注意事项
- linux的x86和arm64架构下均测试通过。
- 程序包含的两个头文件<termios.h> 和<asm/termbits.h>都定义了struct termios,需要将<asm/termbits.h>中的注释掉。
- 先以标准波特率打开串口,再通过termios2 配合ioctrl修改自定义波特率。
- 确保打开串口权限 sudo chmod 777 /dev/ttyUSB0
c++代码
使用SerialInit(int &fd, const char* devname, int baude)完成串口打开与波特率自定义。
- uart.hpp
#ifndef UART_HPP_
#define UART_HPP_
#include <termios.h>
#include <linux/serial.h>
class Uart{
public:
Uart(){}
~Uart(){}
int SerialInit(int &fd, const char* devname, int baude);
private:
struct termios uart;
int baude_changed(int &fd,int speed);
int serial_set_speci_baud(int &fd,int baude);
int setCustomBaudRate(int&fd,int baude);
};
#endif
- uart.cpp
#include <iostream>
#include <errno.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <asm/termbits.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <string.h>
#include "uart.hpp"
int Uart::SerialInit(int &fd, const char* devname, int baude){
fd = open(devname, O_RDWR | O_NOCTTY | O_NDELAY);
if (-1 == fd)
{
printf("Open %s Error!\n",devname);
perror("\n");
return -1;
}
if ((fcntl(fd, F_SETFL, 0)) < 0)
{
perror("Fcntl F_SETFL Error!\n");
return -1;
}
if (tcgetattr(fd, &uart) != 0)
{
perror("tcgetattr error!\n");
return -1;
}
cfmakeraw(&uart);
cfsetispeed(&uart, B115200);
cfsetospeed(&uart, B115200);
uart.c_cflag |= CLOCAL | CREAD;
uart.c_iflag &= ~ICRNL;
uart.c_iflag &= ~ISTRIP;
uart.c_cflag &= ~PARENB;
uart.c_cflag &= ~CSTOPB;
uart.c_cflag &= ~CSIZE;
uart.c_cflag |= CS8;
uart.c_cflag &= ~CRTSCTS;
uart.c_cc[VTIME] = 0;
uart.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
if (tcsetattr(fd, TCSANOW, &uart) != 0)
{
perror("tcsetattr Error!\n");
return -1;
}
baude_changed(fd,baude);
return 1;
}
int Uart::setCustomBaudRate(int &fd,int baude){
}
int Uart::serial_set_speci_baud(int &fd,int baude){
}
int Uart::baude_changed(int &fd,int speed)
{
struct termios2 tio = {0};
tio.c_cflag = BOTHER | CS8 | CLOCAL | CREAD;
tio.c_iflag = IGNPAR;
tio.c_oflag = 0;
tio.c_ispeed = speed;
tio.c_ospeed = speed;
return ioctl(fd, TCSETS2, &tio);
}
linux实现100k波特率的SBUS协议10通道解析
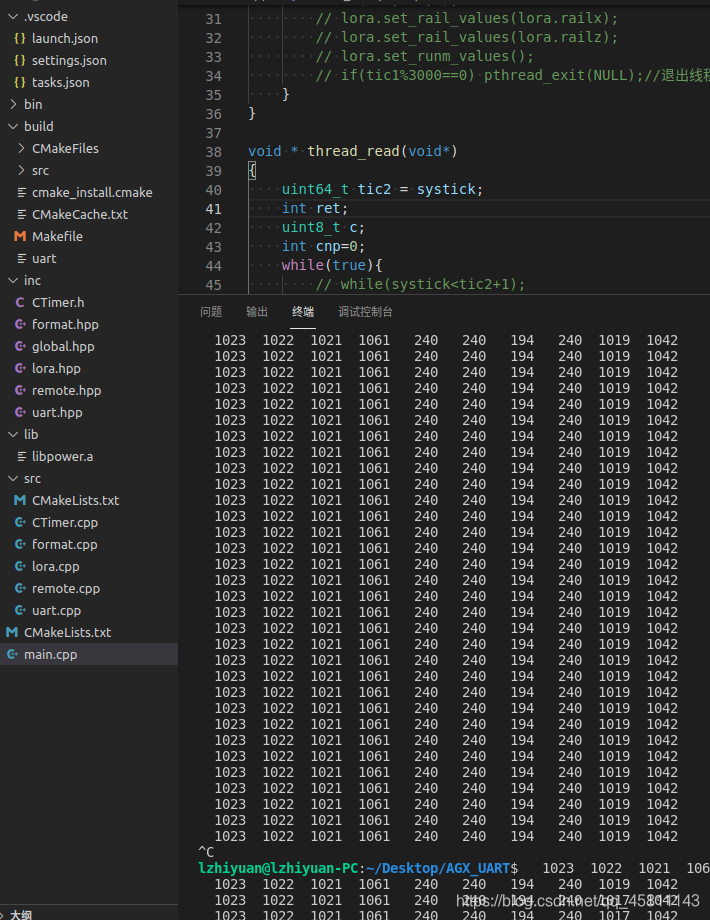
|