扫雷(无🚩)—— C语言实现
1 主要功能
- 初始化雷区
- 打印雷区
- 布置雷区
- 排查雷区(递归展开)
- 计时器
2 代码展示
2.1 头文件
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <windows.h>
#define ROW 9
#define COL 9
#define EASY_COUNT 20
#define ROWS ROW+2
#define COLS COL+2
void Init_Board(char board[ROWS][COLS], int rows, int cols, char set);
void Display_Board(char board[ROWS][COLS], int row, int col);
void Set_Mine(char board[ROWS][COLS], int row, int col);
void Remove_Mine(char mine[ROWS][COLS], char show[ROWS][COLS], int row, int col);
void expand(char mine[ROWS][COLS], char show[ROWS][COLS], int x_coordinate
2.2 执行文件
#include "game.h"
2.2.1 菜单函数
void menu()
{
printf("*********************************\n");
printf("*********************************\n");
printf("***** 1.PLAY 0.EXIT ******\n");
printf("*********************************\n");
printf("*********************************\n");
}
2.2.2 游戏执行函数
void game()
{
char mine[ROWS][COLS] = { 0 };
char show[ROWS][COLS] = { 0 };
Init_Board(mine, ROWS, COLS, '0');
Init_Board(show, ROWS, COLS, '*');
Set_Mine(mine, ROW, COL);
Display_Board(show, ROW, COL);
Remove_Mine(mine, show, ROW, COL);
}
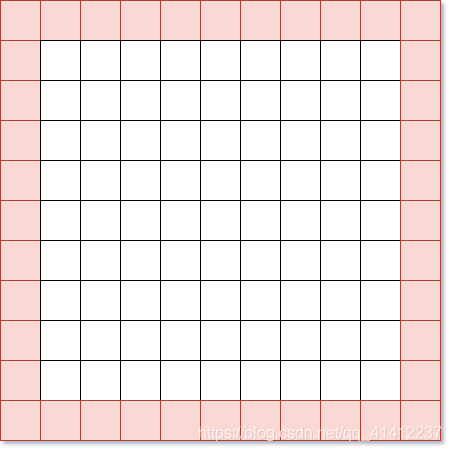
2.2.3 主函数
int main()
{
int input = 0;
srand((unsigned int)time(NULL));
do
{
system("cls");
menu();
printf("Make your choice:>");
scanf("%d", &input);
switch (input)
{
case 1:
game();
break;
case 0:
printf("Game Over...");
break;
default:
break;
}
} while (input);
return 0;
}
2.3 主函数文件
#include "game.h"
2.3.1 初始化雷区
void Init_Board(char board[ROWS][COLS], int rows, int cols, char set)
{
int i = 0;
for (size_t i = 0; i < rows; i++)
{
int j = 0;
for (size_t j = 0; j < cols; j++)
{
board[i][j] = set;
}
}
}
2.3.2 打印雷区
void Display_Board(char board[ROWS][COLS], int row, int col)
{
int i = 0;
printf("-------------------\n");
for (i = 0; i <= col; i++)
{
printf("%d ", i);
}
printf("|");
printf("\n");
for (size_t i = 1; i <= row; i++)
{
int j = 0;
printf("%d ", i);
for (size_t j = 1; j <= col; j++)
{
printf("%c ", board[i][j]);
}
printf("|");
printf("\n");
}
printf("-------------------\n");
}
2.3.3 布置雷区
void Set_Mine(char board[ROWS][COLS], int row, int col)
{
int count = EASY_COUNT;
while (count)
{
int x_coordinates = rand() % row + 1;
int y_coordinates = rand() % col + 1;
if (board[x_coordinates][y_coordinates] != '1')
{
board[x_coordinates][y_coordinates] = '1';
count--;
}
}
}
2.3.4 排查雷区
2.3.4.1 排雷主函数
void Remove_Mine(char mine[ROWS][COLS], char show[ROWS][COLS], int row, int col)
{
int x_coordinates = 0;
int y_coordinates = 0;
int win = 0;
int show_count = 0;
while (1)
{
printf("Input coordinates:>");
scanf("%d %d", &x_coordinates, &y_coordinates);
if (x_coordinates >= 1 && x_coordinates <= row && y_coordinates >= 1 && y_coordinates <= col)
{
if (mine[x_coordinates][y_coordinates] == '1')
{
printf("Dead!\n");
set_time();
printf("Game Over!\n");
Display_Board(mine, ROW, COL);
printf("Please waiting...");
Sleep(10000);
break;
}
else
{
int count = Get_Mine_Count(mine, x_coordinates, y_coordinates);
show[x_coordinates][y_coordinates] = count + '0';
expand(mine, show, x_coordinates, y_coordinates);
show_count = count_show(show);
if (show_count == EASY_COUNT)
{
printf("You win!\n");
set_time();
Display_Board(show, ROW, COL);
printf("Please waiting...");
Sleep(10000);
break;
}
system("cls");
printf("Playing...\n");
Display_Board(show, ROW, COL);
}
}
else
{
printf("Coordinates illegal!\n");
}
}
}
2.3.4.2 获取周围雷区数量
int Get_Mine_Count(char mine[ROWS][COLS], int x_coordinates, int y_coordinates)
{
return (
mine[x_coordinates - 1][y_coordinates] +
mine[x_coordinates - 1][y_coordinates - 1] +
mine[x_coordinates][y_coordinates - 1] +
mine[x_coordinates + 1][y_coordinates - 1] +
mine[x_coordinates + 1][y_coordinates] +
mine[x_coordinates + 1][y_coordinates + 1] +
mine[x_coordinates][y_coordinates + 1] +
mine[x_coordinates - 1][y_coordinates + 1] - 8 * '0');
}
2.3.4.3 计算使用时间
void set_time()
{
printf("Take %u seconds.\n", clock() / CLOCKS_PER_SEC);
}
2.3.4.4 递归展开
void expand(char mine[ROWS][COLS], char show[ROWS][COLS], int x_coordinates, int y_coordinates)
{
int count = Get_Mine_Count(mine, x_coordinates, y_coordinates);
if (count == 0)
{
show[x_coordinates][y_coordinates] = ' ';
if (x_coordinates - 1>= 1 && x_coordinates - 1 <= ROW && y_coordinates >= 1 && y_coordinates <= COL && show[x_coordinates - 1][y_coordinates] == '*')
expand(mine, show, x_coordinates - 1, y_coordinates);
if (x_coordinates + 1 >= 1 && x_coordinates + 1 <= ROW && y_coordinates >= 1 && y_coordinates <= COL && show[x_coordinates + 1][y_coordinates] == '*')
expand(mine, show, x_coordinates + 1, y_coordinates);
if (x_coordinates >= 1 && x_coordinates <= ROW && y_coordinates + 1 >= 1 && y_coordinates + 1<= COL && show[x_coordinates][y_coordinates + 1] == '*')
expand(mine, show, x_coordinates, y_coordinates + 1);
if (x_coordinates >= 1 && x_coordinates <= ROW && y_coordinates - 1 >= 1 && y_coordinates - 1<= COL && show[x_coordinates][y_coordinates] - 1 == '*')
expand(mine, show, x_coordinates, y_coordinates - 1);
}
else
{
show[x_coordinates][y_coordinates] = count + '0';
}
}
2.3.4.5 计算展示数组未排雷部分
int count_show(char show[ROWS][COLS])
{
int row = 0;
int col = 0;
int count = 0;
for (size_t row = 1; row < 10; row++)
{
for (size_t col = 1; col < 10; col++)
{
if (show[row][col] == '*')
{
count++;
}
}
}
return count;
}
2.4 效果展示
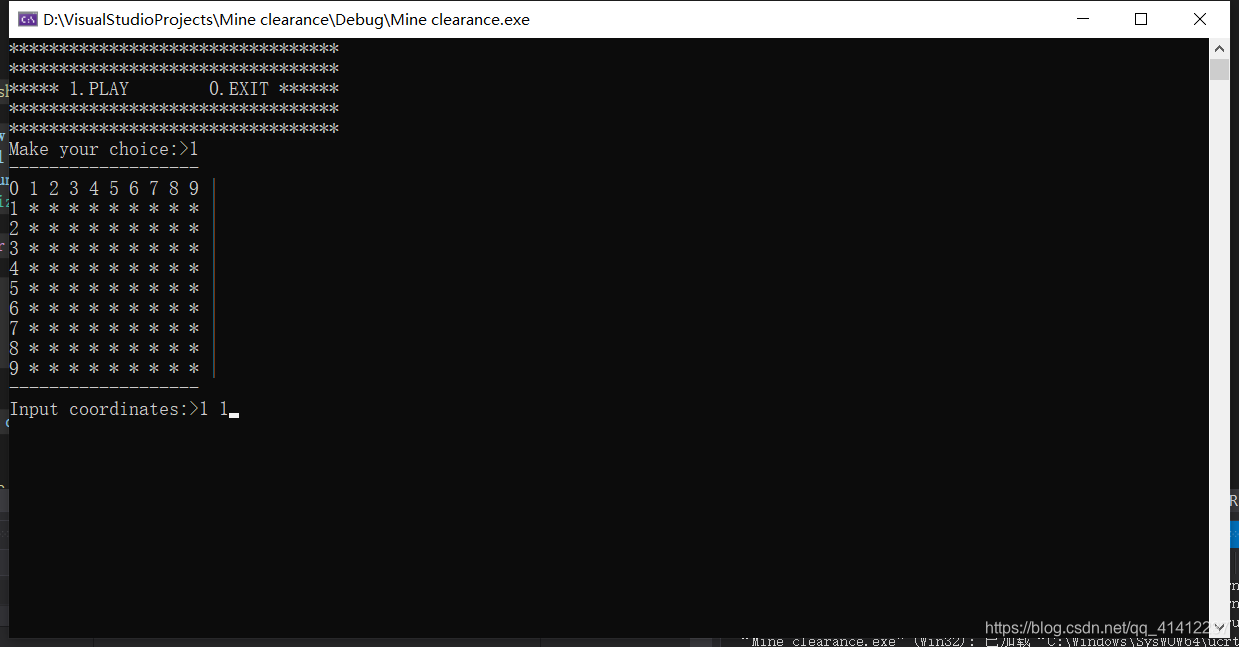 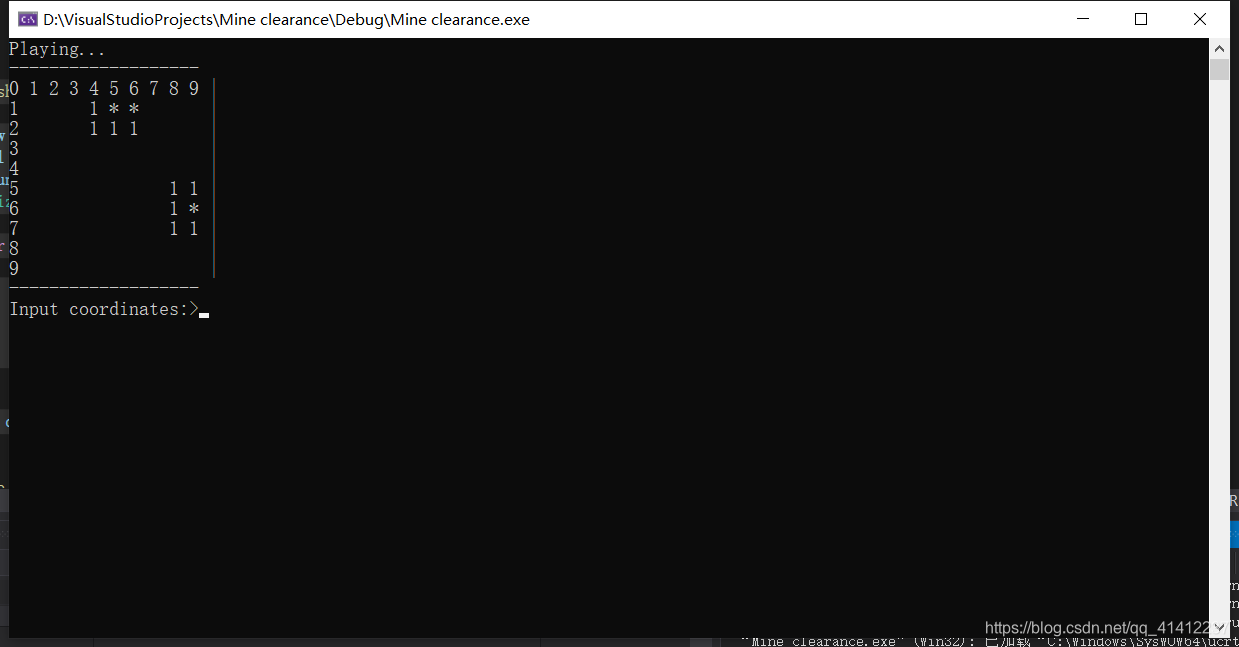 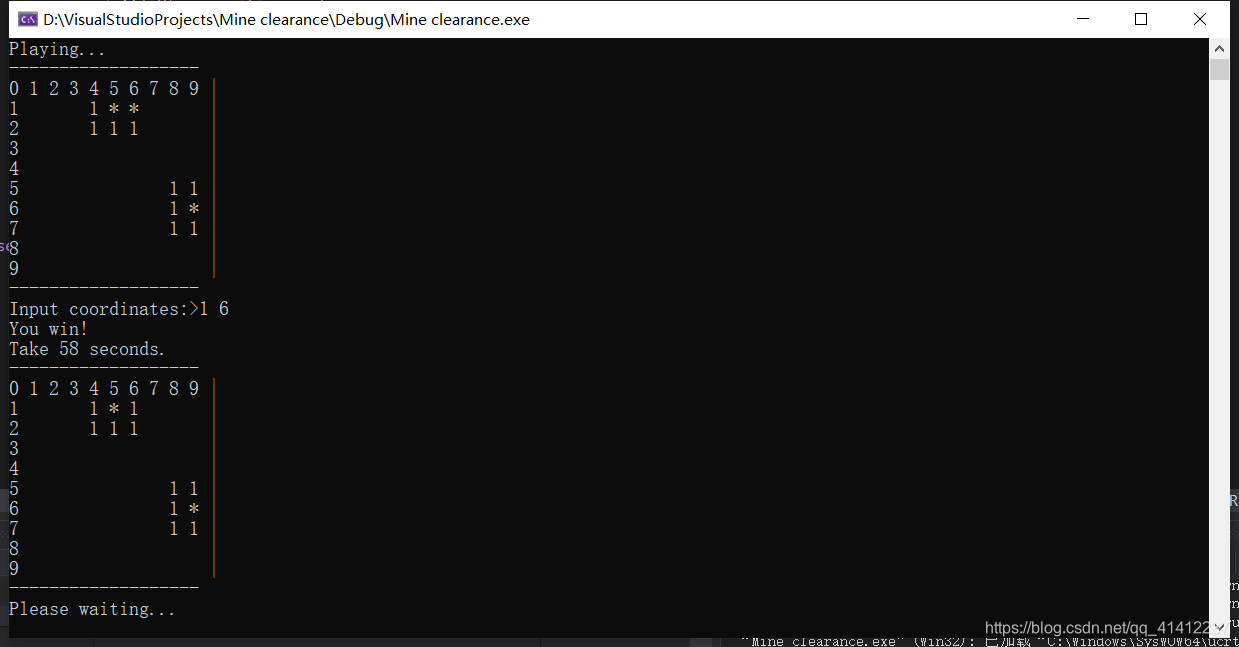
END
|