一. int* pt; pt = &higgens;
#include <iostream>
#include <cstdio>
#include<string>
#include<Cmath>
#include<CString>
using namespace std;
int main()
{
int higgens = 5;
int* pt;
pt = &higgens;
cout << " &higgens " << &higgens << endl;
cout << " pt " << pt << endl;
cout << " * pt " << *pt << endl;
return 0;
}
二.指针和数组的本质区别 1.p3 = p3 + 1;
int main()
{
double* p3 = new double[3];
p3[0] = 0.2;
p3[1] = 0.5;
p3[2] = 0.8;
cout << " p3[0] : " << p3[0] << endl;
p3 = p3 + 1;
cout << " now p3[0] is : " << p3[0] << endl;
cout << " p3[1] : " << p3[1] << endl;
delete[] p3;
return 0;
}
2.*(stacks + 1)、 stacks[1]
int main()
{
double wages[3] = { 10000.0, 20000.0, 30000.0 };
double stacks[3] = { 3,2,1 };
double* pw = wages;
double* ps = &stacks[0];
cout << " pw : " << pw << " *pw : " << *pw << endl;
cout << " ps : " << ps << " *ps : " << *ps << endl;
pw = pw + 1;
ps = ps + 1;
cout << " pw : " << pw << " *pw : " << *pw << endl;
cout << " ps : " << ps << " *ps : " << *ps << endl;
pw = pw - 1;
ps = ps - 1;
cout << " pw : " << pw << " *pw : " << *pw << endl;
cout << " ps : " << ps << " *ps : " << *ps << endl;
cout << " stacks[0] : " << stacks[0] << " stacks[1] : " << stacks[1] << endl;
cout << " *stacks : " << *stacks << " *(stacks + 1) : " << *(stacks + 1) << endl;
return 0;
}
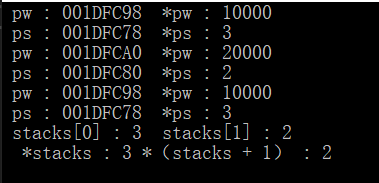 3.指针指向字符串,操作和数组基本一致
int main()
{
char animal[20] = "bear";
const char* bird = "wren";
char* ps;
cout << animal << " and " << bird << endl;
cout << *(bird +1) << endl;
return 0;
}
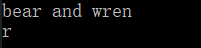 4.创建动态结构体
struct inflatable
{
char name[20];
float volumn;
double price;
};
int main()
{
inflatable* ps = new inflatable;
cout << "enter the name of the inflatable:";
cin.get(ps->name, 20);
cout << "enter the volumn of the inflatable:";
cin >>(*ps).volumn;
cout << "enter the price of the inflatable:";
cin >> ps->price;
cout << "name:" << (*ps).name << endl;
cout << "volumn:" << (*ps).volumn << endl;
cout << "price:" << (*ps).price << endl;
delete ps;
return 0;
}
5 函数返回值为数组地址
char* getname();
int main()
{
char *name;
name = getname();
cout << name << endl;
delete[] name;
return 0;
}
char *getname()
{
char temp[80];
cout << "enter the last name:";
cin >> temp;
char* pn = new char[strlen(temp) + 1];
strcpy_s(pn, strlen(temp) + 1,temp);
return pn;
}
6.array vector 数组
int main()
{
vector<double> a2(4);
a2[0] = 1.0 / 3.0;
a2[1] = 1.0 / 5.0;
a2[2] = 1.0 / 7.0;
a2[3] = 1.0 / 9.0;
array<double, 4> a3 = { 3.14,2.72,1.62,1.41 };
array<double, 4> a4;
a4 = a3;
cout << "a2[2]" << a2[2] << endl;
cout << "a3[2]" << a3[2] << endl;
cout << "a4[2]" << a4[2] << endl;
return 0;
}
|