#include <graphics.h>
#include <stdio.h>
#include <conio.h>
#include <tchar.h>
#include <Windows.h>
#include <stdlib.h>
#include <time.h>
void gameDraw();
void loadImg();
void gameInit();
void playerMove(int speed);
void createBullet();
void CreateEnemyPlane();
void EnemyMove(int speed);
void BulletMove(int speed);
void enemyHP(int i);
bool Timer(int ms, int id);
enum My
{
WIDTH = 480,
HEIGHT = 700,
BULLET_NUM = 15,
ENEMY_PLANE_NUM = 10,
BIG,
SMAIL
};
struct Plane
{
int x;
int y;
bool live;
int width;
int height;
int hp;
int type;
}player, bull[BULLET_NUM], Eplane[ENEMY_PLANE_NUM];
void gameInit()
{
loadImg();
player.x = WIDTH / 2;
player.y = HEIGHT - 120;
player.live = true;
for (int i = 0; i < BULLET_NUM; i++)
{
bull[i].x = 0;
bull[i].y = 0;
bull[i].live = false;
}
for (int i = 0; i < ENEMY_PLANE_NUM; i++)
{
Eplane[i].live = false;
enemyHP(i);
}
}
void playerMove(int speed)
{
if (GetAsyncKeyState(VK_UP))
{
if (player.y > 0)
{
player.y -= speed;
}
}
if (GetAsyncKeyState(VK_DOWN))
{
if (player.y + 100 < HEIGHT)
{
player.y += speed;
}
}
if (GetAsyncKeyState(VK_LEFT))
{
if (player.x + 40 > 0)
{
player.x -= speed;
}
}
if (GetAsyncKeyState(VK_RIGHT))
{
if (player.x + 70 < WIDTH)
{
player.x += speed;
}
}
if (GetAsyncKeyState(VK_SPACE) && Timer(200, 1))
{
createBullet();
}
}
void CreateEnemyPlane()
{
for (int i = 0; i < ENEMY_PLANE_NUM; i++)
{
if (!Eplane[i].live)
{
Eplane[i].live = true;
Eplane[i].x = rand() % WIDTH;
Eplane[i].y = 0;
enemyHP(i);
break;
}
}
}
void EnemyMove(int speed)
{
for (int i = 0; i < ENEMY_PLANE_NUM; i++)
{
if (Eplane[i].live)
{
Eplane[i].y += speed;
if (Eplane[i].y > HEIGHT)
{
Eplane[i].live = false;
}
}
}
}
void enemyHP(int i)
{
if (rand() % 10 == 0 || rand() % 10 == 5)
{
Eplane[i].type = BIG;
Eplane[i].hp = 3;
Eplane[i].width = 104;
Eplane[i].height = 148;
}
else
{
Eplane[i].type = SMAIL;
Eplane[i].hp = 1;
Eplane[i].width = 49;
Eplane[i].height = 40;
}
}
void createBullet()
{
for (int i = 0; i < BULLET_NUM; i++)
{
if (!bull[i].live)
{
bull[i].x = player.x + 50;
bull[i].y = player.y;
bull[i].live = true;
break;
}
}
}
void BulletMove(int speed)
{
for (int i = 0; i < BULLET_NUM; i++)
{
if (bull[i].live)
{
bull[i].y -= speed;
if (bull[i].y < 0)
{
bull[i].live = false;
}
}
}
}
IMAGE bk;
IMAGE img_role[2];
IMAGE bullet[2];
IMAGE Enemy_pic[2][2];
void loadImg()
{
loadimage(&bk, L"C:/Users/LiYan/Desktop/c/c++/飞机大战素材包/images/background.jpg");
loadimage(&img_role[0], L"./飞机大战素材包/images/planeNormal_1.jpg");
loadimage(&img_role[1], L"./飞机大战素材包/images/planeNormal_2.jpg");
loadimage(&bullet[0], L"./飞机大战素材包/images/bullet1.jpg");
loadimage(&bullet[1], L"./飞机大战素材包/images/bullet2.jpg");
loadimage(&Enemy_pic[0][0], L"./飞机大战素材包/images/enemyPlane1.jpg");
loadimage(&Enemy_pic[0][1], L"./飞机大战素材包/images/enemyPlane2.jpg");
loadimage(&Enemy_pic[1][0], L"./飞机大战素材包/images/enemyPlane3.jpg");
loadimage(&Enemy_pic[1][1], L"./飞机大战素材包/images/enemyPlane4.jpg");
}
void gameDraw()
{
putimage(0, 0, &bk);
putimage(player.x, player.y, &img_role[0], NOTSRCERASE);
putimage(player.x, player.y, &img_role[1], SRCINVERT);
for (int i = 0; i < BULLET_NUM; i++)
{
if (bull[i].live)
{
putimage(bull[i].x, bull[i].y, &bullet[0], NOTSRCERASE);
putimage(bull[i].x, bull[i].y, &bullet[1], SRCINVERT);
}
}
for (int i = 0; i < ENEMY_PLANE_NUM; i++)
{
if (Eplane[i].live)
{
if (Eplane[i].type == BIG)
{
putimage(Eplane[i].x, Eplane[i].y, &Enemy_pic[0][0], NOTSRCERASE);
putimage(Eplane[i].x, Eplane[i].y, &Enemy_pic[0][1], SRCINVERT);
}
else
{
putimage(Eplane[i].x, Eplane[i].y, &Enemy_pic[1][0], NOTSRCERASE);
putimage(Eplane[i].x, Eplane[i].y, &Enemy_pic[1][1], SRCINVERT);
}
}
}
}
bool Timer(int ms,int id)
{
static DWORD t[10];
if (clock() - t[id] > ms)
{
t[id] = clock();
return true;
}
return false;
}
void Collide()
{
for (int i = 0; i < ENEMY_PLANE_NUM; i++)
{
if (!Eplane[i].live)
continue;
for (int k = 0; k < BULLET_NUM; k++)
{
if (!bull[k].live)
continue;
if (bull[k].x > Eplane[i].x && bull[k].x < Eplane[i].x + Eplane[i].width
&& bull[k].y > Eplane[i].y && bull[k].y < Eplane[i].y + Eplane[i].height)
{
bull[k].live = false;
Eplane[i].hp--;
}
}
if (Eplane[i].hp <= 0)
{
Eplane[i].live = false;
}
}
}
int main()
{
initgraph(WIDTH, HEIGHT);
gameInit();
BeginBatchDraw();
while (1)
{
gameDraw();
FlushBatchDraw();
playerMove(1);
BulletMove(1);
if (Timer(500,0))
{
CreateEnemyPlane();
}
if (Timer(10, 2))
{
EnemyMove(1);
}
Collide();
}
EndBatchDraw();
return 0;
}
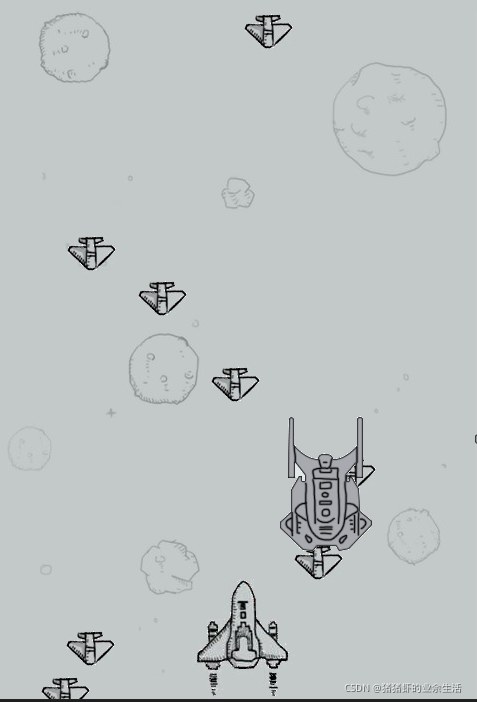
|