多态练习 - 通讯录管理系统
项目使用多态来完成框架,另外含有文件操作,可永久保存数据
main函数中使用WorkerManager类与用户交互:
框架如下:
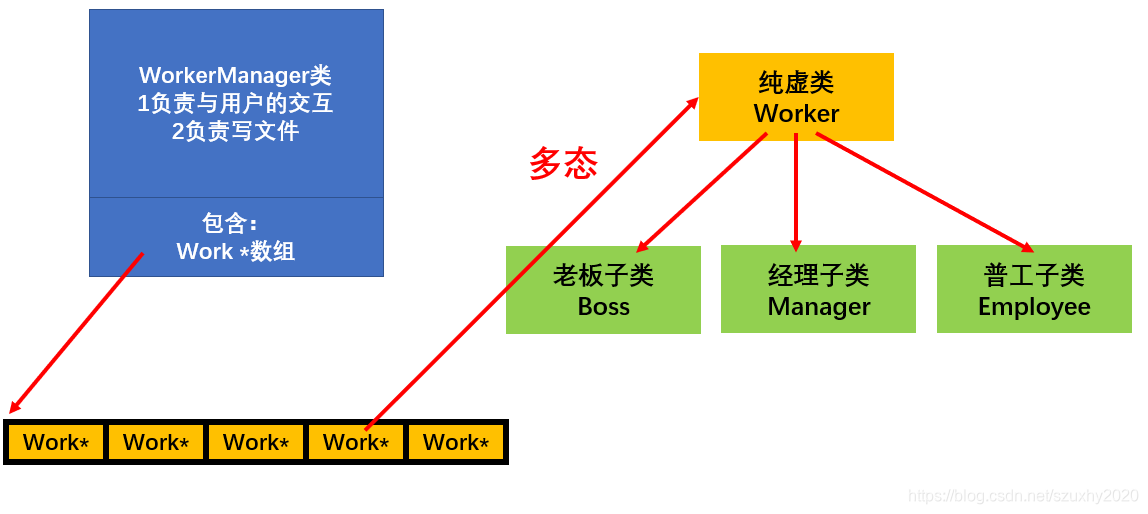
?效果图如下:
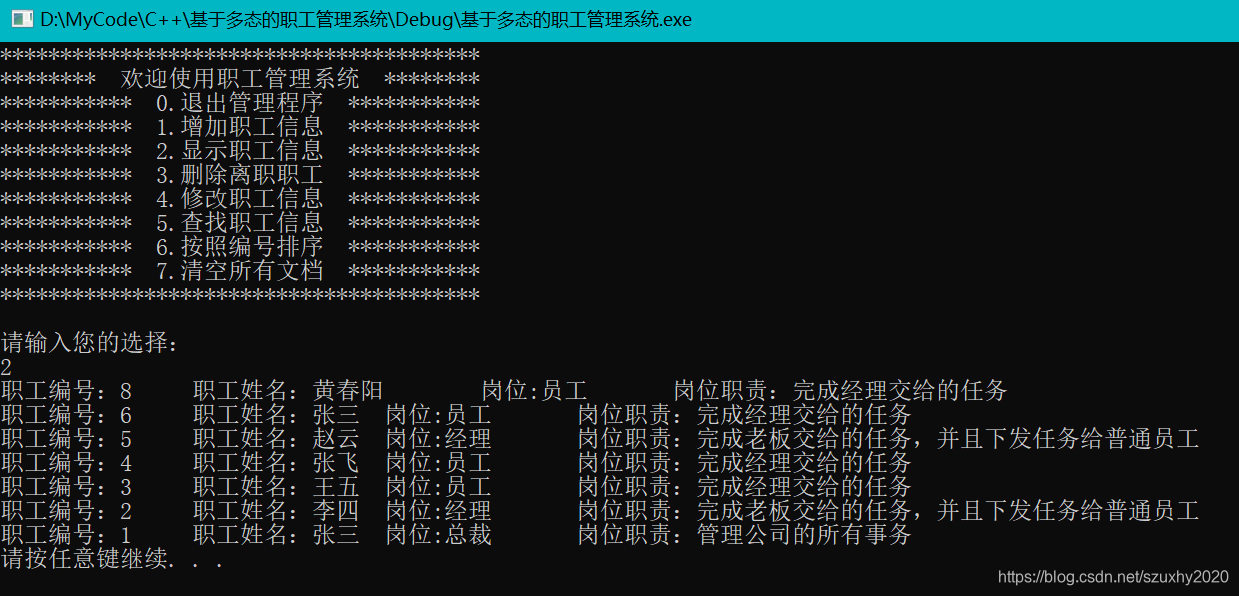
?案例需求:
管理公司内所有员工信息,来实现一个基于多态的管理系统
职工分为三类:普通员工,经理,老板,显示信息时,需要显示编号、姓名、岗位、职责
普通员工职责:完成经理交给的任务
经理职责:完成老板交给的任务,并下达任务给员工
老板职责:管理公司所有的事物
系统需要实现的功能:
- 退出管理程序
- 增加员工信息:实现批量添加的功能 ??编号-姓名-部门编号
- 显示员工信息:显示所有
- 删除离职职工:按编号删除
- 修改职工信息:按编号修改
- 查找员工信息:按编号或姓名查询
- 按照编号排序:排序规则由用户确定
- 清空所有文档:清空所有信息,提供再确认防止误删
代码如下:
main函数:
#include<iostream>
using namespace std;
#include"WorkerManager.h"
int main() {
//实例化对象
WorkerManager wm;
int choice = 0;
while (true) {
wm.Show_Menu();
cout << "请输入您的选择:" << endl;
cin >> choice;//接收用户的选项
switch (choice){
case 0://退出
wm.ExitSystem();
break;
case 1://增加
wm.Add_Emp();
break;
case 2://显示
wm.show_Emp();
break;
case 3://删除
wm.del_Emp();
break;
case 4://修改
wm.mod_Emp();
break;
case 5://查找
wm.find_Emp();
break;
case 6://排序
wm.sort_Emp();
break;
case 7://清空文档
wm.clean_File();
break;
default:
system("cls");
break;
}
}
wm.Show_Menu();
return 0;
}
WorkerManager.h
#pragma once//防止头文件重复包含
#include<iostream>
#include"Worker.h"
#include"Employee.h"
#include"Boss.h"
#include"Manager.h"
using namespace std;//使用标准的命名空间
#include<fstream>
#define FILENAME "empFile.txt"
class WorkerManager {
public:
WorkerManager();
//析构
~WorkerManager();
//展示菜单函数
void Show_Menu();
//退出系统
void ExitSystem();
//添加职工函数
void Add_Emp();
//保存文件
void save();
//添加标志判断文件是否为空
bool m_FileIsEmpty;
//统计文件中的人数
int get_EmpNum();
//初始化员工数组
void init_Emp();
//显示员工
void show_Emp();
//删除员工
void del_Emp();
//判断员工是否存在,存在返回数组中的位置,不存在返回-1
int isExist(int id);
//修改职工
void mod_Emp();
//查找职工
void find_Emp();
//按编号排序
void sort_Emp();
//清空数据
void clean_File();
//记录职工人数
int m_EmpNum;
//职工数组指针
Worker **m_EmpArray;
};
WorkerManager.cpp
#include"WorkerManager.h"
WorkerManager::WorkerManager() {
//1、文件不存在
ifstream ifs;
ifs.open(FILENAME, ios::in);
if (!ifs.is_open()) {
//cout << "文件不存在" << endl;
//初始化属性
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
//2、文件存在,数据为空
char ch;
ifs >> ch;
if (ifs.eof()) {
//文件为空
//cout << "文件为空" << endl;
//初始化属性
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
//3、当文件存在且不为空的情况
int num = this->get_EmpNum();
//cout << "职工人数为:" << num << endl;
this->m_EmpNum = num;
//开辟空间
this->m_EmpArray = new Worker*[this->m_EmpNum];
//将文件中的员工数据读入程序的数组中
this->init_Emp();
//测试代码
/*for (int i = 0; i < this->m_EmpNum; i++) {
cout << this->m_EmpArray[i]->m_Id << " "
<< this->m_EmpArray[i]->m_Name << " "
<< this->m_EmpArray[i]->m_Did << " " << endl;
}*/
}
WorkerManager::~WorkerManager() {
if (this->m_EmpArray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_EmpArray[i];
m_EmpArray[i] = NULL;
}
delete []this->m_EmpArray;
this->m_EmpArray = NULL;
}
}
void WorkerManager:: Show_Menu() {
cout << "****************************************" << endl;
cout << "******** 欢迎使用职工管理系统 ********" << endl;
cout << "*********** 0.退出管理程序 ***********" << endl;
cout << "*********** 1.增加职工信息 ***********" << endl;
cout << "*********** 2.显示职工信息 ***********" << endl;
cout << "*********** 3.删除离职职工 ***********" << endl;
cout << "*********** 4.修改职工信息 ***********" << endl;
cout << "*********** 5.查找职工信息 ***********" << endl;
cout << "*********** 6.按照编号排序 ***********" << endl;
cout << "*********** 7.清空所有文档 ***********" << endl;
cout << "****************************************" << endl;
cout << endl;
}
void WorkerManager::ExitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);//退出程序
}
void WorkerManager::Add_Emp() {
cout << "请输入添加员工的数量:" << endl;
int addNum = 0;//保存用户输入的数量
cin >> addNum;
if (addNum > 0) {
//添加
//计算添加空间的大小
int newSize = this->m_EmpNum + addNum;//新空间人数=原人数+新增加
//开辟新空间
Worker **newSpace = new Worker*[newSize];
//将原来的数据拷贝到新空间中
if (this->m_EmpArray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
newSpace[i] = this->m_EmpArray[i];
}
}
//添加新数据
for (int i = 0; i < addNum; i++) {
int id;
string name;
int dSelect;
cout << "请输入第" << i + 1 << "个新员工编号:" << endl;
//加入标志判断员工编号是否重复,重复则true,一直循环
bool flag = true;
while (flag) {
cin >> id;
flag = false;//默认结束循环,如果下面for循环判断没有重复,那么将会结束
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Id == id) {
cout << "该编号已存在,请重新输入" << endl;
flag = true;
break;
}
}
}
cout << "请输入第" << i + 1 << "个新员工姓名:" << endl;
cin >> name;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cin >> dSelect;
//根据用户输入创建不同的员工对象
Worker *worker = NULL;
switch (dSelect) {
case 1:
worker = new Employee(id, name, 1);
break;
case 2:
worker = new Manager(id, name, 2);
break;
case 3:
worker = new Boss(id, name, 3);
break;
default:
break;
}
//将员工装入新数组
newSpace[this->m_EmpNum + i] = worker;
}
//释放原有空间
delete[]this->m_EmpArray;
//更新员工数组指针
this->m_EmpArray = newSpace;
//更新人数
this->m_EmpNum = newSize;
//提示添加成功
cout << "成功添加" << addNum << "名新员工!" << endl;
//成功添加后应保存到文件中
this->save();
//更新职工不为空的标志
this->m_FileIsEmpty = false;
}
else {
cout << "输入数据有误" << endl;
}
//按任意键后清屏回到上级目录
system("pause");
system("cls");
}
//保存文件
void WorkerManager::save() {
ofstream ofs;
ofs.open(FILENAME, ios::out);//用输出的方式打开文件(写文件)
//开始写文件
for (int i = 0; i < this->m_EmpNum; i++) {
ofs << this->m_EmpArray[i]->m_Id << " "
<< this->m_EmpArray[i]->m_Name << " "
<< this->m_EmpArray[i]->m_Did << " " << endl;
}
//关闭文件
ofs.close();
}
//统计文件中的人数
int WorkerManager::get_EmpNum() {
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id, did;
string name;
int num = 0;//统计人数
while (ifs >> id && ifs >> name && ifs >> did) {
num++;
}
return num;
}
//初始化员工数组
void WorkerManager::init_Emp() {
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id, did;
string name;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> did) {
Worker *worker = NULL;
if (did == 1) {
worker = new Employee(id, name, 1);
}
else if (did == 2) {
worker = new Manager(id, name, 2);
}
else {
worker = new Boss(id, name, 3);
}
this->m_EmpArray[index++] = worker;
}
ifs.close();
}
//显示员工
void WorkerManager::show_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
}
else {
for (int i = 0; i < this->m_EmpNum; i++) {
//利用多态
this->m_EmpArray[i]->showInfo();
}
}
system("pause");
system("cls");
}
//删除员工
void WorkerManager::del_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
}
else {
//按照编号删除
cout << "请输入要删除的员工编号:" << endl;
int id=0;
cin >> id;
int index = this->isExist(id);
if (index != -1) {//说明职工存在
for (int i = index; i < this->m_EmpNum - 1; i++) {
this->m_EmpArray[i] = this->m_EmpArray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功!" << endl;
//清屏
system("pause");
system("cls");
}
else {
cout << "删除失败,未找到该员工" << endl;
system("pause");
system("cls");
}
}
}
//判断员工是否存在,存在返回数组中的位置,不存在返回-1
int WorkerManager::isExist(int id) {
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Id == id) {
return i;
}
}
return -1;
}
//修改信息
void WorkerManager::mod_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或者为空!" << endl;
}
else {
cout << "请输入要修改的员工的编号:" << endl;
int id = 0;
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {//找到员工
delete this->m_EmpArray[ret];
int newId = 0;
string newName = "";
int dSelect = 0;
cout << "查找到第" << ret << "号员工,请输入新职工号:" << endl;
cin >> newId;
cout << "请输入新的姓名:" << endl;
cin >> newName;
cout << "请输入新岗位:" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cin >> dSelect;
Worker *worker = NULL;
switch (dSelect) {
case 1:
worker = new Employee(newId, newName, 1);
break;
case 2:
worker = new Manager(newId, newName, 2);
break;
case 3:
worker = new Boss(newId, newName, 3);
break;
default: {
cout << "输入有误,退出修改功能!" << endl;
system("pause"); system("cls"); return;
break;
}
}
//更新数据到数组中,并保存到文件中
this->m_EmpArray[ret] = worker;
this->save();
cout << "修改成功!" << endl;
}
else {
cout << "修改失败,查无此人!" << endl;
}
}
system("pause");
system("cls");
}
//查找职工
void WorkerManager::find_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或者为空!" << endl;
}
else {
cout << "请输入查找的方式:" << endl;
cout << "1.按职工编号查找" << endl;
cout << "2.按职工姓名查找" << endl;
int select = 0;//获取用户查找方式
cin >> select;
if (select == 1) {
//按编号查找
cout << "请输入您想要查找的编号:" << endl;
int id;
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {
//找到职工
cout << "查找成功!该员工信息如下:" << endl;
this->m_EmpArray[ret]->showInfo();
}
else {
//查找失败
cout << "查找失败,查无此人!" << endl;
}
}
else if (select==2) {
//按姓名查找
string name;
cout << "请输入您要查找的姓名:" << endl;
cin >> name;
bool flag = false;//加入判断是否查找到的标志
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Name == name) {
cout << "查找成功,职工编号为:" << this->m_EmpArray[i]->m_Id
<< "号职工信息如下:" << endl;
flag = true;
//调用显示信息的接口
this->m_EmpArray[i]->showInfo();
}
}
if (flag == false) {
cout << "查找失败,查无此人!" << endl;
}
}
else {
cout << "输入选项有误!" << endl;
}
}
//按任意键清屏
system("pause");
system("cls");
}
//按编号排序
void WorkerManager::sort_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
system("pause");
system("cls");
}
else {
cout << "请选择排序方式:" << endl;
cout << "1.按员工编号升序" << endl;
cout << "2.按员工编号降序" << endl;
int select;
cin >> select;
//进行简单选择排序
if (select == 1) {
//升序
int Min ;//记录最小值下标
for (int i = 0; i < this->m_EmpNum - 1; i++) {
Min = i;
for (int j = i + 1; j < this->m_EmpNum; j++) {
if (this->m_EmpArray[Min]->m_Id > this->m_EmpArray[j]->m_Id) {
Min = j;
}
}
//进行交换
Worker * temp_worker = this->m_EmpArray[Min];
this->m_EmpArray[Min] = this->m_EmpArray[i];
this->m_EmpArray[i] = temp_worker;
}
}
else if(select == 2) {
//降序
int Max;//记录最大值下标
for (int i = 0; i < this->m_EmpNum - 1; i++) {
Max = i;
for (int j = i + 1; j < this->m_EmpNum; j++) {
if (this->m_EmpArray[Max]->m_Id < this->m_EmpArray[j]->m_Id) {
Max = j;
}
}
//进行交换
Worker * temp_worker = this->m_EmpArray[Max];
this->m_EmpArray[Max] = this->m_EmpArray[i];
this->m_EmpArray[i] = temp_worker;
}
}
else {
cout << "排序方式输入有误!" << endl;
system("pause");
system("cls");
return;
}
}
cout << "排序成功!排序后的结果为:" << endl;
this->save();
this->show_Emp();
}
//清空数据
void WorkerManager ::clean_File() {
cout << "确认清空吗?" << endl;
cout << "1.确认" << endl;
cout << "2.取消" << endl;
int select = 0;
cin >> select;
if (select == 1) {
//清空
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
//释放内存
if (this->m_EmpArray != NULL) {
//删除员工对象
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_EmpArray[i];
this->m_EmpArray[i] = NULL;
}
//删除堆区指针
delete[]this->m_EmpArray;
this->m_EmpArray = NULL;
this->m_EmpNum = 0;
this->m_FileIsEmpty = true;
}
cout << "清空成功!" << endl;
system("pause");
system("cls");
}
}
Worker.h
#pragma once
#include<iostream>
using namespace std;
#include<string>
class Worker {
public:
//显示个人信息
virtual void showInfo() = 0;
//获取岗位名称
virtual string getDeptName() = 0;
//析构
int m_Id;
string m_Name;
int m_Did;//部门id
};
Boss.h
#pragma once
#include<iostream>
using namespace std;
#include"Worker.h"
class Boss :public Worker {
public:
//构造函数
Boss(int id, string name, int did);
//显示个人信息
virtual void showInfo();
//获取岗位名称
virtual string getDeptName();
};
Boss.cpp
#include"Boss.h"
//构造函数
Boss::Boss(int id, string name, int did) {
this->m_Id = id;
this->m_Name = name;
this->m_Did = did;
}
//显示个人信息
void Boss::showInfo() {
cout << "职工编号:" << this->m_Id << "\t职工姓名:" << this->m_Name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:管理公司的所有事务" << endl;
}
//获取岗位名称
string Boss::getDeptName() {
return string("总裁");
}
Manager.h
#pragma once
#include<iostream>
using namespace std;
#include"Worker.h"
class Manager:public Worker {
public:
//构造函数
Manager(int id, string name, int did);
//显示个人信息
virtual void showInfo() ;
//获取岗位名称
virtual string getDeptName() ;
};
Manager.cpp
#include"WorkerManager.h"
WorkerManager::WorkerManager() {
//1、文件不存在
ifstream ifs;
ifs.open(FILENAME, ios::in);
if (!ifs.is_open()) {
//cout << "文件不存在" << endl;
//初始化属性
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
//2、文件存在,数据为空
char ch;
ifs >> ch;
if (ifs.eof()) {
//文件为空
//cout << "文件为空" << endl;
//初始化属性
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = true;
ifs.close();
return;
}
//3、当文件存在且不为空的情况
int num = this->get_EmpNum();
//cout << "职工人数为:" << num << endl;
this->m_EmpNum = num;
//开辟空间
this->m_EmpArray = new Worker*[this->m_EmpNum];
//将文件中的员工数据读入程序的数组中
this->init_Emp();
//测试代码
/*for (int i = 0; i < this->m_EmpNum; i++) {
cout << this->m_EmpArray[i]->m_Id << " "
<< this->m_EmpArray[i]->m_Name << " "
<< this->m_EmpArray[i]->m_Did << " " << endl;
}*/
}
WorkerManager::~WorkerManager() {
if (this->m_EmpArray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_EmpArray[i];
m_EmpArray[i] = NULL;
}
delete []this->m_EmpArray;
this->m_EmpArray = NULL;
}
}
void WorkerManager:: Show_Menu() {
cout << "****************************************" << endl;
cout << "******** 欢迎使用职工管理系统 ********" << endl;
cout << "*********** 0.退出管理程序 ***********" << endl;
cout << "*********** 1.增加职工信息 ***********" << endl;
cout << "*********** 2.显示职工信息 ***********" << endl;
cout << "*********** 3.删除离职职工 ***********" << endl;
cout << "*********** 4.修改职工信息 ***********" << endl;
cout << "*********** 5.查找职工信息 ***********" << endl;
cout << "*********** 6.按照编号排序 ***********" << endl;
cout << "*********** 7.清空所有文档 ***********" << endl;
cout << "****************************************" << endl;
cout << endl;
}
void WorkerManager::ExitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);//退出程序
}
void WorkerManager::Add_Emp() {
cout << "请输入添加员工的数量:" << endl;
int addNum = 0;//保存用户输入的数量
cin >> addNum;
if (addNum > 0) {
//添加
//计算添加空间的大小
int newSize = this->m_EmpNum + addNum;//新空间人数=原人数+新增加
//开辟新空间
Worker **newSpace = new Worker*[newSize];
//将原来的数据拷贝到新空间中
if (this->m_EmpArray != NULL) {
for (int i = 0; i < this->m_EmpNum; i++) {
newSpace[i] = this->m_EmpArray[i];
}
}
//添加新数据
for (int i = 0; i < addNum; i++) {
int id;
string name;
int dSelect;
cout << "请输入第" << i + 1 << "个新员工编号:" << endl;
//加入标志判断员工编号是否重复,重复则true,一直循环
bool flag = true;
while (flag) {
cin >> id;
flag = false;//默认结束循环,如果下面for循环判断没有重复,那么将会结束
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Id == id) {
cout << "该编号已存在,请重新输入" << endl;
flag = true;
break;
}
}
}
cout << "请输入第" << i + 1 << "个新员工姓名:" << endl;
cin >> name;
cout << "请选择该职工的岗位:" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cin >> dSelect;
//根据用户输入创建不同的员工对象
Worker *worker = NULL;
switch (dSelect) {
case 1:
worker = new Employee(id, name, 1);
break;
case 2:
worker = new Manager(id, name, 2);
break;
case 3:
worker = new Boss(id, name, 3);
break;
default:
break;
}
//将员工装入新数组
newSpace[this->m_EmpNum + i] = worker;
}
//释放原有空间
delete[]this->m_EmpArray;
//更新员工数组指针
this->m_EmpArray = newSpace;
//更新人数
this->m_EmpNum = newSize;
//提示添加成功
cout << "成功添加" << addNum << "名新员工!" << endl;
//成功添加后应保存到文件中
this->save();
//更新职工不为空的标志
this->m_FileIsEmpty = false;
}
else {
cout << "输入数据有误" << endl;
}
//按任意键后清屏回到上级目录
system("pause");
system("cls");
}
//保存文件
void WorkerManager::save() {
ofstream ofs;
ofs.open(FILENAME, ios::out);//用输出的方式打开文件(写文件)
//开始写文件
for (int i = 0; i < this->m_EmpNum; i++) {
ofs << this->m_EmpArray[i]->m_Id << " "
<< this->m_EmpArray[i]->m_Name << " "
<< this->m_EmpArray[i]->m_Did << " " << endl;
}
//关闭文件
ofs.close();
}
//统计文件中的人数
int WorkerManager::get_EmpNum() {
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id, did;
string name;
int num = 0;//统计人数
while (ifs >> id && ifs >> name && ifs >> did) {
num++;
}
return num;
}
//初始化员工数组
void WorkerManager::init_Emp() {
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id, did;
string name;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> did) {
Worker *worker = NULL;
if (did == 1) {
worker = new Employee(id, name, 1);
}
else if (did == 2) {
worker = new Manager(id, name, 2);
}
else {
worker = new Boss(id, name, 3);
}
this->m_EmpArray[index++] = worker;
}
ifs.close();
}
//显示员工
void WorkerManager::show_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
}
else {
for (int i = 0; i < this->m_EmpNum; i++) {
//利用多态
this->m_EmpArray[i]->showInfo();
}
}
system("pause");
system("cls");
}
//删除员工
void WorkerManager::del_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
}
else {
//按照编号删除
cout << "请输入要删除的员工编号:" << endl;
int id=0;
cin >> id;
int index = this->isExist(id);
if (index != -1) {//说明职工存在
for (int i = index; i < this->m_EmpNum - 1; i++) {
this->m_EmpArray[i] = this->m_EmpArray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功!" << endl;
//清屏
system("pause");
system("cls");
}
else {
cout << "删除失败,未找到该员工" << endl;
system("pause");
system("cls");
}
}
}
//判断员工是否存在,存在返回数组中的位置,不存在返回-1
int WorkerManager::isExist(int id) {
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Id == id) {
return i;
}
}
return -1;
}
//修改信息
void WorkerManager::mod_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或者为空!" << endl;
}
else {
cout << "请输入要修改的员工的编号:" << endl;
int id = 0;
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {//找到员工
delete this->m_EmpArray[ret];
int newId = 0;
string newName = "";
int dSelect = 0;
cout << "查找到第" << ret << "号员工,请输入新职工号:" << endl;
cin >> newId;
cout << "请输入新的姓名:" << endl;
cin >> newName;
cout << "请输入新岗位:" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cin >> dSelect;
Worker *worker = NULL;
switch (dSelect) {
case 1:
worker = new Employee(newId, newName, 1);
break;
case 2:
worker = new Manager(newId, newName, 2);
break;
case 3:
worker = new Boss(newId, newName, 3);
break;
default: {
cout << "输入有误,退出修改功能!" << endl;
system("pause"); system("cls"); return;
break;
}
}
//更新数据到数组中,并保存到文件中
this->m_EmpArray[ret] = worker;
this->save();
cout << "修改成功!" << endl;
}
else {
cout << "修改失败,查无此人!" << endl;
}
}
system("pause");
system("cls");
}
//查找职工
void WorkerManager::find_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或者为空!" << endl;
}
else {
cout << "请输入查找的方式:" << endl;
cout << "1.按职工编号查找" << endl;
cout << "2.按职工姓名查找" << endl;
int select = 0;//获取用户查找方式
cin >> select;
if (select == 1) {
//按编号查找
cout << "请输入您想要查找的编号:" << endl;
int id;
cin >> id;
int ret = this->isExist(id);
if (ret != -1) {
//找到职工
cout << "查找成功!该员工信息如下:" << endl;
this->m_EmpArray[ret]->showInfo();
}
else {
//查找失败
cout << "查找失败,查无此人!" << endl;
}
}
else if (select==2) {
//按姓名查找
string name;
cout << "请输入您要查找的姓名:" << endl;
cin >> name;
bool flag = false;//加入判断是否查找到的标志
for (int i = 0; i < this->m_EmpNum; i++) {
if (this->m_EmpArray[i]->m_Name == name) {
cout << "查找成功,职工编号为:" << this->m_EmpArray[i]->m_Id
<< "号职工信息如下:" << endl;
flag = true;
//调用显示信息的接口
this->m_EmpArray[i]->showInfo();
}
}
if (flag == false) {
cout << "查找失败,查无此人!" << endl;
}
}
else {
cout << "输入选项有误!" << endl;
}
}
//按任意键清屏
system("pause");
system("cls");
}
//按编号排序
void WorkerManager::sort_Emp() {
if (this->m_FileIsEmpty) {
cout << "文件不存在或记录为空!" << endl;
system("pause");
system("cls");
}
else {
cout << "请选择排序方式:" << endl;
cout << "1.按员工编号升序" << endl;
cout << "2.按员工编号降序" << endl;
int select;
cin >> select;
//进行简单选择排序
if (select == 1) {
//升序
int Min ;//记录最小值下标
for (int i = 0; i < this->m_EmpNum - 1; i++) {
Min = i;
for (int j = i + 1; j < this->m_EmpNum; j++) {
if (this->m_EmpArray[Min]->m_Id > this->m_EmpArray[j]->m_Id) {
Min = j;
}
}
//进行交换
Worker * temp_worker = this->m_EmpArray[Min];
this->m_EmpArray[Min] = this->m_EmpArray[i];
this->m_EmpArray[i] = temp_worker;
}
}
else if(select == 2) {
//降序
int Max;//记录最大值下标
for (int i = 0; i < this->m_EmpNum - 1; i++) {
Max = i;
for (int j = i + 1; j < this->m_EmpNum; j++) {
if (this->m_EmpArray[Max]->m_Id < this->m_EmpArray[j]->m_Id) {
Max = j;
}
}
//进行交换
Worker * temp_worker = this->m_EmpArray[Max];
this->m_EmpArray[Max] = this->m_EmpArray[i];
this->m_EmpArray[i] = temp_worker;
}
}
else {
cout << "排序方式输入有误!" << endl;
system("pause");
system("cls");
return;
}
}
cout << "排序成功!排序后的结果为:" << endl;
this->save();
this->show_Emp();
}
//清空数据
void WorkerManager ::clean_File() {
cout << "确认清空吗?" << endl;
cout << "1.确认" << endl;
cout << "2.取消" << endl;
int select = 0;
cin >> select;
if (select == 1) {
//清空
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
//释放内存
if (this->m_EmpArray != NULL) {
//删除员工对象
for (int i = 0; i < this->m_EmpNum; i++) {
delete this->m_EmpArray[i];
this->m_EmpArray[i] = NULL;
}
//删除堆区指针
delete[]this->m_EmpArray;
this->m_EmpArray = NULL;
this->m_EmpNum = 0;
this->m_FileIsEmpty = true;
}
cout << "清空成功!" << endl;
system("pause");
system("cls");
}
}
Employee.h
#pragma once
#include<iostream>
using namespace std;
#include"Worker.h"
class Employee:public Worker {
public:
//构造函数
Employee(int id, string name, int did);
//显示个人信息
virtual void showInfo();
//获取岗位名称
virtual string getDeptName();
};
Employee.cpp
#include"Employee.h"
//构造函数
Employee::Employee(int id,string name,int did) {
this->m_Id = id;
this->m_Name = name;
this->m_Did = did;
}
//显示个人信息
void Employee::showInfo() {
cout << "职工编号:" << this->m_Id << "\t职工姓名:" << this->m_Name
<< "\t岗位:" << this->getDeptName()
<< "\t岗位职责:完成经理交给的任务" << endl;
}
//获取岗位名称
string Employee::getDeptName() {
return string("员工");
}
以上就是全部代码啦
如果想跟着练可以去b站搜黑马的视频,我是跟着黑马练的,加油,晚安。
|