项目链接
项目链接:https://github.com/gaobin4216/openGL_bigWorks 如果觉得有用可以给个小小的star! 项目效果动画展示:https://www.bilibili.com/video/BV17U4y1J7Lv/ 一键三连也可以,谢谢了,给您拜个早年!
说明
西工大2021年春季学期《opengl计算机图形学》课程大作业,附源码和设计说明书&&附加本科时期的一次opengl的大作业,仅供参考 有什么疑惑可以多看看注释和文档,做的时候也参考了不少博客的内容
前言
课程作业,要求如下: 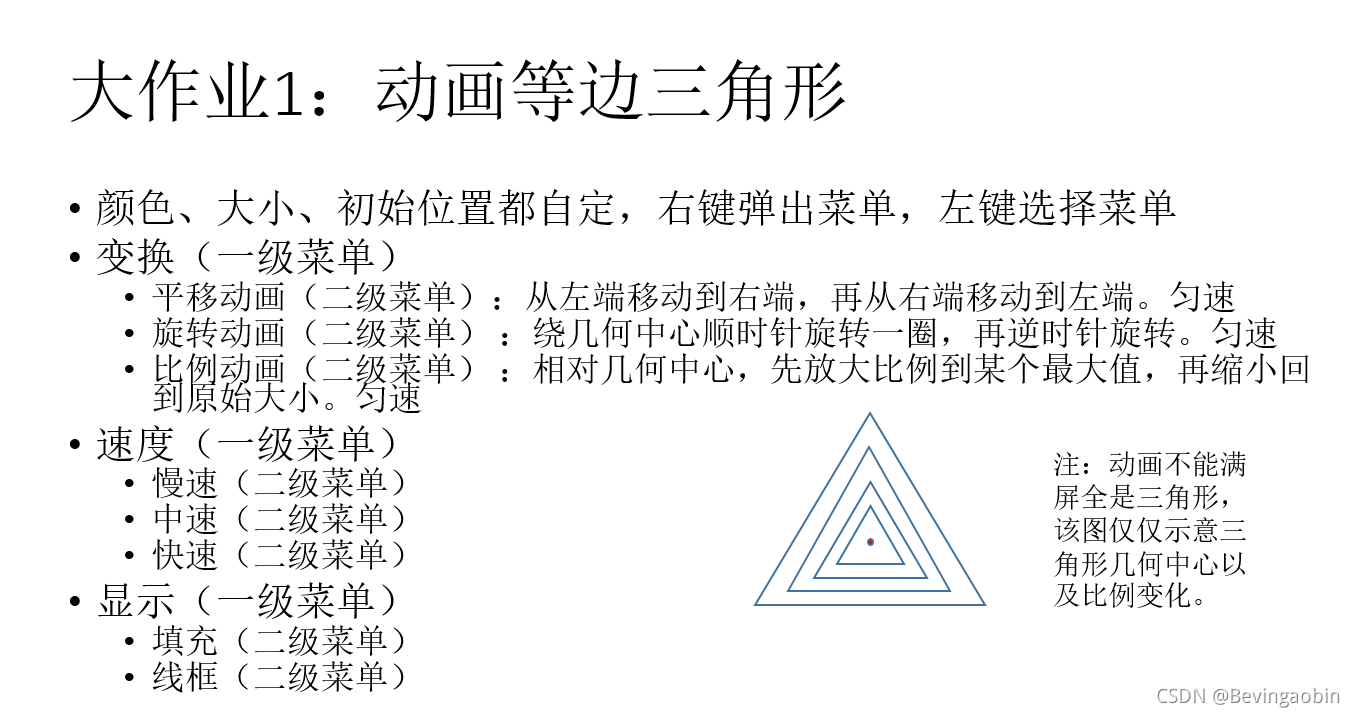 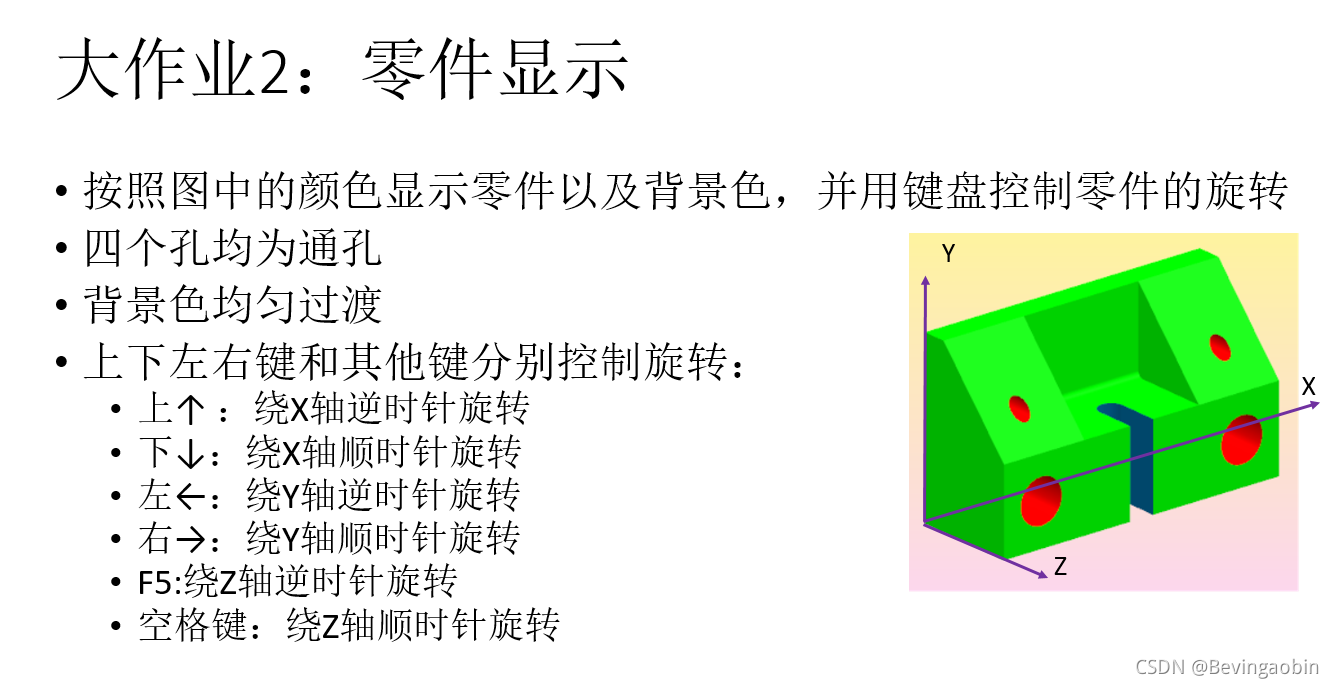
大作业一代码(需配置C++的freeglut库)
#include<Windows.h>
#include<iostream>
#include<gl/GL.h>
#include<gl/glut.h>
#include<gl/freeglut.h>
#include<cmath>
#define PI 3.1415926
static int option_value = 1;
static GLfloat move = 0.0;
static GLfloat spin = 0.0;
static GLfloat size = 1.0;
static double sumT_Count = 0;
static double sumR_Count = 0;
static double sumS_Count = 1;
static double speedlevel = 1;
static double LINE_or_FILL = 0;
void gaobin_Triangle(void)
{
glClearColor(0.0, 0.5, 1.0, 1.0);
glClear(GL_COLOR_BUFFER_BIT);
glPointSize(10.0);
glBegin(GL_POINTS);
glColor3d(1.0, 0.0, 0.0);
glVertex2d(0, 0);
glEnd();
glEnable(GL_POINT_SMOOTH);
if(LINE_or_FILL==0) glPolygonMode(GL_BACK, GL_LINE);
else if(LINE_or_FILL==1)glPolygonMode(GL_BACK, GL_FILL);
glLineWidth(4.0f);
glBegin(GL_TRIANGLES);
glColor3d(0.0, 1.0, 0.0);
glVertex2d(0.25*cos(0), 0.25*sin(0));
glVertex2d(0.25*cos(240 * PI / 180), 0.25*sin(240 * PI / 180));
glVertex2d(0.25*cos(120 * PI / 180), 0.25*sin(120 * PI / 180));
glEnd();
if (move != 0)
{
sumT_Count += move * speedlevel;
if (sumT_Count <= 1)
{
glTranslatef(GLfloat(move * speedlevel), 0, 0);
}
if (sumT_Count >= 1 && sumT_Count < 3)
{
glTranslatef(GLfloat(-move * speedlevel), 0, 0);
}
if (sumT_Count >= 3 && sumT_Count < 4)
{
glTranslatef(GLfloat(move* speedlevel), 0, 0);
}
if (sumT_Count >= 4)
{
move = 0;
}
}
if (spin != 0)
{
sumR_Count += spin * speedlevel;
if (sumR_Count <= 360)
{
glRotatef(GLfloat(-spin * speedlevel), 0, 0, 1);
}
if (sumR_Count > 360 && sumR_Count <= 720)
{
glRotatef(GLfloat(spin* speedlevel), 0, 0, 1);
}
if (sumR_Count > 720)
{
spin = 0;
}
}
if (size != 1)
{
int times = 0;
if (speedlevel == 1)times = 15;
else if (speedlevel == 3)times = 5;
else if(speedlevel == 5)times = 3;
if (sumS_Count < times)
{
glScalef(GLfloat(pow(size,speedlevel)), GLfloat(pow(size, speedlevel)), 1);
sumS_Count +=1;
Sleep(250);
}
if (sumS_Count>=times && sumS_Count <2*times)
{
glScalef(GLfloat(pow(1/size, speedlevel)), GLfloat(pow(1/size, speedlevel)),1);
sumS_Count +=1;
Sleep(250);
}
if (sumS_Count >=2*times)
{
size = 1;
}
}
glutPostRedisplay();
glutSwapBuffers();
}
void TransformMenu(int date)
{
option_value = date;
switch (option_value)
{
case 1:
sumT_Count = 0;
spin = 0;
size = 1;
move = 0.005f ;
break;
case 2:
sumR_Count = 0;
move = 0;
size = 1;
spin = 0.5f;
break;
case 3:
sumS_Count = 0;
move = 0;
spin = 0;
size = 1.08f;
break;
}
}
void VelocityMenu(int date)
{
option_value = date;
switch (option_value)
{
case 4:
speedlevel = 1;
break;
case 5:
speedlevel = 3;
break;
case 6:
speedlevel = 5;
break;
}
}
void DisplayMenu(int date)
{
option_value = date;
switch (option_value)
{
case 7:
LINE_or_FILL = 1;
break;
case 8:
LINE_or_FILL = 0;
break;
}
}
void MianMenu(int data)
{
option_value = data;
glutPostRedisplay();
}
int main(int argc, char* argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB );
glutInitWindowPosition(400, 150);
glutInitWindowSize(800,800);
glutCreateWindow("高斌_大作业1");
int gaobin_TransformMenu = glutCreateMenu(TransformMenu);
glutAddMenuEntry("Translation_Animation", 1);
glutAddMenuEntry("Rotatation_Animation", 2);
glutAddMenuEntry("Proportion_Animation", 3);
int gaobin_VelocityMenu = glutCreateMenu(VelocityMenu);
glutAddMenuEntry("Low_Speed", 4);
glutAddMenuEntry("Medium_Speed", 5);
glutAddMenuEntry("Fast_Speed", 6);
int gaobin_DisplayMenu = glutCreateMenu(DisplayMenu);
glutAddMenuEntry("Padding", 7);
glutAddMenuEntry("Wireframe", 8);
int gaobin_MainMenu = glutCreateMenu(MianMenu);
glutAddSubMenu("TransformMenu", gaobin_TransformMenu);
glutAddSubMenu("VelocityMenu", gaobin_VelocityMenu);
glutAddSubMenu("DisplayMenu", gaobin_DisplayMenu);
glutAttachMenu(GLUT_RIGHT_BUTTON);
glutDisplayFunc(&gaobin_Triangle);
glutMainLoop();
return 0;
}
大作业二代码(需配置C++的freeglut库)
#include<Windows.h>
#include<vector>
#include<iostream>
#include<string>
#include<fstream>
#include<sstream>
#include<gl/GL.h>
#include<gl/glut.h>
#include<gl/freeglut.h>
#include<cmath>
#define PI 3.1415926
GLfloat lightPos1[] = { 0.28f,0.23f,0.3f,1.0f };
GLfloat lightDirection1[] = { 0.0,-1.0,-1.0 };
GLfloat lightPos2[] = { 0.0f,0.5f,0.30f,1.0f };
GLfloat lightDirection2[] = { 1.0,-1.0,-1.0 };
GLfloat lightPos3[] = { -0.5f,-0.0f,0.1f,1.0f };
GLfloat lightDirection3[] = { 1.0,0.0,0.0 };
GLfloat specular[] = { 1.0f,1.0f,1.0f,1.0f };
GLfloat specrof[] = { 1.0f,1.0f,1.0f,1.0f };
GLfloat ambientLight_whole[] = { 1.0f,1.0f,1.0f,1.0f };
GLfloat ambientLight[] = { 0.2f,0.2f,0.2f,1.0f };
static GLfloat xRot = 0.0f;
static GLfloat yRot = 0.0f;
static GLfloat zRot = 0.0f;
class ObjLoader
{
public:
struct vertex
{
float x;
float y;
float z;
};
ObjLoader(std::string filename);
void Draw();
private:
std::vector<std::vector<GLfloat>> v;
std::vector<std::vector<GLint>> f;
};
ObjLoader::ObjLoader(std::string filename)
{
std::ifstream file(filename);
std::string line;
while (getline(file, line))
{
if (line.substr(0, 1) == "v")
{
std::vector<GLfloat> Point;
GLfloat x, y, z;
std::istringstream s(line.substr(2));
s >> x;
s >> y;
s >> z;
Point.push_back(x);
Point.push_back(y);
Point.push_back(z);
v.push_back(Point);
}
else if (line.substr(0, 1) == "f")
{
std::vector<GLint> vIndexSets;
GLint u, v, w;
std::istringstream vtns(line.substr(2));
vtns >> u; vtns >> v; vtns >> w;
vIndexSets.push_back(u - 1);
vIndexSets.push_back(v - 1);
vIndexSets.push_back(w - 1);
f.push_back(vIndexSets);
}
}
file.close();
}
void ObjLoader::Draw()
{
glBegin(GL_TRIANGLES);
for (int i = 0; i < f.size(); i++)
{
vertex a, b, c;
if ((f[i]).size() != 3)
{
std::cout << "ERRER::THE SIZE OF f IS NOT 3!" << std::endl;
}
else {
GLint firstVertexIndex = (f[i])[0];
GLint secondVertexIndex = (f[i])[1];
GLint thirdVertexIndex = (f[i])[2];
a.x = (v[firstVertexIndex])[0];
a.y = (v[firstVertexIndex])[1];
a.z = (v[firstVertexIndex])[2];
b.x = (v[secondVertexIndex])[0];
b.y = (v[secondVertexIndex])[1];
b.z = (v[secondVertexIndex])[2];
c.x = (v[thirdVertexIndex])[0];
c.y = (v[thirdVertexIndex])[1];
c.z = (v[thirdVertexIndex])[2];
if ((a.x >= 0.03&&a.x <= 0.12&&a.y>= 0.03&&a.y<= 0.12)
&&
(b.x >= 0.03&&b.x <= 0.12&&b.y >= 0.03&&b.y<= 0.12)
&&
(c.x >= 0.03&&c.x <= 0.12&&c.y >= 0.03&&c.y <= 0.12))
{
glColor3f(1.0, 0.0, 0.0);
}
else if ((a.x >= 0.43&&a.x <= 0.51&&a.y >= 0.03&&a.y <= 0.12)
&&
(b.x >= 0.43&&b.x <= 0.51&&b.y >= 0.03&&b.y <= 0.12)
&&
(c.x >= 0.43&&c.x <= 0.51&&c.y >= 0.03&&c.y <= 0.12))
{
glColor3f(1.0, 0.0, 0.0);
}
else if ((a.x >= 0.065&&a.x <= 0.095&&a.y >= 0.205&&a.y <= 0.245)
&&
(b.x >= 0.065&&b.x <= 0.095&&b.y >= 0.205&&b.y <= 0.245)
&&
(c.x >= 0.065&&c.x <= 0.095&&c.y >= 0.205&&c.y <= 0.245))
{
glColor3f(1.0, 0.0, 0.0);
}
else if ((a.x >= 0.455&&a.x <= 0.485&&a.y >= 0.205&&a.y <= 0.245)
&&
(b.x >= 0.455&&b.x <= 0.485&&b.y >= 0.205&&b.y <= 0.245)
&&
(c.x >= 0.455&&c.x <= 0.485&&c.y >= 0.205&&c.y <= 0.245))
{
glColor3f(1.0, 0.0, 0.0);
}
else if ((a.x >= 0.455&&a.x <= 0.495&&a.y >= 0.205&&a.y <= 0.245)
&&
(b.x >= 0.455&&b.x <= 0.495&&b.y >= 0.205&&b.y <= 0.245)
&&
(c.x >= 0.455&&c.x <= 0.495&&c.y >= 0.205&&c.y <= 0.245))
{
glColor3f(1.0, 0.0, 0.0);
}
else if ((a.x >= 0.25&&a.x <= 0.30&&a.z >= 0.1&&a.z<= 0.3)
&&
(b.x >= 0.25&&b.x <= 0.30&&b.z >= 0.1 && b.z <= 0.3)
&&
(c.x >= 0.25&&c.x <= 0.30&&c.z >= 0.1 && c.z <= 0.3))
{
glColor3f(0.0, 0.0, 1.0);
}
else glColor3f(0.0, 1.0, 0.0);
glVertex3f(a.x*1.5, a.y*1.5, -a.z*1.5);
glVertex3f(b.x*1.5, b.y*1.5, -b.z*1.5);
glVertex3f(c.x*1.5, c.y*1.5, -c.z*1.5);
}
}
glEnd();
}
ObjLoader monkey = ObjLoader("openglOBJ.obj");
void gaobin_Object(void)
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glBegin(GL_POLYGON);
glColor3f(1.0f, 0.84f, 0.84f);
glVertex3f(-1.0f, -1.0f, 0.6f);
glVertex3f(1.0f, -1.0f,0.6f);
glColor3f(1.0f, 0.76f, 0.45f);
glVertex3f(1.0f, 1.0f, 0.6f);
glVertex3f(-1.0f, 1.0f, 0.6f);
glEnd();
glLoadIdentity();
glMatrixMode(GL_MODELVIEW);
glPushMatrix();
glRotatef(xRot, 1.0f, 0.0f, 0.0f);
glRotatef(yRot, 0.0f, 1.0f, 0.0f);
glRotatef(zRot, 0.0f, 0.0f, 1.0f);
monkey.Draw();
glPopMatrix();
glutPostRedisplay();
glutSwapBuffers();
}
void KeyboardKeys(unsigned char key, int x, int y)
{
if (key == 32)
zRot -= 5.0f;
}
void SpecialKeys(int key, int x, int y)
{
if (key == GLUT_KEY_UP)
xRot -= 5.0f;
if (key == GLUT_KEY_DOWN)
xRot += 5.0f;
if (key == GLUT_KEY_LEFT)
yRot += 5.0f;
if (key == GLUT_KEY_RIGHT)
yRot -= 5.0f;
if (key == GLUT_KEY_F5)
zRot += 5.0f;
glutPostRedisplay();
glutSwapBuffers();
}
void SetupRC(void)
{
glEnable(GL_DEPTH_TEST);
glEnable(GL_LIGHTING);
glLightModelfv(GL_LIGHT_MODEL_AMBIENT, ambientLight_whole);
glLightfv(GL_LIGHT0, GL_DIFFUSE, ambientLight);
glLightfv(GL_LIGHT0, GL_SPECULAR, specular);
glLightfv(GL_LIGHT0, GL_POSITION, lightPos1);
glLightfv(GL_LIGHT0, GL_SPOT_DIRECTION, lightDirection1);
glLightf(GL_LIGHT0, GL_SPOT_CUTOFF,180.0f);
glLightf(GL_LIGHT0, GL_SPOT_EXPONENT,500.0f);
glEnable(GL_LIGHT0);
glLightfv(GL_LIGHT1, GL_DIFFUSE, ambientLight);
glLightfv(GL_LIGHT1, GL_SPECULAR, specular);
glLightfv(GL_LIGHT1, GL_POSITION, lightPos2);
glLightfv(GL_LIGHT1, GL_SPOT_DIRECTION, lightDirection2);
glLightf(GL_LIGHT1, GL_SPOT_CUTOFF, 180.0f);
glLightf(GL_LIGHT1, GL_SPOT_EXPONENT, 500.0f);
glEnable(GL_LIGHT1);
glLightfv(GL_LIGHT2, GL_DIFFUSE, ambientLight);
glLightfv(GL_LIGHT2, GL_SPECULAR, specular);
glLightfv(GL_LIGHT2, GL_POSITION, lightPos3);
glLightfv(GL_LIGHT2, GL_SPOT_DIRECTION, lightDirection3);
glLightf(GL_LIGHT2, GL_SPOT_CUTOFF, 60.0f);
glLightf(GL_LIGHT2, GL_SPOT_EXPONENT, 500.0f);
glEnable(GL_LIGHT2);
glEnable(GL_COLOR_MATERIAL);
glColorMaterial(GL_FRONT, GL_AMBIENT_AND_DIFFUSE);
glMaterialfv(GL_FRONT, GL_SPECULAR, specrof);
glMateriali(GL_FRONT, GL_SHININESS, 128);
glDepthFunc(GL_LEQUAL);
}
int main(int argc, char* argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowPosition(400, 150);
glutInitWindowSize(800, 800);
glutCreateWindow("高斌_大作业2");
glutSpecialFunc(SpecialKeys);
glutKeyboardFunc(KeyboardKeys);
SetupRC();
glutDisplayFunc(&gaobin_Object);
glutMainLoop();
return 0;
}
|