题目链接:https://leetcode-cn.com/problems/design-linked-list/ 题目如下: 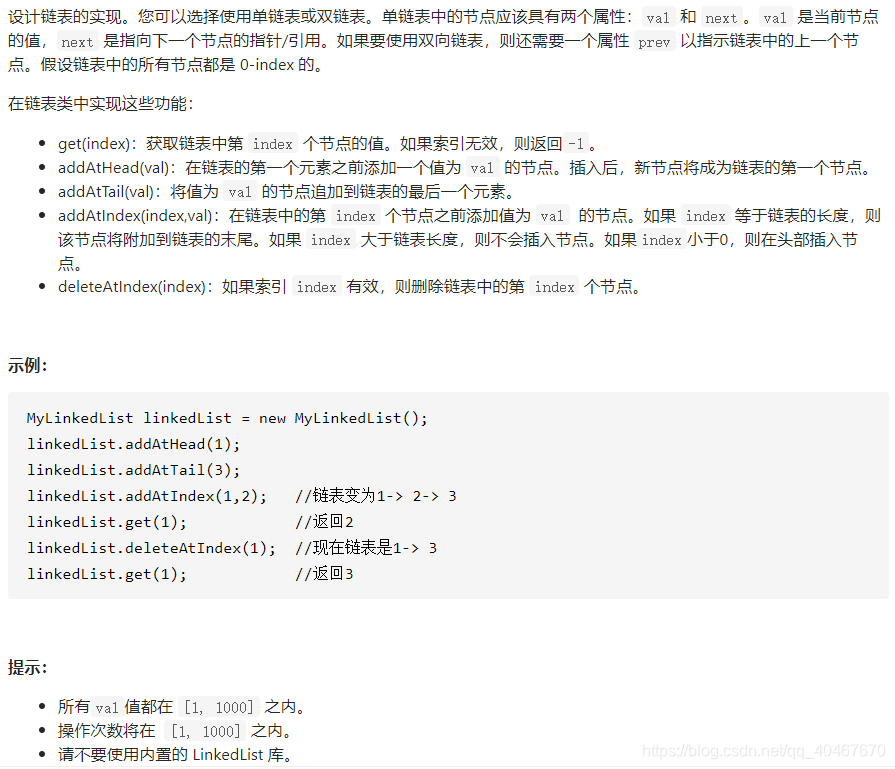
class MyLinkedList {
public:
struct ListNode{
int val;
ListNode* next;
ListNode(int x):val(x),next(NULL){}
};
MyLinkedList() {
m_dummyHead=new ListNode(0);
m_size=0;
}
void print(ListNode* head){
while(head!=NULL){
cout<<head->val<<',';
head=head->next;
}
cout<<endl;
}
int get(int index) {
if(index<0||index>(m_size-1)) return -1;
ListNode* cur=m_dummyHead->next;
while(index--){
cur=cur->next;
}
print(m_dummyHead);
return cur->val;
}
void addAtHead(int val) {
ListNode* node=new ListNode(val);
node->next=m_dummyHead->next;
m_dummyHead->next=node;
m_size++;
}
void addAtTail(int val) {
ListNode* node=new ListNode(val);
ListNode* cur=m_dummyHead;
while(cur->next!=NULL){
cur=cur->next;
}
cur->next=node;
m_size++;
}
void addAtIndex(int index, int val) {
if(index<0||index>m_size) return ;
ListNode* node=new ListNode(val);
ListNode* cur=m_dummyHead;
while(index--){
cur=cur->next;
}
node->next=cur->next;
cur->next=node;
m_size++;
}
void deleteAtIndex(int index) {
if(index<0||index>=m_size) return ;
ListNode* cur=m_dummyHead;
while(index--){
cur=cur->next;
}
ListNode* temp=cur->next;
cur->next=cur->next->next;
delete temp;
m_size--;
}
private:
int m_size;
ListNode* m_dummyHead;
};
|