思路+代码解析
状态转移方程
1、如果只有一家的话,就只能抢这一家,即最优解。 2、如果只有两家的话,最优解为这两家钱最多的一家 3、如果有多于两家的话,关键点为第(n-1)家。如果抢了第(n-1)家,说明在前(n-1)家里已经求得最优解。如果没抢第(n-1)家,说明在前(n-1)家里,小偷在前(n-2)家已经求得最优解,所以小偷也一定会去第n家。那么在n家里最优解为f(n-2)+M(n)。所以在n家里,最优解为f(n-1)和f(n-2)+M(n)中最大值。 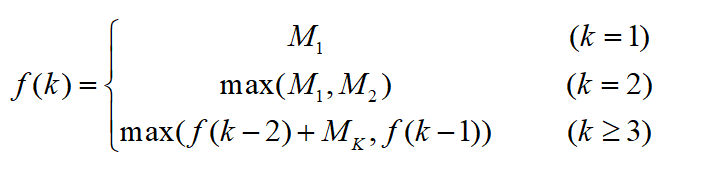
递归算法
int djjs_dg(vector<int> v)
{
int len = v.size();
if (len == 1)
{
return v.at(0);
}
else if (len == 2)
{
return max(v.at(0), v.at(1));
}
else
{
vector<int> v1;
vector<int> v2;
v1.assign(v.begin(), v.end() - 1);
v2.assign(v.begin(), v.end() - 2);
return max(djjs_dg(v1), djjs_dg(v2) + v.at(len - 1));
}
}
DP算法+输出抢的房屋号
vector<int> dp_v;
vector<int> IND;
vector<int> DP;
int djjs_dp(vector<int> v)
{
int len = v.size();
if (len == 1)
{
IND.push_back(0);
return v[0];
}
else if (len == 2)
{
if (v[0] > v[1])
{
IND.push_back(0);
}
else
{
IND.push_back(1);
}
return max(v[0], v[1]);
}
else
{
dp_v.push_back(v[0]);
dp_v.push_back(max(v[0], v[1]));
for (int i = 2; i < len; i++)
{
dp_v.push_back(max(dp_v[i - 1], dp_v[i - 2] + v[i]));
}
DP = dp_v;
cout << "DP数组为:" << endl;
for (vector<int>::iterator it = DP.begin(); it != DP.end(); it++)
{
cout << *it << " ";
}
cout << endl;
auto maxPosition = max_element(dp_v.begin(), dp_v.end());
int po = maxPosition - dp_v.begin();
IND.push_back(po);
while (dp_v[po] > v[po])
{
int m = dp_v[po] - v[po];
vector<int>::iterator it = find(dp_v.begin(), dp_v.end(), m);
po = it - dp_v.begin();
IND.push_back(po);
}
return dp_v[len - 1];
}
}
main
void main(){
vector<int> v;
v.push_back(2);
v.push_back(11);
v.push_back(8);
v.push_back(6);
v.push_back(10);
v.push_back(2);
v.push_back(6);
v.push_back(7);
v.push_back(8);
v.push_back(2);
cout << "初始位置的各金额为:" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
cout << "DP最优解为:" << djjs_dp(v) << endl;
reverse(IND.begin(), IND.end());
cout << "抢了超市的位置是:" << endl;
for (vector<int>::iterator it = IND.begin(); it != IND.end(); it++)
{
cout << *it + 1 << " ";
}
cout << endl;
}
|