难点:
对int类型的容器遍历
1、for_each(vi.begin(), vi.end(), [=](const int &midint){cout << midint << endl;});
2、for_each(v1.begin(), v1.end(), [=](const int a){cout << a << endl;});
对_stu_类对象类型的容器遍历
for_each(s1.begin(), s1.end(), [=](const stu &node){cout << node.num << node.name << endl;});
sort排序函数中的参数
class MYSORT
{
public:
bool operator()(int a,int b)
{
return a<b;
}
};
bool myccc(int a,int b)
{
return a<b;
}
sort(v1.begin(), v1.end(), myccc);
main.cpp
#include <iostream>
#include<cstring>
#include<vector>
#include<algorithm>
using namespace std;
class stu
{
public:
int num;
string name;
public:
stu()
{
this->num = 0;
this->name = new char[1024]();
}
stu(int num , string name):name(name)
{
this->num = num;
}
};
void print(stu node)
{
cout << node.num <<" " << node.name << endl;
}
void play(int i)
{
cout << i << endl;
}
class myplay1
{
public:
bool operator()(int a, int b)
{
return a > b;
}
};
class MYSORT
{
public:
bool operator()(int a,int b)
{
return a<b;
}
};
bool myccc(int a,int b)
{
return a<b;
}
bool mysort(stu a, stu b)
{
return a.num > b.num;
}
class mysortclass
{
public:
bool operator()(stu a, stu b)
{
return a.num > b.num;
}
};
int main()
{
vector<int> v1;
cout << v1.size() << endl;
cout << v1.capacity() << endl;
v1.push_back(5);
v1.push_back(9);
v1.push_back(4);
v1.push_back(3);
cout << v1.front() << endl;
cout << v1.back() << endl;
putchar('\n');
cout<<"......................................"<<endl;
cout <<"对数字从小到大排序 :" << endl;
cout <<"原数字 :" << endl;
for_each(v1.begin(), v1.end(), play);
putchar('\n');
cout <<"排序后(方法2) :" << endl;
sort(v1.begin(), v1.end(), myccc);
for_each(v1.begin(), v1.end(), [=](const int a){cout << a << endl;});
cout<<"......................................"<<endl;
putchar('\n');
cout << *(v1.begin()) << endl;
cout << *(v1.end() - 1) << endl;
putchar('\n');
vector<int>::iterator pstrat = v1.begin();
vector<int>::const_iterator pend = v1.end();
cout<<"元素个数 :" << v1.size() << " ;" << "容器容量大小 :"<< v1.capacity() << endl;
for( ; pstrat != pend ;)
{
cout << *pstrat << endl;
pstrat++;
}
putchar('\n');
cout <<"验证-删除一个顶端元素 :"<<endl;
v1.pop_back();
cout<<"元素个数 :" << v1.size() << " ;" << "容器容量大小 :"<< v1.capacity() << endl;
pstrat = v1.begin();
pend = v1.end();
for( ; pstrat != pend ;)
{
cout << *pstrat << endl;
pstrat++;
}
putchar('\n');
cout <<"验证-在2号位置插入 1 个新元素 :"<<endl;
v1.insert(v1.begin() + 2, 1, 666);
cout<<"元素个数 :" << v1.size() << " ;" << "容器容量大小 :"<< v1.capacity() << endl;
pstrat = v1.begin();
pend = v1.end();
for( ; pstrat != pend ;)
{
cout << *pstrat << endl;
pstrat++;
}
putchar('\n');
cout <<"验证-rbegin-rend-反向输出容器内容 :"<<endl;
vector<int>::reverse_iterator ptstart1 = v1.rbegin();
vector<int>::reverse_iterator pend1 = v1.rend();
cout << *ptstart1 << endl;
cout << *(++ptstart1) << endl;
cout << *(pend1) << endl;
cout << *(--pend1) << endl;
putchar('\n');
cout <<"二、类容器 :"<<endl;
vector<stu> s1;
s1.push_back(stu(43, "上三"));
s1.push_back(stu(24, "里斯"));
s1.push_back(stu(15, "王武"));
s1.push_back(stu(36, "霸邪"));
cout<<"元素个数 :" << s1.size() << " ;" << "容器容量大小 :"<< s1.capacity() << endl;
vector<stu>::iterator pstart2 = s1.begin();
vector<stu>::iterator pend2 = s1.end();
cout << "resize->容器容量大小 :"<< s1.capacity() << endl;
for(; pstart2 != pend2; )
{
cout << pstart2->num <<" " << pstart2->name << endl;
pstart2++;
}
putchar('\n');
cout<<"......................................"<<endl;
cout << "重新分配大小(创建了的空间,旧有空间被释放:):" <<endl;
s1.resize(8);
pstart2 = s1.begin();
pend2 = s1.end();
cout << "for循环-遍历vector容器内容:" <<endl;
for(; pstart2 != pend2; )
{
cout << pstart2->num <<" " << pstart2->name << endl;
pstart2++;
}
cout<<"元素个数 :" << s1.size() << " ;" << "容器容量大小 :"<< s1.capacity() << endl;
cout<<"......................................"<<endl;
putchar('\n');
cout << "for_each遍历vector容器内容(回调函数方式输出):" <<endl;
for_each(s1.begin(), s1.end(), print);
putchar('\n');
cout << "for_each遍历vector容器内容([=]直接输出):" <<endl;
for_each(s1.begin(), s1.end(), [=](const stu &node){cout << node.num << node.name << endl;});
putchar('\n');
cout <<"学号有序排序 :" << endl;
sort(s1.begin(), s1.end(), mysortclass());
for_each(s1.begin(), s1.end(), [=](const stu &node){cout << node.num << node.name << endl;});
putchar('\n');
cout <<"插入一个新的学号,学号有序排序 :" << endl;
s1.insert(s1.begin()+1, 1, stu(99, "共舞"));
sort(s1.begin(), s1.end(), mysortclass());
for_each(s1.begin(), s1.end(), [=](const stu &node){cout << node.num << node.name << endl;});
putchar('\n');
cout<<"API 接口的vector的5种方式:" << endl;
vector<int> vstu;
vstu.push_back(2);
vstu.push_back(4);
vstu.push_back(22);
vstu.push_back(5);
vector<int > vi(vstu.begin(), vstu.begin() +2);
for_each(vi.begin(), vi.end(), [=](const int &midint){cout << midint << endl;});
return 0;
}
图示:
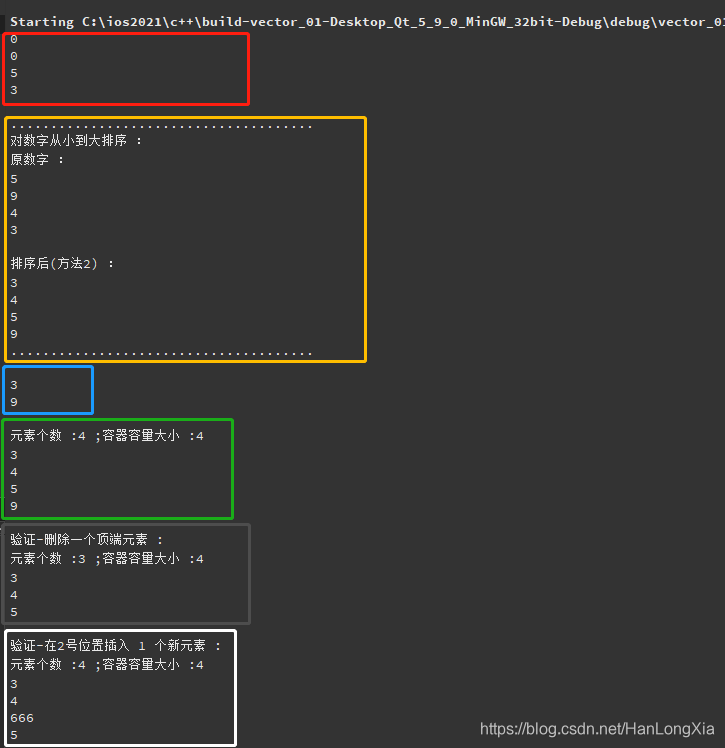 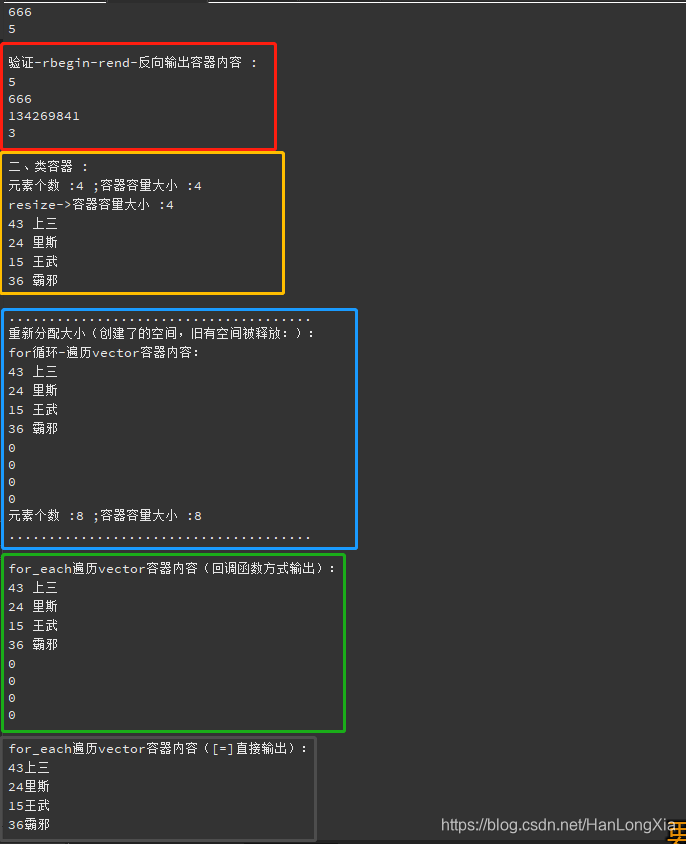
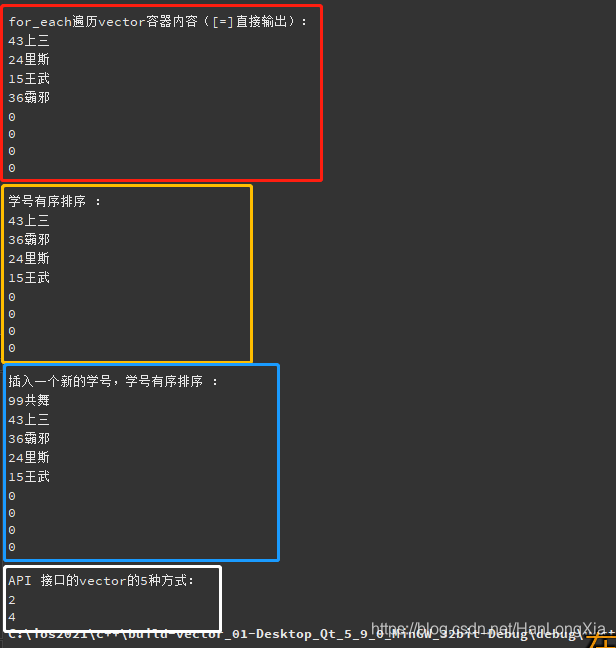
|