#include <iostream>
#include <iomanip>
using namespace std;
int main(void)
{
cout << "float : " << sizeof(float) << endl;
cout << "double : " << sizeof(double) << endl;
cout << "int : " << sizeof(int) << endl;
cout << "short : " << sizeof(short) << endl;
cout << "char : " << sizeof(char) << endl;
cout << "unsigned : " << sizeof(unsigned) << endl;
cout << "long : " << sizeof(long) << endl;
cout << endl;
cout << "float + float = float" << endl;
float a1 = 1.2f, a2 = 2.3f;
cout << "sizeof : " << sizeof(a1 + a2) << endl;
cout << "char + char = int" << endl;
char b1 = 'a', b2 = '\x1';
cout << "sizeof : " << sizeof(b1 + b2) << endl;
cout << "short + short = int" << endl;
short c1 = 12, c2 = 34;
cout << "sizeof : " << sizeof(c1 + c2) << endl;
cout << "char + int = int" << endl;
char d1 = 'b'; int d2 = -1;
cout << "sizeof : " << sizeof(d1 + d2) << endl;
cout << "short + int = int" << endl;
short e1 = 2; int e2 = 1;
cout << "sizeof : " << sizeof(e1 + e2) << endl;
cout << "int + unsigned = unsigned" << endl;
int f1 = 1; unsigned f2 = -2;
cout << "1 + -2 = " << f1 + f2 << endl;
cout << "unsigned + long = unsigned" << endl;
unsigned g1 = -2; long g2 = 1;
cout << "-2 + 1 = " << g1 + g2 << endl;
cout << "long + float = float" << endl;
long h1 = 1; float h2 = 0.1f;
cout << "1 + 0.1 = " << h1 + h2 << endl;
cout << "float + double = double" << endl;
float i1 = 0.2f; double i2 = 0.4;
cout << "sizeof : " << sizeof(i1 + i2) << endl;
return 0;
}
运行结果: 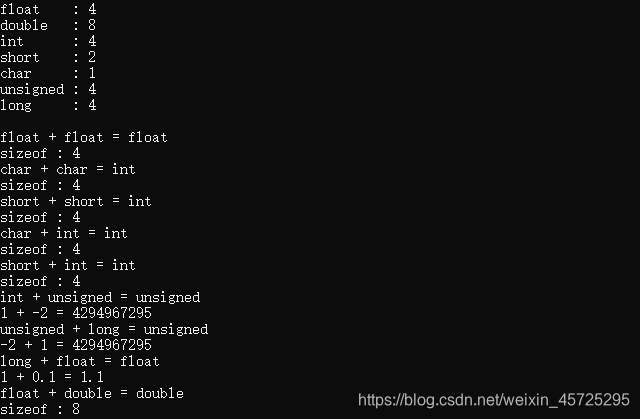
|