文件操作
文件分类
- 文本文件
- 二进制文件
文件的打开方式
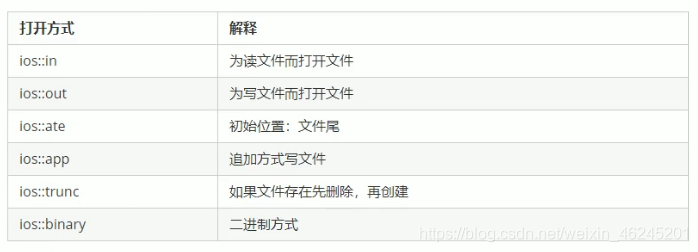
操作文件的三大类
- ofstream:写操作
- ifstream:读操作
- fstream:读写操作
文本文件
写文本文件
#include<iostream>
using namespace std;
#include <fstream>
void test01() {
ofstream ofstream1;
ofstream1.open("test.txt",ios::out);
ofstream1 << "姓名:张三" << endl;
ofstream1 << "年龄:18" << endl;
ofstream1 << "工作:玩游戏" << endl;
ofstream1.close();
}
int main(){
test01();
}
读文本文件
#include<iostream>
using namespace std;
#include <fstream>
#include "string"
void test() {
ifstream ifstream1;
ifstream1.open("test.txt", ios::in);
if (!ifstream1.is_open()) {
cout << "the file opening failure!";
} else {
char c;
while ((c = ifstream1.get()) != EOF) {
cout << c;
}
}
ifstream1.close();
}
int main() {
test();
}
二进制文件
写二进制文件
#include<iostream>
using namespace std;
#include<fstream>
class Person {
public:
char name[64];
int age;
};
void test01() {
ofstream ofs;
ofs.open("person.txt", ios::out | ios::binary);
Person person = {"Tom", 18};
ofs.write((const char *) &person, sizeof(Person));
ofs.close();
}
int main(){
test01();
}
读二进制文件
#include<iostream>
#include<fstream>
using namespace std;
class Person {
public:
char name[64];
int age;
};
void test() {
ifstream ifs;
ifs.open("person.txt", ios::in | ios::binary);
if (ifs.is_open()) {
Person p;
ifs.read((char *) &p, sizeof(Person));
cout << "name:" << p.name << "\nage:" << p.age << endl;
} else {
cout << "File opening failure!" << endl;
return;
}
ifs.close();
}
int main() {
test();
}
|