代码有写的不好的地方,请大家多多指点
MyQueue.h
#define TRUE 1
#define FALSE 0
#define bool _Bool
#ifndef MYQUEUE_H
#define MYQUEUE_H
#include <stdlib.h>
#define NODE_DATA_TYPE int
typedef struct MyQueueNode {
NODE_DATA_TYPE NodeValue;
struct MyQueueNode* Next;
}MyQueueNode;
typedef struct MyQueue {
unsigned int Length;
MyQueueNode* Head;
MyQueueNode* Back;
}MyQueue;
MyQueue* newMyQueue(void);
MyQueueNode* newMyQueueNode(NODE_DATA_TYPE Value);
void pushToQueue(MyQueue* self, NODE_DATA_TYPE Value);
void popFromQueue(MyQueue* self);
bool isEmpty(MyQueue* self);
unsigned int QueueSize(MyQueue* self);
NODE_DATA_TYPE getFront(MyQueue* self);
NODE_DATA_TYPE getBack(MyQueue* self);
void TraverseQueue(MyQueue* self,void(*fcp)(NODE_DATA_TYPE));
#endif
MyQueue.c
#include "MyQueue.h"
MyQueue* newMyQueue(void) {
MyQueue* self = (MyQueue*)
(malloc(sizeof(MyQueue)));
if (self == NULL) {
return NULL;
}
self->Head = self->Back = NULL;
self->Length = 0;
return self;
}
MyQueueNode* newMyQueueNode(NODE_DATA_TYPE Value) {
MyQueueNode* self = (MyQueueNode*)
(malloc(sizeof(MyQueueNode)));
if (self == NULL) {
return NULL;
}
self->Next = NULL;
self->NodeValue = Value;
return self;
}
void pushToQueue(MyQueue* self, NODE_DATA_TYPE Value) {
if (isEmpty(self)) {
self->Head = newMyQueueNode(Value);
self->Back = self->Head;
self->Length++;
return;
}
MyQueueNode* Temp = self->Head;
while (self->Head->Next != NULL) {
self->Head = self->Head->Next;
}
self->Head->Next = newMyQueueNode(Value);
self->Back = self->Head->Next;
self->Head = Temp;
self->Length++;
}
void popFromQueue(MyQueue* self) {
if (isEmpty(self))return;
MyQueueNode* Temp = self->Head;
self->Head = self->Head->Next;
free(Temp);
if (self->Head == NULL) {
self->Back = NULL;
}
self->Length--;
}
bool isEmpty(MyQueue* self) {
return !self->Length;
}
unsigned int QueueSize(MyQueue* self) {
return self->Length;
}
NODE_DATA_TYPE getFront(MyQueue* self) {
return self->Head->NodeValue;
}
NODE_DATA_TYPE getBack(MyQueue* self) {
return self->Back->NodeValue;
}
void TraverseQueue(MyQueue* self,void(*fcp)(NODE_DATA_TYPE)) {
MyQueueNode* Temp = self->Head;
while (self->Head != NULL) {
fcp(self->Head->NodeValue);
self->Head = self->Head->Next;
}
self->Head = Temp;
}
示例
#include <stdio.h>
#include <time.h>
#include "MyQueue.h"
void putNumber(int Number) {
printf("%d\t", Number);
}
int main(int argc, char* argv[]) {
MyQueue* Queue = newMyQueue();
srand(time(NULL));
if (isEmpty(Queue)) {
printf("当前队列Queue为空\r\n");
}
else {
printf("当前队列Queue不为空\r\n");
}
printf("当前队列长度:%d\r\n", QueueSize(Queue));
printf("-------------------------------\r\n");
printf("随机生成了5个值并且添加到队列Queue\r\n");
for (int i = 0,j=0; i < 5; i++) {
j = rand() % 10 + 1;
printf("%d\t", j);
pushToQueue(Queue, j);
}
printf("\r\n-------------------------------\r\n");
printf("当前队列Queue值\r\n");
TraverseQueue(Queue, putNumber);
printf("\r\n-------------------------------\r\n");
printf( "当前队列Queue的长度是%d\r\n"
"当前队列Queue队头节点值是%d\r\n"
"当前队列Queue队尾节点值是%d\r\n",
QueueSize(Queue),getFront(Queue),getBack(Queue));
printf("-------------------------------\r\n");
printf("弹出队头元素\r\n");
popFromQueue(Queue);
printf("当前队列Queue的长度是%d\r\n"
"当前队列Queue队头节点值是%d\r\n"
"当前队列Queue队尾节点值是%d\r\n",
QueueSize(Queue), getFront(Queue), getBack(Queue));
printf("---------------Queue----------------\r\n\r\n");
MyQueue* Queue2 = newMyQueue();
pushToQueue(Queue2, 100);
printf("当前队列Queue2的长度是%d\r\n"
"当前队列Queue2队头节点值是%d\r\n"
"当前队列Queue2队尾节点值是%d\r\n",
QueueSize(Queue2), getFront(Queue2), getBack(Queue2));
printf("弹出队列Queue2队头\r\n");
popFromQueue(Queue2);
printf("当前队列Queue2长度:%d\r\n", QueueSize(Queue2));
int Value[3];
printf("输入三个值用于加入队列Queue2:");
scanf_s("%d %d %d", &Value[0], &Value[1], &Value[2]);
while (QueueSize(Queue2) != 3) {
pushToQueue(Queue2,Value[QueueSize(Queue2)]);
}
printf("当前队列Queue2的值\r\n");
TraverseQueue(Queue2, putNumber);
printf("\r\n");
printf("正在清空当前队列Queue2\r\n");
while (QueueSize(Queue2)) {
popFromQueue(Queue2);
}
printf("当前队列长度为%d,是否为空:%d\r\n",QueueSize(Queue2),isEmpty(Queue2));
printf("---------------Queue2----------------\r\n\r\n");
return 0;
}
示例输入:1234,7890,6666 示例结果: 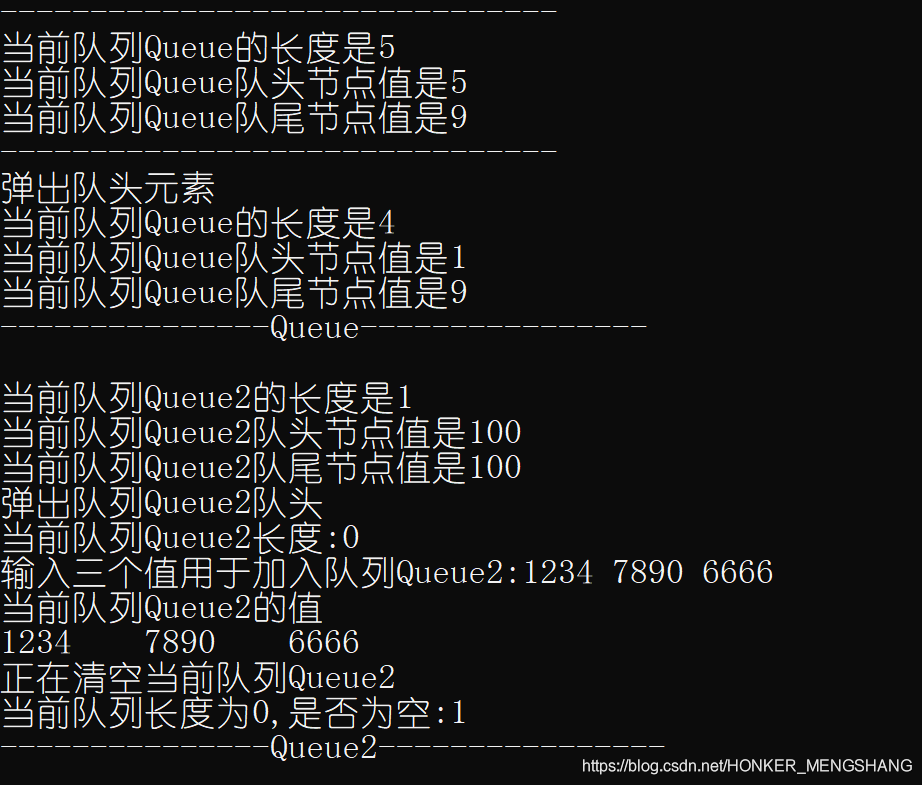
原理部分
队列是一种特殊的线性表,是一种先进先出(FIFO)的数据结构。它只允许在表的前端(front)进行删除操作,而在表的后端(rear)进行插入操作。进行插入操作的端称为队尾,进行删除操作的端称为队头。队列中没有元素时,称为空队列。声明 [转自https://baike.baidu.com/item/queue/4511093)
草图 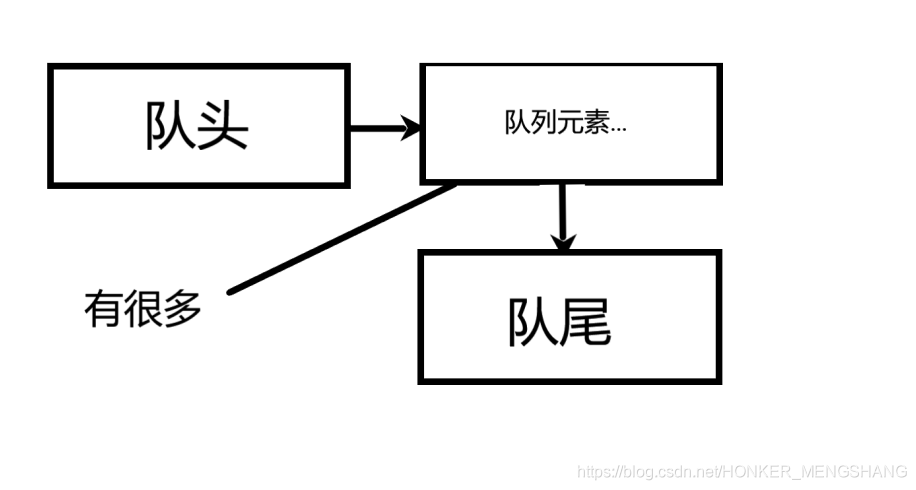 详情原理请看代码注释
|