Given a set of
N
(
>
1
)
N (>1)
N(>1) positive integers, you are supposed to partition them into two disjoint sets
A
1
A_1
A1? and
A
2
A_2
A2? of
n
1
n_1
n1? and
n
2
n_2
n2? numbers, respectively. Let
S
1
S_1
S1? and
S
2
S_2
S2? denote the sums of all the numbers in
A
1
A_1
A1? and
A
2
A_2
A2?, respectively. You are supposed to make the partition so that
∣
n
1
?
n
2
∣
∣n_1?n_2∣
∣n1??n2?∣ is minimized first, and then
∣
S
1
?
S
2
∣
∣S_1 ?S_2∣
∣S1??S2?∣ is maximized.
Input Specification:
Each input file contains one test case. For each case, the first line gives an integer
N
(
2
≤
N
≤
1
0
5
)
N (2≤N≤10^5)
N(2≤N≤105), and then
N
N
N positive integers follow in the next line, separated by spaces. It is guaranteed that all the integers and their sum are less than
2
31
2^{31}
231.
Output Specification:
For each case, print in a line two numbers:
∣
n
1
?
n
2
∣
∣n_1?n_2∣
∣n1??n2?∣ and
∣
S
1
?
S
2
∣
∣S_1?S_2∣
∣S1??S2?∣, separated by exactly one space.
Sample Input 1:
10
23 8 10 99 46 2333 46 1 666 555
Sample Output 1:
0 3611
Sample Input 2:
13
110 79 218 69 3721 100 29 135 2 6 13 5188 85
Sample Output 2:
1 9359
Solution:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main(){
int n;
cin >> n;
vector<int> nums(n);
for(int i = 0; i < n; ++i) cin >> nums[i];
sort(nums.begin(), nums.end());
int s1 = 0, s2 = 0;
for(int i = 0; i < n; ++i){
if(i < n / 2) s1 += nums[i];
else s2 += nums[i];
}
printf("%d %d\n", n % 2, s2 - s1);
return 0;
}
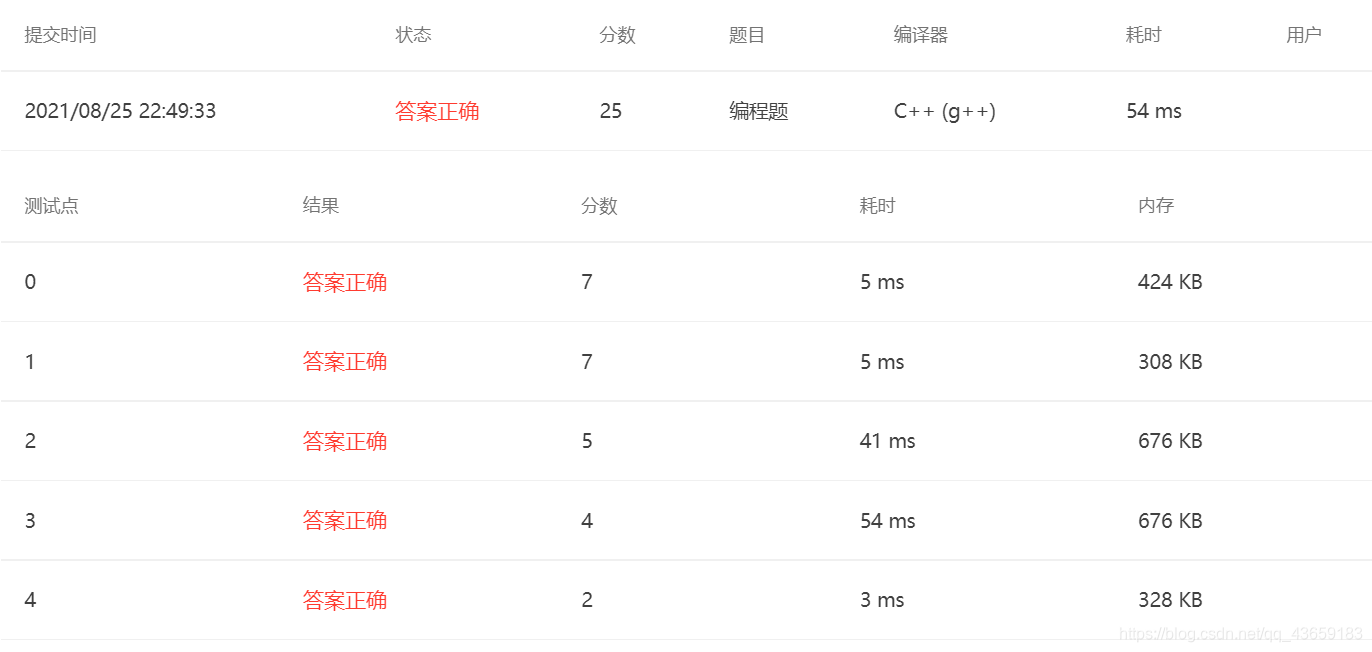
|