------------------------------
//注意std::shared_future的使用
// int a = 5, b = 6;
// std::future<int> iResFut = std::async(std::launch::async, addFunc, a, b);
// iResFut.wait();
// int c = iResFut.get();
// std::cout << a << "+" << b << "=" << c << std::endl;
// std::shared_future<long long> iFacFut = std::async(std::launch::async, factorial, c);
// iFacFut.wait();
// std::cout << "iFacFut.vaild() = " << iFacFut.valid() << std::endl;
// long long d = iFacFut.get();
// std::cout << c << " 的阶乘= " << d << std::endl;
// std::cout << "iFacFut.vaild() = " << iFacFut.valid() << std::endl;
// long long e = iFacFut.get();
// std::cout << c << " 的阶乘= " << e << std::endl;
// long long f = iFacFut.get();
// std::cout << c << " 的阶乘= " << f << std::endl;
//---------------------------------------------------------------------------------------------------------
//std::lauch::deferred
// int a = 10;
// std::future<long long> iResFut = std::async(std::launch::deferred, factorial, a);
// iResFut.wait();
// std::cout << a << " 的阶乘= " << iResFut.get() << std::endl;
//-------------------------------------------------------------------------------
std::cout << "I Love China" << std::endl;
system("pause");
return 0;
}
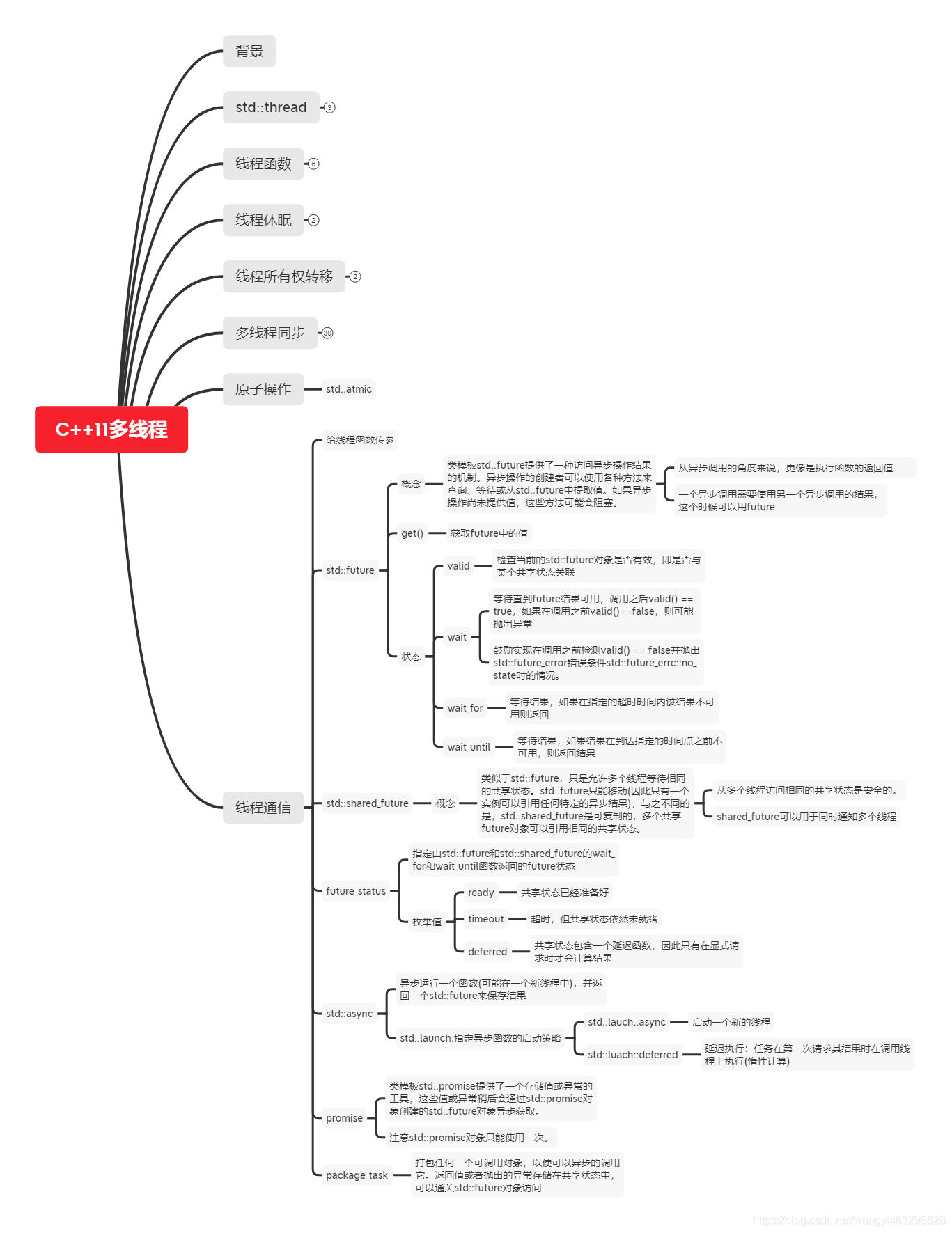
|