#if __cplusplus < 201103L
#error This file requires compiler and library support for the \
ISO C++ 2011 standard. This support is currently experimental, and must be \
enabled with the -std=c++11 or -std=gnu++11 compiler options.
#endif
#include <iostream>
#include <ctime>
#include <thread>
#include <algorithm>
using namespace std;
int NOT_THREAD = (980);
const int maxn = 1000000 + 7;
int A[maxn], B[maxn];
void thread_merge_sort(int L, int R) {
if(L >= R) return;
if(R - L == 1) {
if(A[L] > A[R]) swap(A[L], A[R]);
}else {
int mid = (L + R) >> 1;
if(R - L >= NOT_THREAD) {
thread Lth(thread_merge_sort, L, mid);
thread_merge_sort(mid + 1, R);
Lth.join();
}else {
thread_merge_sort(L, mid);
thread_merge_sort(mid+1, R);
}
int l = L, r = mid+1, m = L;
while(l <= mid && r <= R) {
if(A[l] <= A[r]) B[m ++] = A[l ++];
else B[m ++] = A[r ++];
}
while(l <= mid) B[m ++] = A[l ++];
while(r <= R ) B[m ++] = A[r ++];
for(int i = L; i <= R; i ++) A[i] = B[i];
}
}
int rand(int L, int R) {
int RND = rand();
#ifdef _WIN64
RND = (rand() << 15) | RND;
#endif
#ifdef _WIN32
RND = (rand() << 15) | RND;
#endif
return RND % (R - L + 1) + L;
}
class timer {
private:
long long time_begin;
public:
timer(bool auto_begin = true) {
if(auto_begin) {
time_begin = clock();
}
}
void begin() {
time_begin = clock();
}
double time_now() {
return (clock() - time_begin)/(CLOCKS_PER_SEC * 1.0);
}
};
void Check(bool use_threads = true) {
printf(use_threads ? "=> Threads\t[ available ]\n" : "=> Threads\t[unavailable]\n");
printf(
#ifdef _WIN64
"-> _WIN64\t[ availalbe ]\n"
#endif
#ifdef _WIN32
"-> _WIN32\t[ available ]\n"
#endif
"-> default\t[ available ]\n"
);
ios::sync_with_stdio(false);
int n = 1000000;
for(int i = 1; i <= n; i ++) A[i] = rand(1, 1000000000);
if(!use_threads) NOT_THREAD = 0x7fffffff;
timer Timer;
thread_merge_sort(1, n);
printf("=> n = %d, Timer: %.3lf sec\n\n\n", n, Timer.time_now());
}
int main() {
Check( true);
Check(false);
return 0;
}
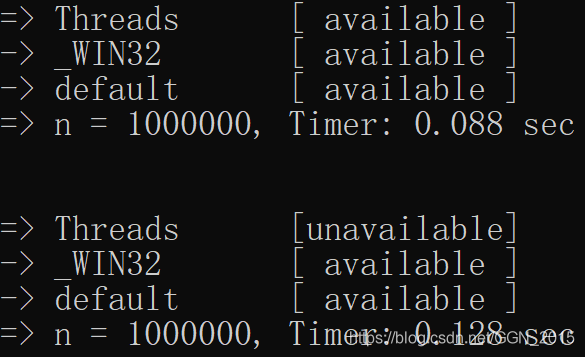
可以看到,多线程归并排序比直接使用归并排序快了
31.25
%
31.25\%
31.25%(因 CPU 而异)。
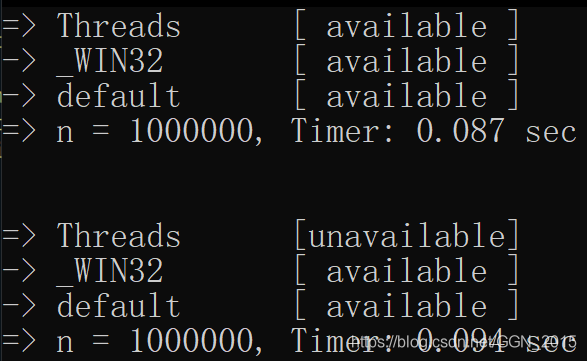
开启 -O2 优化前后,多线程归并排序算法的运行效率提升并不显著,但是普通归并排序的效果有显著提升。
|