std::find系列的函数: std::find, std::find_if, std::find_if_not
Dash种的解释: Returns an iterator to the first element in the range [first, last) that satisfies specific criteria: 翻译:将迭代器返回到满足特定条件的 [first, last) 范围内的 first 元素 说人话就是返回你在一定范围内寻找的值,并且是第一次出现的。
find_if searches for an element for which predicate p returns true find_if_not searches for an element for which predicate q returns false
上面两个如果找到返回ture和false
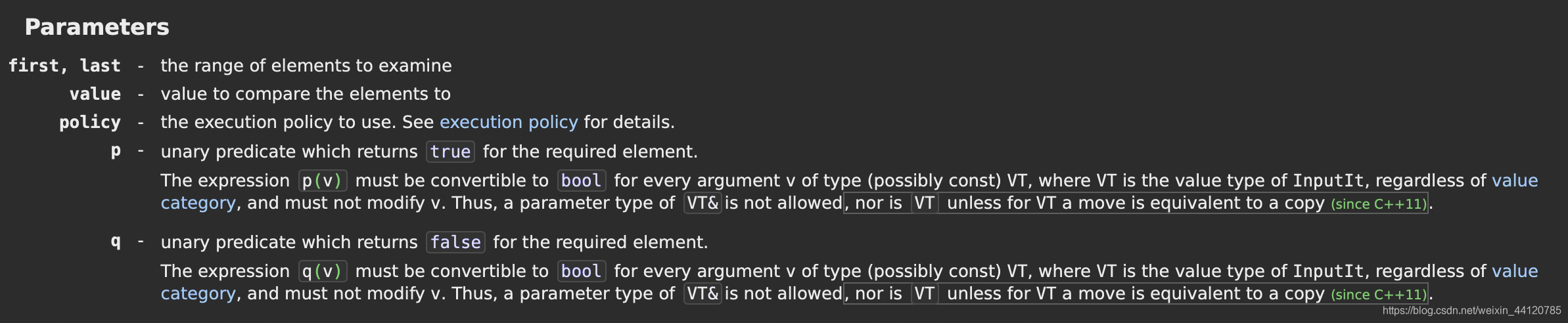
与之等价的函数写法
版本1:
template<class InputIt, class T>
constexpr InputIt find(InputIt first, InputIt last, const T& value)
{
for (; first != last; ++first) {
if (*first == value) {
return first;
}
}
return last;
}
可以看到,传入的是数据两端的地址,进行for循环寻找传入的第三个参数。如果有,返回参数地址。如果没有,那么返回数据最后一个地址。
版本2:
template<class InputIt, class UnaryPredicate>
constexpr InputIt find_if(InputIt first, InputIt last, UnaryPredicate p)
{
for (; first != last; ++first) {
if (p(*first)) {
return first;
}
}
return last;
}```
版本3:
```cpp
template<class InputIt, class UnaryPredicate>
constexpr InputIt find_if_not(InputIt first, InputIt last, UnaryPredicate q)
{
for (; first != last; ++first) {
if (!q(*first)) {
return first;
}
}
return last;
}
应用实例
#include <iostream>
#include <algorithm>
#include <vector>
#include <iterator>
int main()
{
std::vector<int> v{1, 2, 3, 4};
int n1 = 3;
int n2 = 5;
auto is_even = [](int i){ return i%2 == 0; };
auto result1 = std::find(begin(v), end(v), n1);
auto result2 = std::find(begin(v), end(v), n2);
auto result3 = std::find_if(begin(v), end(v), is_even);
(result1 != std::end(v))
? std::cout << "v contains " << n1 << '\n'
: std::cout << "v does not contain " << n1 << '\n';
(result2 != std::end(v))
? std::cout << "v contains " << n2 << '\n'
: std::cout << "v does not contain " << n2 << '\n';
(result3 != std::end(v))
? std::cout << "v contains an even number: " << *result3 << '\n'
: std::cout << "v does not contain even numbers\n";
}
|