//本博主所写的代码仅为阅读者提供参考;
//若有不足之处请提出,博主会尽所能修改;
//附上课后编程练习题目;
//若是对您有用的话请点赞或分享提供给它人
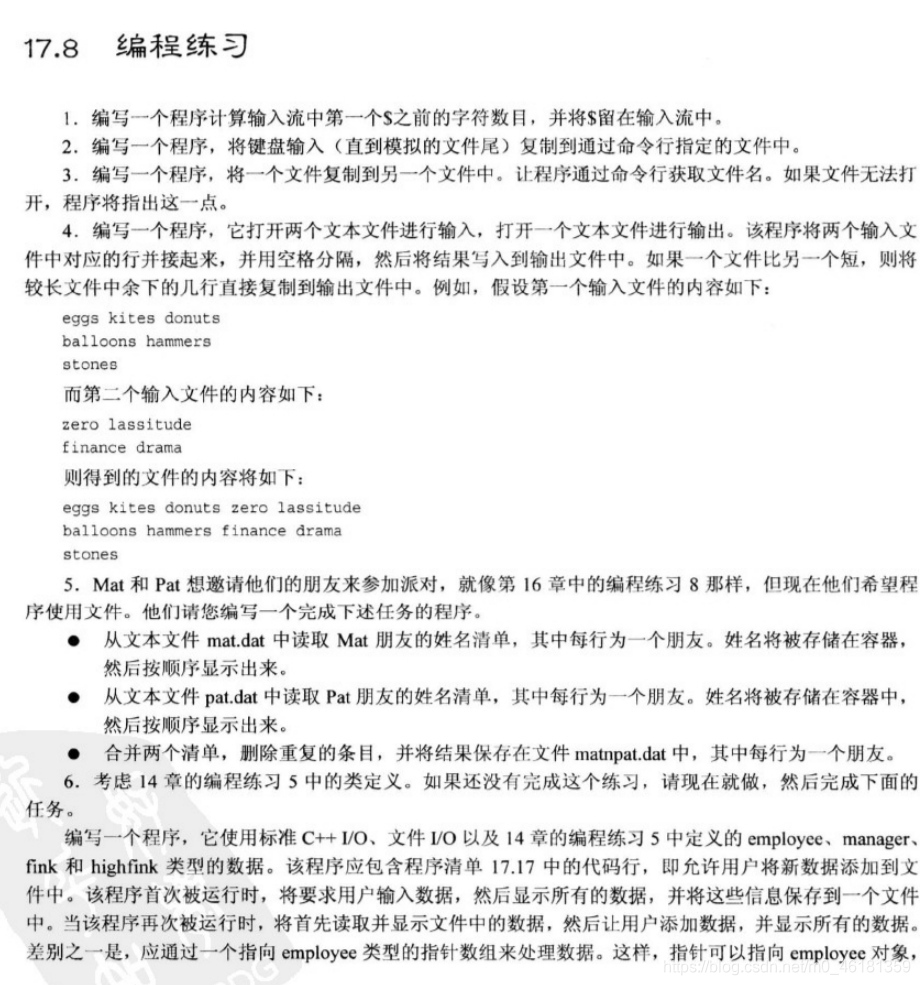
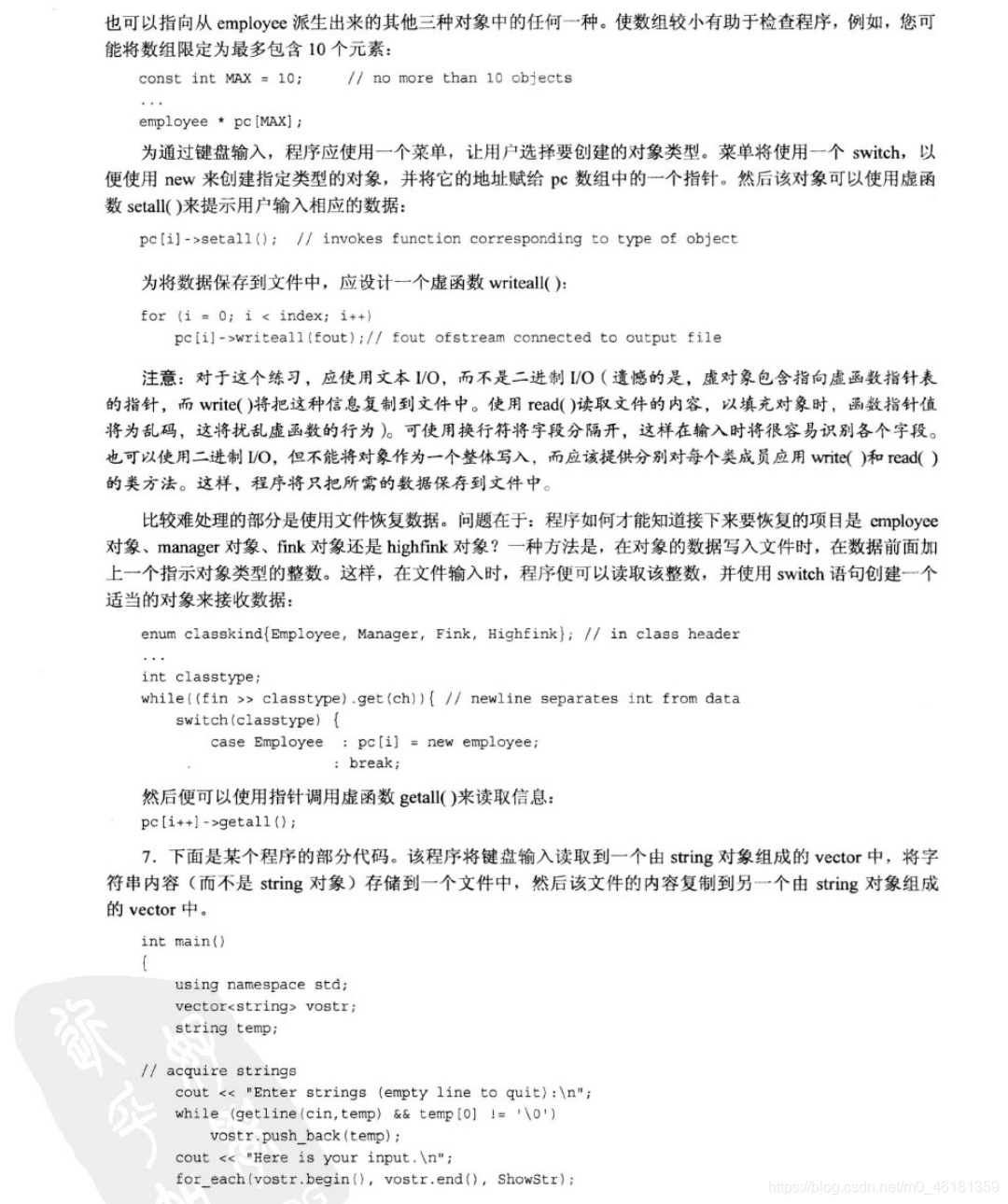
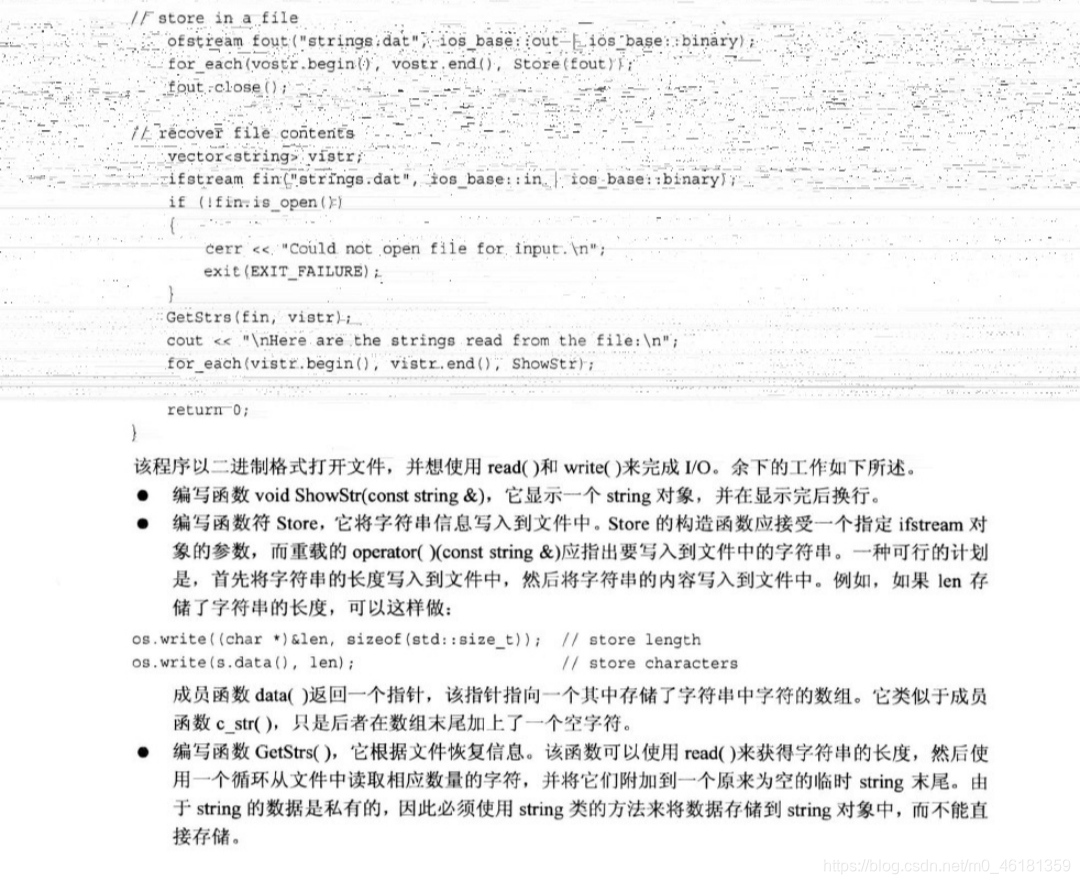 //17.8 - 1.cpp
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = 256;
int main()
{
char ch, str[MAXN];
cout << "Please enter a string ($ to quit): ";
cin.get(str, MAXN, '$');
cout << "Your input: " << str << endl;
cout << "Length: " << strlen(str) << endl;
cout << "Other messages: ";
while ((ch = cin.get()) != '\n')
{
cout << ch;
}
return 0;
}
//---------------------------------------------------------------------------------------------------------
//17.8 - 2.cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{
if (argc != 2)
{
cerr << "Your command arguments are invalid!" << endl;
exit(EXIT_FAILURE);
}
char ch;
ofstream fout(argv[1]);
if (!fout.is_open())
{
cerr << "Can't open the file " << argv[1] << endl;
exit(EXIT_FAILURE);
}
cout << "Please enter some characters: ";
while ((ch = cin.get()) != EOF)
{
fout << ch;
}
cout << "Done.\n";
fout.close();
return 0;
}
//---------------------------------------------------------------------------------------------------------
//17.8 - 3.cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{
if (argc != 3)
{
cerr << "Your command arguments are invalid!" << endl;
exit(EXIT_FAILURE);
}
char ch;
ifstream fin(argv[1]);
ofstream fout(argv[2]);
if (!fin.is_open())
{
cerr << "Can't open the file " << argv[1] << endl;
exit(EXIT_FAILURE);
}
if (!fout.is_open())
{
cerr << "Can't open the file " << argv[2] << endl;
exit(EXIT_FAILURE);
}
while (fin >> ch)
{
fout << ch;
}
cout << "Done.\n";
fin.close();
fout.close();
return 0;
}
//---------------------------------------------------------------------------------------------------------
//17.8 - 4.cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <vector>
#include <string>
using namespace std;
int main()
{
string s;
vector<string> ans1;
vector<string> ans2;
ifstream fin1("in1.txt");
ifstream fin2("in2.txt");
ofstream fout("out.txt");
if (!fin1.is_open())
{
cout << "Can't open the file in1.txt\n";
exit(EXIT_FAILURE);
}
if (!fin2.is_open())
{
cout << "Can't open the file in2.txt\n";
exit(EXIT_FAILURE);
}
if (!fout.is_open())
{
cout << "Can't open the file out.txt\n";
exit(EXIT_FAILURE);
}
while (getline(fin1, s))
{
ans1.push_back(s);
}
while (getline(fin2, s))
{
ans2.push_back(s);
}
for (int i = 0; i < ans1.size() || i < ans2.size(); ++i)
{
if (i >= ans1.size())
{
fout << ans2[i] << endl;
}
else if (i >= ans2.size())
{
fout << ans1[i] << endl;
}
else
{
fout << ans1[i] << ' ' << ans2[i] << endl;
}
}
fin1.close();
fin2.close();
fout.close();
return 0;
}
//---------------------------------------------------------------------------------------------------------
//17.8 - 5.cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <set>
#include <string>
#include <iterator>
#include <algorithm>
using namespace std;
int main()
{
string name;
set<string> mat_friends;
set<string> pat_friends;
ifstream fin1("mat.dat");
ifstream fin2("pat.dat");
ofstream fout("matnpat.dat");
ostream_iterator<string> out1(cout, "\n");
ostream_iterator<string> out2(fout, "\n");
if (!fin1.is_open())
{
cerr << "Can't open the file mat.dat\n";
exit(EXIT_FAILURE);
}
if (!fin2.is_open())
{
cerr << "Can't open the file pat.dat\n";
exit(EXIT_FAILURE);
}
if (!fout.is_open())
{
cerr << "Can't open the file matnpat.dat\n";
exit(EXIT_FAILURE);
}
while (getline(fin1, name))
{
mat_friends.insert(name);
}
while (getline(fin2, name))
{
pat_friends.insert(name);
}
cout << "Here are the Mat's friends:" << endl;
copy(mat_friends.begin(), mat_friends.end(), out1);
cout << "Here are the Pat's friends:" << endl;
copy(pat_friends.begin(), pat_friends.end(), out1);
set_union(mat_friends.begin(), mat_friends.end(), pat_friends.begin(), pat_friends.end(), out2);
cout << "\nDone.\n";
fin1.close();
fin2.close();
fout.close();
return 0;
}
//---------------------------------------------------------------------------------------------------------
//第6题需创建工程项目文件后才能实现完整功能; //17.8 - 6(main).cpp
#include <iostream>
#include <cstdlib>
#include "emp.h"
using namespace std;
const int MAX = 10;
const char *emp_str[4] = {"Employee", "Manager", "Fink", "Highfink"};
int main()
{
char ch;
abstr_emp *pc[MAX];
ifstream fin("test.txt");
if (fin.is_open())
{
cout << "Here are the current contents of the test.txt file:";
int classtype;
int i = 0;
while ((fin >> classtype).get(ch))
{
switch (classtype)
{
case Employee:
{
pc[i] = new employee;
break;
}
case Manager:
{
pc[i] = new manager;
break;
}
case Fink:
{
pc[i] = new fink;
break;
}
case Highfink:
{
pc[i] = new highfink;
break;
}
}
cout << endl;
cout << emp_str[classtype] << endl;
pc[i]->getall(fin);
pc[i++]->ShowAll();
}
}
fin.close();
ofstream fout("test.txt", ios_base::out | ios_base::app);
if (!fout.is_open())
{
cerr << "Can't open the file test.txt.\n";
exit(EXIT_FAILURE);
}
cout << "Enter the employee category:\n";
cout << "e: employee\n";
cout << "m: manager\n";
cout << "f: fink\n";
cout << "h: highfink\n";
cout << "q: quit\n";
int i = 0, index = 0;
while (cin.get(ch).get() && ch != 'q' && index < MAX)
{
switch (ch)
{
case 'E':
case 'e':
{
pc[index] = new employee;
pc[index++]->SetAll();
break;
}
case 'M':
case 'm':
{
pc[index] = new manager;
pc[index++]->SetAll();
break;
}
case 'F':
case 'f':
{
pc[index] = new fink;
pc[index++]->SetAll();
break;
}
case 'H':
case 'h':
{
pc[index] = new highfink;
pc[index++]->SetAll();
break;
}
default:
{
cout << "Try again: ";
break;
}
}
cout << "Enter the employee category:\n";
cout << "e: employee\n";
cout << "m: manager\n";
cout << "f: fink\n";
cout << "h: highfink\n";
cout << "q: quit\n";
}
for (i = 0; i < index; ++i)
{
pc[i]->writeall(fout);
}
fout.close();
cout << "Done.\n";
return 0;
}
//17.8 - 6(emp).h
#ifndef EMP_H_
#define EMP_H_
#include <iostream>
#include <fstream>
#include <string>
enum classkind
{
Employee,
Manager,
Fink,
Highfink
};
class abstr_emp
{
private:
std::string fname;
std::string lname;
std::string job;
public:
abstr_emp();
abstr_emp(const std::string &fn, const std::string &ln, const std::string &j);
virtual void ShowAll() const;
virtual void SetAll();
friend std::ostream &operator<<(std::ostream &os, const abstr_emp &e);
virtual ~abstr_emp() = 0;
virtual void writeall(std::ofstream &os);
virtual void getall(std::ifstream &is);
};
class employee : public abstr_emp
{
public:
employee();
employee(const std::string &fn, const std::string &ln, const std::string &j);
virtual void ShowAll() const;
virtual void SetAll();
virtual void writeall(std::ofstream &os);
virtual void getall(std::ifstream &is);
};
class manager : virtual public abstr_emp
{
private:
int inchargeof;
protected:
int InChargeOf() const { return inchargeof; }
int &InChargeOf() { return inchargeof; }
public:
manager();
manager(const std::string &fn, const std::string &ln, const std::string &j, int ico = 0);
manager(const abstr_emp &e, int ico);
manager(const manager &m);
virtual void ShowAll() const;
virtual void SetAll();
virtual void writeall(std::ofstream &os);
virtual void getall(std::ifstream &is);
};
class fink : virtual public abstr_emp
{
private:
std::string reportsto;
protected:
const std::string ReportsTo() const { return reportsto; }
std::string &ReportsTo() { return reportsto; }
public:
fink();
fink(const std::string &fn, const std::string &ln, const std::string &j, const std::string &rpo);
fink(const abstr_emp &e, const std::string &rpo);
fink(const fink &e);
virtual void ShowAll() const;
virtual void SetAll();
virtual void writeall(std::ofstream &os);
virtual void getall(std::ifstream &is);
};
class highfink : public manager, public fink
{
public:
highfink();
highfink(const std::string &fn, const std::string &ln, const std::string &j, const std::string &rpo, int ico);
highfink(const abstr_emp &e, const std::string &rpo, int ico);
highfink(const fink &f, int ico);
highfink(const manager &m, const std::string &rpo);
highfink(const highfink &h);
virtual void ShowAll() const;
virtual void SetAll();
virtual void writeall(std::ofstream &os);
virtual void getall(std::ifstream &is);
};
#endif
//17.8 - 6(emp).cpp
#include <iostream>
#include "emp.h"
using std::cin;
using std::cout;
using std::endl;
abstr_emp::abstr_emp() : fname("no"), lname("one"), job("none")
{
}
abstr_emp::abstr_emp(const std::string &fn, const std::string &ln,
const std::string &j) : fname(fn), lname(ln), job(j)
{
}
void abstr_emp::ShowAll() const
{
cout << "First name: " << fname << endl;
cout << "Last name: " << lname << endl;
cout << "Job: " << job << endl;
return;
}
void abstr_emp::SetAll()
{
cout << "Please enter your firstname: ";
getline(cin, fname);
cout << "Please enter your lastname: ";
getline(cin, lname);
cout << "Please enter your job: ";
getline(cin, job);
return;
}
std::ostream &operator<<(std::ostream &os, const abstr_emp &e)
{
os << "\nFirst name: " << e.fname << endl;
os << "Last name: " << e.lname << endl;
os << "Job: " << e.job;
return os;
}
abstr_emp::~abstr_emp()
{
}
void abstr_emp::writeall(std::ofstream &os)
{
os << fname << endl;
os << lname << endl;
os << job << endl;
return;
}
void abstr_emp::getall(std::ifstream &is)
{
std::getline(is, fname);
std::getline(is, lname);
std::getline(is, job);
return;
}
employee::employee() : abstr_emp()
{
}
employee::employee(const std::string &fn, const std::string &ln, const std::string &j) : abstr_emp(fn, ln, j)
{
}
void employee::ShowAll() const
{
abstr_emp::ShowAll();
return;
}
void employee::SetAll()
{
abstr_emp::SetAll();
return;
}
void employee::writeall(std::ofstream &os)
{
os << Employee << endl;
abstr_emp::writeall(os);
return;
}
void employee::getall(std::ifstream &is)
{
abstr_emp::getall(is);
return;
}
manager::manager() : abstr_emp(), inchargeof(0) {}
manager::manager(const std::string &fn, const std::string &ln, const std::string &j, int ico) : abstr_emp(fn, ln, j), inchargeof(ico)
{
}
manager::manager(const abstr_emp &e, int ico) : abstr_emp(e), inchargeof(ico)
{
}
manager::manager(const manager &m) : abstr_emp(m), inchargeof(m.inchargeof)
{
}
void manager::ShowAll() const
{
abstr_emp::ShowAll();
cout << "In charge of: " << inchargeof << endl;
return;
}
void manager::SetAll()
{
abstr_emp::SetAll();
cout << "Please enter a number for inchargerof: ";
cin >> inchargeof;
while (cin.get() != '\n')
continue;
return;
}
void manager::writeall(std::ofstream &os)
{
os << Manager << endl;
abstr_emp::writeall(os);
os << inchargeof << endl;
return;
}
void manager::getall(std::ifstream &is)
{
abstr_emp::getall(is);
is >> inchargeof;
return;
}
fink::fink() : abstr_emp(), reportsto("none")
{
}
fink::fink(const std::string &fn, const std::string &ln,
const std::string &j, const std::string &rpo) : abstr_emp(fn, ln, j), reportsto(rpo)
{
}
fink::fink(const abstr_emp &e, const std::string &rpo) : abstr_emp(e), reportsto(rpo)
{
}
fink::fink(const fink &e) : abstr_emp(e), reportsto(e.reportsto) {}
void fink::ShowAll() const
{
abstr_emp::ShowAll();
cout << "Reports to: " << reportsto << endl;
return;
}
void fink::SetAll()
{
abstr_emp::SetAll();
cout << "Please enter a string for reportsto: ";
getline(cin, reportsto);
return;
}
void fink::writeall(std::ofstream &os)
{
os << Fink << endl;
abstr_emp::writeall(os);
os << reportsto << endl;
return;
}
void fink::getall(std::ifstream &is)
{
abstr_emp::getall(is);
getline(is, reportsto);
return;
}
highfink::highfink() : abstr_emp(), manager(), fink()
{
}
highfink::highfink(const std::string &fn, const std::string &ln,
const std::string &j, const std::string &rpo,
int ico) : abstr_emp(fn, ln, j), manager(fn, ln, j, ico), fink(fn, ln, j, rpo)
{
}
highfink::highfink(const abstr_emp &e, const std::string &rpo, int ico) : abstr_emp(e), manager(e, ico), fink(e, rpo)
{
}
highfink::highfink(const fink &f, int ico) : abstr_emp(f), manager(f, ico), fink(f)
{
}
highfink::highfink(const manager &m, const std::string &rpo) : abstr_emp(m), manager(m), fink(m, rpo)
{
}
highfink::highfink(const highfink &h) : abstr_emp(h), manager(h), fink(h)
{
}
void highfink::ShowAll() const
{
abstr_emp::ShowAll();
cout << "In charge of: " << manager::InChargeOf() << endl;
cout << "Reports to: " << fink::ReportsTo() << endl;
return;
}
void highfink::SetAll()
{
abstr_emp::SetAll();
cout << "Please enter a number for inchargerof: ";
cin >> InChargeOf();
while (cin.get() != '\n')
continue;
cout << "Please enter a string for reportsto: ";
getline(cin, ReportsTo());
return;
}
void highfink::writeall(std::ofstream &os)
{
os << Highfink << endl;
abstr_emp::writeall(os);
os << InChargeOf() << endl;
os << ReportsTo() << endl;
return;
}
void highfink::getall(std::ifstream &is)
{
abstr_emp::getall(is);
is >> InChargeOf();
is >> ReportsTo();
return;
}
//---------------------------------------------------------------------------------------------------------
//17.8 - 7.cpp
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
class Store
{
private:
ofstream &fout;
public:
Store(ofstream &os) : fout(os) {}
void operator()(const string &s)
{
auto len = s.size();
fout.write((char *)&len, sizeof(size_t));
fout.write(s.data(), len);
return;
}
};
void ShowStr(const string &s);
void GetStrs(ifstream &is, vector<string> &s);
int main()
{
using namespace std;
vector<string> vostr;
string temp;
cout << "Enter strings (empty line to quit):\n";
while (getline(cin, temp) && temp[0] != '\0')
{
vostr.push_back(temp);
}
cout << "Here is your input.\n";
for_each(vostr.begin(), vostr.end(), ShowStr);
ofstream fout("strings.dat", ios_base::out | ios_base::binary);
for_each(vostr.begin(), vostr.end(), Store(fout));
fout.close();
vector<string> vistr;
ifstream fin("strings.dat", ios_base::in | ios_base::binary);
if (!fin.is_open())
{
cerr << "Coult not open file for input.\n";
exit(EXIT_FAILURE);
}
GetStrs(fin, vistr);
cout << "\nHere are the strings read from the file:\n";
for_each(vistr.begin(), vistr.end(), ShowStr);
return 0;
}
void ShowStr(const string &s)
{
cout << s << endl;
return;
}
void GetStrs(ifstream &is, vector<string> &s)
{
char *tmp;
size_t len;
while (is.read((char *)&len, sizeof(size_t)))
{
tmp = new char[len + 1];
is.read(tmp, len);
tmp[len] = '\0';
s.push_back(tmp);
}
return;
}
//-----------------------------------------------------------------
//------------------------------------------2021年8月5日 ----------------------------------------------
|