游戏筑基开发之利用文件函数读出文件数据及处理(反序列化)(C语言)
文件格式如下(txt文件): 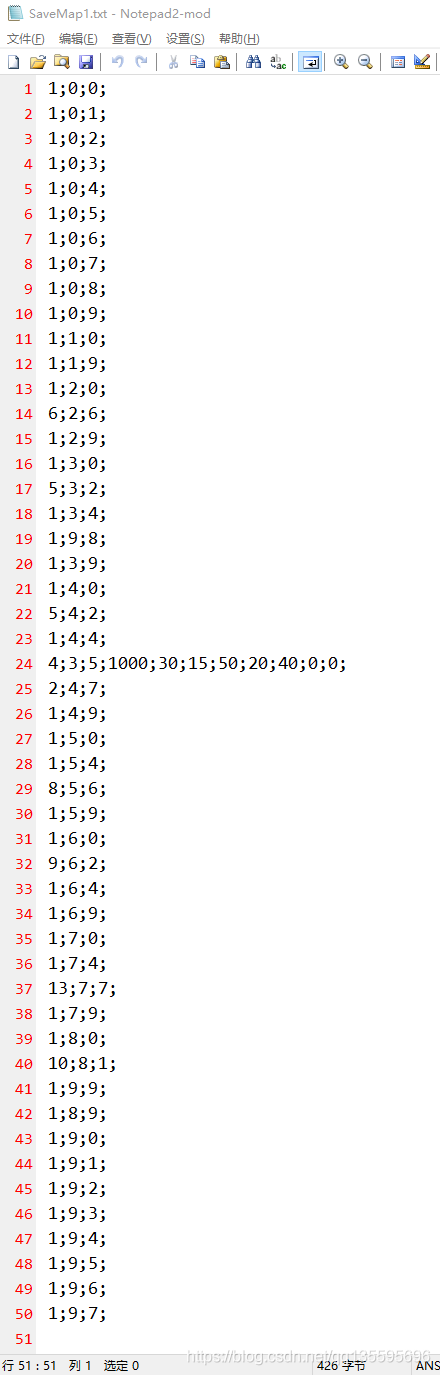
TIPS:最后一行,即50行末尾有一个\n(换行符)
具体实现如下:
可优化,思路仅供参考。
Ini.h
#ifndef INI_H_
#define INI_H_
#define N 1024
typedef struct tagIniNode
{
int value[N];
}TIniNode, *PTIniNode;
typedef struct tagIni
{
PTIniNode pNodes;
int len;
int lenMax;
}TIni, *PTIni;
PTIni InitIni();
void UnInitIni(PTIni *ppIni);
void LoadIni(PTIni pIni, const char* const pPath);
#endif
Ini.c
#include "Ini.h"
#include <stdio.h>
#include <string.h>
PTIni InitIni()
{
PTIni pIni = malloc(sizeof(TIni));
pIni->len = 0;
pIni->lenMax = 100;
pIni->pNodes = malloc(sizeof(TIniNode) * pIni->lenMax);
return pIni;
}
void UnInitIni(PTIni *ppIni)
{
if (*ppIni == NULL)
return NULL;
free((*ppIni)->pNodes);
free(*ppIni);
}
void LoadIni(PTIni pIni, const char* const pPath)
{
FILE *pFile = fopen(pPath, "r");
if (NULL == pFile)
{
printf("打开文件失败!");
return;
}
while (0 == feof(pFile))
{
char buffer[1024] = { 0 };
fgets(buffer, 1024, pFile);
int strLen = strlen(buffer);
if (strLen == 0)
{
fclose(pFile);
return;
}
int temp = 0;
memset(pIni->pNodes[pIni->len].value, -1, sizeof(int) * 1024);
for (int i = 0, j = 0; i < strLen; i++)
{
if (buffer[i] != ';' && buffer[i] != '\n')
temp = temp * 10 + (buffer[i] - 48);
else if (';' == buffer[i])
{
pIni->pNodes[pIni->len].value[j++] = temp;
temp = 0;
}
}
pIni->len++;
}
fclose(pFile);
pFile = NULL;
}
|